Time and Space Complexity Explained Simply
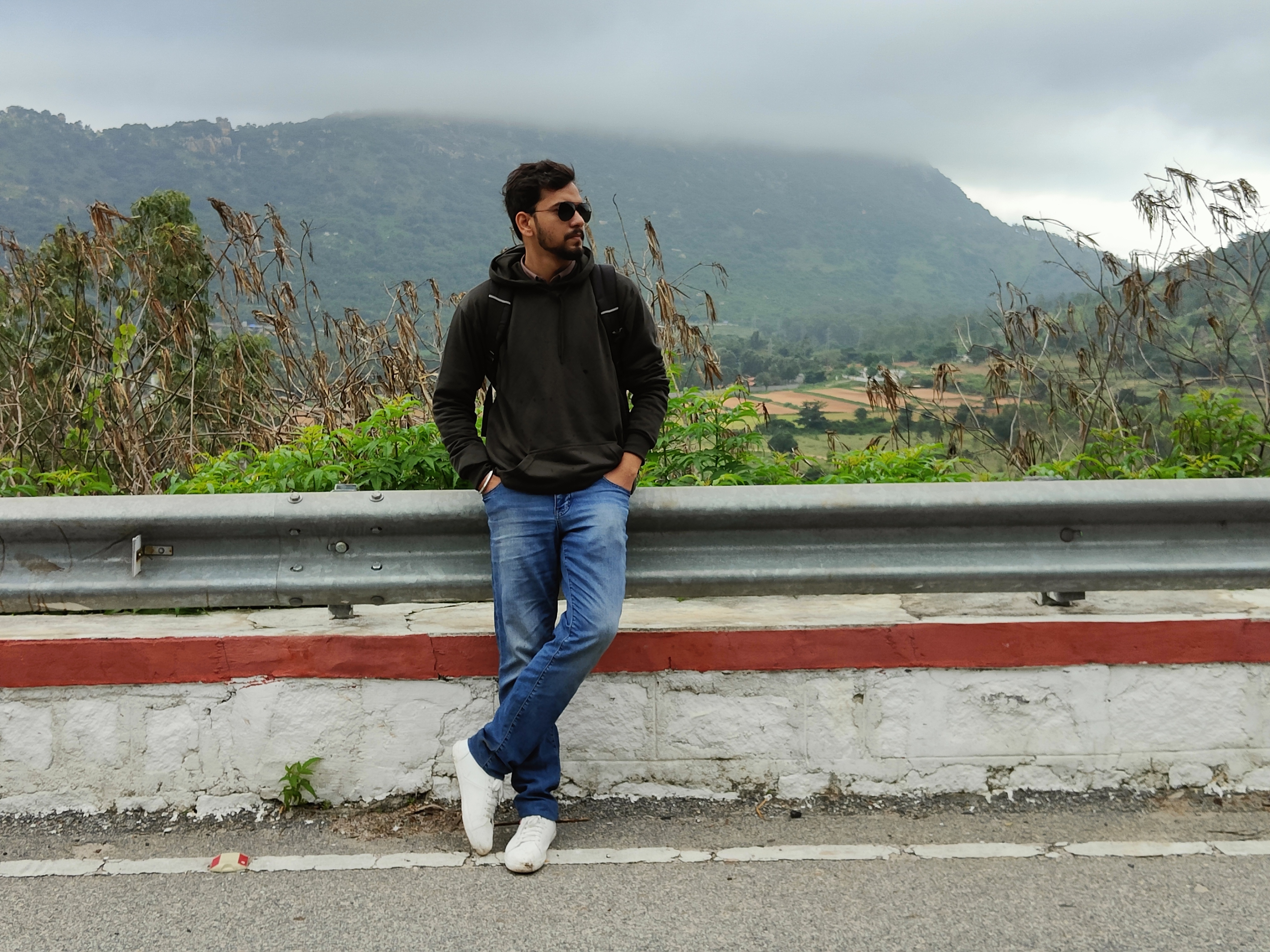
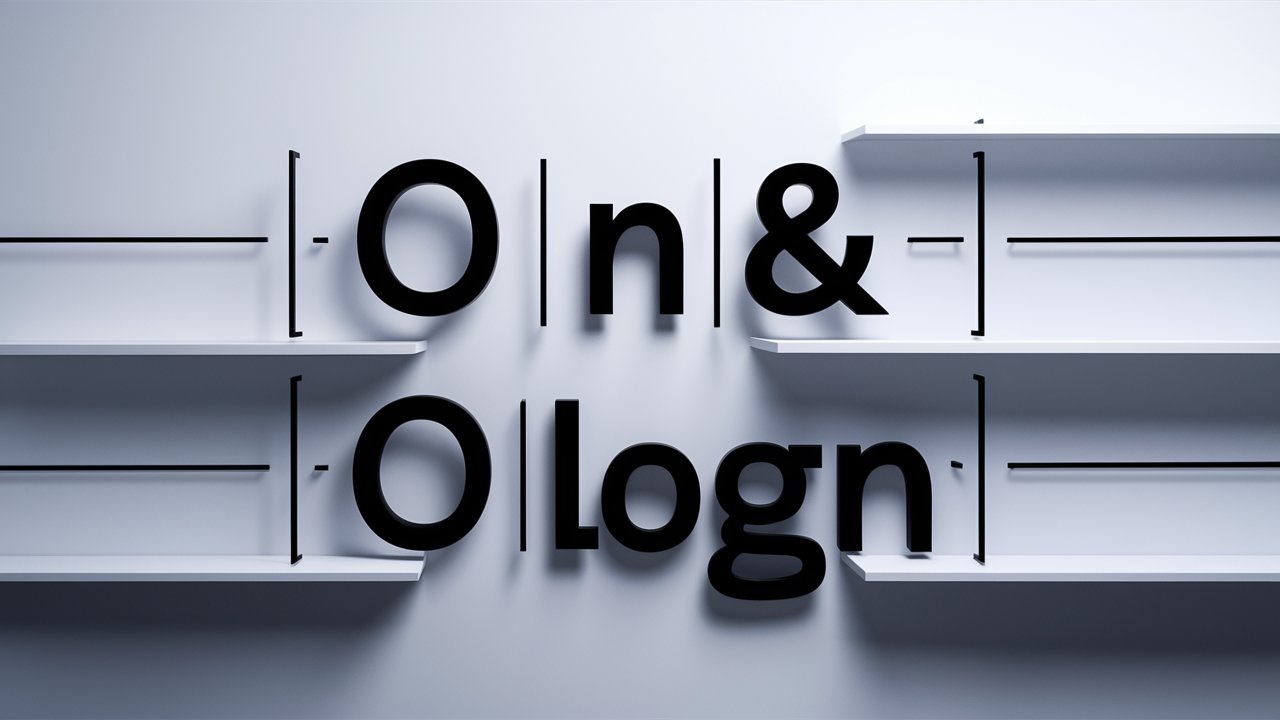
Understanding time and space complexity is fundamental in analyzing the efficiency of algorithms. Below we will explore the concepts of time and space complexity, their common notations, and provide examples in C++ to illustrate these concepts.
Why do we need to study time and space complexity?
Performance Analysis:
Understanding the time and space complexity of an algorithm helps in evaluating its performance. It allows you to predict how the algorithm will behave as the size of the input grows, which is essential for ensuring that applications run efficiently and do not consume excessive resources.
Optimization:
By analyzing the complexity, you can identify bottlenecks and optimize the code to improve its efficiency. This might involve choosing a more appropriate algorithm or data structures that offer better performance for the given problem.
Resource Management:
Efficient use of resources (CPU time and memory) is critical, especially in environments with limited resources. Analyzing complexity helps in writing code that is both time-efficient and space-efficient, ensuring optimal resource utilization.
Studying time and space complexity equips you with the tools to write efficient, scalable, and, resource-conscious code. It helps in making informed decisions about algorithms and data structure selection, ultimately leading to better software design and performance.
Unit to Represent Complexity:
Big O Notation (Upper Bound)
Big O Notation describes the upper bound of the time or space complexity. It gives the worst-case scenario, providing an upper limit on the growth rate of an algorithm.
Omega Notation (Lower Bound)
Omega Notation describes the lower bound of the time or space complexity. It gives the best-case scenario, providing a lower limit on the growth rate of an algorithm.
Theta Notation (Average Case)
Theta Notation describes the exact bound of the time or space complexity. It provides both an upper and lower limit on the growth rate of an algorithm, indicating the exact asymptotic behavior.
