🚀Doctor Strange's Guide to Collections in Java🧙♂️✨
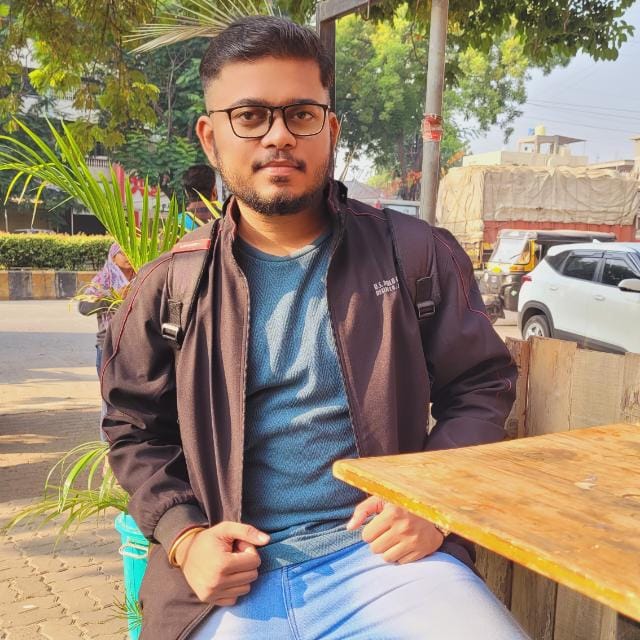
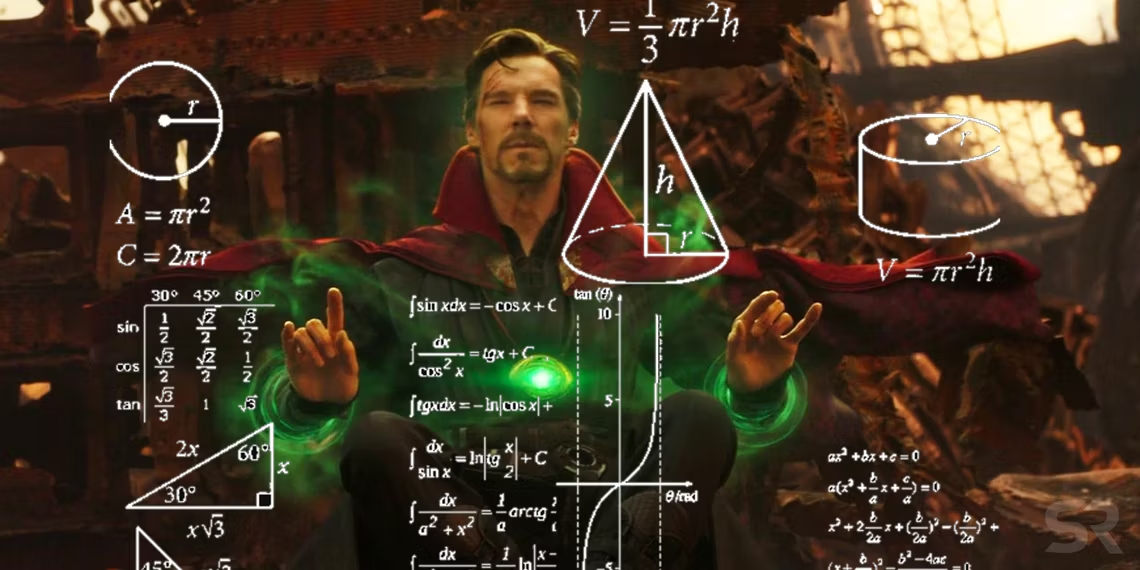
Greetings, fellow coders! 🚀
Today, we're diving into the mystical realm of Java Collections Framework, guided by none other than the Sorcerer Supreme himself, Doctor Strange!
🌀 Let's explore List, Set, and Map through the enchanting world of spells, artifacts, and portals.
👨💻 Ready to cast some coding magic? 🌟
🔮 Library of Spells
List Imagine having access to the Sanctum Sanctorum's library filled with ancient and powerful spells!
📚 In Java, we can use a List to store these spells. Lists maintain the order of insertion and allow duplicates, making them perfect for our spell collection.
📋 Lists are great for keeping things in order, just like how Doctor Strange meticulously organizes his Spell-Book! 🪄
🏺Artifacts of the Sanctum Sanctorum
Set Next, let's look at the unique and powerful artifacts housed in the Sanctum Sanctorum.
🏺 To ensure no duplicates, we use a Set. Sets are perfect for collections where each item must be unique.
