Mastering Memory Management in C
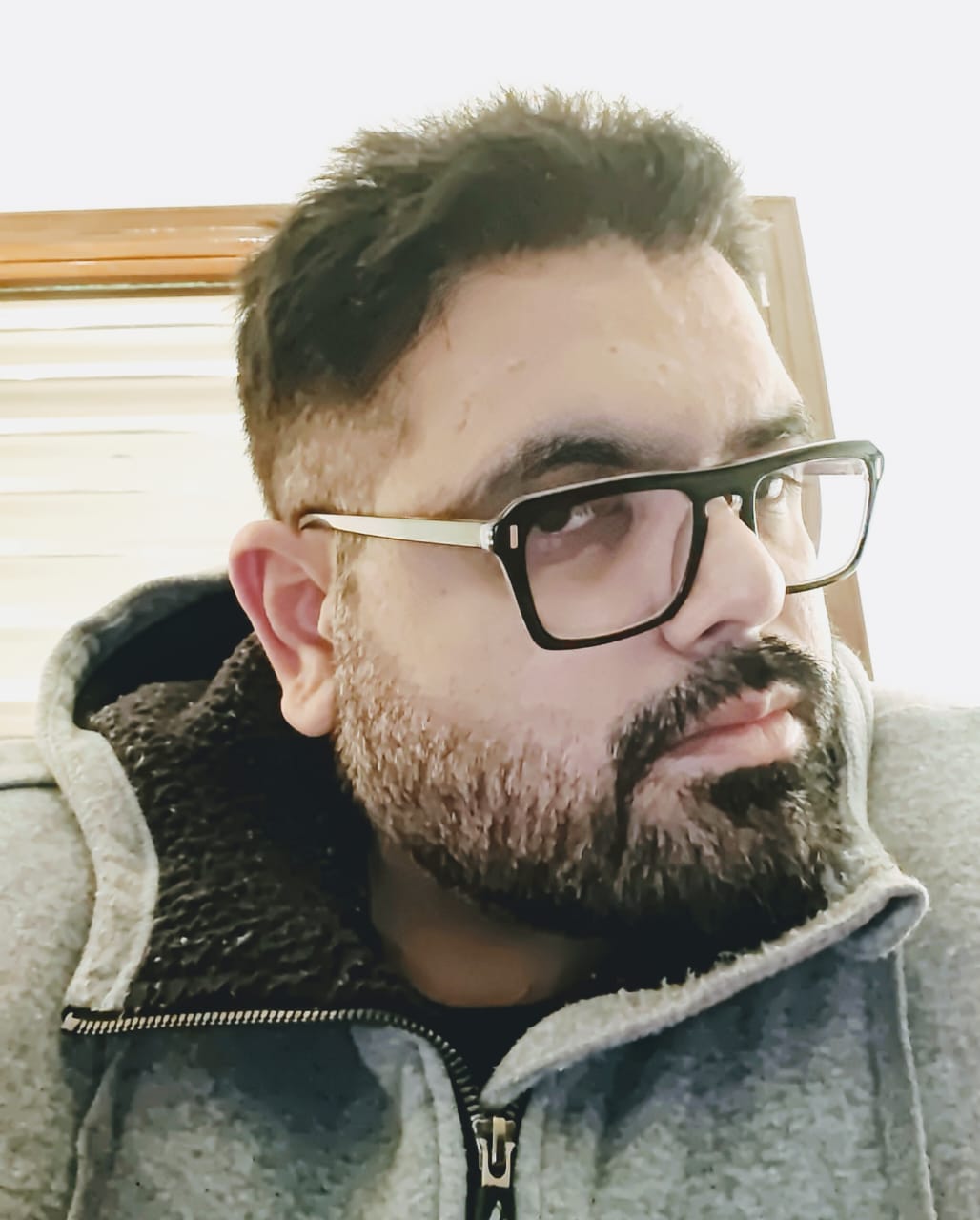
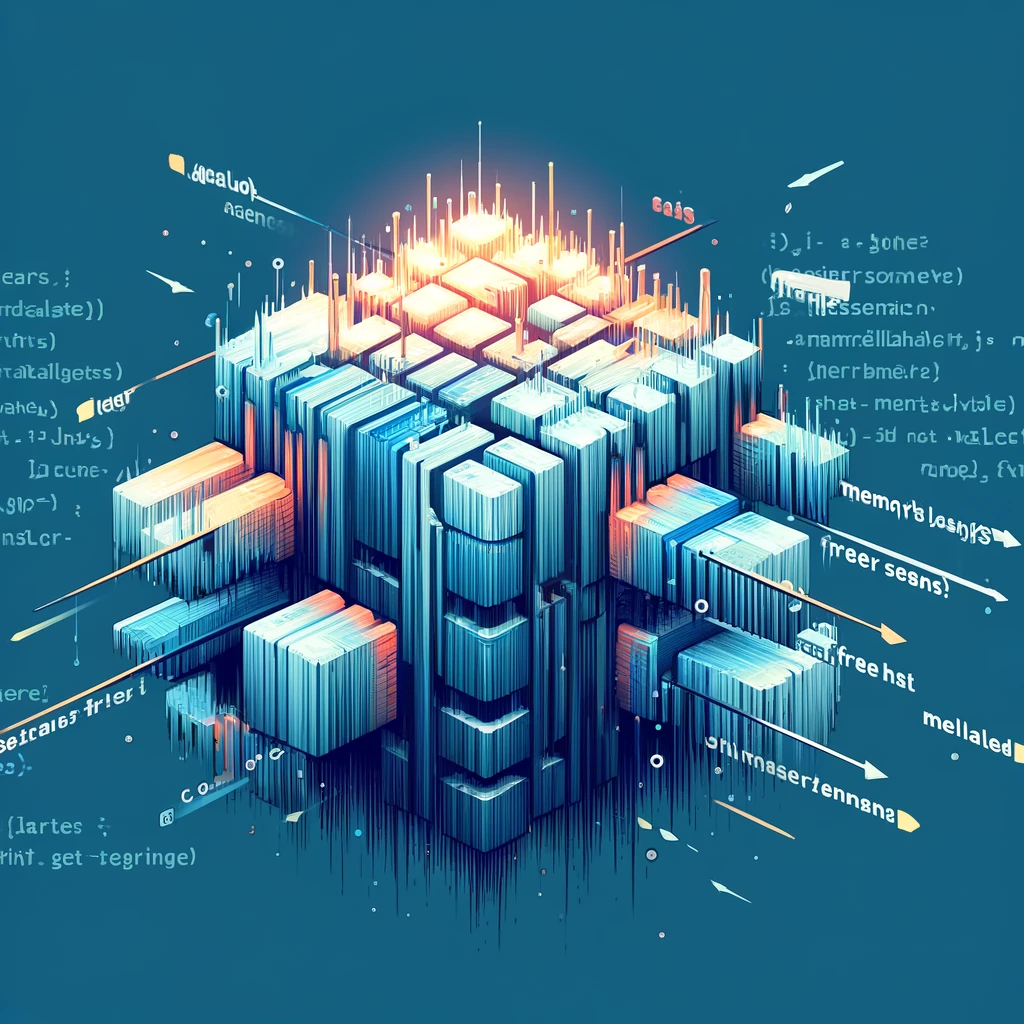
In the realm of modern computing, where artificial intelligence (AI) and supercomputers are pushing the boundaries of what's possible, the importance of secure and efficient programming practices cannot be overstated. One foundational aspect that underpins robust software development, particularly in low-level languages like C, is proper memory management. Failure to handle memory allocation and deallocation correctly can lead to vulnerabilities that can be exploited by malicious actors, compromising the security and integrity of systems. In this article, we'll dive into the intricacies of memory management in C, explore code examples, and understand why this skill is crucial in today's world of advanced computing.
