Unleash the Power of Asynchronous Processing: Laravel Jobs and Queues
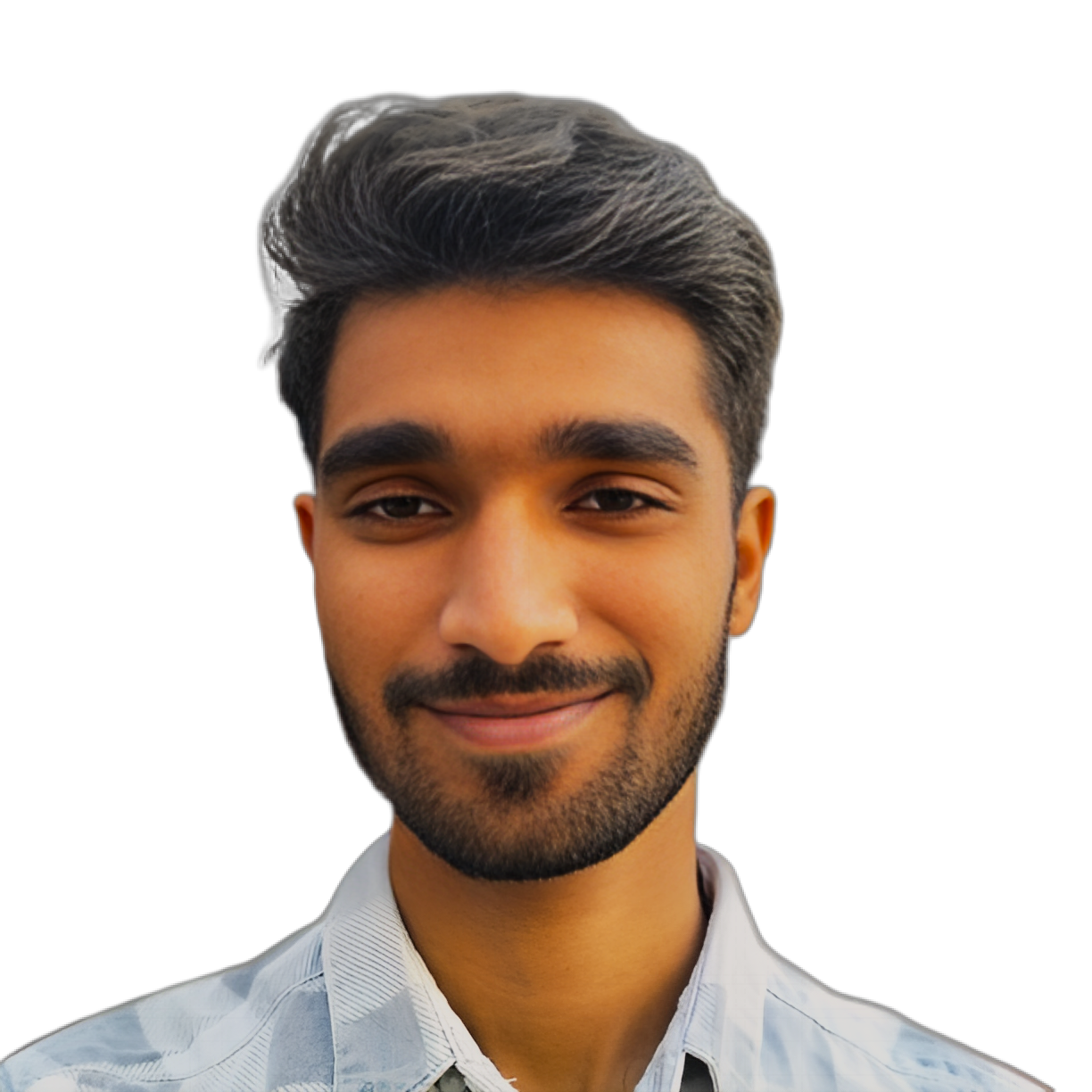
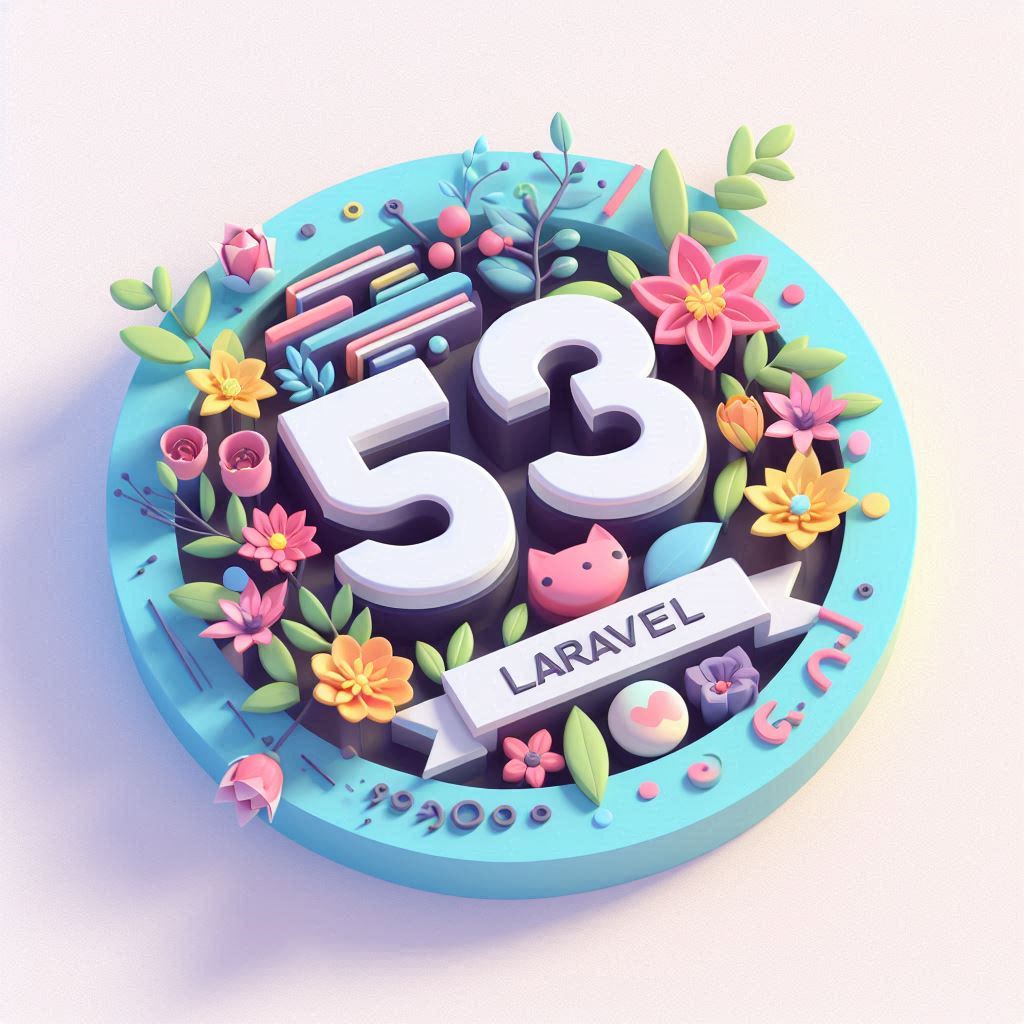
Introduction
In the realm of web development, user experience reigns supreme. Sluggish applications bogged down by time-consuming tasks can leave users frustrated and clicking away. This is where Laravel's ingenious Jobs and Queues system steps in, offering a potent solution to streamline background processing and elevate your application's performance.
The Challenge: Slow Web Requests
Imagine a user submitting a form, eager for confirmation. But behind the scenes, the application grinds to a halt, sending an email notification. This delays the user's response, leading to a perception of sluggishness or even failure. These long-running tasks, like email processing, data manipulation, or image resizing, can significantly hamper responsiveness.
The Solution: Enter Laravel Jobs and Queues
Laravel Queues provide a robust mechanism for delegating these lengthy tasks to a dedicated worker process, effectively decoupling them from the web request cycle. This magic unfolds through the following steps:
Job Creation: You define the task logic within a Job class using the
php artisan make:job MyJob
command. This class encapsulates the code that needs to be executed asynchronously.Dispatching the Job: Within your controller or service, you dispatch the job to the queue using
Job::dispatch()
. This adds the job to the queue, akin to placing it in a waiting line.Worker Process: A worker process, independent of the web server, continuously retrieves jobs from the queue, executes them one by one, and handles any potential failures.
