Python Learning Journey - Day 2
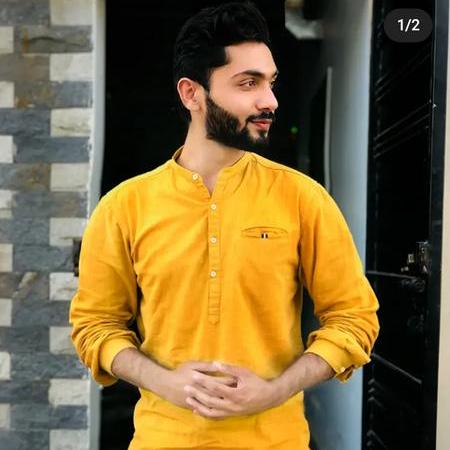
Deep Dive Guide to Learning About Data Types and Structures
Table of Contents:
Type Conversion
Type Function
Numbers
Strings
4.1 Mutable vs Immutable Data Types
4.2 Indexing vs Slicing
4.3 Creating Strings
4.4 Accessing Strings
4.5 Editing Strings
4.6 Deleting Strings
4.7 Strings Operations
4.8 Methods of Strings
4.9 Strings Programs
Lists
5.1 Lists vs Arrays
5.2 Creating Lists
5.3 Accessing Lists Elements
5.4 Editing Lists
5.5 Deleting Lists Elements
5.6 Functions of Lists
5.7 Operations on Lists
5.8 Nested Lists
5.9 Lists Programs
Conclusion
References
1. Type Conversion
Type conversion, also known as type casting, is the process of converting a value from one data type to another. This is an important concept in programming as it allows for flexibility in handling different types of data within a program. In Python, type conversions can be performed implicitly or explicitly.
- Implicit Type Conversion: Implicit type conversion, also known as coercion, is when Python automatically converts one data type to another without explicit instruction from the programmer. This usually happens when mixed-type operations are performed.
x = 10
y = 3.5
result = x + y # Implicitly converts x to float
print(result)
# Output: 13.5
Explicit Type Conversion: Explicit type conversion, also known as type casting, requires the programmer to manually convert one data type to another using built-in functions. This method gives the programmer more control over the data conversion process. Here are some common type conversions functions.
int():
Converts a value to an integer.
x = "10"
y = int(x)
print(y)
# Output: 10
float():
Converts a value to a float.
x = "10.5"
y = float(x)
print(y)
# Output: 10.5
str():
Converts a value to a string.
x = 10
y = str(x)
print(y)
# Output: "10"
list():
Converts a value to a list.
x = (1, 2, 3)
y = list(x)
print(y)
# Output: [1, 2, 3]
tuple():
Converts a value to a tuple.
x = [1, 2, 3]
y = tuple(x)
print(y)
# Output: (1, 2, 3)
2. Type Function
To verify the type of an object, use the type function. Here are some examples of type function.
x = 1
y = 3565622
z = -3255
print(type(x))
print(type(y))
print(type(z))
# Output:
<class 'int'>
<class 'int'>
<class 'int'>
x = 35e3
y = 12E4
z = -87.7e100
print(type(x))
print(type(y))
print(type(z))
# Output:
<class 'float'>
<class 'float'>
<class 'float'>
x = 3+5j
y = 5j
z = -5j
print(type(x))
print(type(y))
print(type(z))
# Output:
<class 'complex'>
<class 'complex'>
<class 'complex'>
3. Numbers
In Python, numbers are one of the most common data types, and they are used extensively in various operations and calculations. Python supports several types of numbers, including integers, floating-point numbers, and complex numbers.
Types of Numbers in Python
(i) Integers(int)
Integers are whole numbers without a decimal point.
They can be positive or negative.
x = 10
y = -5
print(type(x))
# Output: <class 'int'>
(ii) Floating-Point Numbers (float)
Floats are numbers that contain a decimal point.
They are used for more precise calculations.
a = 10.5
b = -3.14
print(type(a))
# Output: <class 'float'>
(iii) Complex Numbers (complex)
Complex numbers are numbers with a real part and an imaginary part.
They are written in the form a + bj, where a is the real part and b is the imaginary part.
c = 2 + 3j
d = -1 + 0.5j
print(type(c))
# Output: <class 'complex'>
4. Strings
String is a collection of alphabets, words or other characters. It is one of the primitive data structures and are the building blocks for data manipulation. Python has a built-in string class named "str". In python, Strings are sequence of uni code character.