Understanding Memory Management in C
In C, developers have direct control over memory allocation and deallocation. Unlike higher-level languages with automatic memory management (e.g., garbage collection), C programmers must manually manage memory using functions like malloc()
, calloc()
, realloc()
, and free()
. This low-level control offers performance benefits but also introduces the risk of memory-related errors, such as memory leaks and buffer overflows, which can have severe security implications.
Allocating Memory: malloc()
and calloc()
The malloc()
function is used to allocate a block of memory of a specified size. Here's an example:
#include <stdio.h>
#include <stdlib.h>
int main() {
int *ptr = (int*) malloc(sizeof(int) * 5); // Allocate memory for 5 integers
if (ptr == NULL) {
printf("Memory allocation failed.");
return 1;
}
// Use the allocated memory
for (int i = 0; i < 5; i++) {
ptr[i] = i + 1;
}
// Free the allocated memory
free(ptr);
return 0;
}
In this example, we allocate memory for an array of 5 integers using malloc()
. It's crucial to check if the allocation was successful (i.e., if ptr
is not NULL
) before using the allocated memory.
The calloc()
function is similar to malloc()
but initializes the allocated memory to zero.
Deallocating Memory: free()
Once you're done using the allocated memory, it's essential to release it using the free()
function to avoid memory leaks. Memory leaks can lead to resource exhaustion, potentially making your system vulnerable to denial-of-service (DoS) attacks.
free(ptr); // Deallocate the memory pointed to by ptr
Failing to free dynamically allocated memory can result in a steady depletion of available memory, leading to system instability and potential security vulnerabilities.
Buffer Overflows: A Severe Security Risk
Buffer overflows are a common security vulnerability that can occur when writing data beyond the boundaries of an allocated memory buffer. This can lead to corruption of adjacent memory areas, potentially allowing an attacker to execute malicious code or gain unauthorized access to sensitive data.
#include <stdio.h>
#include <string.h>
int main() {
char buffer[10];
strcpy(buffer, "This string is too long"); // Buffer overflow!
return 0;
}
In this example, we attempt to copy a string that is longer than the allocated buffer size, resulting in a buffer overflow. This type of vulnerability can be exploited by attackers to gain control over the program's execution flow or access restricted memory areas.
The Importance of Memory Management in Information Security
In the age of AI and supercomputers, where vast amounts of data are processed and analyzed, memory management takes on even greater significance. These advanced systems often handle sensitive information, making secure programming practices an absolute necessity.
Protecting Sensitive Data: Proper memory management helps prevent unauthorized access to sensitive data, such as personal information, financial records, or confidential business data. Memory-related vulnerabilities like buffer overflows can potentially expose this data to malicious actors.
Maintaining System Stability: Memory leaks and other memory-related issues can lead to resource exhaustion, system crashes, and performance degradation. In critical systems like those used in cybersecurity, finance, or healthcare, system stability is paramount to ensure uninterrupted operation and protect against potential attacks or data breaches.
Securing AI and Supercomputer Systems: As AI and supercomputers become more prevalent in various domains, ensuring the security and reliability of the underlying software becomes increasingly crucial. Memory management errors in these systems can have far-reaching consequences, potentially compromising sensitive data, computational integrity, or even physical systems controlled by these advanced technologies.
By mastering memory management in C, developers can write more secure and robust code, minimizing the risk of vulnerabilities that could be exploited by cybercriminals or other malicious actors. This skill is particularly valuable in the context of information security, where protecting sensitive data and maintaining system integrity is of utmost importance.
Conclusion
Memory management in C programming is a critical skill that directly impacts the security and reliability of software systems. By understanding the intricacies of memory allocation, deallocation, and common vulnerabilities like buffer overflows, developers can write more secure code and mitigate the risk of potential attacks. In the era of AI and supercomputers, where sensitive data and critical systems are at stake, mastering memory management is not just a programming best practice – it's a necessity for safeguarding information and ensuring the integrity of advanced computing technologies.
