The Mystery of Leading Zeros in JavaScript: A Fun Discovery
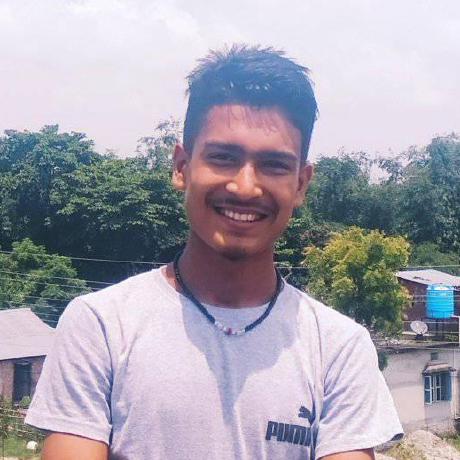
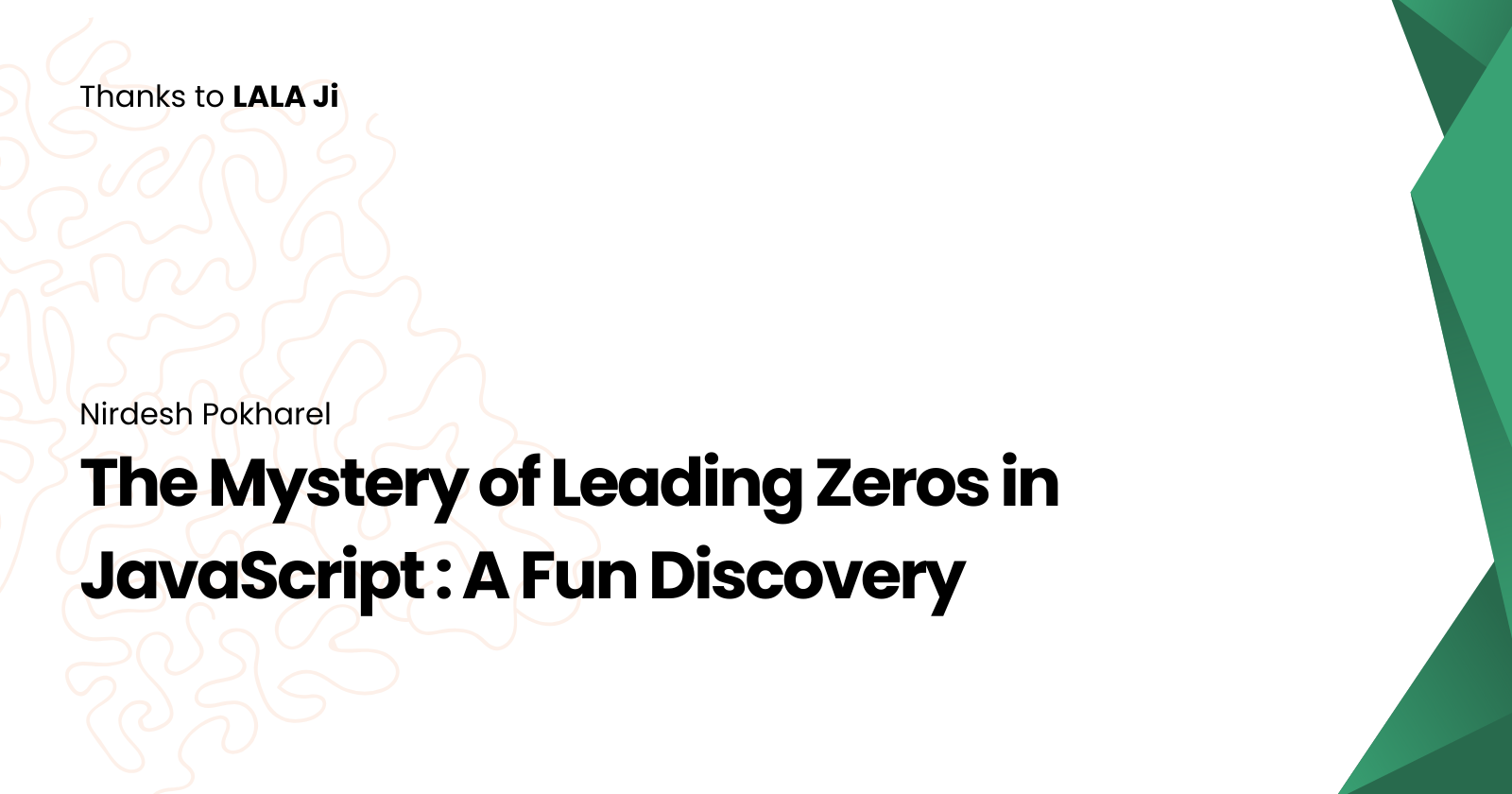
One day, our intern, Mr. Shailendra, whom we call Lala Ji, found something strange. While working on a JavaScript project, he noticed that numbers with leading zeros were acting weird. This made me curious, so I decided to look into it more. What I found was interesting and taught me a lot about JavaScript's quirks and oddities.
