File Uploads with Multer and Cloudinary: Part 1
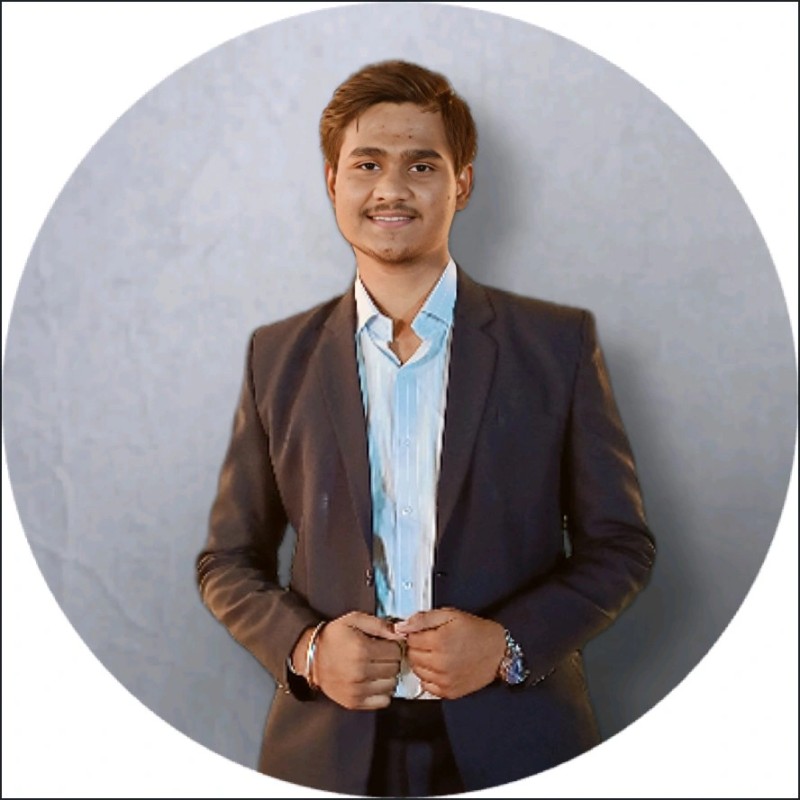
Table of contents
- What is Cloudinary?
- To Install Multer and Cloudinary in your Node.js project, you can use npm, the package manager for Node.js. Here's how you can install them:
- Usage Example:
- Summary:
- Checkout part 2 : https://mywebdevelopmentjouney.hashnode.dev/how-to-implement-file-uploads-in-backend-development-using-multer-and-cloudinarypart-2
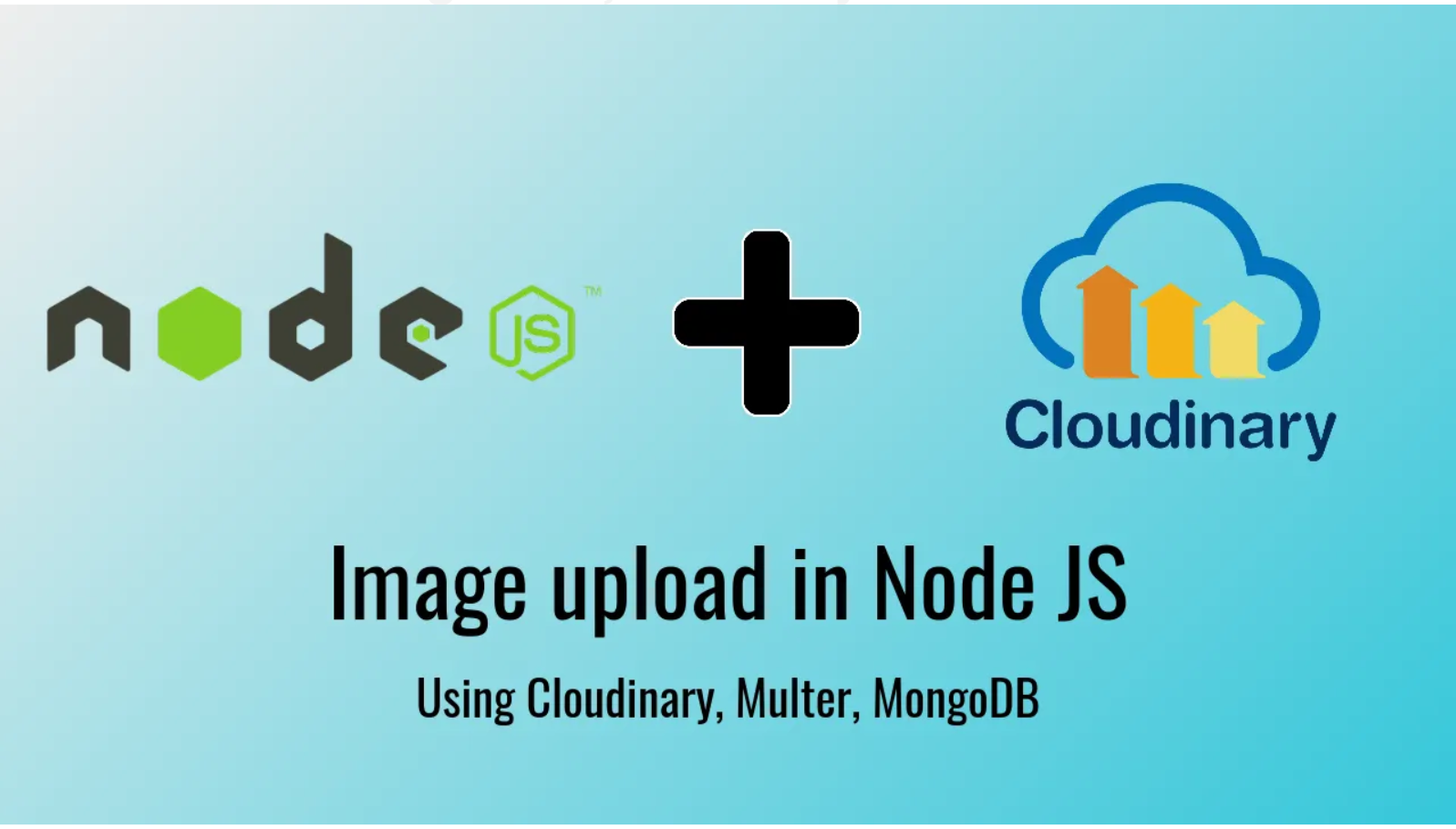
Today's Learning
What is Cloudinary?
Cloudinary is a cloud-based media management platform that provides solutions for:
Uploading: Media files to the cloud.
Storage: Secure and reliable.
Manipulation: Resize, crop, filter via APIs.
Optimization: Fast loading, reduced bandwidth.
Delivery: Efficient global CDN.
Learn how to use Multer for handling file uploads in backend development, including installation, configuration, and implementation steps with cloudinary.
To Install Multer and Cloudinary in your Node.js project, you can use npm, the package manager for Node.js. Here's how you can install them:
Install Multer: npm install multer
Install Cloudinary:npm install cloudinary
These commands will download and install the latest versions of Multer and Cloudinary from the npm registry and add them to your project's package.json
file as dependencies.
After installation, you can import and use Multer and Cloudinary in your Node.js application as needed. Remember to configure Cloudinary with your account credentials to start using its services for media management.
Import Statements:
