Python Data Visualization Interview Questions

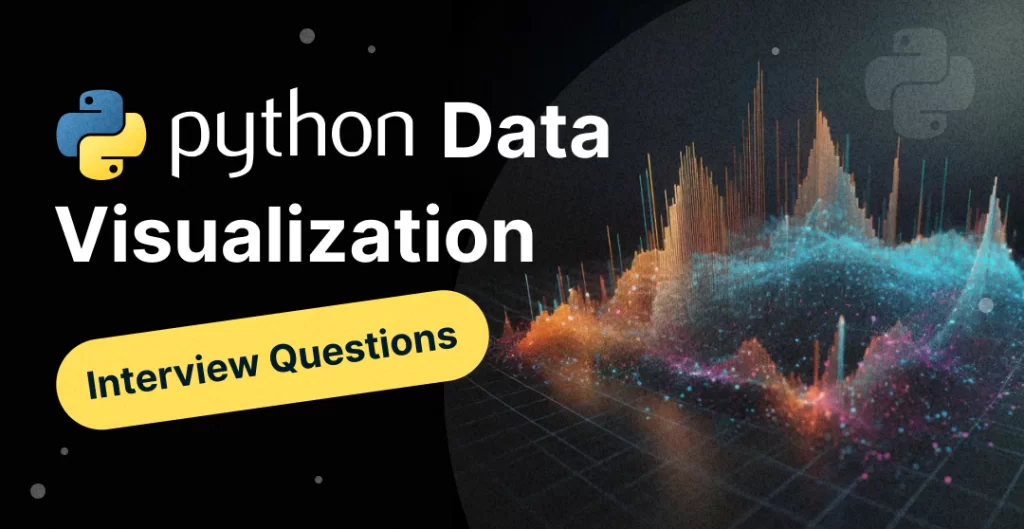
Python, known for its extensive range of powerful visualization libraries like Matplotlib, Seaborn, and Plotly, has become the go-to language for creating informative and visually compelling visualizations. Technical interviews often feature data visualization questions to evaluate a candidate’s ability to communicate data-driven insights through meaningful graphs.
This article aims to guide you through the Python data visualization interview process by exploring essential concepts, key skills, and the types of questions you can expect. We’ll cover everything from basic plots to advanced charts and real-world scenarios, providing you with practical examples and tips to refine your visualization techniques. Whether you’re a seasoned data scientist or preparing for your first technical interview, this guide will help you navigate the common challenges and highlight the best practices for presenting your data effectively.
Key Concepts and Skills in Python Data Visualization
Effective data visualization in Python requires a deep understanding of plotting fundamentals and the ability to translate complex data into clear visual stories. We explore the key concepts and skills necessary to excel in Python data visualization interviews.
Basic Plotting and customization
Data visualization often starts with basic plots like bar charts, line graphs, or scatter plots. These foundational visualizations allow you to present comparisons, trends, and distributions. For instance, a bar chart can represent product sales across different regions, while a line plot may illustrate revenue trends over time. Here’s a simple example of a bar chart using Matplotlib:
import matplotlib.pyplot as plt
regions = ['North', 'South', 'East', 'West']
sales = [350, 420, 290, 510]
plt.bar(regions, sales, color=['blue', 'green', 'red', 'purple'])
plt.xlabel('Regions')
plt.ylabel('Sales')
plt.title('Product Sales by Region')
plt.show()
In interviews, you might be asked to refine such plots by adjusting axes, adding labels, or changing color palettes. This level of customization ensures that visualizations align with the brand or are clear and intuitive.
Storytelling through data
A critical aspect of visualization is storytelling. Engineers must be able to transform raw data into a compelling visual narrative. For instance, when analyzing customer retention rates, a cohort analysis chart can reveal behavioral trends over time:
import seaborn as sns
import pandas as pd
# Simulating a dataset for demonstration purposes
data = pd.DataFrame({
'month': ['Jan', 'Feb', 'Mar', 'Apr', 'May'],
'cohort1': [100, 80, 60, 50, 40],
'cohort2': [90, 70, 65, 55, 45],
'cohort3': [85, 75, 70, 60, 50]
})
sns.lineplot(data=data, x='month', y='cohort1', label='Cohort 1')
sns.lineplot(data=data, x='month', y='cohort2', label='Cohort 2')
sns.lineplot(data=data, x='month', y='cohort3', label='Cohort 3')
plt.xlabel('Month')
plt.ylabel('Retention Rate')
plt.title('Cohort Analysis Over Time')
plt.legend()
plt.show()
Visualizing retention cohorts allows stakeholders to identify patterns and tailor customer engagement strategies.
Working with multivariate data
In interviews, you may be asked to visualize multivariate data using advanced techniques like heatmaps, pair plots, and violin plots. Heatmaps, for instance, provide a matrix view of correlations between numerical variables, helping to identify relationships at a glance. Here’s an example using Seaborn:
import seaborn as sns
# Simulating a correlation matrix
correlation_data = pd.DataFrame({
'Feature1': [1, 0.8, 0.6, 0.2],
'Feature2': [0.8, 1, 0.5, 0.3],
'Feature3': [0.6, 0.5, 1, 0.1],
'Feature4': [0.2, 0.3, 0.1, 1]
}, index=['Feature1', 'Feature2', 'Feature3', 'Feature4'])
sns.heatmap(correlation_data, annot=True, cmap='coolwarm')
plt.title('Correlation Heatmap')
plt.show()
Handling large datasets
Data engineers frequently work with large datasets, and visualizing them efficiently requires aggregation and specialized tools. Libraries like Datashader can render billions of points quickly without sacrificing quality. Aggregating data into meaningful summaries is crucial when presenting high-level insights.
