Comprehensive list of LINQ methods
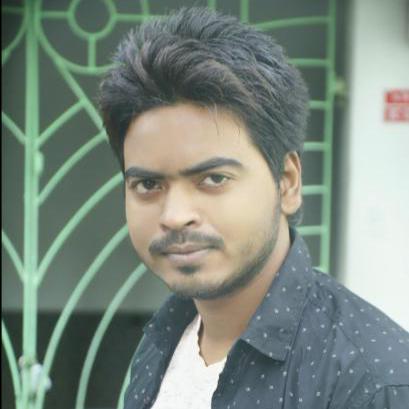
Filtering
Where Filters a sequence of values based on a predicate.
var result = collection.Where(item => item.Condition);
// Example
var result = people.Where(p => p.Age > 18);
OfType Filters the elements of an IEnumerable based on a specified type.
var result = collection.OfType<DesiredType>();
// Example
var result = mixedList.OfType<int>();
Take Returns a specified number of contiguous elements from the start of a sequence.
var result = collection.Take(count);
// Example
var result = numbers.Take(3);
Skip Bypasses a specified number of elements in a sequence and then returns the remaining elements.
var result = collection.Skip(count);
// Example
var result = numbers.Skip(3);
TakeWhile Returns elements from a sequence as long as a specified condition is true.
var result = collection.TakeWhile(item => item.Condition);
// Example
var result = numbers.TakeWhile(n => n < 5);
SkipWhile Bypasses elements in a sequence as long as a specified condition is true and then returns the remaining elements.
var result = collection.SkipWhile(item => item.Condition);
// Example
var result = numbers.SkipWhile(n => n < 5);
Projection
Select Projects each element of a sequence into a new form.
var result = collection.Select(item => new { item.Property });
// Example
var result = people.Select(p => new { p.Name, p.Age });
SelectMany Projects each element of a sequence to an IEnumerable and flattens the resulting sequences into one sequence.
var result = collection.SelectMany(item => item.CollectionProperty);
// Example
var result = companies.SelectMany(c => c.Employees);
Sorting
OrderBy Sorts the elements of a sequence in ascending order.
var result = collection.OrderBy(item => item.Property);
// Example
var result = people.OrderBy(p => p.Name);
OrderByDescending Sorts the elements of a sequence in descending order.
var result = collection.OrderByDescending(item => item.Property);
// Example
var result = people.OrderByDescending(p => p.Name);
ThenBy Performs a subsequent ordering of the elements in a sequence in ascending order.
var result = collection.OrderBy(item => item.Property).ThenBy(item => item.SecondProperty);
// Example
var result = people.OrderBy(p => p.Name).ThenBy(p => p.Age);
ThenByDescending Performs a subsequent ordering of the elements in a sequence in descending order.
var result = collection.OrderBy(item => item.Property).ThenByDescending(item => item.SecondProperty);
// Example
var result = people.OrderBy(p => p.Name).ThenByDescending(p => p.Age);
Grouping
GroupBy Groups the elements of a sequence according to a specified key selector function.
var result = collection.GroupBy(item => item.Key);
// Example
var result = people.GroupBy(p => p.City);
Joining
Join Correlates the elements of two sequences based on matching keys.
var result = collectionA.Join(collectionB, a => a.Key, b => b.Key, (a, b) => new { a.Property, b.Property });
// Example
var result = customers.Join(orders, c => c.Id, o => o.CustomerId, (c, o) => new { c.Name, o.OrderDate });
GroupJoin Correlates the elements of two sequences based on matching keys and groups the results.
var result = collectionA.GroupJoin(collectionB, a => a.Key, b => b.Key, (a, bGroup) => new { a.Property, bGroup });
// Example
var result = customers.GroupJoin(orders, c => c.Id, o => o.CustomerId, (c, os) => new { c.Name, Orders = os });
Set Operations
Distinct Returns distinct elements from a sequence.
var result = collection.Distinct();
// Example
var result = numbers.Distinct();
Union Produces the set union of two sequences.
var result = collectionA.Union(collectionB);
// Example
var result = numbersA.Union(numbersB);
Intersect Produces the set intersection of two sequences.
var result = collectionA.Intersect(collectionB);
// Example
var result = numbersA.Intersect(numbersB);
Except Produces the set difference of two sequences.
var result = collectionA.Except(collectionB);
// Example
var result = numbersA.Except(numbersB);
Aggregation
Count Counts the elements in a sequence.
var result = collection.Count();
// Example
var result = people.Count();
LongCount Counts the elements in a sequence, using Int64.
var result = collection.LongCount();
// Example
var result = people.LongCount();
Sum Computes the sum of the sequence of numeric values.
var result = collection.Sum(item => item.NumericProperty);
// Example
var result = numbers.Sum();
Min Finds the minimum value in a sequence.
var result = collection.Min(item => item.NumericProperty);
// Example
var result = numbers.Min();
Max Finds the maximum value in a sequence.
var result = collection.Max(item => item.NumericProperty);
// Example
var result = numbers.Max();
Average Computes the average of the sequence of numeric values.
var result = collection.Average(item => item.NumericProperty);
// Example
var result = numbers.Average();
Aggregate Applies an accumulator function over a sequence.
var result = collection.Aggregate((acc, item) => acc + item);
// Example
var result = numbers.Aggregate((acc, n) => acc + n);
Element Operations
First Returns the first element of a sequence.
var result = collection.First();
// Example
var result = people.First();
FirstOrDefault Returns the first element of a sequence, or a default value if no element is found.
