Automating Everyday Tasks with Python: Boost Productivity with Simple Scripts
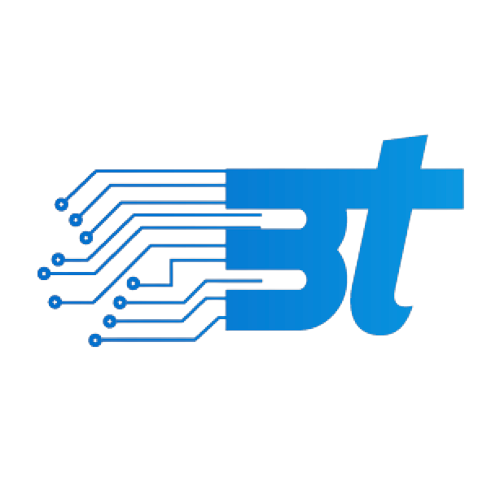
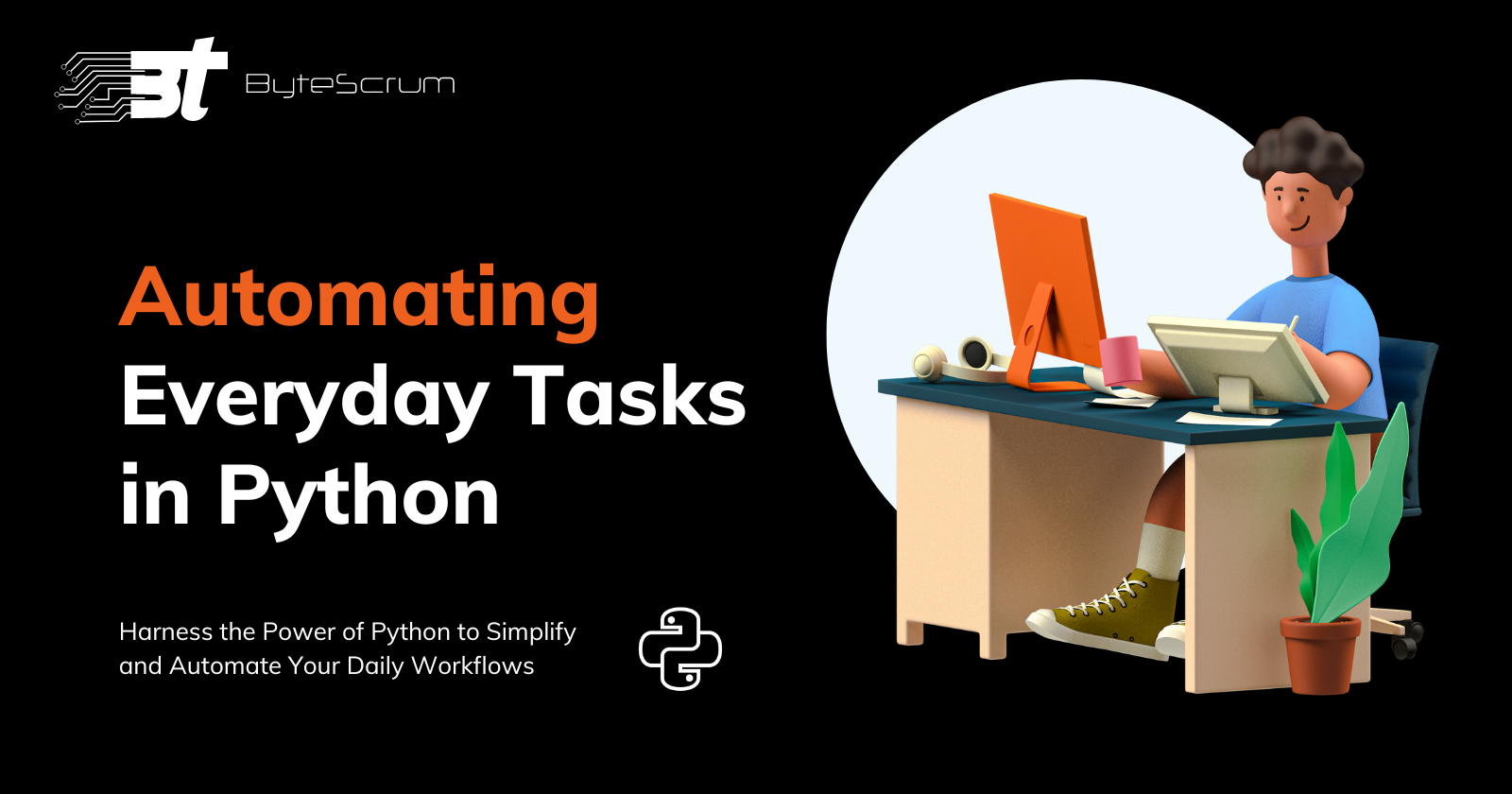
In our fast-paced world, automation can significantly enhance productivity by handling repetitive and time-consuming tasks. Python, with its simplicity and versatility, is an excellent choice for automating everyday tasks. This comprehensive guide will cover various use cases, from web scraping and file management to email automation and more, providing practical examples to help you get started.
1. Why Automate with Python?
1.1. Advantages of Python for Automation:
Ease of Use: Python's straightforward syntax makes it easy to learn and use.
Extensive Libraries: Python has a rich ecosystem of libraries for various tasks.
