Data Poisoning in AI
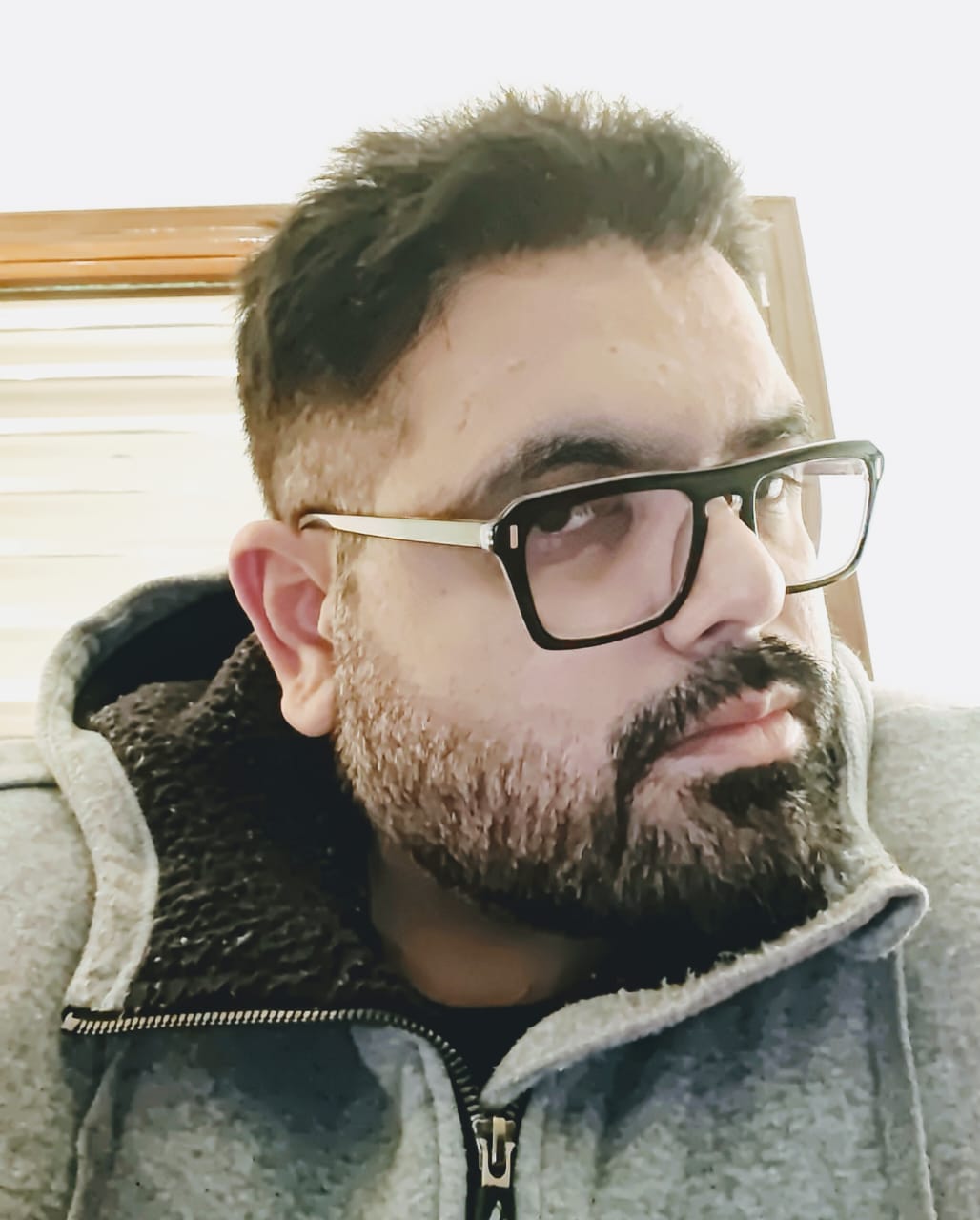
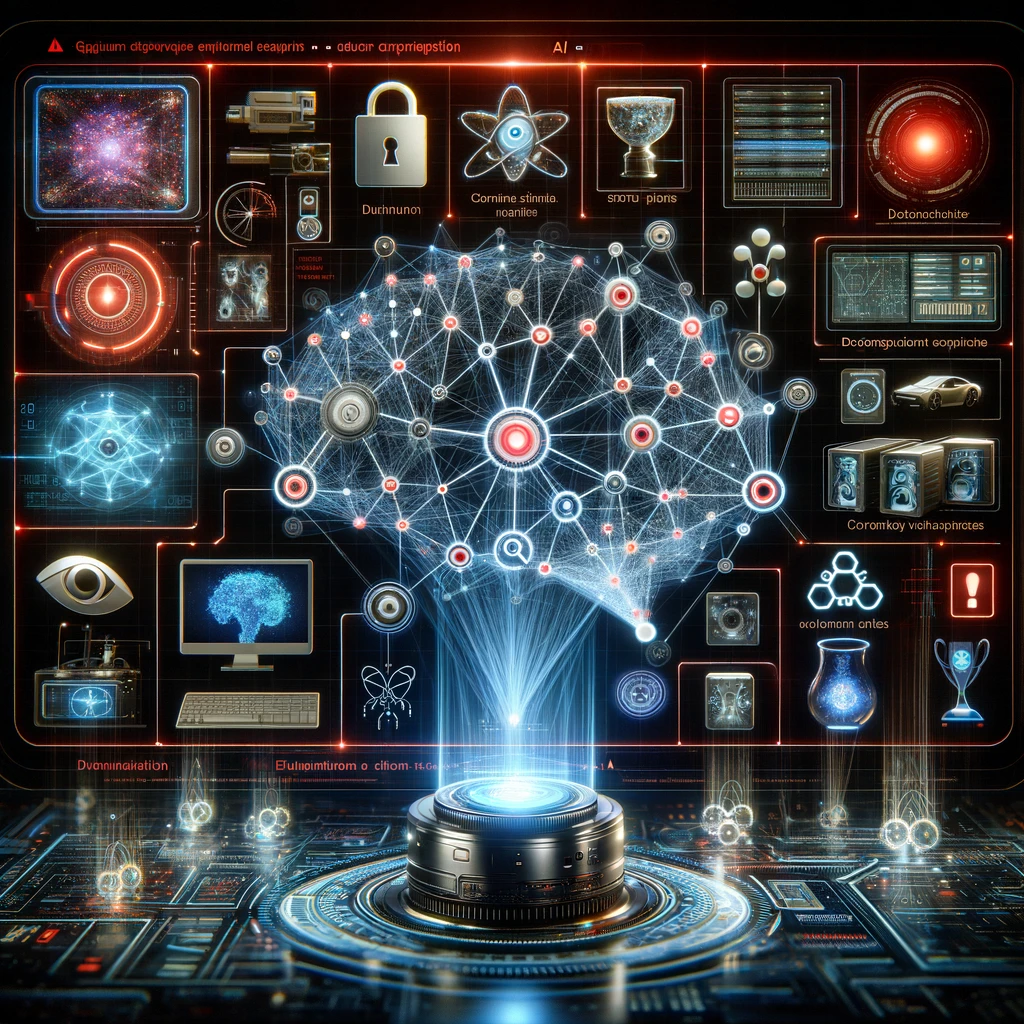
As Artificial Intelligence (AI) systems become more prevalent in various sectors, the integrity of the data used to train these systems is paramount. One of the emerging threats to AI models is data poisoning, a malicious attack that aims to corrupt the training data, leading to compromised AI models. This article explores the concept of data poisoning, its implications for information security, and practical measures to mitigate its effects, complete with code examples and real-life scenarios.
What is Data Poisoning?
Data poisoning involves intentionally injecting misleading or false data into the training dataset of an AI model. The goal is to manipulate the model's output, causing it to make incorrect predictions or classifications. This type of attack can have severe consequences, especially in critical applications such as healthcare, finance, and autonomous systems.
Real-Life Scenario: Data Poisoning in Autonomous Vehicles
Consider an autonomous vehicle relying on AI models for object detection and navigation. If an attacker manages to poison the training data with incorrect labels (e.g., labeling stop signs as yield signs), the AI model might fail to recognize stop signs accurately, leading to potentially dangerous situations on the road.
Code Example: Simulating Data Poisoning
Here's a simple example of how data poisoning can affect a machine learning model. We'll use a basic dataset and introduce poisoned data to see its impact.
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Generate a synthetic dataset