var result = collection.FirstOrDefault();
// Example
var result = people.FirstOrDefault();
Last Returns the last element of a sequence.
var result = collection.Last();
// Example
var result = people.Last();
LastOrDefault Returns the last element of a sequence, or a default value if no element is found.
var result = collection.LastOrDefault();
// Example
var result = people.LastOrDefault();
Single Returns the only element of a sequence, and throws an exception if there is not exactly one element in the sequence.
var result = collection.Single();
// Example
var result = people.Single(p => p.Id == 1);
SingleOrDefault Returns the only element of a sequence, or a default value if the sequence is empty; this method throws an exception if there is more than one element in the sequence.
var result = collection.SingleOrDefault();
// Example
var result = people.SingleOrDefault(p => p.Id == 1);
ElementAt Returns the element at a specified index in a sequence.
var result = collection.ElementAt(index);
// Example
var result = numbers.ElementAt(2);
ElementAtOrDefault Returns the element at a specified index in a sequence, or a default value if the index is out of range.
var result = collection.ElementAtOrDefault(index);
// Example
var result = numbers.ElementAtOrDefault(10);
Quantifiers
Any Determines whether any element of a sequence satisfies a condition.
var result = collection.Any(item => item.Condition);
// Example
var result = people.Any(p => p.Age > 18);
All Determines whether all elements of a sequence satisfy a condition.
var result = collection.All(item => item.Condition);
// Example
var result = people.All(p => p.Age > 18);
Contains Determines whether a sequence contains a specified element.
var result = collection.Contains(item);
// Example
var result = numbers.Contains(5);
SequenceEqual Determines whether two sequences are equal by comparing their elements by using a specified IEqualityComparer.
var result = collectionA.SequenceEqual(collectionB);
// Example
var result = numbersA.SequenceEqual(numbersB);
Conversion
ToList Creates a List from an IEnumerable.
var result = collection.ToList();
// Example
var result = people.ToList();
ToArray Creates an array from an IEnumerable.
var result = collection.ToArray();
// Example
var result = people.ToArray();
ToDictionary Creates a Dictionary from an IEnumerable according to a specified key selector function.
var result = collection.ToDictionary(item => item.Key);
// Example
var result = people.ToDictionary(p => p.Id);
AsEnumerable Returns the input typed as IEnumerable.
var result = collection.AsEnumerable();
// Example
var result = people.AsEnumerable();
AsQueryable Converts an IEnumerable to an IQueryable.
var result = collection.AsQueryable();
// Example
var result = people.AsQueryable();
Cast Casts the elements of an IEnumerable to the specified type.
var result = collection.Cast<DesiredType>();
// Example
var result = mixedList.Cast<int>();
Concatenation
Concat Concatenates two sequences.
var result = collectionA.Concat(collectionB);
// Example
var result = numbersA.Concat(numbersB);
Generation
DefaultIfEmpty Returns the elements of the specified sequence or the specified value in a singleton collection if the sequence is empty.
var result = collection.DefaultIfEmpty();
var resultWithDefault = collection.DefaultIfEmpty(defaultValue);
// Example
var result = emptyNumbers.DefaultIfEmpty();
var resultWithDefault = emptyNumbers.DefaultIfEmpty(0);
Range Generates a sequence of integral numbers within a specified range.
var result = Enumerable.Range(start, count);
// Example
var result = Enumerable.Range(1, 10);
Repeat Generates a sequence that contains one repeated value.
var result = Enumerable.Repeat(value, count);
// Example
var result = Enumerable.Repeat("Hello", 5);
Appending and Prepending
Append: Appends an element to the end of the sequence.
var collection = new List<int> { 1, 2, 3, 4 }; var newItem = 5; var result = collection.Append(newItem); // Result: { 1, 2, 3, 4, 5 }
Prepend: Prepends an element to the start of the sequence.
var collection = new List<int> { 2, 3, 4, 5 }; var newItem = 1; var result = collection.Prepend(newItem); // Result: { 1, 2, 3, 4, 5 }
Additional Methods
Zip Applies a specified function to the corresponding elements of two sequences, producing a sequence of the results.
var result = collectionA.Zip(collectionB, (a, b) => a.Property + b.Property);
// Example
var result = numbersA.Zip(numbersB, (a, b) => a + b);
Subscribe to my newsletter
Read articles from Md Asif Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
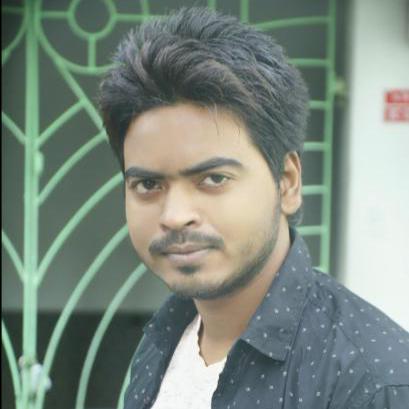
Md Asif Alam
Md Asif Alam
๐ Full Stack .NET Developer & React Enthusiast ๐จโ๐ป About Me: With 3+ years of experience, I'm passionate about crafting robust solutions and seamless user experiences through code. ๐ผ Expertise: Proficient in .NET Core API, ASP.NET MVC, React.js, and SQL. Skilled in backend architecture, RESTful APIs, and frontend development. ๐ Achievements: Led projects enhancing scalability by 50%, delivered ahead of schedule, and contributed to open-source initiatives. ๐ Future Focus: Eager to embrace new technologies and drive innovation in software development. ๐ซ Let's Connect: Open to new opportunities and collaborations. Reach me on LinkedIn or GitHub!