4.1 Mutable vs Immutable Data types
- Mutable Data Types: Mutable objects are those whose state can be changed after they have been created. Like list, dictionaries, sets.
mylist = [1, 2, 3]
mylist[0] = 0
print(mylist)
# Output: [0, 2, 3]
- Immutable Data Types: Immutable objects are those whose state can't be changed after they have been created such as String, tuple, integers.
string_example = "Hello"
# string_example[0] = "h" # This will raise an error
new_string = string_example.lower()
print(new_string)
# Output: hello
4.2 Indexing vs Slicing
- Indexing: Accessing individual characters in a string using their position (index).
text = "Hello"
print(text[0])
# Output: H
print(text[-1])
# Output: o
- Slicing: Accessing a substring from a string using a range of indices. Remember that, slicing is inspired from indexing.
# indexing using the slicing
# [start: stop: step]
text = "Hello"
print(text[1:4])
# Output: ell
print(text[:2])
# Output: He
print(text[3:])
# Output: lo
4.3 Creating Strings:
- Strings can be created using single quotes, double quotes, or triple quotes for multi-line strings.
v= 'Ahmad Mirza'
print(v)
# Output:
# Ahmad Mirza
v="It's raining outside today"
g= '''Mirza Ahmad Awais is a passionate learner and problem solver.'''
print(v)
print(g)
# Output:
# It's raining outside today
# Mirza Ahmad Awais is a passionate learner and problem solver.
4.4 Accessing Strings
- Strings can be accessed using indexing and slicing, which we discussed earlier.
text = "Hello"
print(text[0])
# Output: H
print(text[1:4])
# Output: ell
4.5 Editing Strings
- Strings are immutable, so individual characters cannot be changed, but new strings can be created from parts of the original.
text = "Hello"
new_text = text[:2] + "y" + text[3:]
print(new_text)
# Output: Heylo
4.5 Editing Strings
- Strings are immutable, so individual characters cannot be changed, but new strings can be created from parts of the original.
text = "Hello"
new_text = text[:2] + "y" + text[3:]
print(new_text)
# Output: Heylo
4.6 Deleting Strings
- Entire strings can be deleted using the
del
statement.
text = "Hello"
del text
# print(text) # This will raise an error since text is deleted
4.7 Strings Operations
- Strings support various operations such as concatenation, repetition, and membership testing.
text1 = "Hello"
text2 = "World"
concatenated = text1 + " " + text2 # Concatenation
print(concatenated)
# Output: Hello World
repeated = text1 * 3 # Repetition
print(repeated)
# Output: HelloHelloHello
print("H" in text1) # Membership testing
# Output: True
4.8 Methods of Strings
- Strings have many built-in methods for various operations.
text = "hello world"
print(text.upper()) # Converts to uppercase
# Output: HELLO WORLD
print(text.capitalize()) # Capitalizes the first letter
# Output: Hello world
print(text.replace("world", "Python")) # Replaces substring
# Output: hello Python
print('Mirza Ahmad Awais'.swapcase())
# Output: mIRZA aHMAD aWAIS
print("'It's raning today".count(' '))
# Output: 2
4.9 String Programs
- Reversing a String:
text = "Hello"
string_reverse= text[-1::-1]
print(string_reverse)
# Output: olleH
- Palindrome Check:
text = "madam"
print(text == text[::-1])
# Output: True
5. Lists
Lists are a versatile data type in Python that can store a collection of items. These items can be of any data type and are ordered, mutable, and allow duplicate values.
5.1 Lists vs Arrays
Lists:
Can contain elements of different data types.
Part of Python's standard library.
Flexible and easy to use for general purposes.
my_list = [1, "hello", 3.14, True]
Arrays:
Typically contain elements of the same data type.
More efficient for numerical operations.
