Automation Testing
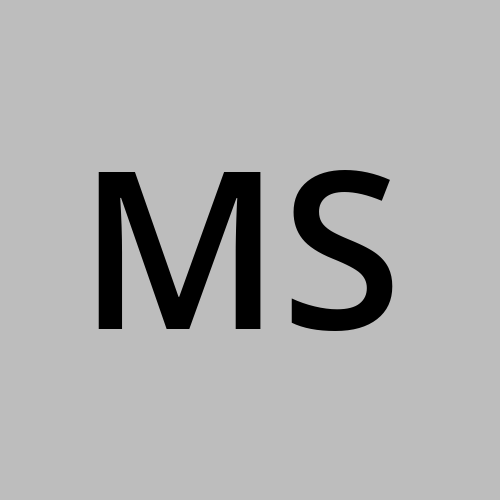
1.Explain the difference between Selenium IDE, Selenium WebDriver, and Selenium Grid
Selenium is a suite of tools designed for automating web browsers. It comprises several components, each serving different purposes in web application testing. The main components are Selenium IDE, Selenium WebDriver, and Selenium Grid. Here’s a detailed explanation of each:
Selenium IDE Integrated Development Environment (IDE)
Purpose: Selenium IDE is a simple, user-friendly tool for creating and running tests. Functionality: It provides a graphical interface for recording user interactions with the web application and playing them back. This is useful for quickly creating tests without needing to write code. Usage: Primarily used for quick, record-and-playback test creation and for generating code scripts to be used with Selenium WebDriver. Ease of Use: Very beginner-friendly, making it suitable for users who may not have extensive programming skills. Platform: It’s a browser extension available for Firefox and Chrome.
Selenium WebDriver:
Purpose: Selenium WebDriver is a programming interface that allows more advanced and granular control over web browser automation. Functionality: It interacts directly with the browser using browser-specific drivers (like ChromeDriver for Chrome, GeckoDriver for Firefox, etc.), enabling it to mimic real user interactions more accurately. Usage: Preferred for more complex test scenarios that require detailed control over browser actions. It supports multiple programming languages like Java, C#, Python, Ruby, and JavaScript. Flexibility: Highly flexible, allowing integration with various frameworks and tools for assertions, reporting, and other testing needs. Platform: Works across multiple browsers and operating systems.
Selenium Grid Distributed Testing Infrastructure
Purpose: Selenium Grid is designed to run tests on multiple machines and browsers in parallel. Functionality: It enables the distribution of tests across a network of interconnected nodes (machines), allowing for parallel execution of tests. This significantly reduces the time taken to execute a large test suite. Usage: Ideal for testing on different browser and OS combinations simultaneously. It is particularly useful in CI/CD (Continuous Integration/Continuous Deployment) environments where tests need to run quickly and efficiently. Architecture: Consists of a central hub that manages multiple nodes. The hub receives test requests and distributes them to appropriate nodes based on the requested browser/OS configuration.
2.Write a Selenium script in Java to open Google and search for "Selenium Browser Driver."
package seleniumjava;
import org.openqa.selenium.By; import org.openqa.selenium.Keys; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
public class GoogleSearchSelenium {
public static void main(String[] args) throws InterruptedException {
WebDriverManager.chromedriver().setup(); WebDriver driver = new ChromeDriver(); driver.get("https://www.google.com"); driver.manage().window().maximize();
// Find the search box using name attribute and enter the search term, then press Enter WebElement searchBox = driver.findElement(By.name("q")); searchBox.sendKeys("Selenium Browser Driver" + Keys.ENTER);
// Optional: Wait for a few seconds to observe the action (for demonstration purposes) Thread.sleep(3000);
// Close the browser driver.close(); } }
o/p: It opens Google and types Selenium Browser Driver and enters.
3.What is Selenium? How it is useful in Automation Testing?
Selenium is an open-source framework for automating web applications. It provides a set of tools and libraries to interact with web browsers, enabling developers and testers to automate the testing and interaction with web applications. Selenium supports multiple programming languages, including Python, Java, C#, and more, making it a versatile choice for automation across various platforms.
Features:
Cross-Browser Compatibility Testing: Selenium allows automation across different web browsers such as Chrome, Firefox, Safari, and Internet Explorer. This ensures that web applications are compatible and function correctly across various browsers.
Platform Independence: Selenium provides platform-independent automation, allowing testers and developers to write scripts that can run on different operating systems like Windows, macOS, and Linux. This ensures consistent behavior regardless of the underlying platform.
