Mastering Real-Time Web Development with Laravel and WebSockets
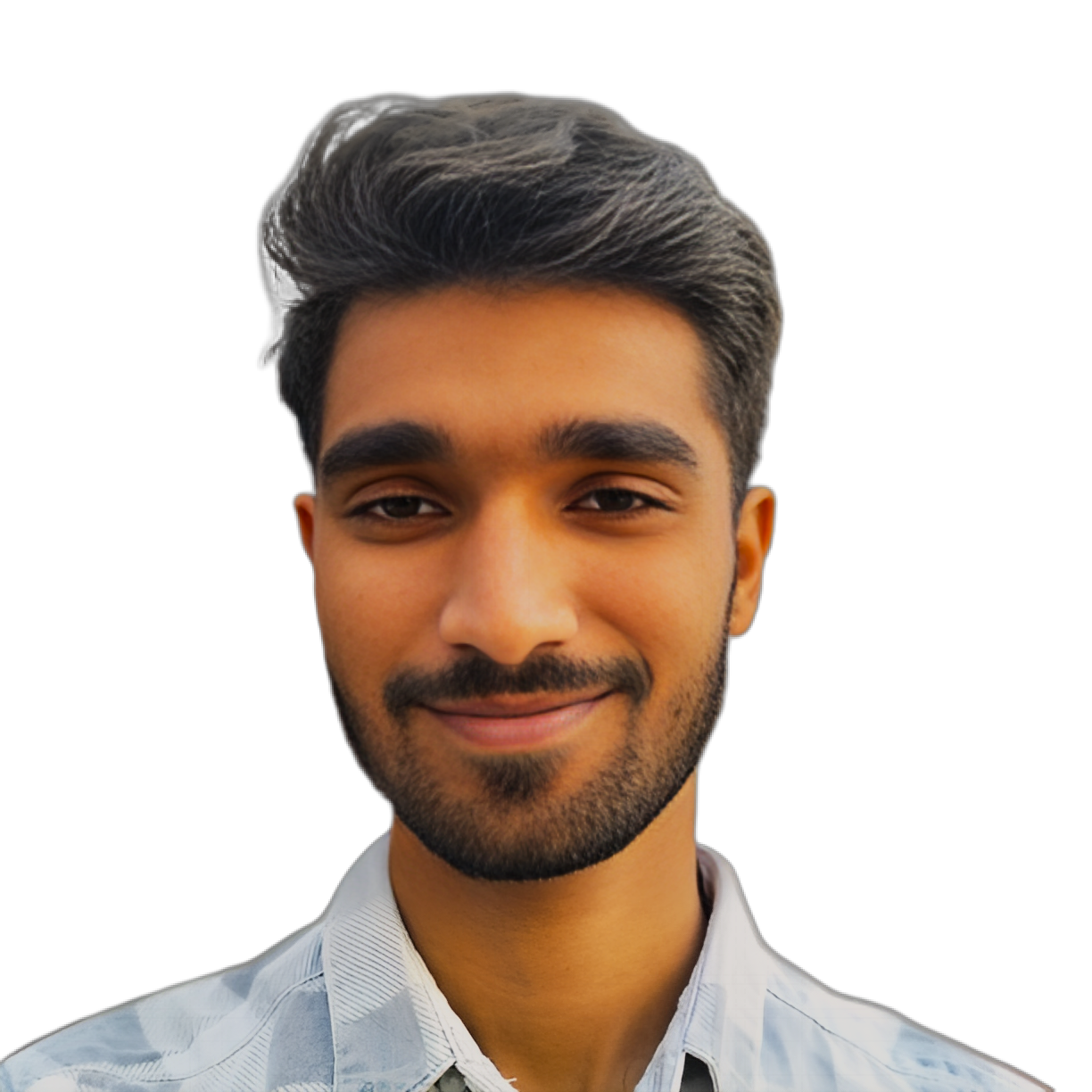
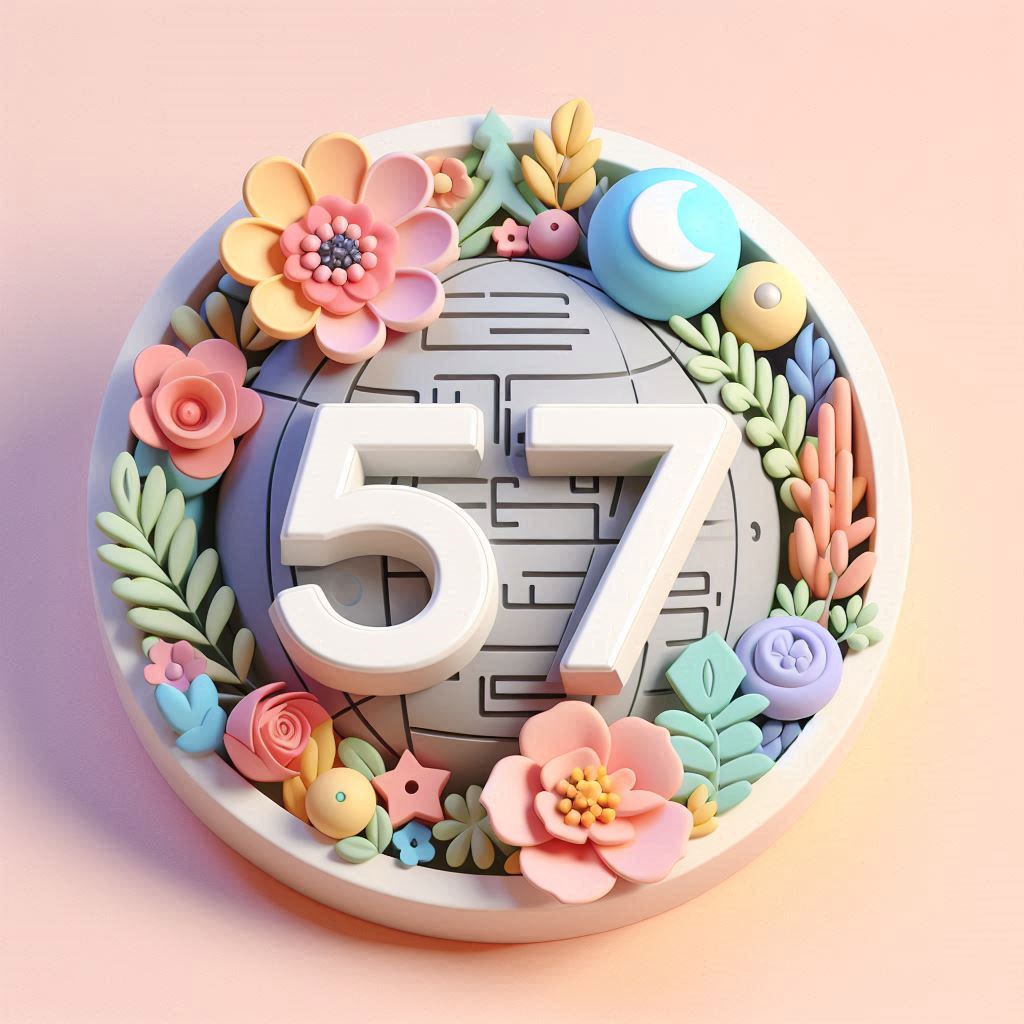
Hey there, fellow web developers! ๐
Today, we're diving into an exciting topic that's all about making your web applications more dynamic and interactive: using Laravel with WebSockets. If you've ever wanted to create real-time features like chat applications, live notifications, or instant updates, then this is the guide for you. So, grab a cup of coffee, and let's get started on this journey together!
What is Laravel?
Before we get into the nitty-gritty of WebSockets, let's do a quick recap on Laravel. Laravel is an open-source PHP framework designed to make web development easier and more efficient. With its elegant syntax, Laravel simplifies many common tasks like routing, authentication, and caching. It's like having a Swiss Army knife for web development in your toolbox.
Here's why developers love Laravel:
Elegant Syntax: Makes your code more readable and maintainable.