import { v2 as cloudinary } from 'cloudinary'; import fs from 'fs';
cloudinary
: The Cloudinary library is imported to handle file uploads to Cloudinary.fs
: The Node.js file system module is imported to interact with the local filesystem, specifically to delete the file after upload.
Cloudinary Configuration:
cloudinary.config({ cloud_name: process.env.CLOUDINARY_CLOUD_NAME, api_key: process.env.CLOUDINARY_API_KEY, api_secret: process.env.CLOUDINARY_SECRET, });
This configures the Cloudinary client with your account credentials, which are stored in environment variables for security. The credentials include:
cloud_name
: Your Cloudinary cloud name.api_key
: Your Cloudinary API key.api_secret
: Your Cloudinary API secret.
uploadOnCloudinary Function:
const uploadOnCloudinary = async (localFilePath) => { try { if (!localFilePath) return null;
This function is asynchronous (indicated by
async
) because it involves I/O operations.It takes
localFilePath
as an argument, which is the path of the file you want to upload.The first check is to see if
localFilePath
is provided. If not, it returnsnull
.
File Upload:
const response = await cloudinary.uploader.upload(localFilePath, { resource_type: "auto" }); console.log("File is uploaded on Cloudinary:", response.url);
The
cloudinary.uploader.upload
function is called with the local file path.resource_type: "auto"
tells Cloudinary to automatically detect the type of file (image, video, etc.).await
ensures that the function waits for the upload to complete before proceeding.Upon successful upload, the response from Cloudinary is logged, which includes the URL of the uploaded file.