X, y = make_classification(n_samples=1000, n_features=20, random_state=42)
# Introduce poisoning by flipping the labels of a portion of the data
def poison_data(X, y, poison_ratio=0.1):
num_poison = int(poison_ratio * len(y))
poisoned_indices = np.random.choice(len(y), num_poison, replace=False)
y_poisoned = np.copy(y)
y_poisoned[poisoned_indices] = 1 - y_poisoned[poisoned_indices]
return X, y_poisoned
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Poison the training data
X_train_poisoned, y_train_poisoned = poison_data(X_train, y_train, poison_ratio=0.1)
# Train a logistic regression model on clean and poisoned data
model_clean = LogisticRegression()
model_poisoned = LogisticRegression()
model_clean.fit(X_train, y_train)
model_poisoned.fit(X_train_poisoned, y_train_poisoned)
# Evaluate the models
y_pred_clean = model_clean.predict(X_test)
y_pred_poisoned = model_poisoned.predict(X_test)
print("Accuracy on clean data:", accuracy_score(y_test, y_pred_clean))
print("Accuracy on poisoned data:", accuracy_score(y_test, y_pred_poisoned))
# Plotting the impact
plt.bar(['Clean Model', 'Poisoned Model'], [accuracy_score(y_test, y_pred_clean), accuracy_score(y_test, y_pred_poisoned)])
plt.ylabel('Accuracy')
plt.title('Impact of Data Poisoning on Model Accuracy')
plt.show()
Importance of Data Poisoning in Information Security
In the context of interconnected devices (IoT), cyber threats, and the rise of quantum computing, data poisoning poses a significant risk to the integrity and reliability of AI systems. Protecting against such attacks is crucial to maintain trust and ensure the safe deployment of AI technologies.