MVC Architecture: Separates your logic from your presentation.
Eloquent ORM: Simplifies database interactions.
Robust Ecosystem: Tons of packages and libraries available.
What are WebSockets?
Now, onto WebSockets. WebSockets provide a full-duplex communication channel over a single, long-lived TCP connection. In simpler terms, this means both the server and the client can send and receive data at any time, which is perfect for real-time applications.
Imagine you're building a chat app. With WebSockets, messages can be sent and received instantly without needing to refresh the page. Cool, right?
Why Combine Laravel with WebSockets?
Combining Laravel with WebSockets opens up a world of possibilities for creating real-time, interactive web applications. Laravel handles the backend logic while WebSockets manage the real-time data flow, giving you the best of both worlds.
Setting Up Laravel with WebSockets
Let's get our hands dirty with some code! We'll start by setting up a new Laravel project and installing the beyondcode/laravel-websockets
package.
Create a New Laravel Project:
bashCopy codecomposer create-project --prefer-dist laravel/laravel laravel-websockets-demo cd laravel-websockets-demo
Install the WebSockets Package:
bashCopy codecomposer require beyondcode/laravel-websockets
Run the WebSocket Server:
bashCopy codephp artisan websockets:serve
Great! Now we have our Laravel project set up and the WebSocket server running. Next, weโll create a WebSocket server that listens for connections and broadcasts messages to all connected clients.
Creating a WebSocket Server
We'll use the Ratchet
library to create our WebSocket server. Here's a basic implementation:
phpCopy codeuse Ratchet\MessageComponentInterface;
use Ratchet\ConnectionInterface;
class WebSocketServer implements MessageComponentInterface {
public function onOpen(ConnectionInterface $conn) {
echo "New connection! ({$conn->resourceId})\n";
}
public function onMessage(ConnectionInterface $from, $msg) {
foreach ($this->clients as $client) {
$client->send($msg);
}
}
public function onClose(ConnectionInterface $conn) {
echo "Connection {$conn->resourceId} has disconnected\n";
}
public function onError(ConnectionInterface $conn, \Exception $e) {
echo "An error has occurred: {$e->getMessage()}\n";
$conn->close();
}
}
This server will handle new connections, broadcast messages to all connected clients, and handle errors and disconnections. Pretty neat, huh?
Broadcasting Events in Laravel
Laravel makes it super easy to broadcast events using WebSockets. You can use Laravel's event broadcasting feature to broadcast server-side events to client-side listeners.
Create an Event:
phpCopy codeuse Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; class MessageSent implements ShouldBroadcast { public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat'); } }
Broadcast the Event:
phpCopy codeevent(new MessageSent('Hello, WebSockets!'));
This will broadcast the MessageSent
event to all clients listening on the chat
channel.
Building a Real-Time Chat Application
Now, letโs put everything together and build a simple real-time chat application.
Client-Side Setup:
htmlCopy code<!DOCTYPE html> <html> <head> <title>Chat Application</title> </head> <body> <div id="chat"> <ul id="messages"></ul> <input id="message" type="text" placeholder="Type a message..."> <button onclick="sendMessage()">Send</button> </div> <script> const socket = new WebSocket('ws://localhost:6001/app/my-app-key?protocol=7&client=js&version=4.3.1'); socket.onmessage = function(event) { const message = JSON.parse(event.data); const messages = document.getElementById('messages'); const messageItem = document.createElement('li'); messageItem.textContent = message; messages.appendChild(messageItem); }; function sendMessage() { const messageInput = document.getElementById('message'); const message = messageInput.value; socket.send(JSON.stringify({ message })); messageInput.value = ''; } </script> </body> </html>
Server-Side Broadcasting:
phpCopy codeevent(new MessageSent('Hello, WebSockets!'));
When you run this code, you'll have a basic chat application where messages sent from one client are instantly broadcasted to all other connected clients.
Conclusion and Next Steps
Congratulations! ๐ You've just taken your first steps into the world of Laravel and WebSockets. With these tools, you can build amazing real-time applications that provide a dynamic user experience. From here, you can explore more advanced features, optimize your WebSocket server for performance, and create more complex real-time applications.