Benefits of Using Laravel Queues
By embracing Laravel Queues, you unlock a treasure trove of advantages for your application:
Enhanced User Experience: Faster response times equate to a smoother and more delightful user experience. Users won't be left waiting while long-running tasks execute.
Increased Concurrency: Your application can handle more requests concurrently, as the web server is no longer bogged down by time-consuming tasks. This translates to better scalability for high-traffic scenarios.
Improved Scalability: Easily add more worker processes to handle surging traffic volumes. This ensures your application can gracefully scale to meet growing demands.
Background Processing: Offloading tasks to the queue frees up valuable web server resources for critical tasks that require immediate attention.
Tutorial: Getting Started with Laravel Queues
Let's delve into a practical example to illustrate how you can leverage Laravel Queues in your projects:
Create a Job:
Bash
php artisan make:job SendWelcomeEmail
This command generates a
SendWelcomeEmail
job class within theapp/Jobs
directory.Define the Job Logic:
Open the
SendWelcomeEmail
job class and modify thehandle
method to encapsulate the email sending logic:PHP
<?php namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; use Mail; class SendWelcomeEmail implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; public $user; public function __construct($user) { $this->user = $user; } public function handle() { // Send a welcome email to the user Mail::send('emails.welcome', ['user' => $user], function ($message) { $message->to($this->user->email, $this->user->name)->subject('Welcome to our App!'); }); } }
Here, we're leveraging Laravel's Mail facade to send a welcome email to the newly registered user.
Dispatch the Job:
In your controller or service where you handle user registration, dispatch the job to the queue:
PHP
public function register(Request $request) { // ... user registration logic ... SendWelcomeEmail::dispatch($user); return redirect()->route('home'); }
By dispatching the
SendWelcomeEmail
job, you've effectively delegated the email sending task to the queue, ensuring a smooth user registration experience.
Configuration Considerations
While Laravel Queues offer a seamless out-of-the-box experience, understanding some configuration options can further enhance their effectiveness:
1. Queue Drivers:
Laravel supports a variety of queue drivers to suit your specific needs. The default driver, database
, utilizes your database to store jobs. However, for high-traffic applications, consider more robust drivers like:
Redis: A high-performance in-memory data store that excels in queue processing speed. To use Redis, configure the connection details in your
config/queue.php
file.Amazon SQS: A managed message queuing service from Amazon Web Services (AWS) that offers scalability and reliability. You'll need to create an SQS queue and configure its credentials in
config/queue.php
.Beanstalkd: Another popular queueing system known for its simplicity and efficiency. If you choose Beanstalkd, provide its connection details in the configuration file.
2. Queue Connections and Names:
The config/queue.php
file houses the configuration for queue drivers, connections, and queues. Here's an example breakdown:
PHP
return [
'default' => 'database',
'connections' => [
'database' => [
'driver' => 'database',
'connection' => 'default', // Your database connection name
'queue' => 'default', // Default queue name for this connection
'expire' => 60, // Job lifetime in seconds before retry (optional)
],
// ... other connections for Redis, SQS, etc.
],
'queues' => [
'default' => [], // Default queue options
'high-priority' => [], // Options for high-priority queue
// ... other named queues
],
];
You can define multiple connections and queues within each connection. Jobs are dispatched to specific queues based on your preference. For instance, you might have a high-priority
queue for critical tasks or a long-running
queue for computationally intensive jobs.
3. Job Retries and Timeout:
Jobs may occasionally encounter errors. By default, Laravel will attempt to retry failed jobs a certain number of times before considering them permanently failed. You can configure these retry settings in the config/queue.php
file within the connection or queue options. Additionally, you can set a timeout for jobs to prevent them from running indefinitely.
4. Supervisor Integration
In production environments, consider using a process supervisor like Supervisor or Laravel Horizon to manage your worker processes. Supervisors ensure that worker processes are automatically started, restarted upon failure, and scaled as needed.
