Navigating Connections: A Beginner's Guide to Graph Databases with Neo4j
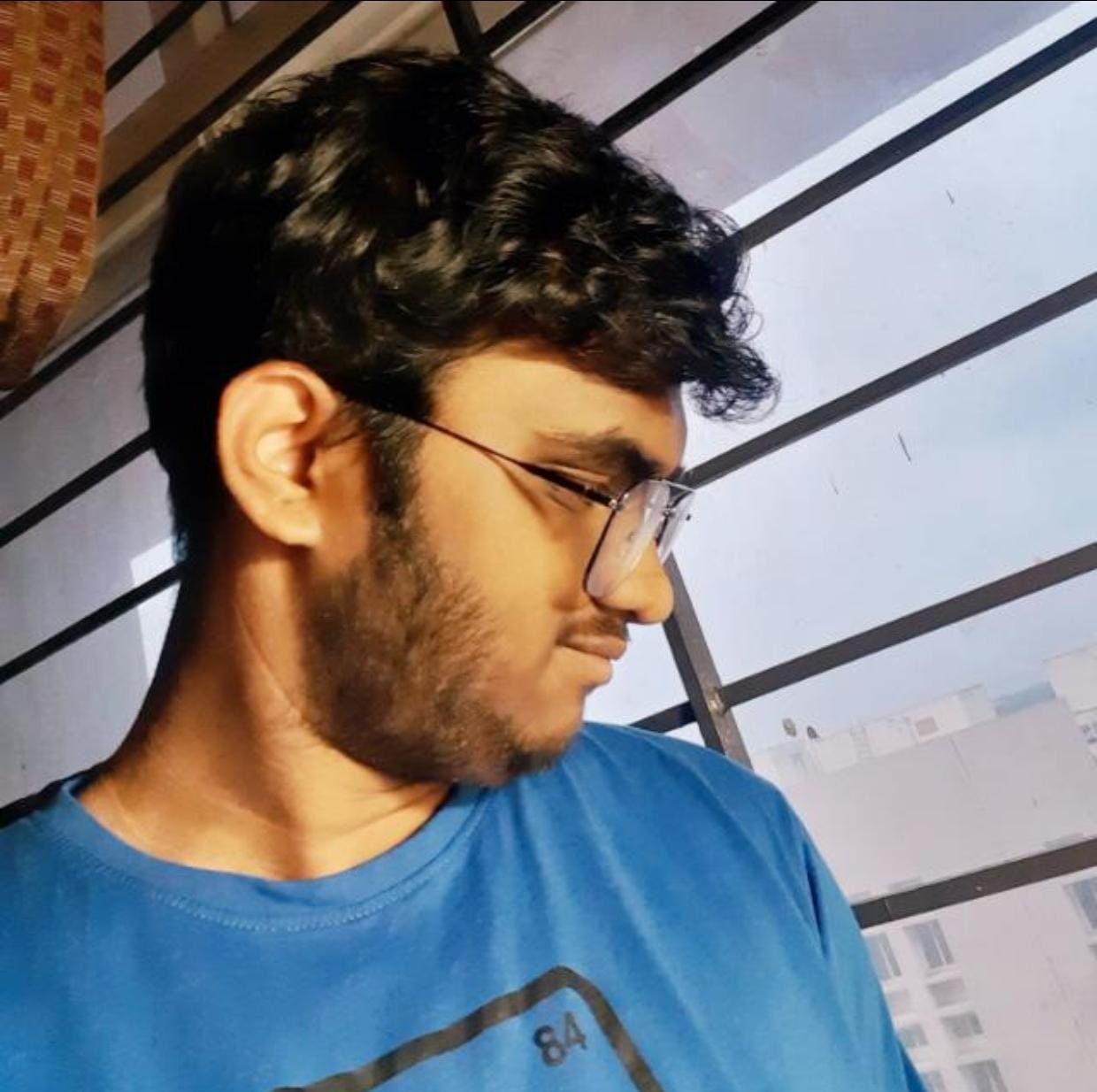
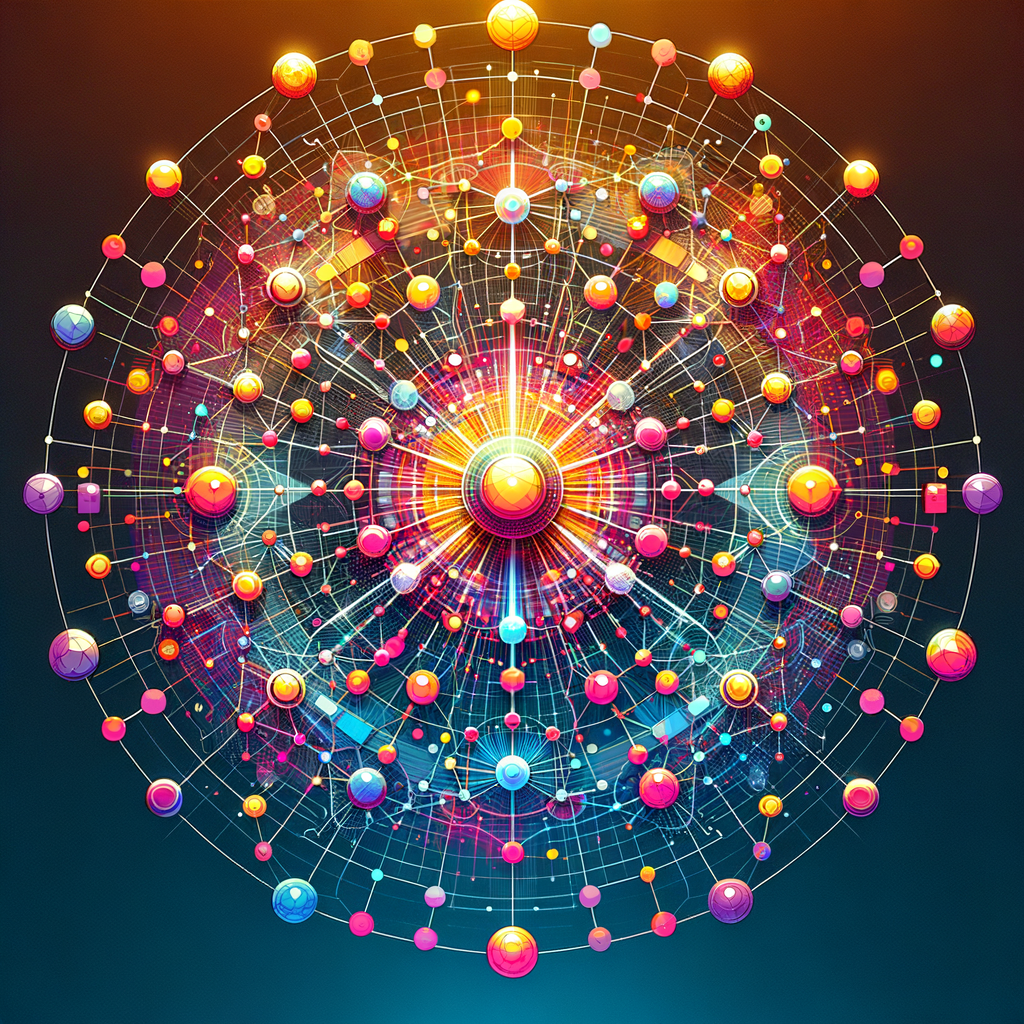
In today's data-driven world, managing and analyzing complex relationships efficiently is crucial. Traditional relational databases often struggle with handling connected data effectively. This is where graph databases shine, offering a more intuitive and powerful way to represent and query data. Neo4j is a leading graph database known for its performance, flexibility, and user-friendliness. In this guide, we'll dive into the basics of implementing a graph database using Neo4j, providing detailed steps to get you started.
What is a Graph Database?
Graph databases store data in the form of nodes, relationships, and properties:
Nodes: Represent entities such as people, products, or events.
Relationships: Represent connections between entities, such as friendships or transactions.
Properties: Store additional information about nodes and relationships, such as names, dates, or quantities.
Why Neo4j?
Neo4j stands out for several reasons:
High Performance: Efficiently manages and queries highly connected data.
Scalability: Handles large datasets and complex queries with ease.
Flexibility: Schema-free model allows for dynamic and evolving data structures.
Cypher Query Language: Intuitive and powerful language designed specifically for graph data.
Getting Started with Neo4j
Installation
First, let's get Neo4j installed and running on your machine:
Download Neo4j: Visit the Neo4j Download Center and select the appropriate version for your operating system.
Install Neo4j: Follow the installation instructions specific to your OS.
Start Neo4j: Once installed, start the Neo4j server. This can be done via the command line or using the Neo4j Desktop application.