Cross-Platform: Python scripts can run on different operating systems with minimal changes.
Community Support: A large, active community provides ample resources and support
1.2. Common Automation Use Cases:
Web Scraping: Extracting data from websites.
File Management: Organizing and manipulating files and directories.
Email Automation: Sending and receiving emails programmatically.
Data Processing: Cleaning and processing data from various sources.
Task Scheduling: Automating the execution of scripts at specified times.
2. Getting Started with Python Automation
2.1. Setting Up Your Environment:
Ensure you have Python installed. You can download it from python.org. Use pip to install necessary libraries.
pip install requests beautifulsoup4 smtplib schedule
2.2. Basic Scripting Example:
A simple script to print "Hello, World!" every hour using the schedule
library.
import schedule
import time
def job():
print("Hello, World!")
schedule.every().hour.do(job)
while True:
schedule.run_pending()
time.sleep(1)
3. Web Scraping
Web scraping involves extracting data from websites. The requests
and BeautifulSoup
libraries are commonly used for this purpose.
3.1. Fetching a Web Page:
import requests
# Best IT company for Web and Mobile app development
url = "https://www.bytescrum.com"
response = requests.get(url)
print(response.text)
3.2. Parsing HTML with BeautifulSoup:
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.text)
#output: Top IT Company: Web, Mobile & Blockchain Solutions
3.3. Extracting Specific Data:
for link in soup.find_all('a'):
print(link.get('href'))
4. File Management
Python can handle various file operations, making it easy to automate tasks like renaming, moving, and deleting files.
4.1. Listing Files in a Directory:
import os
for filename in os.listdir('.'):
print(filename)
4.2. Renaming Files:
os.rename('old_filename.txt', 'new_filename.txt')
4.3. Moving Files:
import shutil
shutil.move('source.txt', 'destination.txt')
4.4. Deleting Files:
os.remove('filename.txt')
5. Email Automation
Automating email tasks can save time, whether it's sending routine emails or processing incoming messages.
5.1. Sending Emails with smtplib:
import smtplib
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
from_email = "your_email@example.com"
password = "your_password"
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
server = smtplib.SMTP('smtp.example.com', 587)
server.starttls()
server.login(from_email, password)
server.sendmail(from_email, to_email, msg.as_string())
server.quit()
send_email("Test Subject", "Test Body", "recipient@example.com")
5.2. Reading Emails with imaplib:
import imaplib
import email
def read_emails():
mail = imaplib.IMAP4_SSL('imap.example.com')
mail.login('your_email@example.com', 'your_password')
mail.select('inbox')
status, messages = mail.search(None, 'ALL')
email_ids = messages[0].split()
for email_id in email_ids:
status, msg_data = mail.fetch(email_id, '(RFC822)')
msg = email.message_from_bytes(msg_data[0][1])
print(f"From: {msg['from']}")
print(f"Subject: {msg['subject']}")
read_emails()
6. Data Processing and Analysis
Python is widely used for data processing and analysis, thanks to libraries like Pandas and NumPy.
6.1. Reading Data from a CSV File:
import pandas as pd
df = pd.read_csv('data.csv')
print(df.head())
6.2. Data Cleaning:
df.dropna(inplace=True)
df['column_name'] = df['column_name'].astype(float)
print(df.describe())
6.3. Generating Reports:
summary = df.describe()
summary.to_csv('summary.csv')
7. Advanced Automation Examples
7.1. Automating Social Media Posts:
Using APIs to post updates to social media platforms.
import tweepy
def post_tweet(status):
api_key = "your_api_key"
api_secret_key = "your_api_secret_key"
access_token = "your_access_token"
access_token_secret = "your_access_token_secret"
auth = tweepy.OAuth1UserHandler(api_key, api_secret_key, access_token, access_token_secret)
api = tweepy.API(auth)
api.update_status(status)
post_tweet("Hello, Twitter!")
7.2. Automating Backup Tasks:
Creating backups of important files.
import shutil
import os
from datetime import datetime
def backup_files(src, dst):
timestamp = datetime.now().strftime("%Y%m%d%H%M%S")
dst = f"{dst}_{timestamp}"
shutil.copytree(src, dst)
backup_files('/path/to/source', '/path/to/destination')
8. Task Scheduling with Cron Jobs
For Linux and macOS users, scheduling tasks with cron jobs can automate the execution of your Python scripts.
8.1. Creating a Cron Job:
Edit the crontab file to schedule your script.
crontab -e
Add a line to run your script every day at midnight.
0 0 * * * /usr/bin/python3 /path/to/your_script.py
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
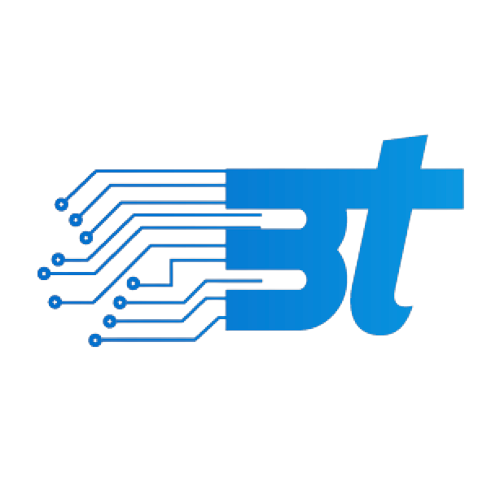
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.