Understanding Type Aliases in TypeScript
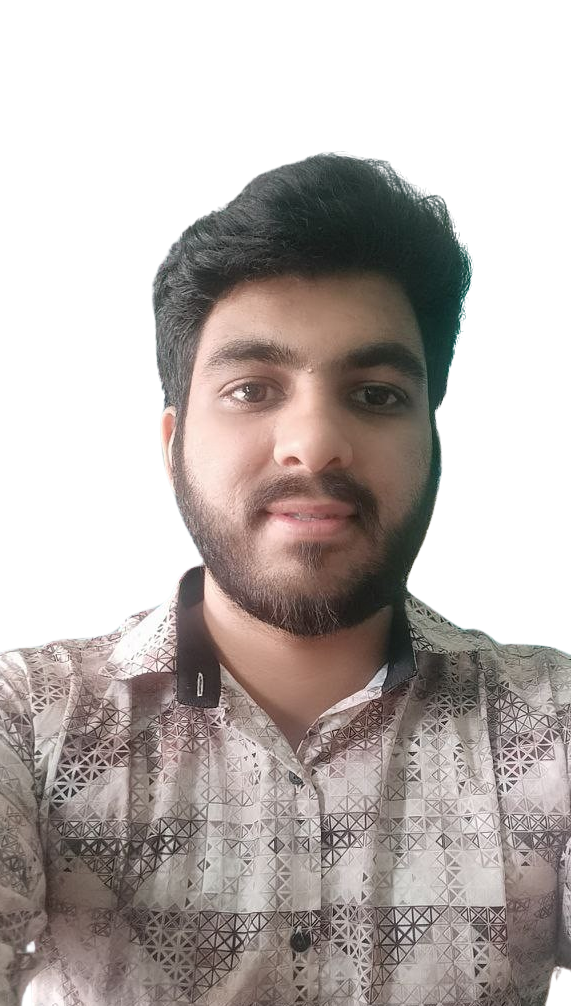
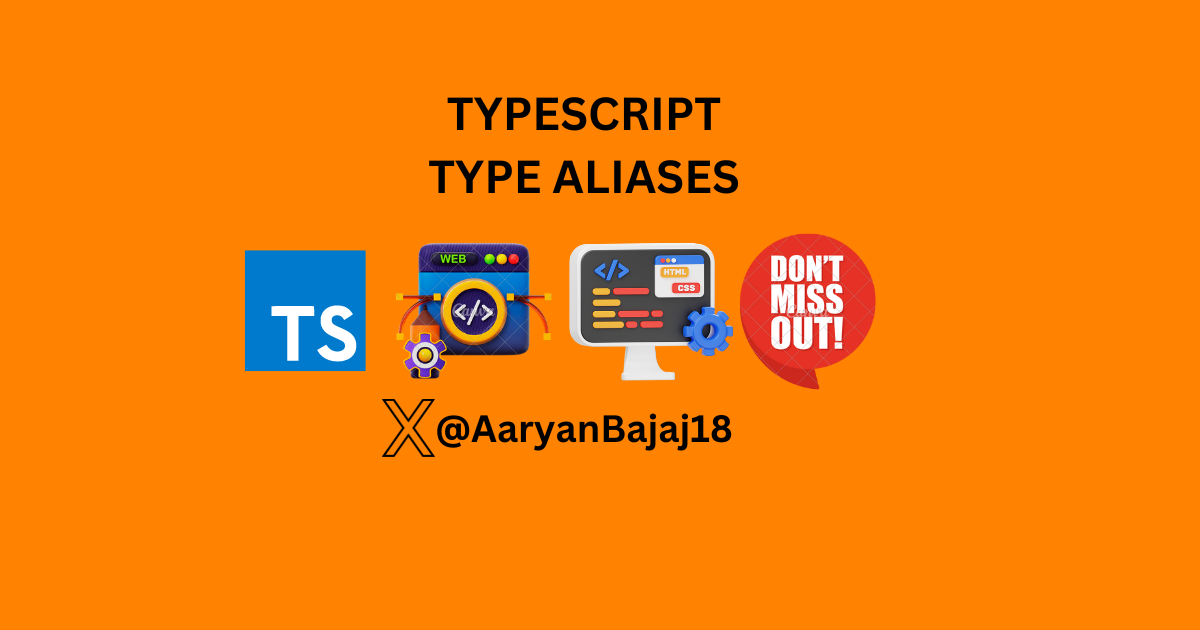
Introduction
Have you ever found yourself writing the same type definition repeatedly in your TypeScript code? It can be tedious and error-prone.
TypeScript offers a powerful feature to streamline your code and make it more maintainable: type aliases.