Interactive visualizations
Interactive plots are becoming increasingly popular, allowing users to explore data by zooming, filtering, or adjusting parameters. Plotly and Bokeh are excellent for building interactive dashboards. In interviews, be ready to demonstrate how to handle user inputs or create responsive charts.
Advanced customization and styling
In corporate settings, consistency in visual style across different charts is essential. Engineers must be capable of applying themes, customizing annotations, and ensuring that visualizations meet specific guidelines or preferences. This involves selecting fonts, adjusting color palettes, and controlling layout settings.
Top Python Data Visualization Interview Questions
When it comes to Python data visualization interviews, questions typically focus on assessing your knowledge of visualization libraries, your ability to create clear and informative plots, and your proficiency in conveying data insights through effective storytelling. You can expect questions that range from basic plotting techniques to more advanced tasks like customizing plots, working with multivariate data, or developing interactive visualizations.
Common types of questions:
Basic plotting questions
These assess your ability to create simple plots like bar charts, line graphs, and scatter plots using libraries such as Matplotlib or Seaborn.Customization and styling
These questions evaluate your skill in adjusting axes, modifying labels, and customizing colors, grids, or annotations to improve plot clarity and align with brand guidelines.
- Advanced techniques
More advanced questions may require creating complex visualizations like violin plots, correlation heatmaps, or geographic data maps. You could also be asked to work with multivariate datasets or handle missing values.
- Interactive visualization
Questions might include building interactive dashboards or handling user inputs using Plotly or Bokeh.
- Storytelling and interpretation
Interviewers often look for your ability to interpret visualizations and explain data insights in a compelling narrative.
Example questions:
How would you create a bar chart showing product sales across different regions using Matplotlib?
Create a subplot that displays multiple line plots, each representing monthly revenue trends for different years.
Visualize a correlation matrix using Seaborn’s heatmap. Add annotations to make each value readable.
Develop an interactive plot with Plotly that allows users to filter data by a date range.
Describe the most effective way to handle missing data when creating a time series visualization.
For a complete list of questions and the opportunity to practice solutions, check out the courses at Data Engineer Academy.
The Python Data Engineer Interview course at Data Engineer Academy is specifically designed to help you practice solving real-world data visualization questions that often appear in interviews with major tech companies. Through this course, you’ll learn how to create clear and compelling visualizations, refine your storytelling skills, and practice crafting custom and interactive plots.
FAQ: Preparing for Python Data Visualization Interviews
Q: What skills do I need to master to excel in Python data visualization interviews?
A: To excel in Python data visualization interviews, you should be proficient with libraries like Matplotlib, Seaborn, and Plotly. Key skills include creating and customizing different types of plots, handling multivariate data, storytelling through visualizations, and building interactive dashboards. Understanding data storytelling and plot customization are crucial.
How To Learn $80,000 Working In Data?
Q: What types of visualization questions are commonly asked in interviews?
A: Common questions include creating basic bar charts and line plots, generating complex visualizations like correlation matrices and violin plots, and designing interactive plots. You may also be asked to analyze real-world scenarios such as time series trends or geographic data.
Q: How can I practice real-world interview questions effectively?
A: You can practice by using real datasets to replicate interview-style problems. Data Engineer Academy provides hands-on projects and mock challenges that simulate the interview environment. Working on open datasets can also offer valuable practice.
Q: What are some tips for creating clear and compelling visualizations?
A: Choose the most suitable chart type for your data, add concise titles and labels, and ensure colors and markers are distinguishable. Maintain consistency across multiple plots, avoid clutter, and always strive to tell a story that matches your data analysis.
Q: How important is storytelling in data visualization interviews?
A: Storytelling is essential because it shows how well you can interpret data and present actionable insights. Employers are looking for candidates who can build narratives that connect visual trends with business goals, making storytelling a crucial skill.
Q: How can I handle missing or inconsistent data in my visualizations?
A: Techniques like interpolation or aggregation can help fill gaps in time series data. Imputing missing values with averages or medians can also smooth inconsistencies. Be prepared to explain your approach to managing incomplete data.
Q: Is it necessary to build interactive plots for Python visualization interviews?
A: While not always required, interactive plots can showcase your advanced skills and help create more engaging data presentations. Familiarity with Plotly, Bokeh, or Dash allows you to add filtering, zooming, and other interactive features.
Takeaways
Sign up for the Python Data Engineer Interview course at Data Engineer Academy and gain access to expert-led training, practical projects, and mock interview challenges. Learn how to master data visualization, develop compelling narratives, and tackle complex interview questions with confidence. Your journey toward becoming a proficient data engineer starts here.
Subscribe to my newsletter
Read articles from Christopher Garzon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Christopher Garzon
Christopher Garzon
The goal of data engineer academy is to help people do two things. Learn the skills needed to be in data related roles and learn how to ace the interviews as well. We believe data related roles are in the early phases of their growth. And in addition to learning the skills, learning how to pass the interviews are just as important and our goal is to create clarity when it comes to both of those skillets.