Conclusion
Laravel's Jobs and Queues empower you to create highly efficient and scalable web applications. By embracing asynchronous processing, you can enhance user experience, optimize resource utilization, and pave the way for a more robust and responsive application. The configuration options discussed here provide a finer level of control, allowing you to tailor the queue system to your specific project requirements. So, leverage this powerful feature and unlock the full potential of your Laravel applications!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
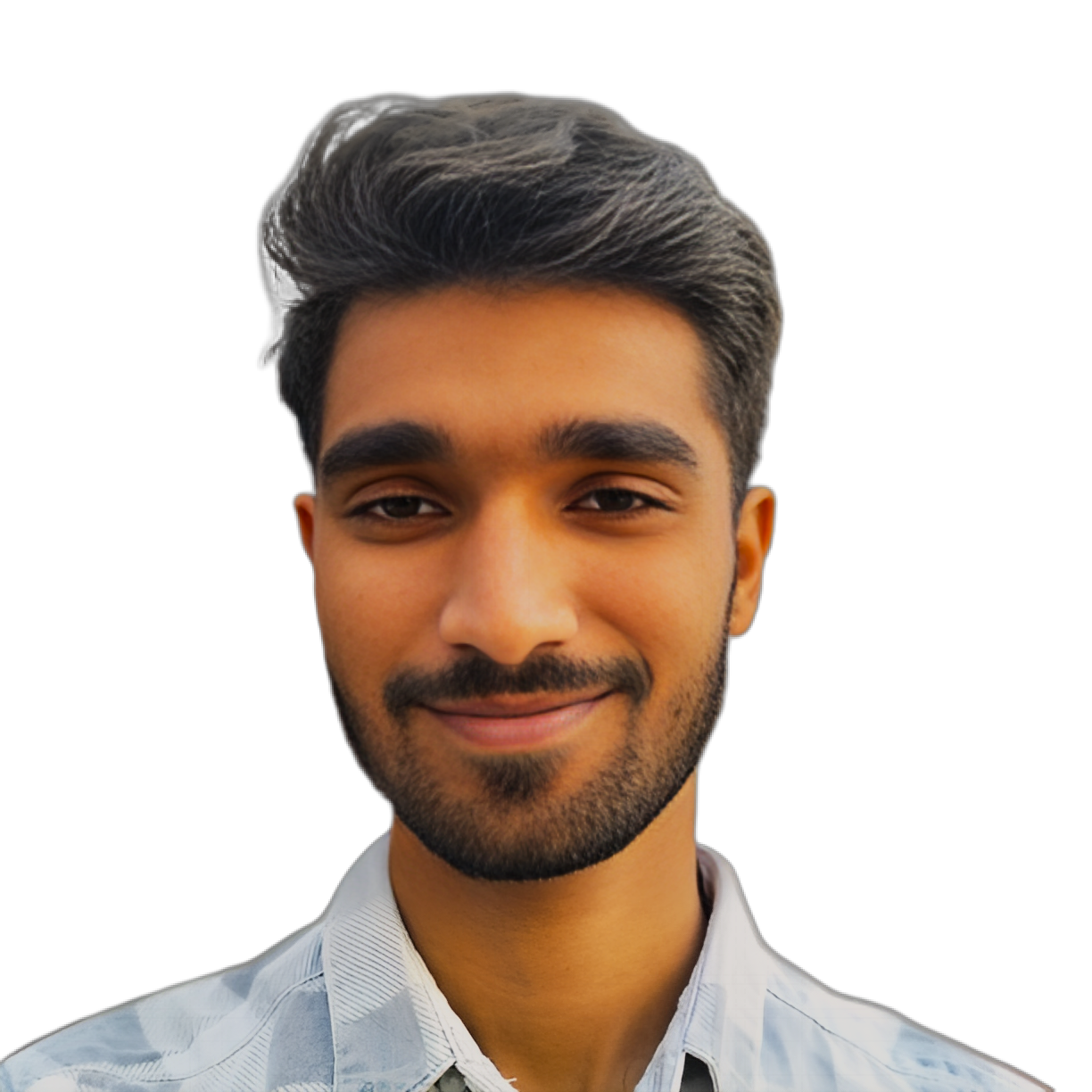
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.