Using TypeScript in Frontend Development: Benefits and Best Practices

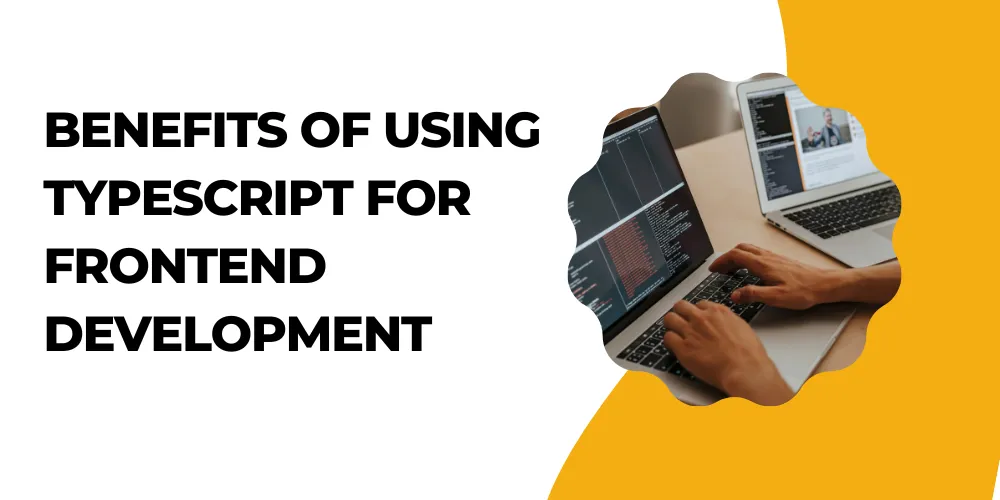
Introduction
In the fast-evolving world of frontend development, maintaining robust, maintainable, and scalable codebases is paramount. TypeScript, a superset of JavaScript, has emerged as a popular tool among developers for its ability to enhance the development experience. By introducing static types, TypeScript helps catch errors early, streamline collaboration, and improve code readability. This blog post explores the benefits of using TypeScript in frontend development and outlines best practices to maximize its potential.
Benefits of Using TypeScript
1. Enhanced Code Quality and Early Bug Detection
TypeScript's static type checking enables developers to catch errors at compile time rather than runtime. This early detection of potential issues can significantly reduce the likelihood of bugs making it to production. By defining types for variables, function parameters, and return values, TypeScript ensures that the code adheres to the expected structure and behavior.
2. Improved Developer Experience
With TypeScript, developers benefit from better tooling support, including intelligent code completion, navigation, and refactoring. These features are powered by TypeScript’s understanding of the codebase, leading to a more efficient and enjoyable development experience. IDEs like Visual Studio Code offer robust support for TypeScript, making it easier to write and maintain code.
3. Scalability and Maintainability
As projects grow in size and complexity, maintaining a JavaScript codebase can become challenging. TypeScript helps by enforcing clear interfaces and type definitions, making the code more predictable and easier to refactor. This is particularly beneficial in large teams where multiple developers are working on the same codebase, as it reduces the chances of introducing breaking changes.
4. Seamless Integration with Existing JavaScript Code
TypeScript is designed to be incremental, meaning you can gradually introduce it into an existing JavaScript project. This flexibility allows teams to adopt TypeScript at their own pace, without having to rewrite the entire codebase from scratch. TypeScript files (with the .ts
extension) can coexist with JavaScript files, enabling a smooth transition.
Best Practices for Using TypeScript in Frontend Development
1. Leverage Type Inference
TypeScript has powerful type inference capabilities, which means it can often infer the type of a variable without explicit annotations. Use type inference to reduce verbosity in your code while still benefiting from type safety. However, when the type is not obvious, provide explicit type annotations to improve readability.
let age = 30; // TypeScript infers that 'age' is a number
let name: string = 'Alice'; // Explicitly annotating the type
2. Use Interfaces and Type Aliases
Define interfaces and type aliases to describe the shape of objects and functions. This practice promotes code reuse and helps maintain consistency across your codebase.
interface User {
id: number;
name: string;
email: string;
}
const getUser = (userId: number): User => {
// Implementation
};
3. Embrace Strict Mode
Enable TypeScript's strict mode to enforce stricter type-checking rules. This setting can help catch subtle bugs and ensure more robust code.
// tsconfig.json
{
"compilerOptions": {
"strict": true
}
}
Strict mode encompasses several options, such as strictNullChecks
, noImplicitAny
, and strictFunctionTypes
, which collectively enhance type safety.
4. Utilize Utility Types
TypeScript provides a set of utility types (e.g., Partial
, Pick
, Omit
, Record
) that can simplify common type transformations. These utility types can help create more flexible and reusable type definitions.
interface User {
id: number;
name: string;
email: string;
}
type PartialUser = Partial<User>; // All properties of User are optional
5. Write Declaration Files for Third-Party Libraries
When using third-party JavaScript libraries that lack TypeScript definitions, create declaration files (.d.ts
) to describe the library's types. This practice ensures type safety when integrating external libraries into your TypeScript code.
// myLibrary.d.ts
declare module 'myLibrary' {
export function doSomething(arg: string): void;
}
6. Keep TypeScript Up-to-Date
TypeScript is actively maintained and regularly updated with new features and improvements. Stay current with the latest TypeScript version to take advantage of new capabilities and bug fixes. Regularly updating your TypeScript version ensures your code remains compatible with the latest best practices.
Conclusion
Incorporating TypeScript into your frontend development workflow can lead to significant improvements in code quality, developer productivity, and project scalability. By leveraging TypeScript's static typing, powerful tooling, and gradual adoption approach, you can build more robust and maintainable applications. Adopting best practices, such as leveraging type inference, using interfaces, embracing strict mode, utilizing utility types, writing declaration files, and keeping TypeScript up-to-date, will help you maximize the benefits of this powerful language. Whether you are starting a new project or enhancing an existing one, TypeScript is a valuable addition to your development toolkit.
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.