Level Up Your Laravel APIs: Secure Authentication with Sanctum
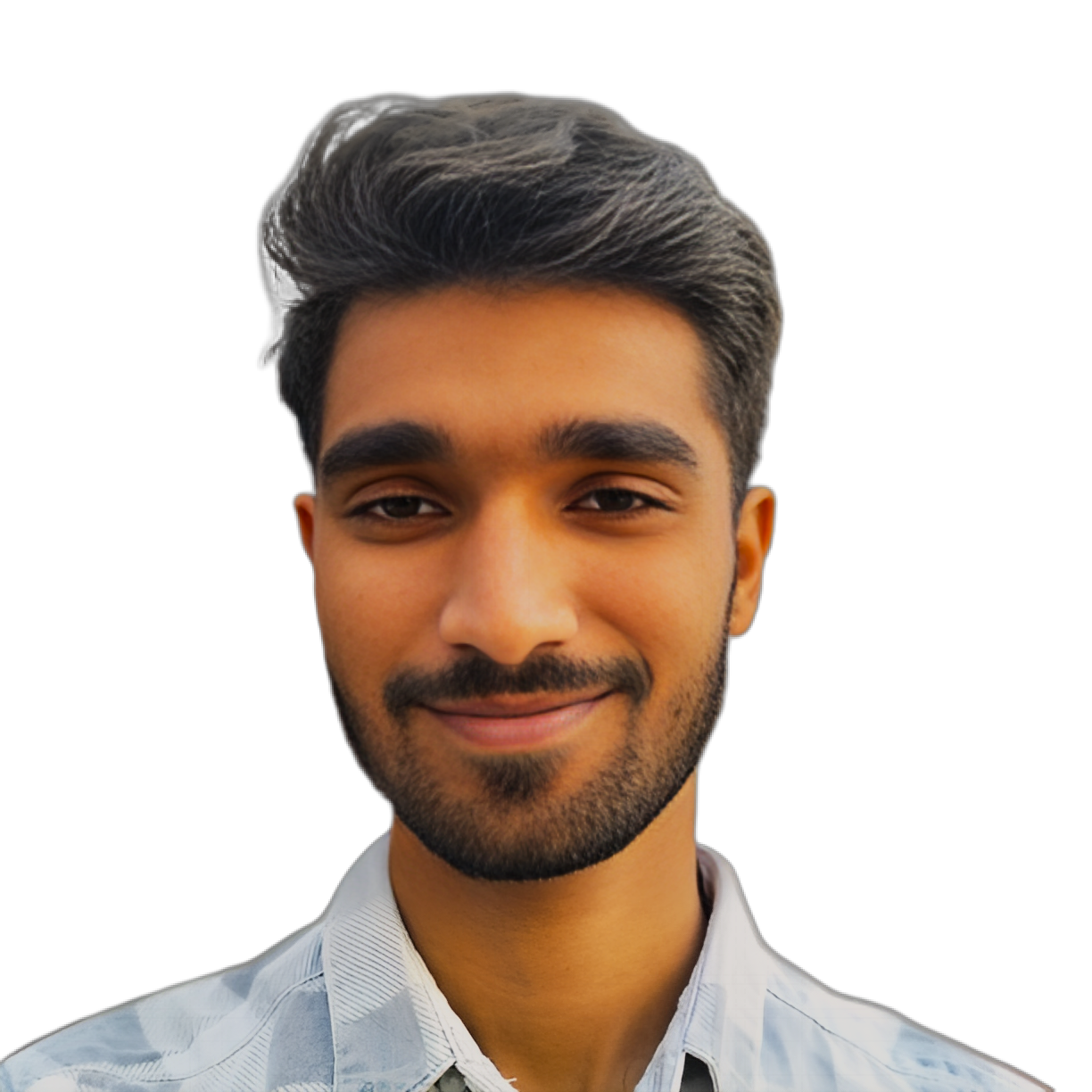
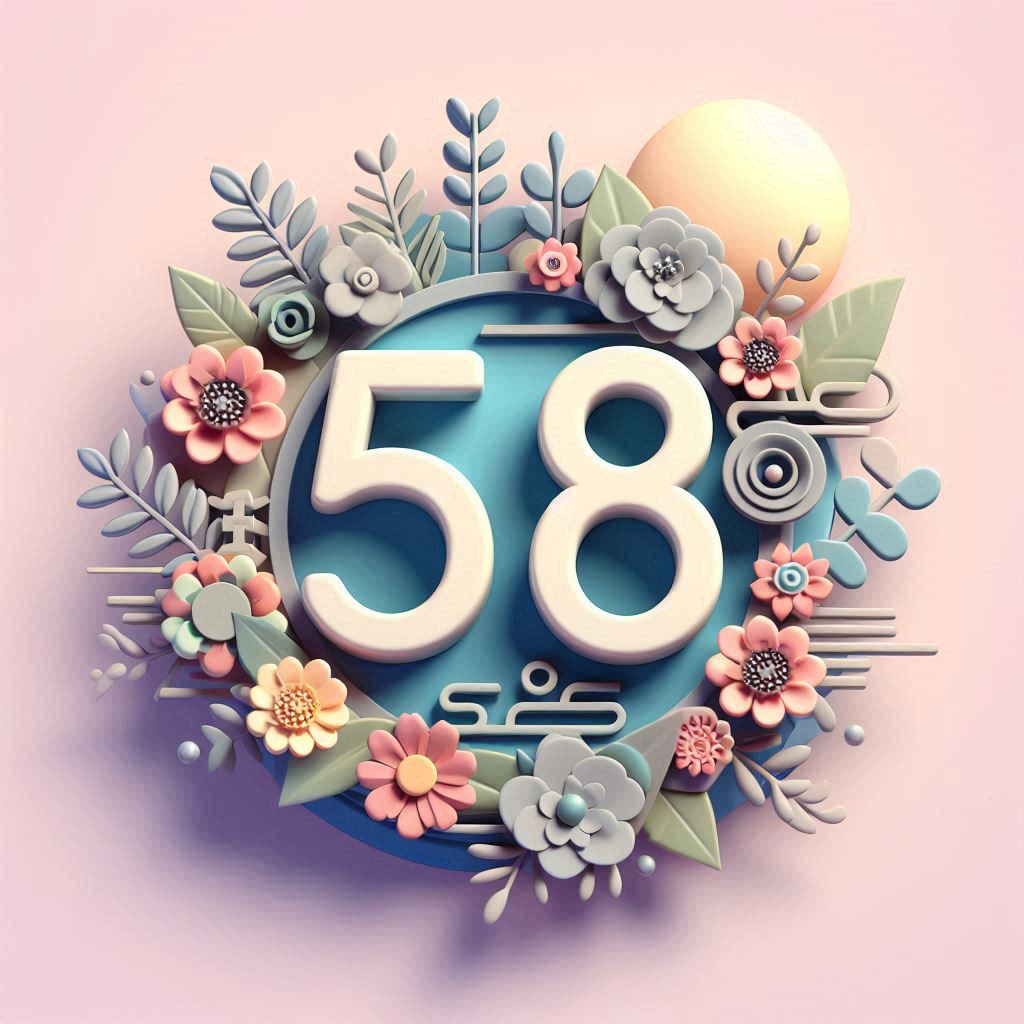
Hey there, web dev enthusiasts! Today, we're diving into the world of Laravel and exploring a fantastic tool called Sanctum. Sanctum is all about supercharging your Laravel application's APIs with robust yet lightweight token-based authentication. It's perfect for modern web applications that leverage Single Page Applications (SPAs) and mobile clients. So, if you're looking to simplify user access control and keep your API endpoints safe and sound, buckle up – Sanctum's about to become your new best friend!
Why Sanctum? You Ask? Well, Let Me Tell You...
Traditional authentication methods like OAuth can feel like untangling a giant ball of yarn. Sanctum throws that yarn ball out the window and offers a refreshingly simple approach with its token-based system. This not only makes user access control a breeze but also keeps security top-notch. Sanctum shines even brighter when it comes to SPA development. It integrates seamlessly, eliminating the need for complex OAuth configurations.