Subscribe to my newsletter
Read articles from Rai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
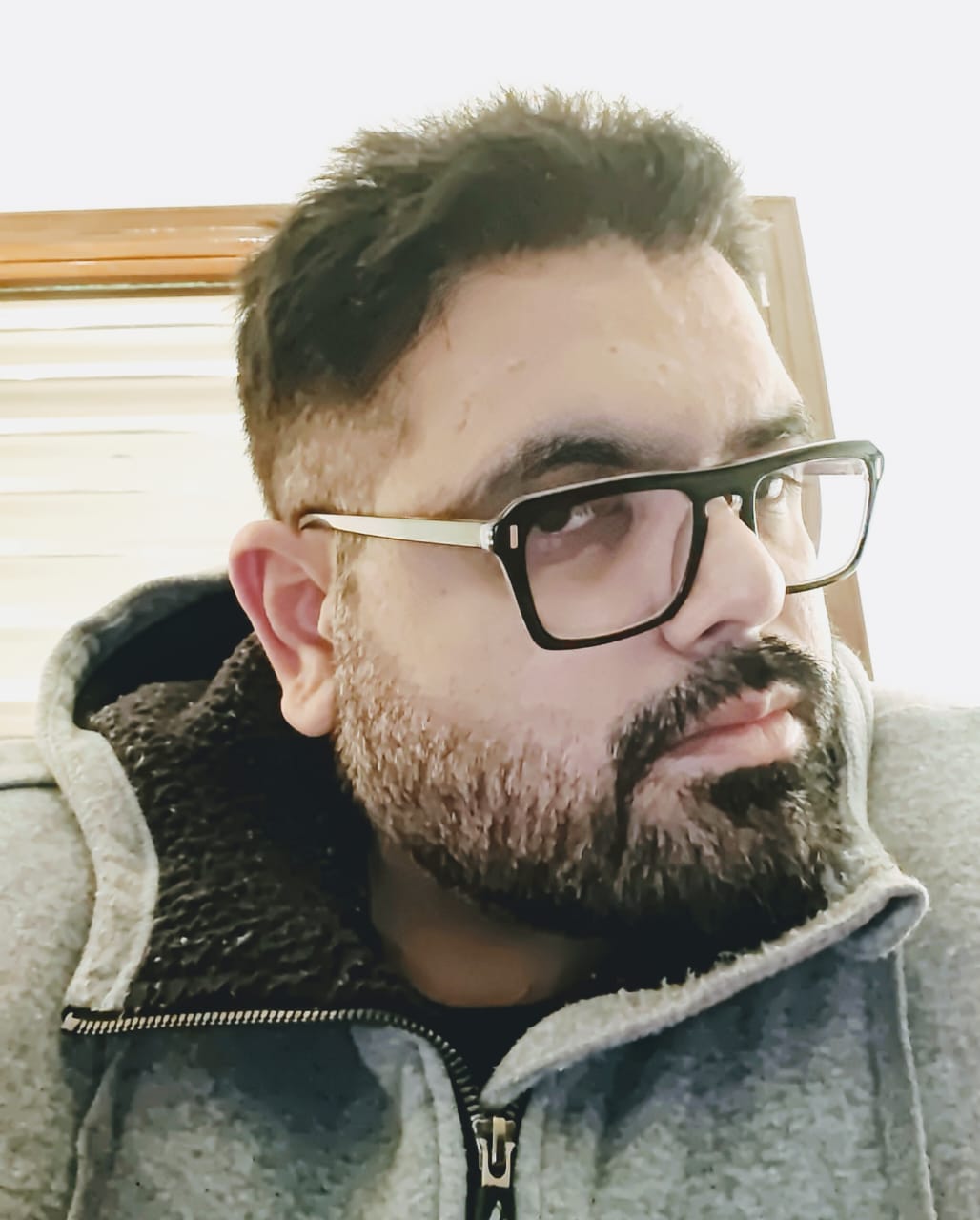
Rai
Rai
Independent Software Systems Engineer