Site Search for Headless site run on Vercel
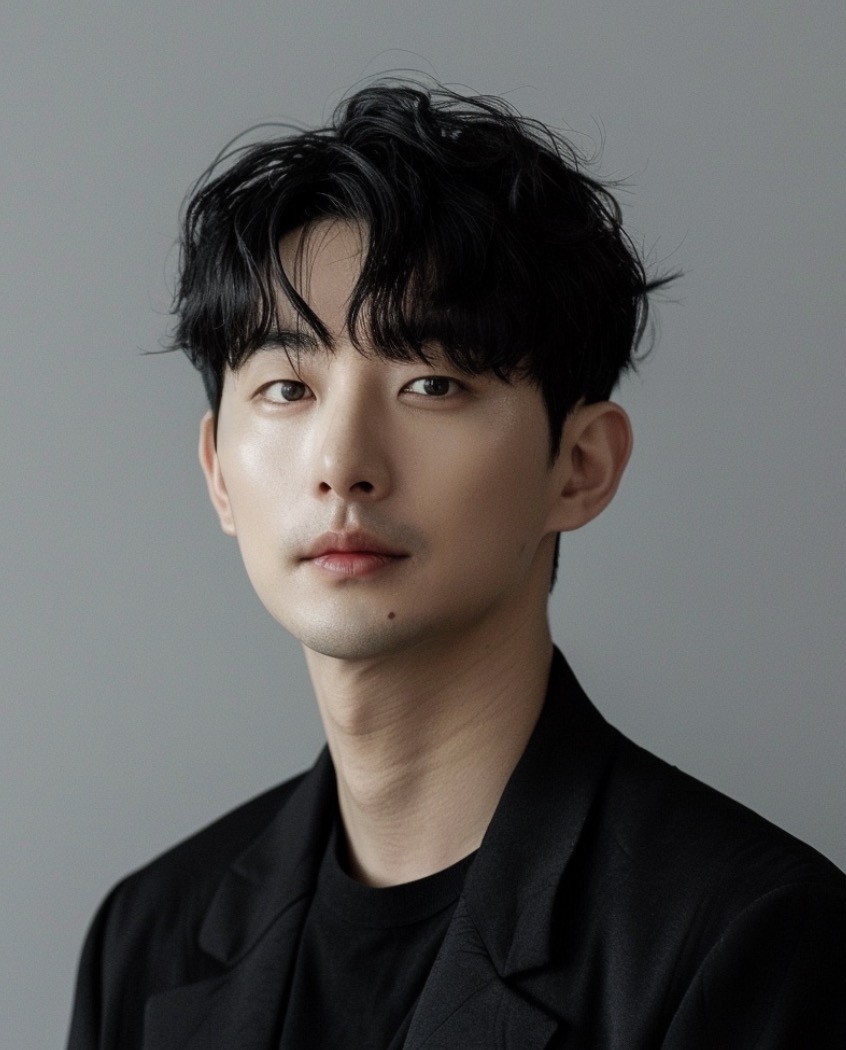
Table of contents
- 1. Algolia
- 2. Elasticsearch
- 3. Lunr.js
- 4. Firebase
- 5. Custom Solution with Backend Search Service
- Choosing the Right Solution
- Implementation Example: Algolia
- Github Repo
- 1. Set Up Meilisearch
- 2. Indexing Your Data
- 3. Front-End Integration
- 4. Customization and Optimization
- 5. Deployment
- Example Repositories
- Additional Resources
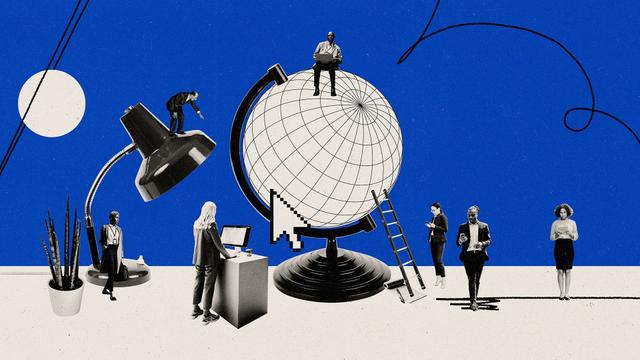
Implementing an on-site search functionality on your website hosted on Vercel with a headless stack involves several potential solutions. Here are some common and effective approaches:
1. Algolia
Algolia is a powerful hosted search engine that provides a robust, scalable search solution. It offers fast and relevant search results with a highly customizable API.
Setup Algolia:
Create an Algolia account and set up an application.
Add your data to Algolia indices.
Install Algolia's JavaScript library (e.g., InstantSearch.js) on your website.