But that's not all! Sanctum empowers you to define token scopes. Think of these scopes like special permissions you can assign to tokens. This gives you granular control over what actions users can perform within your API. Imagine a user who only needs to update their profile information – you can create a token with a limited scope that restricts access to profile editing functionalities. Pretty cool, right? And to top it all off, Sanctum lets you revoke compromised tokens with ease, ensuring the overall security of your application.
Getting Started with Sanctum: A Piece of Cake!
Setting up Sanctum is as easy as, well, making a cake (minus the flour fight, hopefully). Here's a quick walkthrough:
- First things first, let's get Sanctum on board! Open up your terminal and run the following command using Composer:
Bash
composer require laravel/sanctum
- Migrations are essential for creating those database tables Sanctum needs. Let's run the migrations to get things ready:
Bash
php artisan migrate
- For SPAs, we need to configure cookies. Head over to your
app/Http/Kernel.php
file and add theLaravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful
middleware to theweb
middleware group. Here's a code snippet to help you out:
PHP
// app/Http/Kernel.php
protected $middleware = [
// ... other middleware
\Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
\App\Http\Middleware\TrimStrings::class,
\App\Http\Middleware\ConvertEmptyStringsToNull::class,
\App\Http\Middleware\TrustProxies::class,
\Fruitcake\LaravelCors\HandleCors::class,
\App\Http\Middleware\CheckForMaintenanceMode::class,
\Illuminate\Foundation\Http\Middleware\ValidatePostRequest::class,
\App\Http\Middleware\Authenticate::class,
\App\Http\Middleware\Session::class,
\App\Http\Middleware\HandleInertiaRequests::class,
];
Alright, Sanctum is all set up! Now, Let's See It in Action...
(Disclaimer: This is a simplified example to illustrate the concept. In a real application, you'd likely have controllers and proper API routes)
Generating a Secure API Token
Imagine you have a user model with an api_tokens
relationship, as shown previously. To generate a secure API token for a user, you can use something like this in your controller:
PHP
// app/Http/Controllers/UserController.php
public function generateToken(Request $request)
{
$user = $request->user(); // Assuming you have user authentication
$tokenName = $request->input('name', 'my-api-token'); // Allow customization (optional)
$token = $user->createToken($tokenName);
return response()->json([
'access_token' => $token->plainTextToken, // Don't expose this in production!
'token_type' => 'Bearer',
]);
}
This code retrieves the authenticated user from the request and allows the user to optionally provide a name for the token. It then creates a new token record with the specified name and returns a JSON response containing the access_token
(the plain text token – remember, this should never be exposed in production code) and the token_type
("Bearer").
Protecting API Endpoints
Now, let's create a protected API endpoint that requires a valid token for access. Here's an example using Laravel's route middleware:
PHP
// routes/api.php
Route::middleware('auth:sanctum')->get('/protected-resource', function () {
// Access user information from the authenticated user via request()->user()
return response()->json(['message' => 'Success! You accessed the protected resource.']);
});
The auth:sanctum
middleware checks for the presence of a valid Sanctum token in the Authorization header. If the token is valid, the request proceeds, and you can access the authenticated user's information using request()->user()
. If the token is invalid or missing, an unauthorized error response will be sent.
Consuming the API with a Token
With the token securely stored on your SPA or mobile client, you can make authenticated API requests by including the token in the Authorization header. Here's an example using JavaScript's Fetch API:
JavaScript
fetch('/api/protected-resource', {
headers: {
'Authorization': `Bearer YOUR_TOKEN_HERE`,
},
})
.then(response => response.json())
.then(data => {
console.log(data); // Output: { message: 'Success! You accessed the protected resource.' }
})
.catch(error => console.error(error));
This code snippet fetches the protected API endpoint, including the Authorization
header with the Bearer token type and your actual token value. Upon successful authentication, the response data is logged to the console.
Remember:
Never expose the plain text token in your application code! Use secure methods to transmit it to your client.
Implement proper error handling for unauthorized access attempts.
Consider using token scopes for granular control over user permissions within your API.