Setting Up Your Database
After installation, access the Neo4j Browser at http://localhost:7474
. This web-based interface allows you to interact with your database using Cypher, Neo4j's query language.
Creating Nodes and Relationships
Let's create a simple social network to understand the basics of nodes and relationships.
Creating Nodes
Nodes are created using the CREATE
statement. For example, to create nodes representing people:
CREATE (alice:Person {name: 'Alice', age: 30})
CREATE (bob:Person {name: 'Bob', age: 25})
CREATE (carol:Person {name: 'Carol', age: 35})
Here, Person
is a label assigned to the nodes, and the properties name
and age
provide additional information.
Creating Relationships
Relationships between nodes are also created using the CREATE
statement. Let's create friendships between these people:
MATCH (a:Person {name: 'Alice'}), (b:Person {name: 'Bob'})
CREATE (a)-[:FRIEND]->(b);
MATCH (a:Person {name: 'Alice'}), (c:Person {name: 'Carol'})
CREATE (a)-[:FRIEND]->(c);
In this case, FRIEND
is the type of relationship connecting the nodes.
Querying the Graph
With our basic graph in place, let's explore how to query it. To find all friends of Alice:
MATCH (alice:Person {name: 'Alice'})-[:FRIEND]->(friends)
RETURN friends
This query matches the node labeled Person
with the name 'Alice' and returns all nodes connected to it via the FRIEND
relationship.
More Complex Queries
Graph databases are particularly powerful for handling complex queries involving multiple hops. For example, to find friends of friends of Alice:
MATCH (alice:Person {name: 'Alice'})-[:FRIEND]->()-[:FRIEND]->(fof)
RETURN fof
This query finds nodes that are two hops away from Alice, effectively returning her friends of friends.
Managing Data
In addition to querying, you will often need to update and delete nodes and relationships.
View the Data
To view the graph we can use
MATCH (n)
RETURN n;
We can see the Nodes and the relationship between them.
Updating Nodes
To update a node's properties:
MATCH (alice:Person {name: 'Alice'})
SET alice.age = 31
RETURN alice
This query finds the node labeled Person
with the name 'Alice' and updates her age to 31 as you can see on the right side in Node Properties.
Deleting Nodes and Relationships
To delete a relationship:
MATCH (alice:Person {name: 'Alice'})-[r:FRIEND]->(bob:Person {name: 'Bob'})
DELETE r
To delete a node along with its relationships:
MATCH (alice:Person {name: 'Alice'})
DETACH DELETE alice
The DETACH DELETE
statement ensures that all relationships connected to the node are also removed.
Advanced Features
Neo4j offers numerous advanced features to optimize and enhance your database, such as indexing, constraints, and full-text search.
Indexing and Constraints
Indexing improves query performance, while constraints ensure data integrity. To create an index on the name
property of Person
nodes:
CREATE INDEX ON :Person(name)
To ensure unique names for Person
nodes:
CREATE CONSTRAINT ON (p:Person) ASSERT p.name IS UNIQUE
Full-Text Search
Neo4j's full-text search capabilities allow for more sophisticated text queries. To set up a full-text index:
CALL db.index.fulltext.createNodeIndex('personIndex', ['Person'], ['name'])
Searching within this index:
CALL db.index.fulltext.queryNodes('personIndex', 'Alice')
YIELD node, score
RETURN node.name, score
Real-World Application Example
Let's implement a more comprehensive example to illustrate how Neo4j can be used in a real-world scenario. Consider a simple recommendation system for a social media app.
Building a Social Media App with Neo4j
Graph databases are a natural fit for social media applications due to their ability to efficiently handle complex relationships and connections. In this guide, we’ll explore how to implement a basic social media app using Neo4j, and discuss how platforms like Instagram or Facebook might leverage graph databases for more complex use cases.