Provided by external libraries like NumPy.
import numpy as np my_array = np.array([1, 2, 3, 4])
5.2 Creating the Lists
- Lists can be created using square brackets
[]
or thelist()
constructor.
a= []
print(a)
# Output: []
from_list = list(range(5))
print(from_list)
# Output: [1,2,3,4,5]
number_list = [1, 2, 3, 4, 5]
print(number_list)
# Output: [1, 2, 3, 4, 5]
mixed_list = [1, "hello", 3.14, True]
print(mixed_list)
# Output: [1, "hello", 3.14, True]
5.3 Accessing the List Elements
- Access elements by their index, which starts from 0.
my_list = [10, 20, 30, 40, 50]
print(my_list[0])
# Output: 10
print(my_list[-1])
# Output: 50
print(my_list[0:5]) # slicing
# Output: [10, 20, 30, 40]
5.4 Editing Lists
- Lists are mutable, so you can change their elements. There are some built-in functions to edit the lists
append()
,extend()
.
my_list = [10, 20, 30, 40, 50]
my_list[1] = 25
print(my_list)
# Output: [10, 25, 30, 40, 50]
my_list[4], mylist[0:2]= 60, [80, 90]
# Output: [80, 90, 30, 40, 60]
# append method
my_list.append(60)
print(my_list)
# Output: [10, 20, 30, 40, 50, 60]
# If we want to add multiple items then use the extend function.
my_list.extend([1,2,3,4,5,6])
print(my_list)
# Output: [10, 20, 30, 40, 50, 60, [1,2,3,4,5,6]]
5.5 Deleting List Elements
- Remove elements using
del
,remove()
, orpop()
.
my_list = [10, 20, 30, 40, 50]
del my_list[2]
print(my_list)
# Output: [10, 20, 40, 50]
# When we don't know the index then use the remove
my_list.remove(20)
print(my_list)
# Output: [10, 40, 50]
# Pop Remove the last element from the list
my_list.pop()
print(my_list)
# Output: [10, 40]
5.6 Functions of Lists
- Common functions include
len()
,min()
,max()
,sum()
,sorted()
andsort()
.
my_list = [10, 20, 30, 40, 50]
print(len(my_list))
# Output: 5
print(min(my_list))
# Output: 10
print(max(my_list))
# Output: 50
print(sum(my_list))
# Output: 150
new_SortedList= sorted([5,4,3,2,1])
print(new_SortedList)
# Output: [1,2,3,4,5]
my_list.sort(reverse=True)
# Output: [50,40,30,20,10]
5.7 Operations on Lists
- Lists support concatenation, repetition, and membership testing.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
concatenated = list1 + list2
print(concatenated)
# Output: [1, 2, 3, 4, 5, 6]
repeated = list1 * 2
print(repeated)
# Output: [1, 2, 3, 1, 2, 3]
print(3 in list1)
# Output: True
print([] and "Word")
# Output: False
5.8 Nested Lists
- Lists can contain other lists, creating nested lists.
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(nested_list[0])
# Output: [1, 2, 3]
print(nested_list[0][1])
# Output: 2
# 3d lists
l3 = [[
[1,2], [3,4]],
[[6,7],[8,9]]
]
print(len(l3[-1]))
# Output: 2
5.10 List Programs
- Program to find the sum of elements in a list:
my_list = [1, 2, 3, 4, 5]
print(sum(my_list))
# Output: 15
- Program to find the largest number in a list:
my_list = [1, 2, 3, 4, 5]
print(max(my_list))
# Output: 5
- Program to reverse a list:
my_list = [1, 2, 3, 4, 5]
print((my_list[-1::-1]))
# Output: [5, 4, 3, 2, 1]
6. Conclusion
We have established the foundation for proficiency in Python by examining its foundational ideas. We now have a firm grasp on strings, lists, numerical data types, and type conversions. These basic principles give us the ability to handle and manipulate data effectively, which is an essential ability for any developer. As we continue to learn Python, keep in mind that the best ways to increase your programming skills and knowledge are to practice frequently, learn new concepts, and make use of available resources.
7. References
Happy Learning!
Subscribe to my newsletter
Read articles from Mirza Ahmad Awais directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
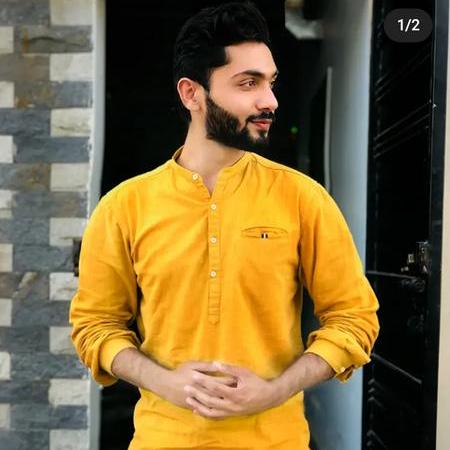