Why does JavaScript interpret leading zeros differently?
A long time ago, in many programming languages like C, numbers with leading zeros were treated as octal (base 8) numbers. JavaScript followed this rule too. So, when a number starts with a zero (0
), JavaScript thinks it’s an octal number.
Surprising Examples of Leading Zeros in JavaScript
Here’s what Lala Ji found:
let number = 010;
console.log(number); // Output: 8
You might think number
would be 10
, but it's actually 8
! That’s because 010
is seen as an octal number, which is 8
in decimal (base 10).
Understanding Different Number Bases in JavaScript
JavaScript also understands other bases:
Hexadecimal (base 16): Starts with
0x
or0X
.Binary (base 2): Starts with
0b
or0B
.Octal (base 8): In modern JavaScript (ES6), use
0o
or0O
.
For example:
let hex = 0x1A; // Hexadecimal 1A -> Decimal 26
let binary = 0b1010; // Binary 1010 -> Decimal 10
let octal = 0o10; // Octal 10 -> Decimal 8
How parseInt
Handles Leading Zeros in JavaScript
The parseInt
function turns strings into numbers. It can take a second argument called the radix, which tells it what base to use.
Without the radix, parseInt
might confuse you:
let number1 = parseInt('010'); // Thinks it's octal -> 8
let number2 = parseInt('10'); // Thinks it's decimal -> 10
Always give the radix to avoid confusion:
let number1 = parseInt('010', 10); // Parse as decimal -> 10
let number2 = parseInt('010', 8); // Parse as octal -> 8
Best Practices to Avoid Confusion with Leading Zeros in JavaScript
Don't Use Leading Zeros: Don’t use leading zeros for decimal numbers. Use strings if you need to pad.
let number = 10; // Correct let paddedNumber = '010'; // Use a string for padding
Use Clear Prefixes: Use
0o
for octal,0b
for binary, and0x
for hexadecimal.let octal = 0o10; // Clear octal let binary = 0b1010; // Clear binary let hex = 0x1A; // Clear hexadecimal
Use Strict Mode: Turn on strict mode to stop using old octal numbers.
'use strict'; let number = 010; // Error: Octal numbers not allowed in strict mode.
Specify Radix with
parseInt
: Always give the radix when usingparseInt
.let decimal = parseInt('10', 10); // Clear decimal let octal = parseInt('10', 8); // Clear octal
Conclusion
Thanks to Lala Ji, we learned something cool about JavaScript. Knowing these quirks helps us write better code. Always be curious and keep learning!
Happy coding!
Subscribe to my newsletter
Read articles from Nirdesh pokharel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
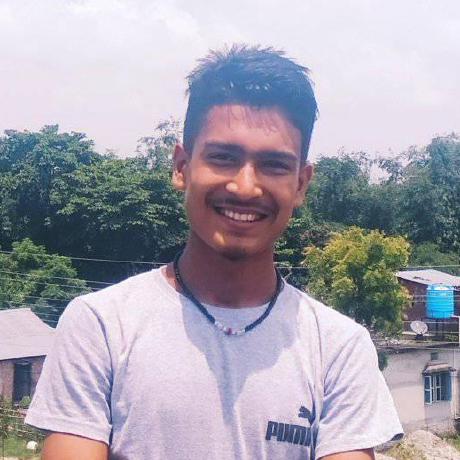