In Conclusion
Laravel Sanctum simplifies API authentication in your Laravel applications, providing a secure and lightweight solution for user access control and token management. With its ease of use and integration with SPAs and mobile clients, Sanctum empowers you to build robust and scalable web applications.
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
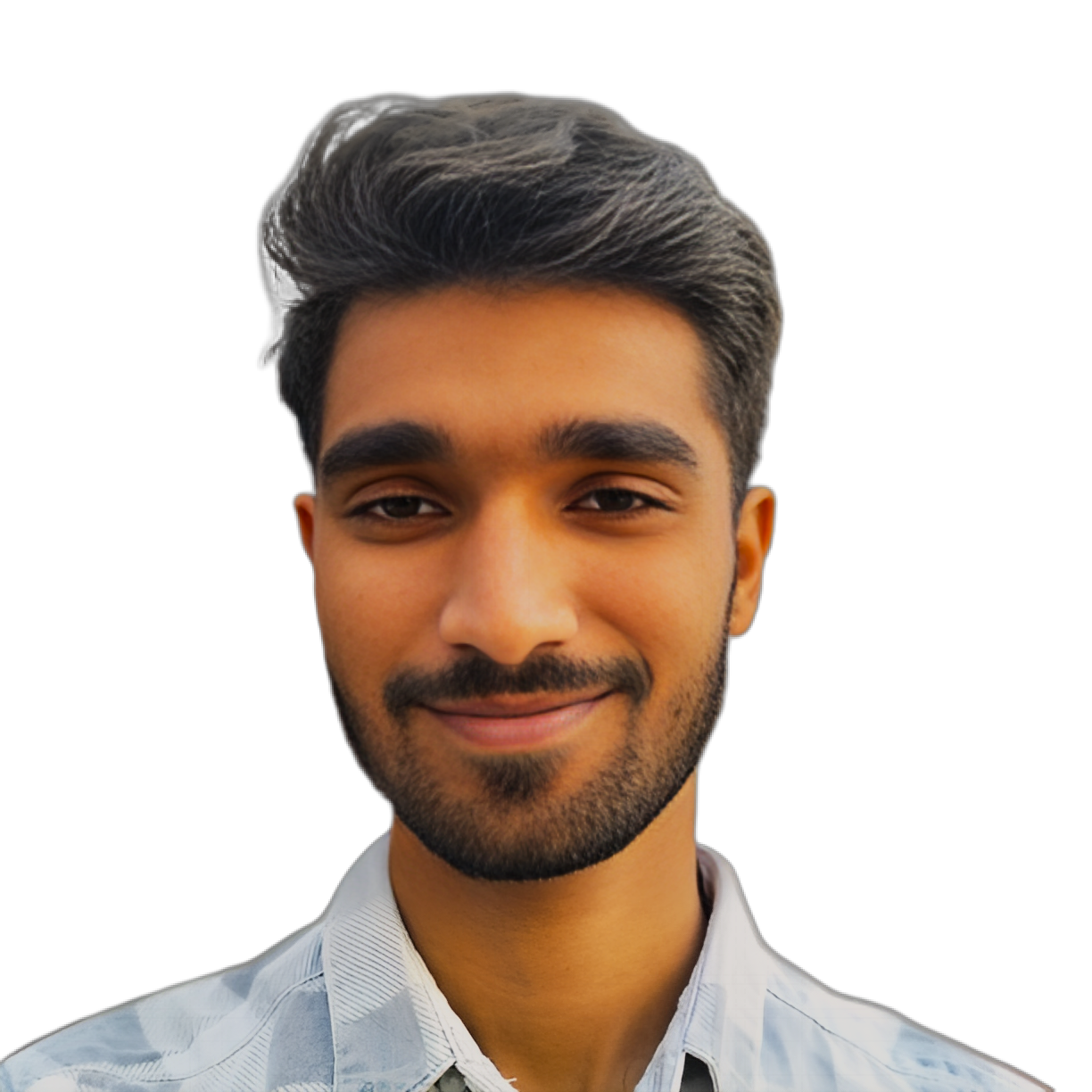
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.