/* Create User nodes */
CREATE (alice:User {username: 'alice', name: 'Alice', age: 25})
CREATE (bob:User {username: 'bob', name: 'Bob', age: 22})
CREATE (carol:User {username: 'carol', name: 'Carol', age: 30})
/* Create Post nodes */
CREATE (post1:Post {id: 1, content: 'Hello World!', timestamp: '2024-01-01T10:00:00'})
CREATE (post2:Post {id: 2, content: 'My first post!', timestamp: '2024-01-02T12:00:00'})
/* Create Comment nodes */
CREATE (comment1:Comment {id: 1, content: 'Nice post!', timestamp: '2024-01-01T11:00:00'})
CREATE (comment2:Comment {id: 2, content: 'Welcome!', timestamp: '2024-01-02T13:00:00'})
/* Create relationships */
MATCH (alice:User {username: 'alice'}), (bob:User {username: 'bob'})
CREATE (alice)-[:FRIEND]->(bob)
MATCH (alice:User {username: 'alice'}), (post1:Post {id: 1})
CREATE (alice)-[:POSTED]->(post1)
MATCH (bob:User {username: 'bob'}), (post2:Post {id: 2})
CREATE (bob)-[:POSTED]->(post2)
MATCH (carol:User {username: 'carol'}), (comment1:Comment {id: 1}), (post1:Post {id: 1})
CREATE (carol)-[:COMMENTED_ON]->(comment1)-[:ON]->(post1)
MATCH (alice:User {username: 'alice'}), (comment2:Comment {id: 2}), (post2:Post {id: 2})
CREATE (alice)-[:COMMENTED_ON]->(comment2)-[:ON]->(post2)
MATCH (alice:User {username: 'alice'}), (post2:Post {id: 2})
CREATE (alice)-[:LIKED]->(post2)
Querying the Database
Find All Posts by Friends of a User
To find all posts made by friends of a user:
MATCH (user:User {username: 'alice'})-[:FRIEND]->(friend)-[:POSTED]->(post:Post)
RETURN friend.username, post.content, post.timestamp
Find All Comments on a User’s Posts
To find all comments on posts made by a user:
MATCH (user:User {username: 'bob'})-[:POSTED]->(post:Post)<-[:ON]-(comment:Comment)<-[:COMMENTED_ON]-(commenter:User)
RETURN post.content, commenter.username, comment.content, comment.timestamp
Find All Users Who Liked a Specific Post
To find all users who liked a specific post:
MATCH (post:Post {id: 2})<-[:LIKED]-(user:User)
RETURN user.username
Advanced Use Case: Instagram or Facebook
Platforms like Instagram or Facebook use graph databases to handle large volumes of interconnected data efficiently. Here are some more complex queries and schema expansions that such platforms might use.
Schema Expansion
Adding Hashtags and User Tags
/* Create Hashtag nodes */
CREATE (hashtag1:Hashtag {name: '#fun'})
CREATE (hashtag2:Hashtag {name: '#travel'})
/* Create relationships for hashtags */
MATCH (post1:Post {id: 1}), (hashtag1:Hashtag {name: '#fun'})
CREATE (post1)-[:HAS_HASHTAG]->(hashtag1)
/* Create User Tag relationships */
MATCH (post1:Post {id: 1}), (bob:User {username: 'bob'})
CREATE (post1)-[:TAGGED]->(bob)
Complex Queries
Trending Hashtags
To find the most used hashtags:
MATCH (hashtag:Hashtag)<-[:HAS_HASHTAG]-(post:Post)
RETURN hashtag.name, COUNT(post) AS usageCount
ORDER BY usageCount DESC
LIMIT 10
Recommended Friends Based on Mutual Connections
To recommend friends based on mutual connections:
MATCH (user:User {username: 'alice'})-[:FRIEND]->(friend)-[:FRIEND]->(mutualFriend)
WHERE NOT (user)-[:FRIEND]->(mutualFriend)
RETURN mutualFriend.username, COUNT(friend) AS mutualConnections
ORDER BY mutualConnections DESC
LIMIT 5
User Engagement Analysis
To analyze user engagement (likes and comments on posts):
MATCH (user:User)-[:POSTED]->(post:Post)
OPTIONAL MATCH (post)<-[:LIKED]-(liker:User)
OPTIONAL MATCH (post)<-[:ON]-(comment:Comment)<-[:COMMENTED_ON]-(commenter:User)
RETURN user.username, COUNT(DISTINCT liker) AS likeCount, COUNT(DISTINCT commenter) AS commentCount
ORDER BY likeCount + commentCount DESC
LIMIT 10
Conclusion
Neo4j provides a robust and flexible platform for building and managing social media applications. By leveraging the power of graph databases, platforms like Instagram and Facebook can efficiently handle complex relationships and large volumes of data. This guide has provided a foundational understanding and practical examples to help you get started with Neo4j for social media app development. As you delve deeper, you'll discover more advanced features and capabilities that can further enhance your application's functionality and performance.
