React components go through a lifecycle of events.
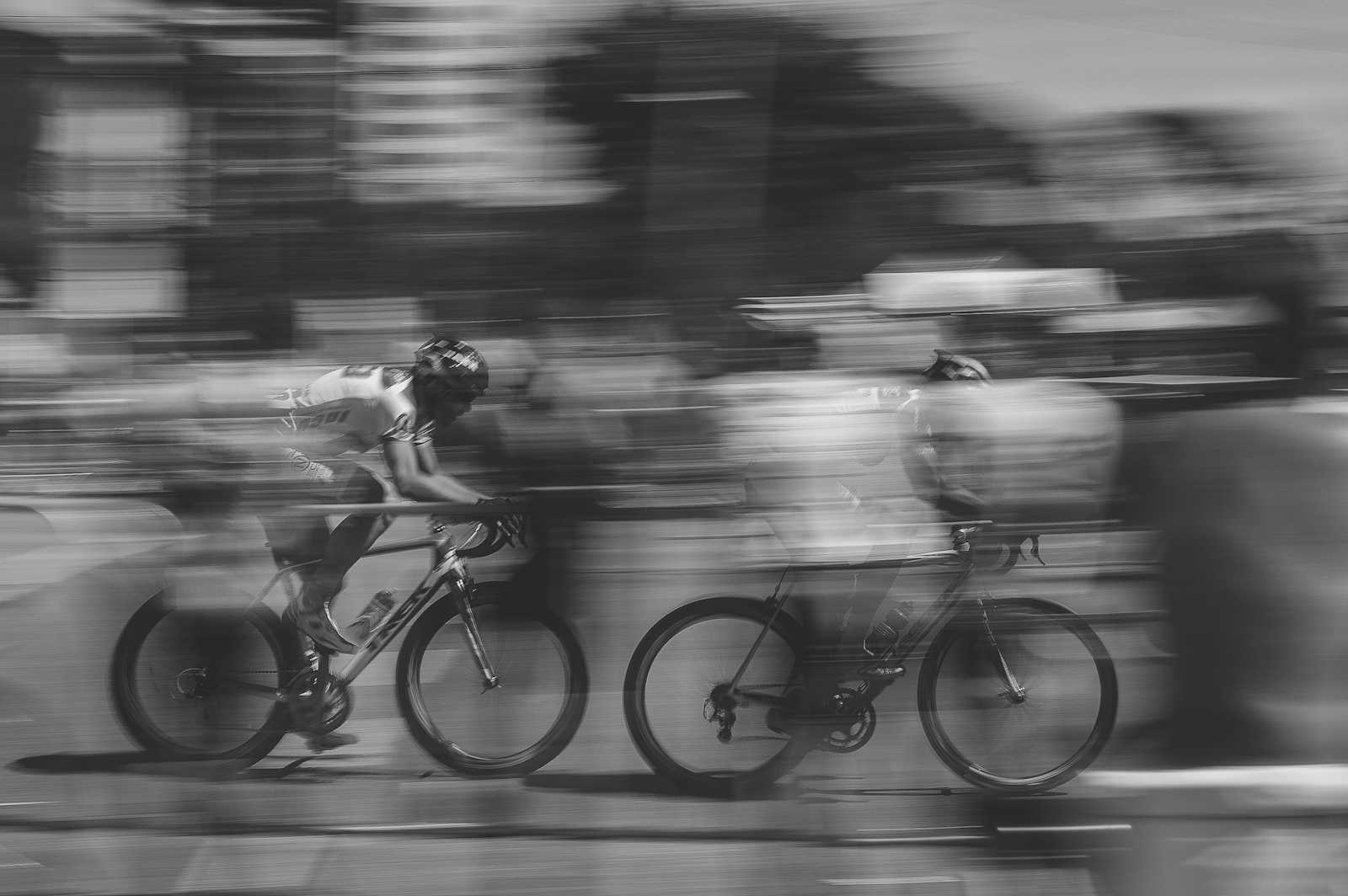
This is a common question asked by frontend developers. There are many articles and videos available on the internet. Now, AI is also available to help.
There isn't much in my post, but if you find something interesting and helpful, it means a lot to me.
Here’s a simplified explanation of the key lifecycle methods
Mounting
These methods are called when a component is being created and added to the DOM.
Functional Components:
useEffect(() => { /* effect */ }, []);
The equivalent of componentDidMount in functional components.
The empty array means this effect runs only once after the initial render.
2. Updating
These methods are called when a component is being re-rendered as a result of changes to props or state.
Functional Components:
useEffect(() => { /* effect */ }, [dependencies])
Runs the effect when dependencies (props/state) change.
It is a combination of componentDidMount and componentDidUpdate.
Unmounting
- This method is called when a component is being removed from the DOM.
Functional Components:
useEffect(() => { return () => { /* cleanup */ } }, [])
- The return function inside the effect acts as componentWillUnmount.
Error Handling
- This method is called when there is an error during rendering, in a lifecycle method, or in the constructor of any child component.
Functional Components:
- Use the ErrorBoundary pattern (class component) to catch errors in functional components.
import React, { useState, useEffect } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
console.log('Component did mount');
return () => {
console.log('Component will unmount');
};
}, []);
useEffect(() => {
console.log('Component did update');
}, [count]);
return (
<div>
<p>{count}</p>
<button onClick={() => setCount(count + 1)}>
Increment
</button>
</div>
);
}
export default MyComponent;
Summary:-
Now you have reached here.
Thank you so much for your patience.
Subscribe to my newsletter
Read articles from Sumit Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by