In this blog, we'll dive into what type aliases are, why they're useful, and how to use them effectively.
By the end, you'll be equipped with the knowledge to write cleaner and more efficient TypeScript code.
What are Type Aliases?
Type aliases in TypeScript allow you to create a new name for an existing type.
This can be particularly helpful when dealing with complex types or when you want to make your code more readable.
A type alias does not create a new type; it simply gives a type a new name.
Syntax
type UserID = string;
In this example, UserID
is an alias for the string
type. You can now use UserID
wherever you would use a string.
Why Use Type Aliases?
Readability: Type aliases can make complex types more understandable at a glance.
Reusability: Define a type once and reuse it throughout your codebase.
Maintainability: Update the type definition in one place and have it reflected everywhere.
Using Type Aliases for Complex Types
Type aliases shine when dealing with complex types, such as objects or unions.
type User = {
id: UserID;
name: string;
email: string;
};
In this example, User
is a type alias for an object with id
, name
, and email
properties. You can now use User
to type variables, function parameters, and return values.
Example with a Union Type
type Status = 'active' | 'inactive' | 'suspended';
Here, Status
is a type alias for a union of string literals. This is useful for defining a set of allowed values for a variable.
Real Life Example
Let's consider a real-life scenario where you are building a user management system. You need to define types for users and their statuses.
type UserID = string;
type User = {
id: UserID;
name: string;
email: string;
status: Status;
};
type Status = 'active' | 'inactive' | 'suspended';
// Function to create a new user
const createUser = (id: UserID, name: string, email: string, status: Status): User => {
return { id, name, email, status };
};
// Example usage
const newUser = createUser('123', 'Jane Doe', 'jane.doe@example.com', 'active');
console.log(newUser);
In this example, type aliases help make the code more readable and maintainable. You can easily understand the structure of a User
and the possible values for Status
.
Conclusion
Type aliases in TypeScript are a powerful tool for improving the readability, reusability, and maintainability of your code.
They allow you to give meaningful names to complex types, making your code easier to understand and work with.
By incorporating type aliases into your TypeScript projects, you can write cleaner, more efficient code that is easier to maintain.
Thank You Everyone For The Read ....
Happy Coding! ๐ค๐ป
๐ Hello, I'm Aaryan Bajaj .
๐ฅฐ If you liked this article, consider sharing it.
Subscribe to my newsletter
Read articles from AARYAN BAJAJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
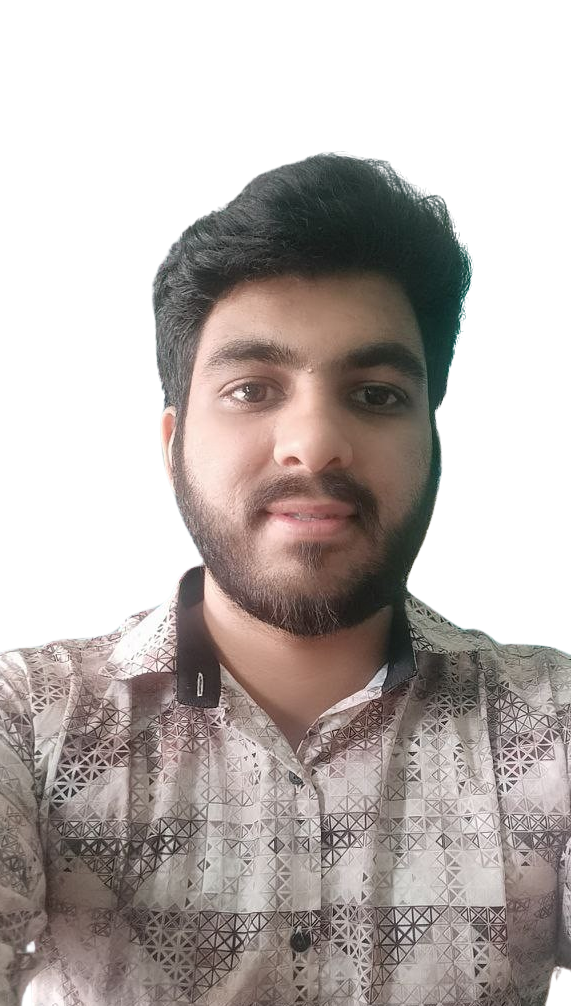
AARYAN BAJAJ
AARYAN BAJAJ
Hi Everyone ๐๐ป , I'm Aaryan Bajaj , a Full Stack Web Developer from India(๐ฎ๐ณ). I share my knowledge through engaging technical blogs on Hashnode, covering web development.