Time Complexity:
Time Complexity is a measure used in Computer Science to describe the amount of time it takes to run an algorithm as a function of the length of the input. It gives an estimate of the number of basic operations an algorithm performs and how this number grows with the size of the input.
Common Time Complexities:
Constant Time - O(1):
The time taken by the algorithm is constant and does not change with the size of the input.
Logarithmic Time - O(log n):
The time complexity grows logarithmically with the input size. Algorithms that have the input size at each step typically have logarithmic time complexity.
Linear Time - O(n):
The time taken grows linearly with the size of the input.
Linearithmic Time - O(n log n):
The time complexity grows in proportion to the input size times the logarithm of the input size.
Quadratic Time - O(n²):
The time taken grows proportionally to the square of the size of the input. Often found in algorithms that involve nested loops over the input.
Cubic Time - O(n³):
The time taken grows proportionally to the cube of the size of the input. This is often seen in algorithms with three nested loops over the input.
Exponential Time - O(2^n):
The time taken grows exponentially with the size of the input. This is often seen in algorithms that solve problems by trying every possible solution.
For example:
O(n) :
O(n^2):
Currently, we are focusing on O(n) and O(n^2) complexities. As we progress and explore various algorithms, we will delve deeper into different types of complexities. Each algorithm employs distinct time and space complexities, which often involve data structures such as stacks, queues, and strings. Discussing these complexities now may be challenging to understand without a foundational knowledge of these data structures.
Space Complexity:
Space complexity is a measure of the amount of working storage an algorithm needs. It accounts for both the space used by the input and the auxiliary space required for the computation. Understanding space complexity is crucial for optimizing algorithms to ensure they run efficiently within the available memory constraints.
