๐ Understanding Deferred Execution in LINQ ๐
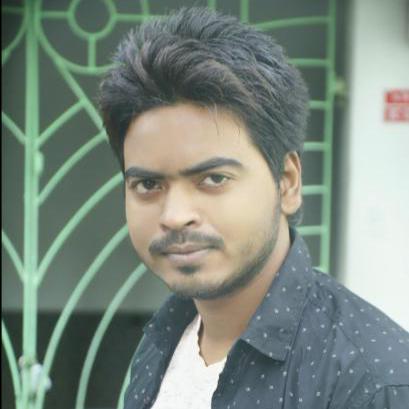
Have you ever wondered how LINQ queries can be so efficient and flexible? The secret lies in a powerful concept known as deferred execution. Let's dive into what deferred execution is and why it matters.
What is Deferred Execution? Deferred execution means that the evaluation of a LINQ query is delayed until its results are actually accessed. This allows for efficient query construction and execution, providing several benefits in terms of performance and resource management.
How It Works: When you create a LINQ query, you're essentially building a query expression. However, this expression isn't executed immediately. Instead, the query is stored as an object that can be executed later. The actual execution happens when you:
Enumerate the query using a foreach loop.
Call methods that enumerate the query, such as ToList(), ToArray(), Count(), etc.
Benefits of Deferred Execution:
Efficiency: Queries are only executed when needed, which can save resources and improve performance.
Flexibility: You can compose complex queries dynamically without immediate execution, allowing you to build and modify queries based on runtime conditions.
Resource Management: Deferred execution can reduce memory usage since the query results are not stored in memory until explicitly requested.
Example in C#:
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
// Create a LINQ query
var query = numbers.Where(n => n > 3);
// The query is not executed here
Console.WriteLine("Query created");
// Execute the query by enumerating it
foreach (var number in query)
{
Console.WriteLine(number);
}
Key Takeaway: Deferred execution is a core feature of LINQ that makes it both powerful and efficient. By understanding how it works, you can write more performant and adaptable code.
Are you leveraging deferred execution in your LINQ queries? Share your experiences or ask questions below!
Subscribe to my newsletter
Read articles from Md Asif Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
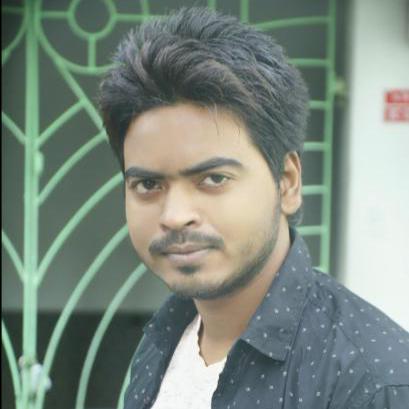
Md Asif Alam
Md Asif Alam
๐ Full Stack .NET Developer & React Enthusiast ๐จโ๐ป About Me: With 3+ years of experience, I'm passionate about crafting robust solutions and seamless user experiences through code. ๐ผ Expertise: Proficient in .NET Core API, ASP.NET MVC, React.js, and SQL. Skilled in backend architecture, RESTful APIs, and frontend development. ๐ Achievements: Led projects enhancing scalability by 50%, delivered ahead of schedule, and contributed to open-source initiatives. ๐ Future Focus: Eager to embrace new technologies and drive innovation in software development. ๐ซ Let's Connect: Open to new opportunities and collaborations. Reach me on LinkedIn or GitHub!