Support for Multiple Programming Languages: Selenium supports multiple programming languages, including Java, Python, C#, Ruby, and JavaScript. This flexibility allows automation engineers to choose the language they are most comfortable with or that best fits the project requirements.
Open-Source and Extensible: Selenium is an open-source project, making it freely available for use. The open-source nature encourages collaboration and contributions from the community, leading to regular updates and improvements. Additionally, Selenium can be extended through plugins and integrations with other tools.
Automated Testing of Web Applications: Selenium is widely used for automated testing of web applications. It allows testers to simulate user interactions with the application, perform functional testing, and validate the correctness of web elements and behaviors.
Parallel Execution: Selenium Grid enables parallel execution of tests on multiple machines and browsers simultaneously. This helps in reducing the overall test execution time, making automation more efficient.
Robust Ecosystem: Selenium has a robust ecosystem with additional tools and frameworks, such as Selenium WebDriver for browser automation, Selenium Grid for parallel execution, and various third-party libraries that enhance its capabilities.
In summary, Selenium is a powerful and versatile tool for automating web applications, providing the means to ensure the reliability, functionality, and compatibility of web-based software across different browsers and platforms.
4.What are all Browser driver used in Selenium?
Selenium WebDriver interacts with web browsers through browser-specific drivers. Each browser has its own driver, and Selenium supports a variety of browsers. Here are some of the commonly used browser drivers in Selenium:
ChromeDriver: Used for interacting with the Google Chrome browser. You need to download the ChromeDriver executable and set its path in your Selenium script. GeckoDriver (Firefox): Used for interacting with the Mozilla Firefox browser. It is required for automating Firefox with Selenium. Similar to ChromeDriver, you need to download GeckoDriver and set its path in your script. Microsoft WebDriver (Edge): Used for interacting with the Microsoft Edge browser. It is necessary for automating Edge with Selenium. The EdgeDriver is available as a separate download. InternetExplorerDriver (IE): Used for interacting with the Internet Explorer browser. It is required for automating Internet Explorer with Selenium. Note that Internet Explorer is being phased out, and Microsoft recommends using Microsoft Edge instead. SafariDriver (Safari): Used for interacting with the Safari browser. SafariDriver comes bundled with Safari, and you need to enable the “Remote Automation” option in Safari’s Develop menu to use it. OperaDriver (Opera): Used for interacting with the Opera browser. Similar to Chrome and Firefox, you need to download the OperaDriver executable and set its path in your script.
- What Are The Steps To Create A Simple Web Driver Script?Explain with code.
Step 1: Set up your development environment
Before you start, make sure you have the following components installed and set up:
Java Development Kit (JDK) Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA Selenium WebDriver Java bindings WebDriver executable (e.g., ChromeDriver for Chrome browser) Step 2: Create a Java project
Create a new Java project in your IDE.
Step 3: Add Selenium WebDriver library
Add the Selenium WebDriver library to your Java project. You can typically do this by adding the Selenium JAR files to your project’s build path.
Step 4: Write the WebDriver script
Here’s a basic example of a WebDriver script in Java that opens Google and then closes the browser:
package seldemo;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.edge.EdgeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
public class SeleniumBrowserDriver {
public static void main(String[] args) {
WebDriverManager.chromedriver().setup();
WebDriver driver = new ChromeDriver();
driver.get(“https://www.google.com/");
driver.close();
}
}
Step 5: Run the script
You can run the script from your IDE. Ensure that your browser and WebDriver versions are compatible. The script will open Google and then close the browser.
Subscribe to my newsletter
Read articles from Mourice Seraphine directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
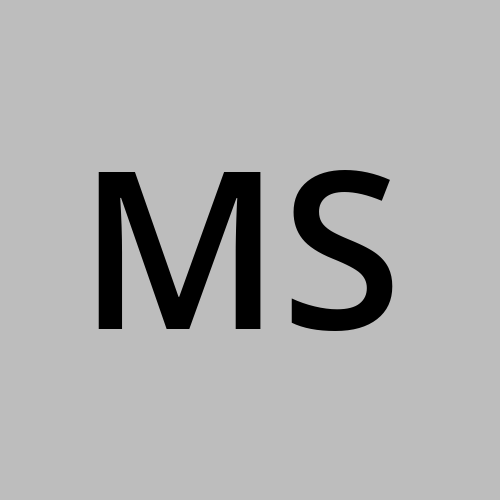