Components of Space Complexity:
Fixed Part: This includes space required for constants, simple variables, fixed-size structured variables, etc. This part of the space requirement is independent of the size of the input and is typically constant.
Variable Part: This includes space required for dynamic memory allocation and dynamically allocated variables. This part of the space requirement depends on the size of the inputs.
Common space Complexity:
O(1) - Constant Space: The algorithm uses a fixed amount of memory regardless of the input size.
O(n) - Linear Space: The algorithm memory usage grows linearly with the input size.
O(n^2) - Quadratic Space: The memory usage grows quadratically with the input size.
For example:
int a = 5 Fixed part, if int takes 4 bytes, a takes 20 bytes of storage.
int b[n] Variable part, it depends on user input.
Space complexity is a vital aspect of algorithm analysis, complementing time complexity to provide a full picture of an algorithm's efficiency. It is particularly important in environments with limited memory resources, ensuring that algorithms run within the available memory constraints while maintaining performance. Understanding space complexity allows for better optimization and more efficient algorithm design.
Subscribe to my newsletter
Read articles from Rishabh Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
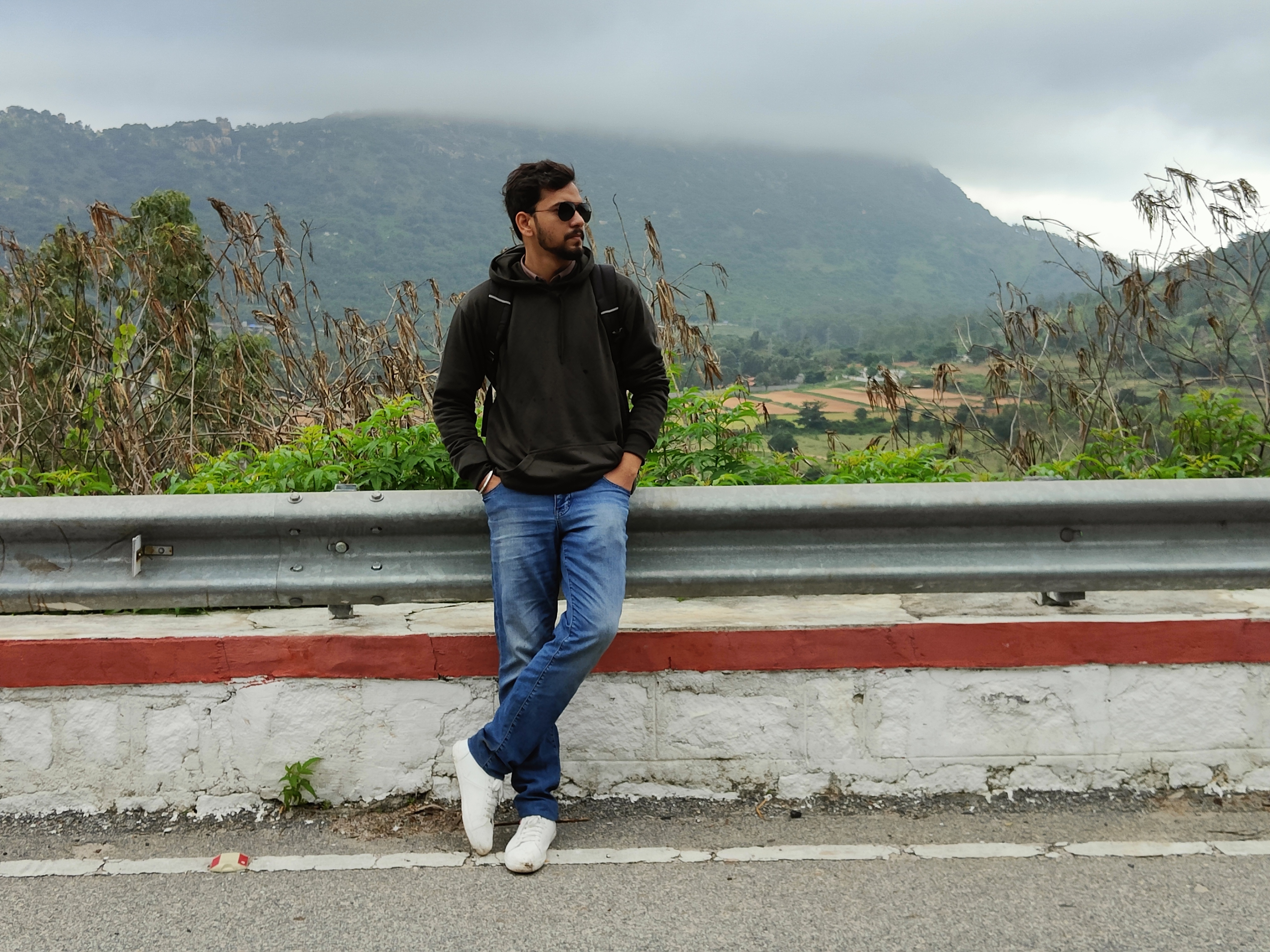