Choosing the Right Cipher Algorithm and Mode for Secure Encryption in Java
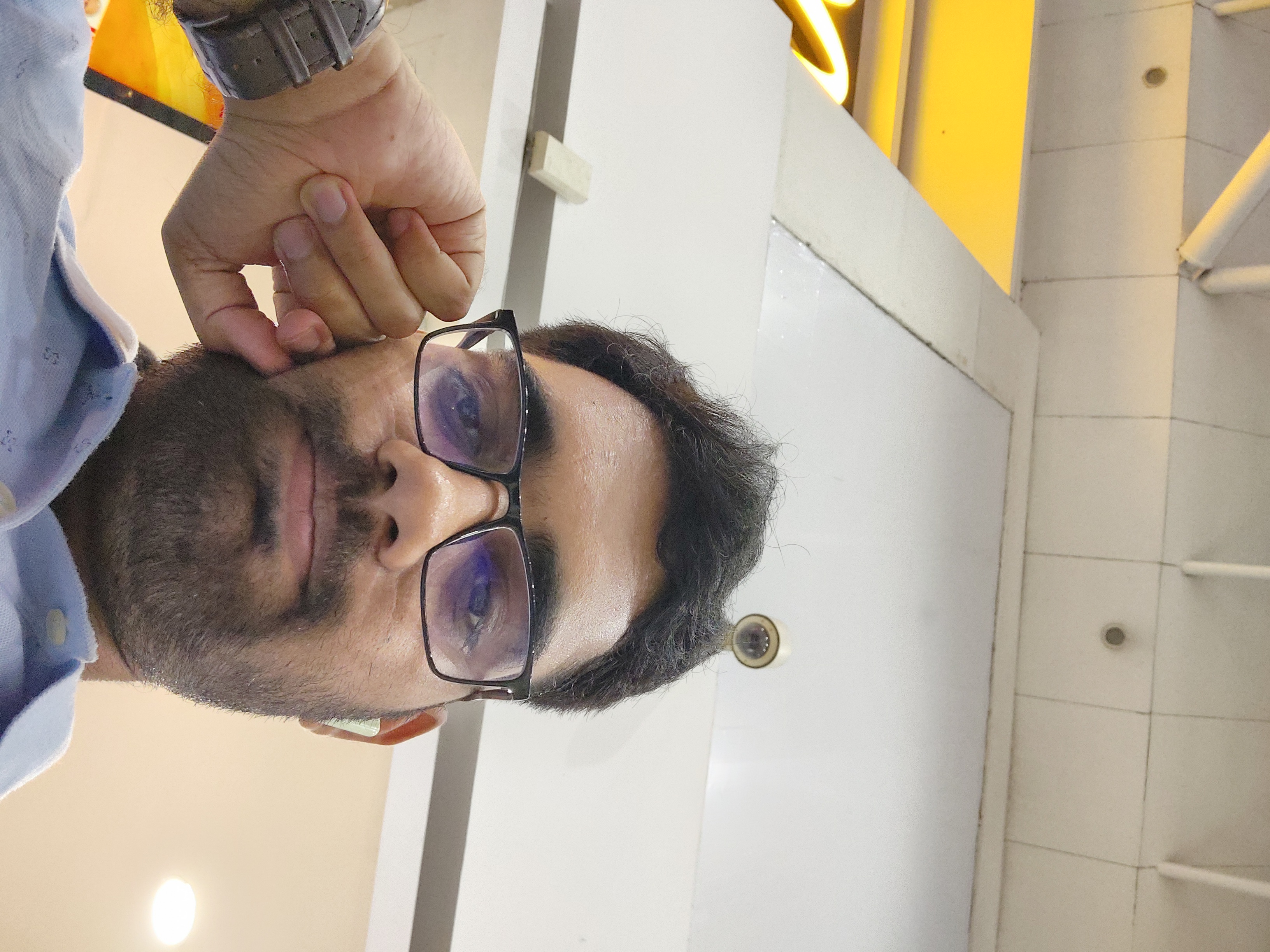
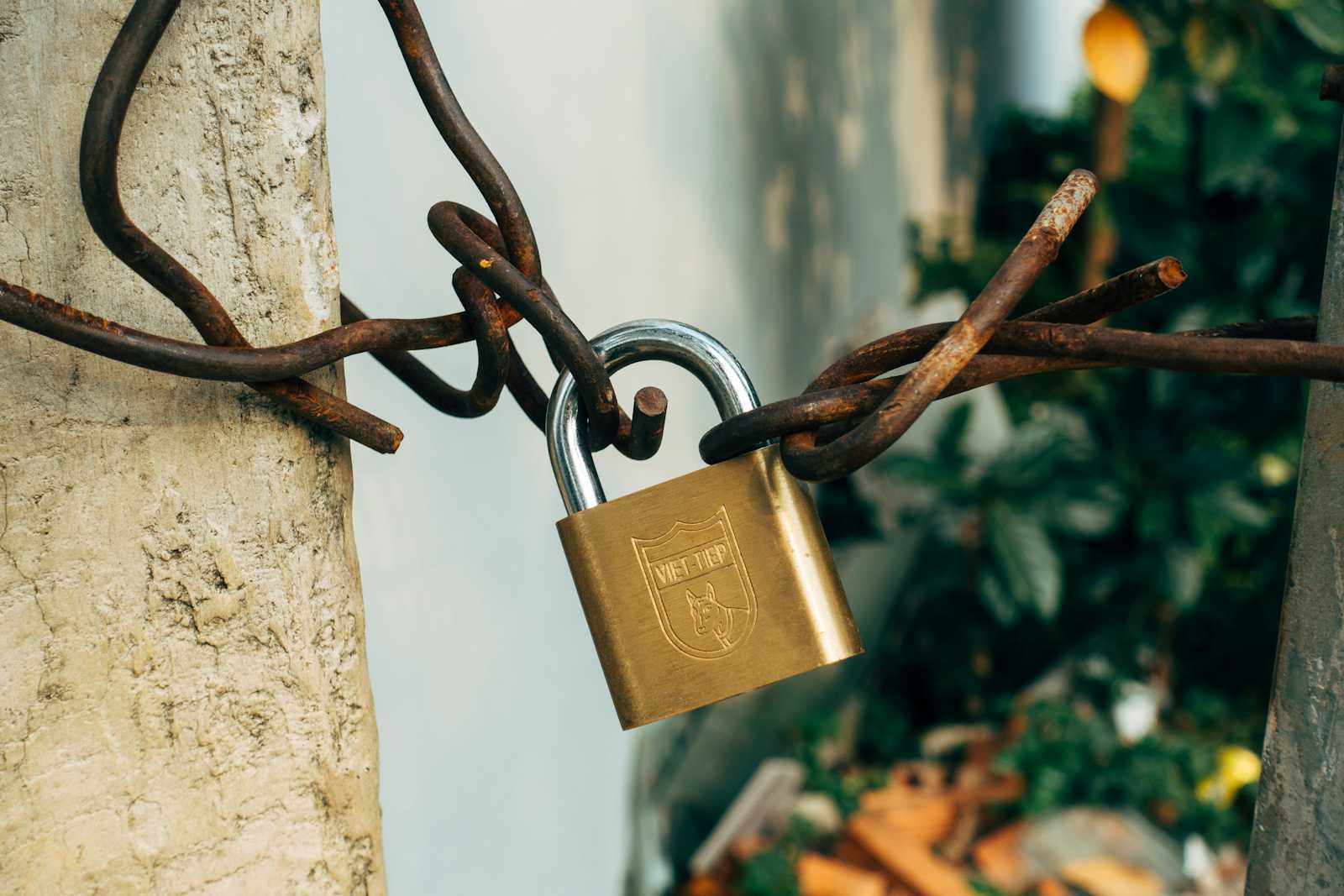
When it comes to encrypting sensitive data in Java, the choice of cipher algorithm and mode can significantly impact the security of your application. In this post, we'll explore common pitfalls associated with certain cipher modes and algorithms, and provide insights into selecting the most secure options for your encryption needs.
The Pitfalls of Weak Cipher Modes
Consider the following code snippet:
Cipher cipher = Cipher.getInstance(ALGORITHM);
While this might seem innocuous at first glance, it opens the door to potential vulnerabilities if not configured properly. Let's delve into some common pitfalls:
Weak Cipher Modes: CBC and ECB
In the realm of symmetric encryption, the Cipher Block Chaining (CBC) and Electronic Codebook (ECB) modes are notorious for their susceptibility to attacks.
CBC (Cipher Block Chaining)
CBC operates by XORing each plaintext block with the previous ciphertext block before encryption, introducing a dependency between blocks. This dependency makes CBC vulnerable to padding oracle attacks, where an attacker can decrypt the ciphertext by exploiting information leaked through padding errors. Additionally, CBC does not provide integrity protection, making it susceptible to tampering attacks.
ECB (Electronic Codebook)
ECB mode encrypts each plaintext block independently, making it vulnerable to patterns in the data. Identical plaintext blocks result in identical ciphertext blocks, which can leak information about the underlying message. ECB should be avoided in most scenarios due to its lack of security guarantees.
Padding Vulnerabilities
Padding schemes are essential for ensuring that the plaintext message is properly aligned with the block size of the cipher algorithm. However, improper padding schemes can introduce vulnerabilities. For example, using padding with CBC mode can expose the encrypted message to padding oracle attacks, as mentioned earlier.
Choosing Secure Cipher Modes
To mitigate the risks associated with weak cipher modes and padding vulnerabilities, it's crucial to opt for stronger alternatives. One such option is the Galois/Counter Mode (GCM), which provides both confidentiality and integrity protection.
GCM (Galois/Counter Mode)
GCM is a mode of operation for symmetric key cryptographic block ciphers. It combines the Counter (CTR) mode of encryption with a universal hash function for authenticated encryption and data authenticity. GCM is generally considered more computationally efficient than CBC, especially for encryption, and provides built-in integrity protection, making it resistant to tampering attacks.