Local File Deletion:
fs.unlinkSync(localFilePath); return response; } catch (error) { console.error("Error uploading file to Cloudinary:", error);
After a successful upload, the local file is deleted using
fs.unlinkSync
.The function then returns the response from Cloudinary, which contains details about the uploaded file.
Error Handling:
if (fs.existsSync(localFilePath)) { fs.unlinkSync(localFilePath); } return null; } };
If an error occurs during the upload process, it is caught by the
catch
block.The error is logged to the console.
The code checks if the file still exists locally and deletes it to ensure no leftover files.
The function returns
null
to indicate that the upload failed.
Exporting the Function:
export default uploadOnCloudinary;
- The function is exported as the default export of the module, making it available for import in other parts of your application.
Usage Example:
To use the uploadOnCloudinary
function, you would import it and call it with the path to the local file you wish to upload. Here’s how you might use it in practice:
import uploadOnCloudinary from './path/to/your/uploadFunction';
const localFilePath = 'path/to/your/local/file.jpg';
uploadOnCloudinary(localFilePath).then(response => {
if (response) {
console.log('Upload successful:', response);
} else {
console.log('Upload failed.');
}
});
Summary:
The function configures Cloudinary with your credentials.
It uploads a file to Cloudinary and deletes the local file upon successful upload.
If an error occurs, it logs the error, deletes the local file if it exists, and returns
null
.The function is designed to be reusable and is exported for use in other modules.
Implementing file uploads in backend development using Multer and Cloudinary provides a robust solution for handling media files. With Multer, you can easily manage file uploads in your Node.js application, while Cloudinary offers powerful features for uploading, storing, manipulating, optimizing, and delivering media files. By following the steps outlined, you can configure these tools to seamlessly upload files to the cloud and manage them efficiently, ensuring a smooth and secure media management process in your backend development projects.
Checkout part 2 : https://mywebdevelopmentjouney.hashnode.dev/how-to-implement-file-uploads-in-backend-development-using-multer-and-cloudinarypart-2
Subscribe to my newsletter
Read articles from Akash Satpute directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
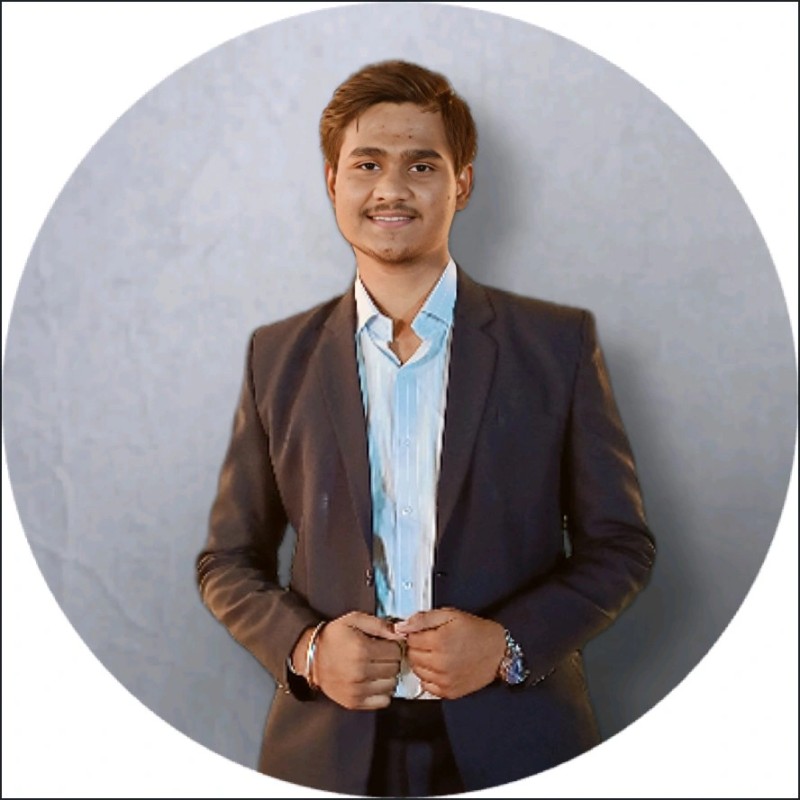