Using Repository Pattern in .NET and EF Core
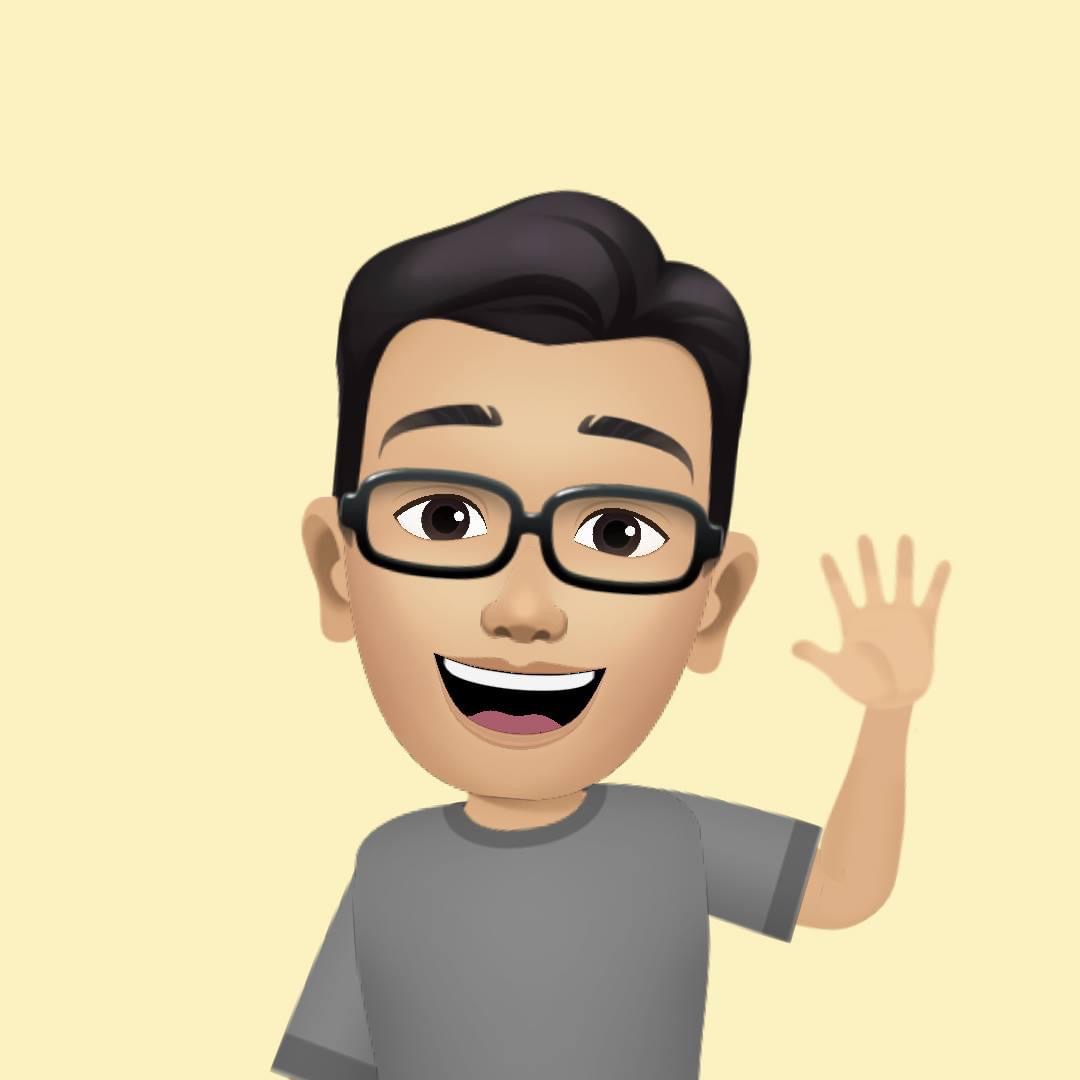
Introduction
The repository pattern is a popular way to achieve separation of concerns in .NET applications. It acts as a bridge between the data access layer and the business logic layer of an application. The aim of this blog post is to delve into the repository pattern, its implementation in .NET, and how it can be combined with Entity Framework Core (EF Core) to enhance data operations.
Understanding the Repository Pattern
The repository pattern is a design pattern that mediates data from and to the domain and data mapping layers (like Entity Framework). It provides a simple, clean way to implement CRUD operations. One of the main benefits of using a repository pattern is the abstraction it provides. You can swap out your data access layer or change your data source without altering the business logic that uses the data. This makes your application more maintainable and flexible.
Implementing Repository Pattern in .NET
In .NET, implementing the repository pattern involves creating an interface that defines the methods for accessing data, and then implementing this interface in a concrete class. A typical repository might have methods like GetById, Insert, Update, and Delete. These methods provide a consistent way to manage data, regardless of the underlying data source or how the data is stored.