Configure the frontend to connect to your Algolia indices and display search results.
Pros:
Extremely fast and highly scalable.
Advanced features like typo tolerance, filtering, and faceting.
Easy to integrate with a wide range of frameworks.
Cons:
- Can become expensive as your usage scales.
2. Elasticsearch
Elasticsearch is an open-source, distributed search and analytics engine. It's highly customizable and can handle complex queries.
Setup Elasticsearch:
Deploy an Elasticsearch instance (can be self-hosted or use a service like Elastic Cloud).
Index your data into Elasticsearch.
Use a client library (e.g., elasticsearch-js for JavaScript) to query Elasticsearch from your website.
Pros:
Powerful and flexible search capabilities.
Can handle large datasets and complex search queries.
Cons:
Requires more setup and maintenance compared to hosted solutions.
Might be overkill for smaller projects.
3. Lunr.js
Lunr.js is a lightweight, client-side search library. It indexes content in the browser and performs searches without any server-side components.
Setup Lunr.js:
Install Lunr.js via npm or include it via a CDN.
Index your content using Lunr.js on the client side.
Implement a search input that queries the Lunr.js index and displays results.
Pros:
No server setup required.
Ideal for smaller datasets and static sites.
Easy to integrate and use.
Cons:
Not suitable for large datasets due to performance limitations in the browser.
Limited to basic search functionalities.
4. Firebase
Firebase offers a hosted NoSQL database and various other services. You can use Firebase to store your search data and perform queries.
Setup Firebase:
Create a Firebase project and set up Firestore.
Add your data to Firestore.
Use Firebase's client SDK to query Firestore from your website.
Pros:
Easy to set up and use with real-time updates.
Scalable and integrates well with other Firebase services.
Cons:
May require additional configuration to achieve complex search functionalities.
Cost can increase with high usage.
5. Custom Solution with Backend Search Service
You can create a custom search solution by building a backend service to handle search queries. This involves using a backend framework (e.g., Node.js with Express) and a database that supports full-text search (e.g., PostgreSQL, MongoDB).
Setup Custom Solution:
Set up a backend server (Node.js/Express) on Vercel or another hosting service.
Choose a database with full-text search capabilities and set it up.
Index your data in the database.
Create API endpoints to handle search queries.
Implement the frontend to call your search API and display results.
Pros:
Fully customizable to meet specific requirements.
Can leverage existing backend infrastructure.
Cons:
Requires more development and maintenance effort.
Might not be as performant as specialized search solutions.
Choosing the Right Solution
The best solution depends on your specific needs, such as the size of your dataset, the complexity of your search requirements, and your budget. For a simple, cost-effective solution, Lunr.js or Firebase might be suitable. For more advanced needs, consider Algolia or Elasticsearch. If you need full control over the search functionality and already have a backend in place, a custom solution might be the way to go.
Implementation Example: Algolia
Here's a brief implementation example using Algolia with a Next.js front end:
Install Algolia Libraries:
npm install algoliasearch instantsearch.js
Configure Algolia Client:
// lib/algolia.js import algoliasearch from 'algoliasearch/lite'; const searchClient = algoliasearch('YourApplicationID', 'YourSearchOnlyAPIKey'); export const algoliaIndex = searchClient.initIndex('your_index_name');
Create Search Component:
// components/Search.js import { InstantSearch, SearchBox, Hits } from 'react-instantsearch-dom'; import { algoliaIndex } from '../lib/algolia'; const Search = ( ) => ( <InstantSearch searchClient={algoliaIndex} indexName="your_index_name"> <SearchBox /> <Hits hitComponent={Hit} /> </InstantSearch> ); const Hit = ({ hit }) => ( <div> <h4>{hit.title}</h4> <p>{hit.description}</p> </div> ); export default Search;
Include Search Component in Your Page:
// pages/index.js import Search from '../components/Search'; const HomePage = ( ) => ( <div> <h1>My Website</h1> <Search /> </div> ); export default HomePage;
This is a basic setup, and you can further customize the search experience with more advanced features provided by Algolia or other chosen solutions.
Github Repo
To implement an on-site search for your Vercel-hosted website using a headless stack, one of the most popular and effective solutions is utilizing the open-source repository "Meilisearch." Meilisearch is a powerful, fast, and easy-to-use search engine that can be integrated seamlessly with various front-end frameworks.
Here are the steps and considerations for implementing Meilisearch:
1. Set Up Meilisearch
- Deploy Meilisearch: You can deploy Meilisearch on your own server or use a hosted solution. Vercel can integrate well with a serverless function that interfaces with Meilisearch.
2. Indexing Your Data
Data Preparation: Ensure your data is properly formatted for indexing. Meilisearch accepts JSON objects, and each object represents a document in your index.