Computational Analysis: AES-GCM vs. AES-CBC
A benchmark comparison between AES-GCM and AES-CBC reveals the computational efficiency of GCM over CBC, particularly for encryption tasks. GCM's parallelizable nature allows for faster encryption compared to CBC, which requires sequential decryption due to its block dependency.
Conclusion
When it comes to securing your data through encryption in Java, choosing the right cipher algorithm and mode is paramount. By steering clear of weak cipher modes like CBC and ECB, and opting for stronger alternatives like GCM, you can bolster the security of your application and safeguard against potential vulnerabilities. Remember, encryption is only as strong as its weakest link – so choose wisely to ensure the confidentiality and integrity of your sensitive data.
Subscribe to my newsletter
Read articles from Keshav Carpenter directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
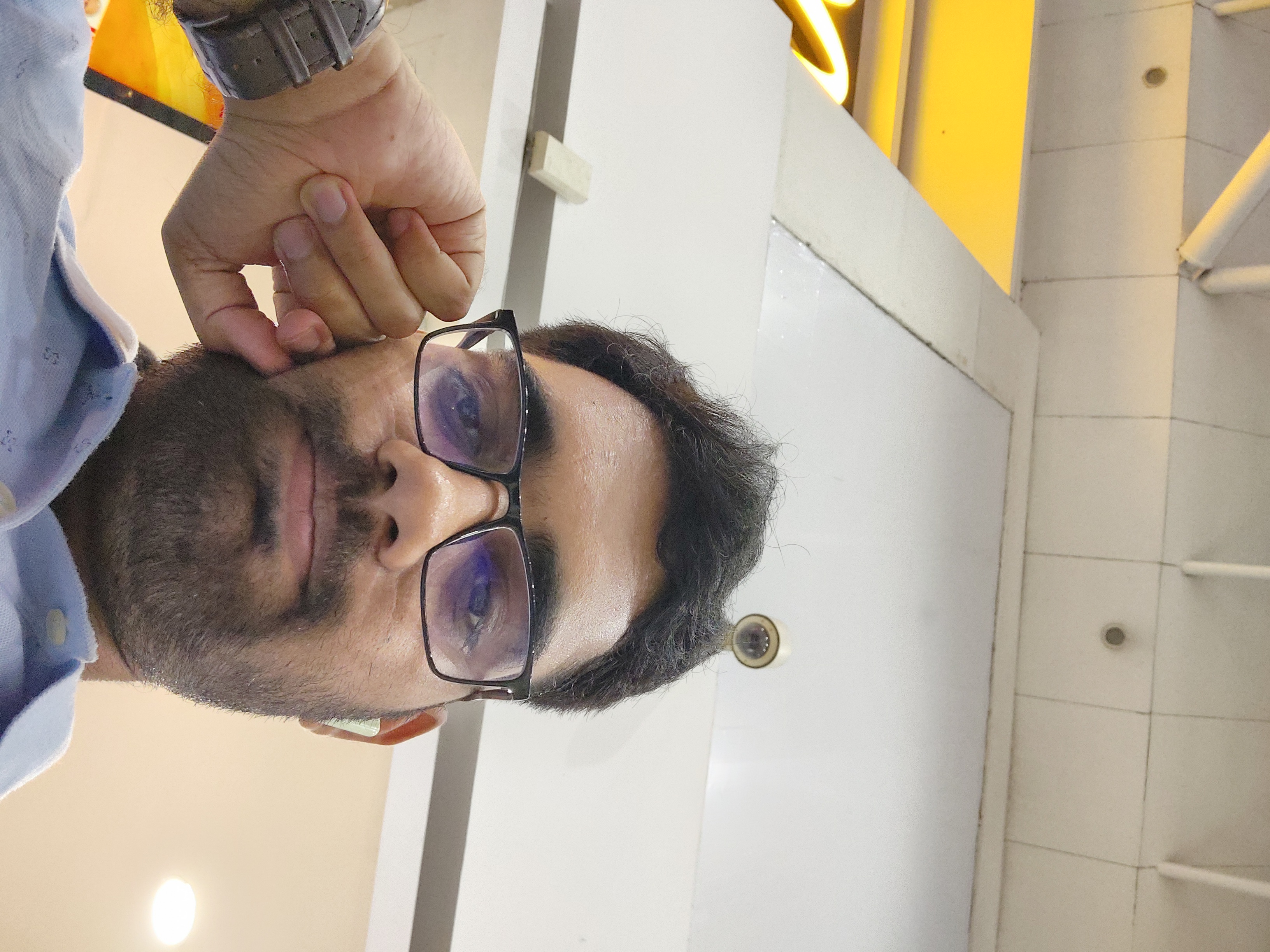
Keshav Carpenter
Keshav Carpenter
I am a software engineer with a passion for creating efficient and user-friendly web applications. I have experience in developing scalable and maintainable web applications using modern technologies and frameworks. I spent my time writing clean, robust, reusable and maintainable code.