🔗 Sets ensure that each artifact is one-of-a-kind, just like the mystical items Doctor Strange relies on! 🔮
🌌 Portal Coordinates💫
Map Lastly, let's navigate through the multiverse using portals!
🌌 A Map is perfect for storing portal coordinates, mapping dimension names to their specific locations.
🗺️ Maps are ideal for handling key-value pairs, ensuring Doctor Strange can always find his way through the multiverse! 🌠
✨ Bonus:
Sorting the Collections! Just like organizing spells and artifacts, sorting can be magical too!
🧹 Using Collections.sort(), we can keep our lists neat and ready for any adventure.
🔮 Sorting helps keep our collections organized, ready to face any mystical threat that comes our way! 🧙♂️📜
Embrace the magic of Java Collections and may your code be as powerful as the Sorcerer Supreme! 💻🔮
#Java #Coding #CollectionsFramework #DoctorStrange #Programming #Hashnode #Medium #Tech #Automation #SoftwareEngineering #ArtificialIntelligence
🚀🚀Full Code Here🚀🚀
import java.util.*;
public class DoctorStrangeCollections {
public static void main(String[] args) {
// List - Sanctum Sanctorum's Library of Spells
List spells = new ArrayList<>();
spells.add("Winds of Watoomb");
spells.add("Crimson Bands of Cyttorak");
spells.add("Vapors of Valtorr");
spells.add("Bolts of Bedevilment");
System.out.println("Library of Spells:");
for (String spell : spells) {
System.out.println(spell);
}
// Set - Artifacts of the Sanctum Sanctorum (No duplicates allowed)
Set artifacts = new HashSet<>();
artifacts.add("Eye of Agamotto");
artifacts.add("Cloak of Levitation");
artifacts.add("Book of Vishanti");
artifacts.add("Wand of Watoomb");
artifacts.add("Eye of Agamotto"); // Duplicate entry, won't be added
System.out.println("\nArtifacts in the Sanctum Sanctorum:");
for (String artifact : artifacts) {
System.out.println(artifact);
}
// Map - Portal coordinates to different dimensions
Map<String, String> portals = new HashMap<>();
portals.put("Dark Dimension", "13°N, 77°E");
portals.put("Mirror Dimension", "23°S, 45°W");
portals.put("Astral Dimension", "0°, 0°");
portals.put("Dark Dimension", "14°N, 76°E"); // Overwriting previous entry
System.out.println("\nPortal Coordinates:");
for (Map.Entry<String, String> portal : portals.entrySet()) {
System.out.println(portal.getKey() + ": " + portal.getValue());
}
// Extra - Using Collections methods
Collections.sort(spells);
System.out.println("\nSorted Library of Spells:");
for (String spell : spells) {
System.out.println(spell);
}
List sortedArtifacts = new ArrayList<>(artifacts);
Collections.sort(sortedArtifacts);
System.out.println("\nSorted Artifacts in the Sanctum Sanctorum:");
for (String artifact : sortedArtifacts) { System.out.println(artifact);
}
}
}
Happy coding, fellow sorcerers! 🧙♂️✨
Subscribe to my newsletter
Read articles from Rishikesh Vajre directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
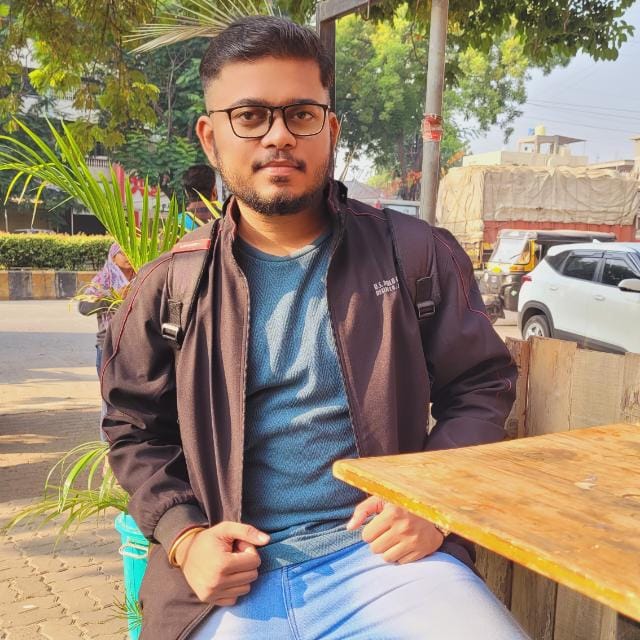
Rishikesh Vajre
Rishikesh Vajre
Software Development Engineer in Test (SDET) | Automation Expert | Quality Assurance Specialist | Java | Selenium | REST API Testing