Mitigation Strategies
Robust Data Validation: Implement rigorous data validation processes to detect and filter out anomalous data.
Anomaly Detection: Use AI-driven anomaly detection techniques to identify and mitigate suspicious patterns in training data.
Differential Privacy: Incorporate differential privacy techniques to add noise to the training data, making it difficult for attackers to manipulate the dataset without being detected.
Model Robustness: Develop models that are robust to minor changes in the training data, reducing the impact of poisoned data.
Real-Life Scenario: Healthcare
In the healthcare sector, AI models are used for diagnosing diseases based on medical imaging. Data poisoning in this context could lead to incorrect diagnoses, affecting patient care. Ensuring the integrity of training data is crucial to maintaining the accuracy and reliability of these models.
Conclusion
As AI continues to integrate into various aspects of our lives, ensuring the integrity of the training data is paramount to maintaining robust and reliable AI systems. Data poisoning is a critical threat that must be addressed through comprehensive security measures and advanced detection techniques. By understanding and mitigating data poisoning, we can safeguard information security in today's interconnected, AI-driven world.
Subscribe to my newsletter
Read articles from Rai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
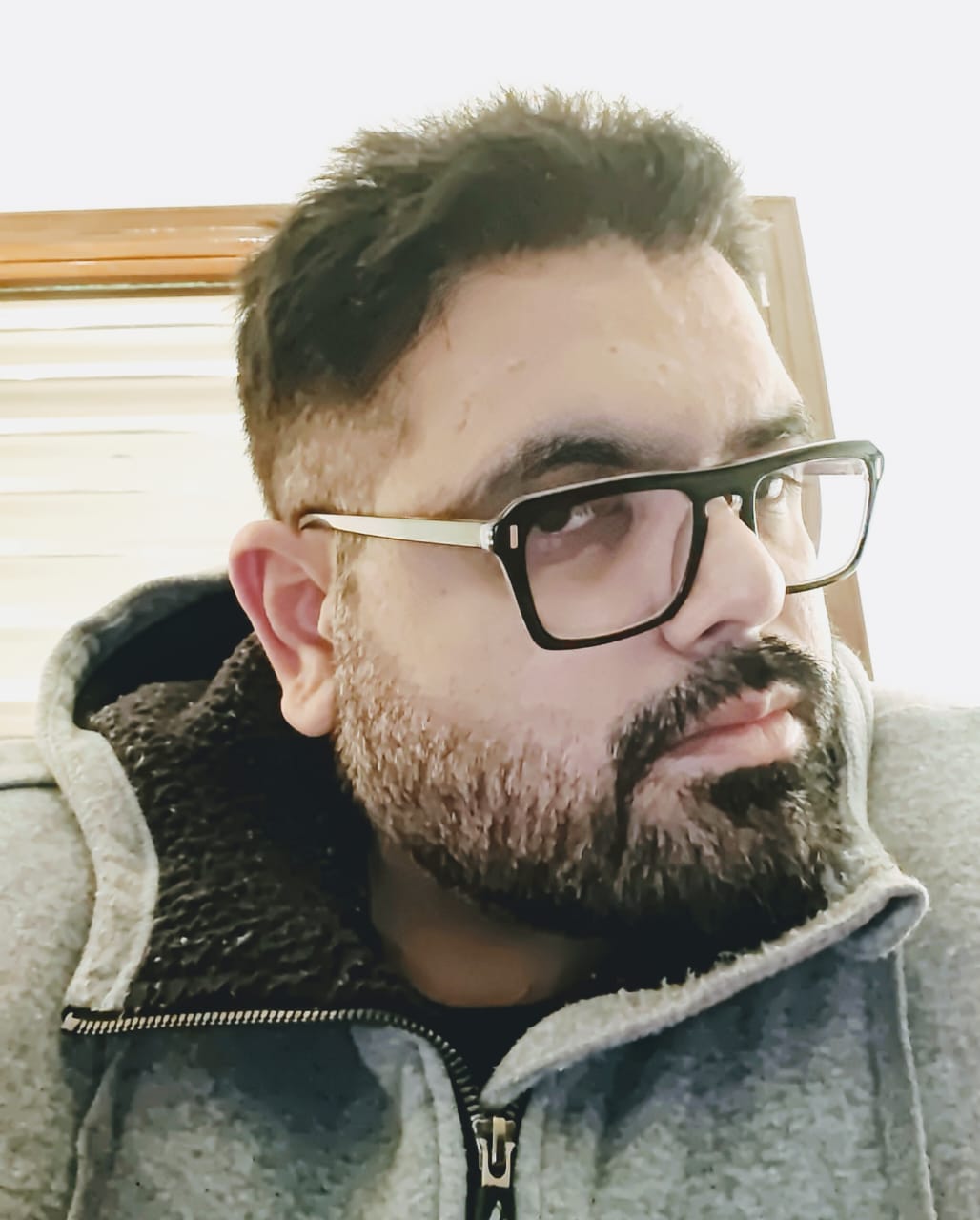
Rai
Rai
Independent Software Systems Engineer