Here is an example of what a simple repository interface might look like:
public interface IRepository<T> where T : class
{
T GetById(int id);
IEnumerable<T> GetAll();
void Insert(T entity);
void Update(T entity);
void Delete(T entity);
}
And here is how you might implement this interface:
public class Repository<T> : IRepository<T> where T : class
{
private readonly DbContext context;
private DbSet<T> entities;
public Repository(DbContext context)
{
this.context = context;
entities = context.Set<T>();
}
public T GetById(int id)
{
return entities.Find(id);
}
// Other methods omitted for brevity...
}
Combining Repository Pattern with EF Core
EF Core is a lightweight, extensible, and open-source version of the popular Entity Framework data access technology. Combining the repository pattern with EF Core can greatly simplify your .NET applications. EF Core handles the database side of things, allowing you to focus on your application's business logic. Your repository will call EF Core methods to retrieve data, and then return the data to the business logic layer in a consistent, predictable way.
For example, your repository might use EF Core's Find method to retrieve data:
public T GetById(int id)
{
return entities.Find(id);
}
Conclusion
The repository pattern, when used in combination with technologies like .NET and EF Core, can provide a robust and scalable solution for managing data operations in an application. It not only simplifies the development process but also makes the application more maintainable and flexible. While it may take some time to set up, the benefits it offers make the repository pattern a worthy addition to any .NET developer's toolkit.
Subscribe to my newsletter
Read articles from Awdesh Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
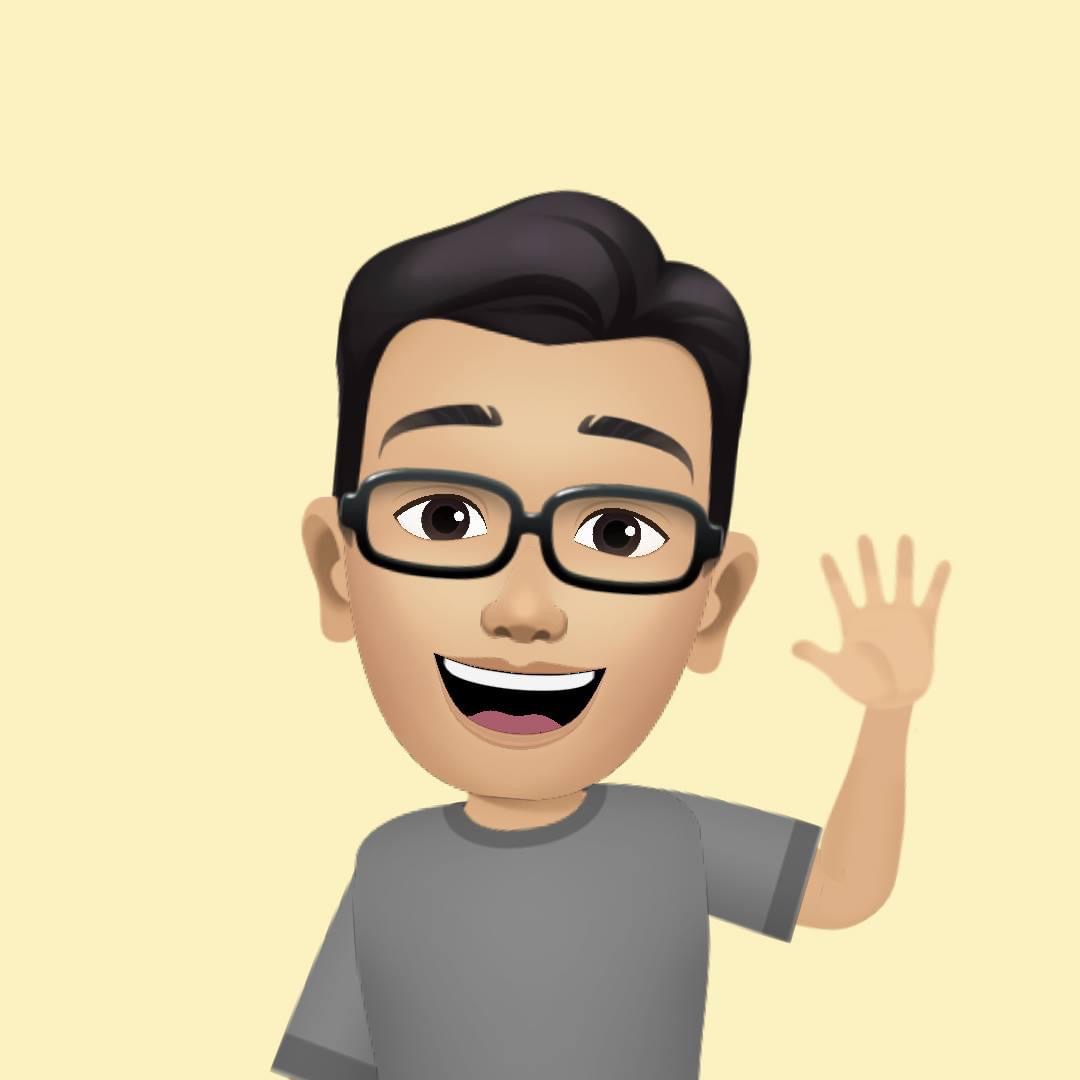