Stripe Data Engineer Interview Guide

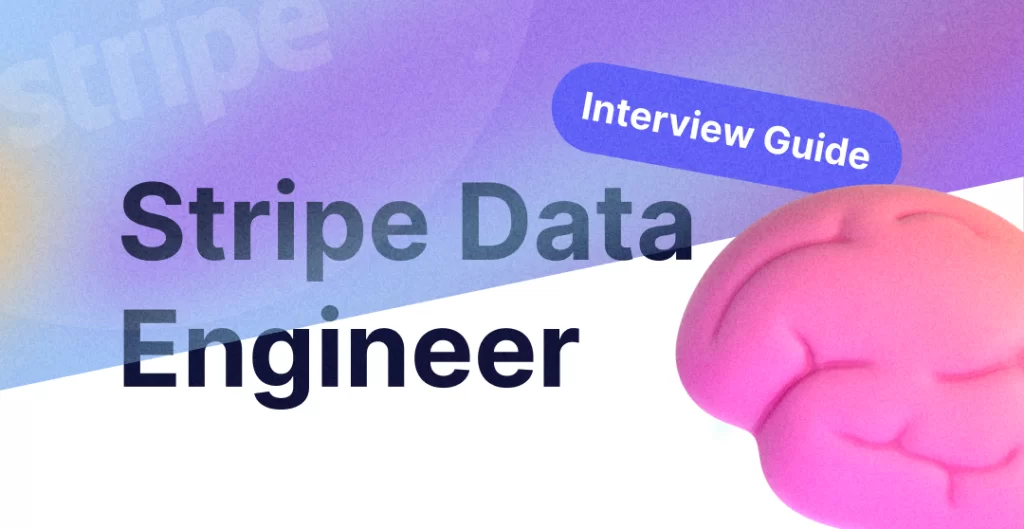
Stripe Interview Process
The interview process at Stripe is designed to assess a candidate’s technical expertise, problem-solving skills, and alignment with the company’s values. It begins with an initial screening to verify your experience and gauge your fit for the data engineering role. In this initial conversation, you can expect questions about your background in data engineering, key projects, and familiarity with the tools and technologies commonly used at Stripe.
After the initial screening, the technical assessment phase focuses on coding challenges and problem-solving exercises. Here, candidates are tested on their ability to write clean, efficient code using programming languages like Python or Java. SQL skills are also assessed through complex query-writing tasks, reflecting the real-world data transformation and manipulation scenarios encountered at Stripe.
The system design interview is next, requiring candidates to architect scalable data solutions for challenges such as designing distributed storage or real-time data pipelines. This is an opportunity to demonstrate your ability to structure comprehensive, logical designs while explaining and justifying your technical choices.
The behavioral interviews explore a candidate’s approach to teamwork and problem-solving, providing insights into their work style and cultural alignment with Stripe. You will discuss how you’ve tackled challenging projects, collaborated with teams, and adapted to changing circumstances.
Finally, the process often culminates in multiple interviews with senior engineers and managers, where your technical depth, strategic thinking, and compatibility with Stripe’s broader goals are assessed. These conversations involve deep technical dives, strategic discussions, and system design problem-solving.
Key stages of the stripe interview process:
Resume verification and preliminary fit assessment.
Coding challenges focus on data structures, algorithms, and SQL.
Architecting scalable data pipelines and distributed storage.
Understanding teamwork, adaptability, and problem-solving skills.
Comprehensive evaluation by senior engineers and managers.
This interview process is designed to assess each candidate’s qualifications, ensuring the right skills and mindset for data engineering roles at Stripe. The following sections will delve deeper into each stage, providing targeted preparation strategies for technical challenges, system design, and behavioral questions.
Stripe Interview: Technical Preparation
Technical preparation for the Stripe interview involves a solid understanding of data structures, algorithms, SQL, and system design. Let’s dive deeper into each topic, providing multiple examples and practice solutions.
Data structures and algorithms
Questions related to data structures and algorithms often focus on solving practical problems using core programming techniques. You may need to work with arrays, linked lists, hash tables, trees, or graphs. Algorithm challenges will frequently require you to implement or optimize sorting, searching, and pathfinding solutions.
Example 1: Finding the longest substring without repeating characters
“Given a string, find the length of the longest substring without repeating characters.”
Solution (Python):
def longest_unique_substring(s):
char_index = {}
max_length = start = 0
for end, char in enumerate(s):
if char in char_index and char_index[char] >= start:
start = char_index[char] + 1
char_index[char] = end
max_length = max(max_length, end - start + 1)
return max_length
# Example usage:
print(longest_unique_substring("abcabcbb")) # Output: 3 ("abc")
Example 2: Sum of two numbers
“Write a function that takes an array of integers and a target value, and returns two numbers that add up to the target.”
Solution (Python):
def two_sum(nums, target):
num_to_index = {}
for index, num in enumerate(nums):
complement = target - num
if complement in num_to_index:
return [num_to_index[complement], index]
num_to_index[num] = index
return []
# Example usage:
print(two_sum([2, 7, 11, 15], 9)) # Output: [0, 1] (indices of 2 and 7)
SQL queries
In the SQL portion, Stripe’s interview questions may involve joining tables, filtering data, writing subqueries, or implementing window functions.
Example 1: Customers who placed multiple orders
“Write a SQL query to find customers who placed more than three orders in the past year.”
Solution (SQL):
SELECT customer_id
FROM orders
WHERE order_date >= DATE_SUB(NOW(), INTERVAL 1 YEAR)
GROUP BY customer_id
HAVING COUNT(*) > 3;
Example 2: Employee salary ranks
“Write a SQL query to find the ranks of employee salaries, ordered by department.”