Index Creation: Use the Meilisearch dashboard or API to create an index and upload your documents.
3. Front-End Integration
API Client: Use the Meilisearch JavaScript client to interact with the search API. You can install it via npm:
bash
Copy code
npm install meilisearch
Search Implementation: Add the search functionality to your website by integrating the Meilisearch client with your front-end framework. This involves setting up a search input, handling API requests, and displaying results.
4. Customization and Optimization
Search Relevance: Customize the search relevance settings such as ranking rules, synonyms, and stop-words to improve search accuracy and relevance.
UI/UX Enhancements: Consider using autocomplete, instant search results, and filters to enhance user experience.
5. Deployment
- Continuous Deployment: Integrate your Meilisearch setup into your Vercel deployment pipeline to ensure that your search index is updated with each deployment if your data changes frequently.
Example Repositories
Meilisearch: Meilisearch GitHub Repository
InstantSearch.js: For front-end components, you can look into Algolia’s InstantSearch.js, which can be adapted to work with Meilisearch.
Additional Resources
Documentation: Refer to the Meilisearch documentation for detailed setup and integration guides.
Community: Engage with the Meilisearch community on GitHub for support and updates.
Using Meilisearch provides a robust solution for on-site search, offering high performance and ease of integration suitable for modern web applications hosted on platforms like Vercel.
Subscribe to my newsletter
Read articles from Ewan Mak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
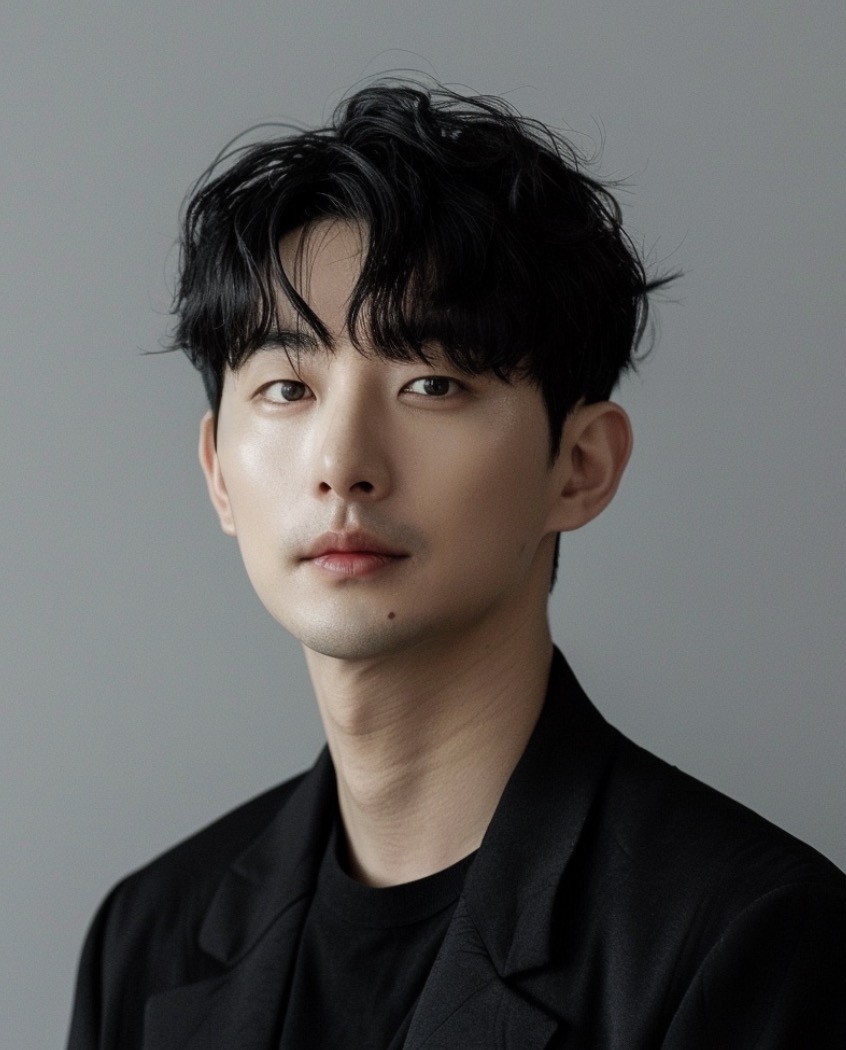
Ewan Mak
Ewan Mak
Crafting seamless user experiences with a passion for headless CMS, Vercel deployments, and Cloudflare optimization. I'm a Full Stack Developer with expertise in building modern web applications that are blazing fast, secure, and scalable. Let's connect and discuss how I can help you elevate your next project!