Score of The String : LeetCode [ 3110 ]

Table of contents
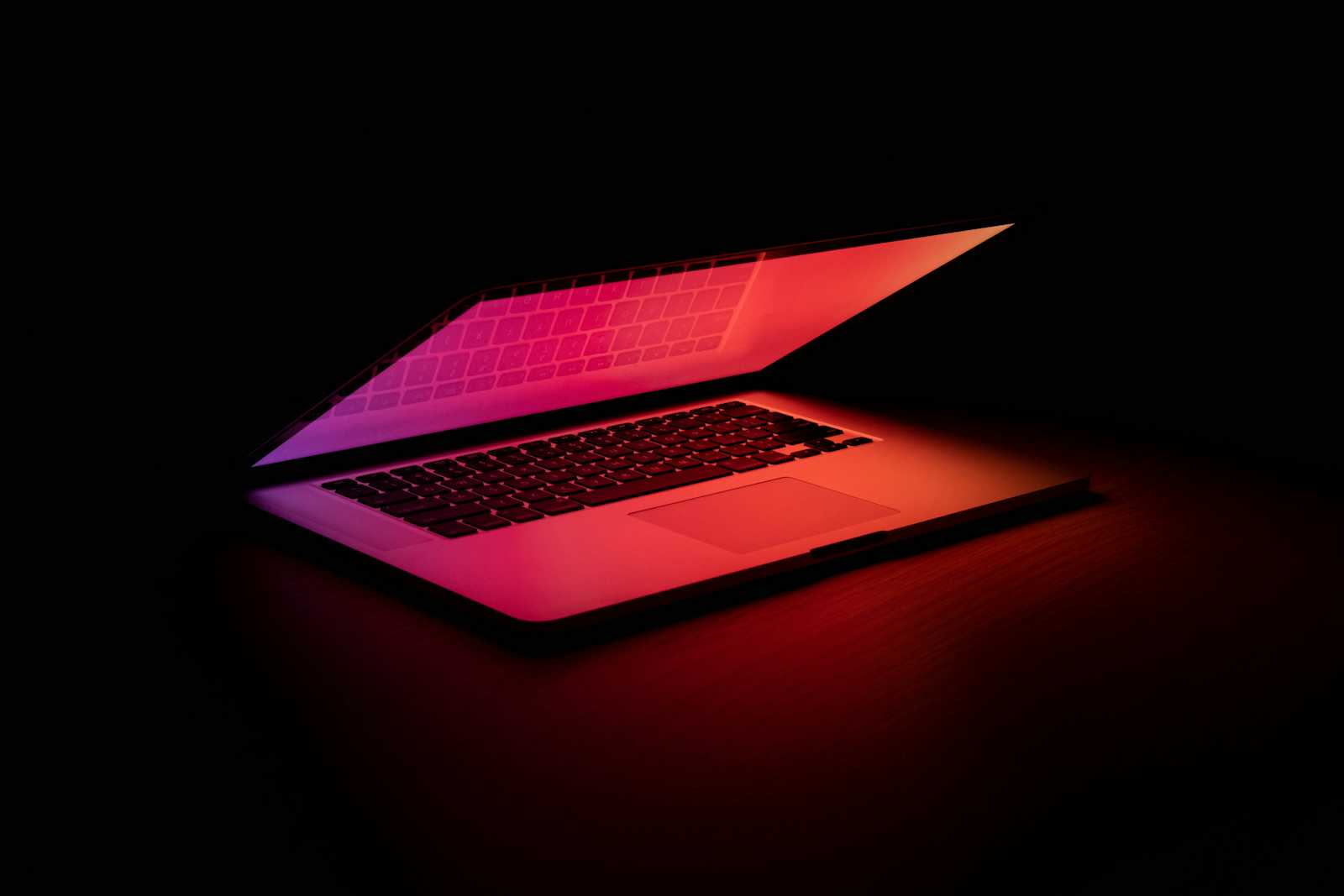
You are given a string s. The score of a string is defined as the sum of the absolute difference between the ASCII values of adjacent characters.
Neighbour means one character to the left and one character to the right of a character i.e; neighbour of (i)th character is (i-1)th character and (i+1)th character.
The first character of string would not have preceding neighbour and last character of the string would not have the succeeding neighbour in the string .... [illustrated in the picture above .. ]
Example 1:
Input: s = "hello"
Output: 13
Explanation:
The ASCII values of the characters in s
are: 'h' = 104
, 'e' = 101
, 'l' = 108
, 'o' = 111
. So, the score of s
would be |104 - 101| + |101 - 108| + |108 - 108| + |108 - 111| = 3 + 7 + 0 + 3 = 13
.
As per the question, we have find score, so while iterating to each character of the string we can know the ascii value of of each character of the string using int(string_name[index]) C++ library function.
We even know about abs() [absolute value function], that it return a positive integer even after getting a negative value as argument to it.
Approach
Initialize the score of the string as 0.
After reaching the characters in the string calculate their ascii value difference to the next element.
Take absolute value of the difference.
Add difference to the score.
After iterating to the index (n-2) we could have also reached to the absolute value difference for the last character.
Return the score.
Complexity
- Time complexity: O(n) --> Since, we need to iterate to all the elements of the string. So, it can not be reduced.
- Space complexity: O(0) : Since, extra space is required.
Code
C++ and Python
class Solution {
public:
int scoreOfString(string s) {
int score = 0;
for (int i = 0; i<s.size()-1 ; i++)
{
score = score + abs(int(s[i])-int(s[i+1]));
}
return score;
}
};
class Solution:
def scoreOfString(self, s: str) -> int:
score = 0
for i in range(len(s) - 1):
score += abs(ord(s[i]) - ord(s[i + 1]))
return score
I hope this blog post has been informative and insightful. If you have any questions or would like to explore more about the LeetCode Problems, feel free to reach out or explore my other blog posts.
Twitter/X -- im_awadh_
LinkedIn -- imawadh
Instagram -- im_awadh_
Subscribe to my newsletter
Read articles from Awadh Kishor Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Awadh Kishor Singh
Awadh Kishor Singh
IIT Madras --- B.S in Data Science and Application Intermediate --- C.H.S [B.H.U] High School --- P.G. Senior Secondary School