Solution (SQL):
SELECT department, employee_name, salary,
RANK() OVER (PARTITION BY department ORDER BY salary DESC) AS salary_rank
FROM employees;
System Design
System design challenges assess your ability to architect scalable, resilient solutions. You must analyze requirements, explain the data flow, and design a system that handles real-world constraints like high traffic and fault tolerance.
Example: Designing a data pipeline
“Design a real-time data pipeline to process payment transactions and update customer accounts.”
Key Components to Consider:
Ingestion Layer. Use Apache Kafka to collect transaction events from various sources.
Processing Layer. Implement Apache Flink or Apache Spark for real-time processing.
Storage Layer. Write processed transactions to a distributed NoSQL database (e.g., Cassandra or DynamoDB) and a data warehouse (e.g., Snowflake or BigQuery) for analysis.
Monitoring and Alerts. Set up monitoring via Prometheus or Grafana to ensure data integrity and alert on failures.
Master your technical preparation with DE Academy courses, where you can practice data structures, SQL queries, and system design challenges. Sign up to access interactive lessons and tutorials. The DE Academy Coaching Program provides personalized guidance and mock interviews to help you excel in the Stripe data engineering interview.
Mock Interviews and Practice Strategies
To establish a realistic interview setting, set a timer for coding challenges and system design problems, aiming to solve them within 30 to 45 minutes. Pick challenges reflecting common data engineering topics like algorithms, SQL queries, and system design tasks. After solving the problems, evaluate your approach and communication style by recording yourself or seeking feedback from a partner.
Coding challenges
For coding challenges, focus on improving proficiency in data structures and algorithms. You might encounter questions about finding the longest palindromic substring or writing a function to identify two numbers that add up to a target sum. To maximize your preparation:
Practice under time constraints to improve speed and accuracy.
Work with data structures like arrays, hash tables, and trees for optimized solutions.
Explain your approach out loud to mimic an interview scenario and clarify your thought process.
SQL queries
SQL problems require a solid understanding of database querying and manipulation. You may be tasked with writing queries to analyze customer transactions or rank employee salaries using window functions like RANK() or DENSE_RANK(). Use these strategies to practice:
Tackle challenges requiring complex joins, subqueries, or window functions.
Simulate real-world scenarios like aggregating sales data or filtering outliers.
Write your queries in SQL IDEs that allow immediate feedback on query results.
System design
System design problems require a comprehensive approach. For instance, if asked to design a recommendation system for an e-commerce platform, outline the architecture using scalable components like Kafka for data ingestion, Apache Flink for processing, and Cassandra for storage. Consider these elements:
Understand the core requirements and constraints of the system.
Break down the design into key components like ingestion, processing, and storage.
Explain your choice of technologies and strategies for scalability, fault tolerance, and data consistency.
Behavioral interviews
Behavioral interviews focus on your approach to teamwork and problem-solving. Prepare by using the STAR (Situation, Task, Action, Result) framework to describe past experiences:
Situation: Provide context by describing the challenge or problem.
Task: Clarify your specific role and responsibilities.
Action: Describe the steps you took to address the challenge.
Result: Highlight the measurable impact of your actions.
For instance, when asked about a time you solved a challenging technical problem, describe the problem’s context, your role in devising the solution, and how your actions positively affected the team or project.
Finding the right practice partners or coaches is vital. Partner with colleagues or join technical interview preparation forums and online communities to share resources and strategies. Alternatively, professional coaching programs can provide personalized feedback and guidance, allowing you to refine your approach and excel in Stripe’s data engineering interview.
By consistently practicing mock interviews and applying these strategies, you’ll develop stronger problem-solving skills and build the confidence necessary to tackle Stripe’s technical challenges.
FAQ: Stripe Interview Recommendations
Q: What technical skills should I focus on when preparing for the Stripe data engineering interview?
A: Emphasize your understanding of data structures, algorithms, and SQL, as they are core components of the technical assessments. Review system design concepts, practice designing scalable data pipelines, and ensure you’re comfortable with distributed systems and real-time data processing.
Q: How should I structure my answers for behavioral questions?
A: Use the STAR (Situation, Task, Action, Result) framework. Provide context for the problem, clarify your role, describe your approach to solving it, and share the measurable impact. This approach ensures concise yet detailed responses.
Q: What types of system design problems can I expect in the interview?
A: You might be asked to design a data pipeline, distributed storage system, or real-time analytics platform. Be ready to explain your architectural choices, data flow, and how you’d handle scalability, fault tolerance, and latency.
Q: How can I best demonstrate my technical expertise during coding challenges?
A: Clearly explain your thought process as you work through coding problems, focusing on clean, efficient solutions. Highlight optimizations and ensure your code is well-structured.
Q: Are there specific programming languages that Stripe prefers for coding interviews?
A: While Stripe typically supports multiple programming languages, Python and Java are frequently used. Choose a language you’re comfortable with and focus on showcasing your problem-solving skills.
Q: How can I make the best impression during the initial screening interview?
A: Be concise and articulate when describing your background and projects. Align your experience with Stripe’s requirements, and be ready to discuss the tools, technologies, and methodologies you’ve worked with in previous roles.
Q: Should I research Stripe’s products and business model before the interview?
A: Absolutely. Understanding Stripe’s core products, market focus, and recent achievements will help you tailor your responses. This knowledge shows that you’re genuinely interested in the company and can help align your answers with its goals.
Subscribe to my newsletter
Read articles from Christopher Garzon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Christopher Garzon
Christopher Garzon
The goal of data engineer academy is to help people do two things. Learn the skills needed to be in data related roles and learn how to ace the interviews as well. We believe data related roles are in the early phases of their growth. And in addition to learning the skills, learning how to pass the interviews are just as important and our goal is to create clarity when it comes to both of those skillets.