Keep experimenting, keep coding, and most importantly, have fun with it! If you have any questions or run into any issues, feel free to reach out. Happy coding! ๐
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
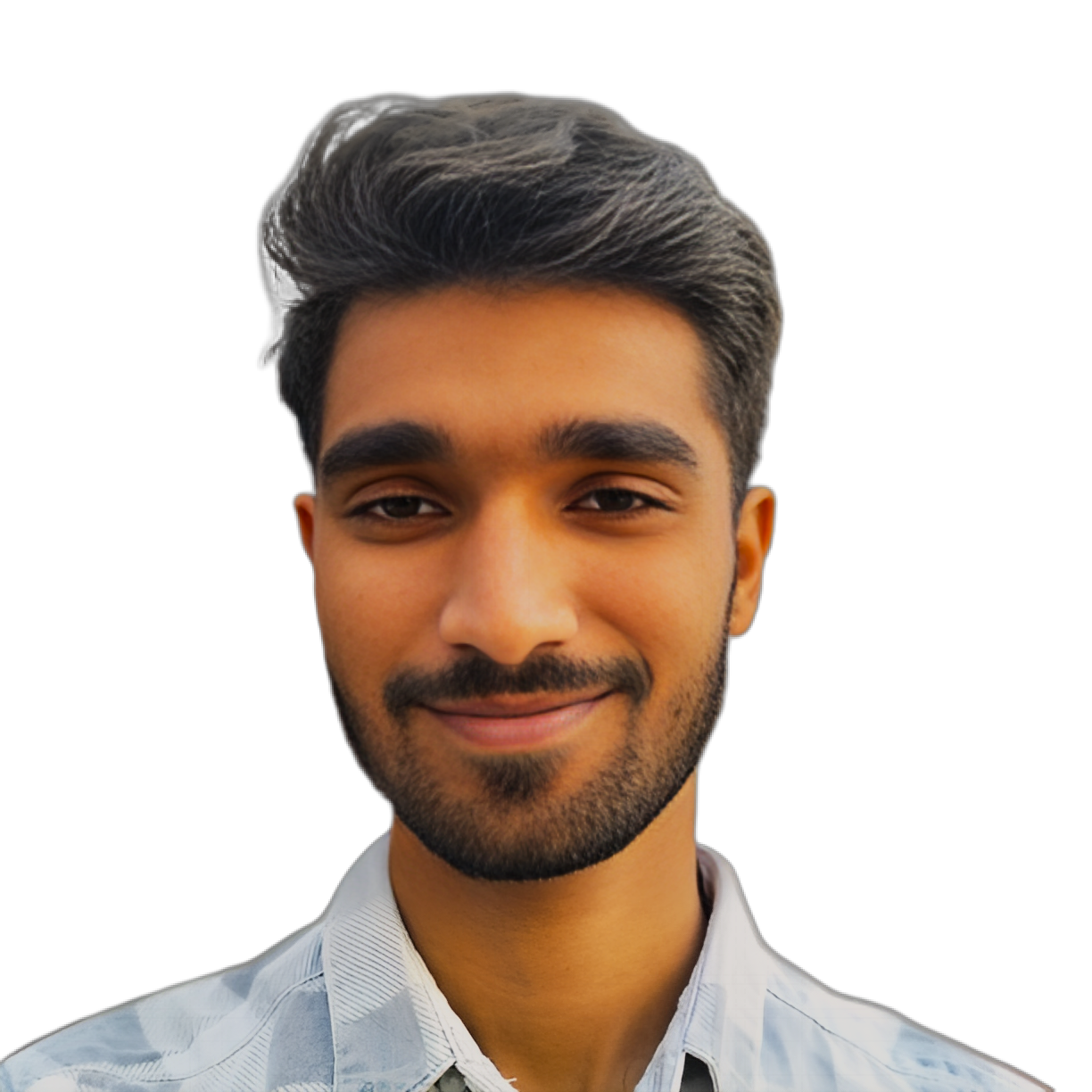
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.