Subscribe to my newsletter
Read articles from Souvik Mukherjee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
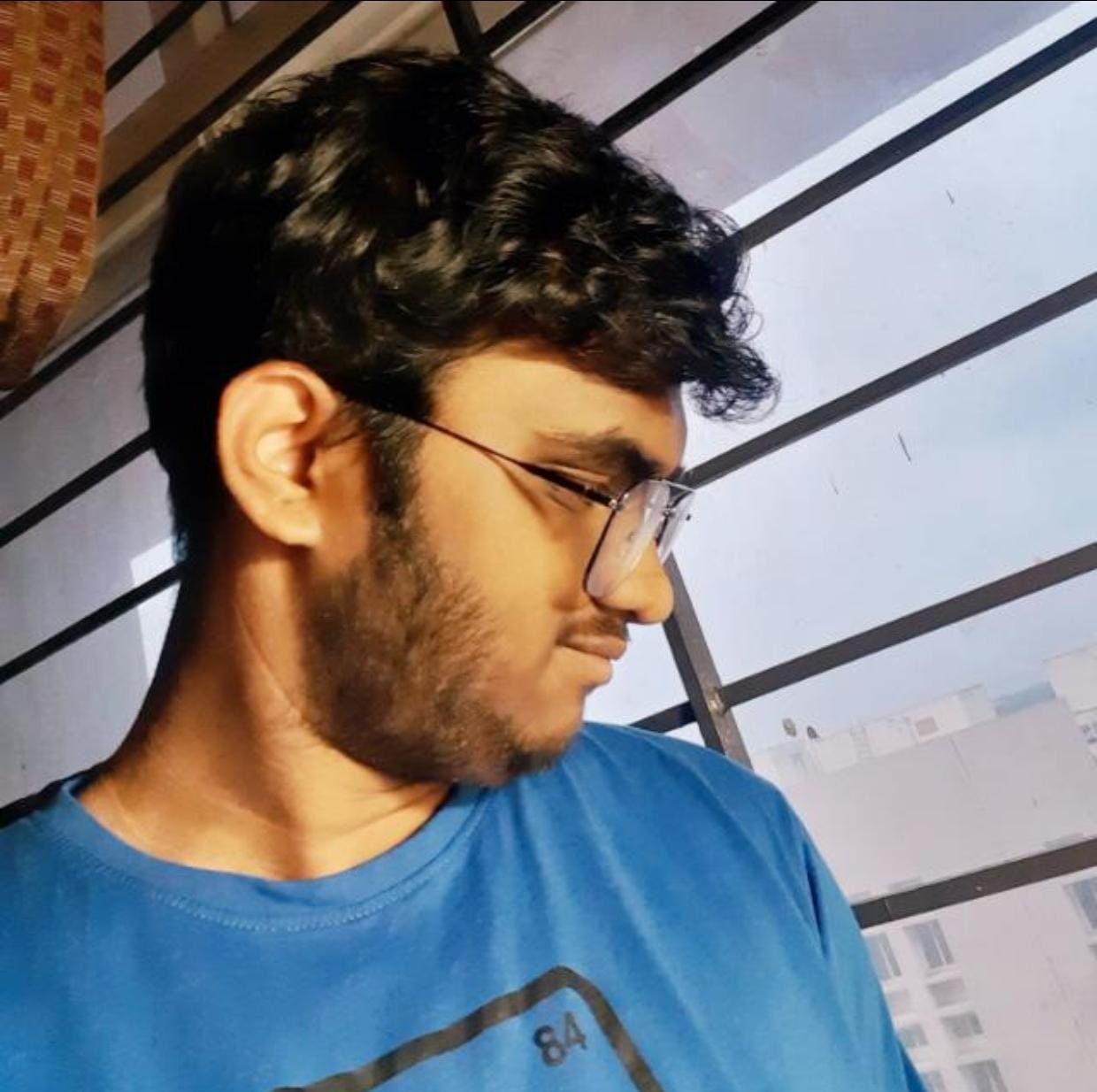
Souvik Mukherjee
Souvik Mukherjee
Hello! I'm Souvik Mukherjee, a passionate Cloud and Devops Engineer with a strong commitment to building scalable and robust backend infrastructure. My background is rich in experience, encompassing the design and implementation of complex backend architectures, with a special focus on serverless and microservices stack.