Best Practices for Safe JavaScript "onclick" Events
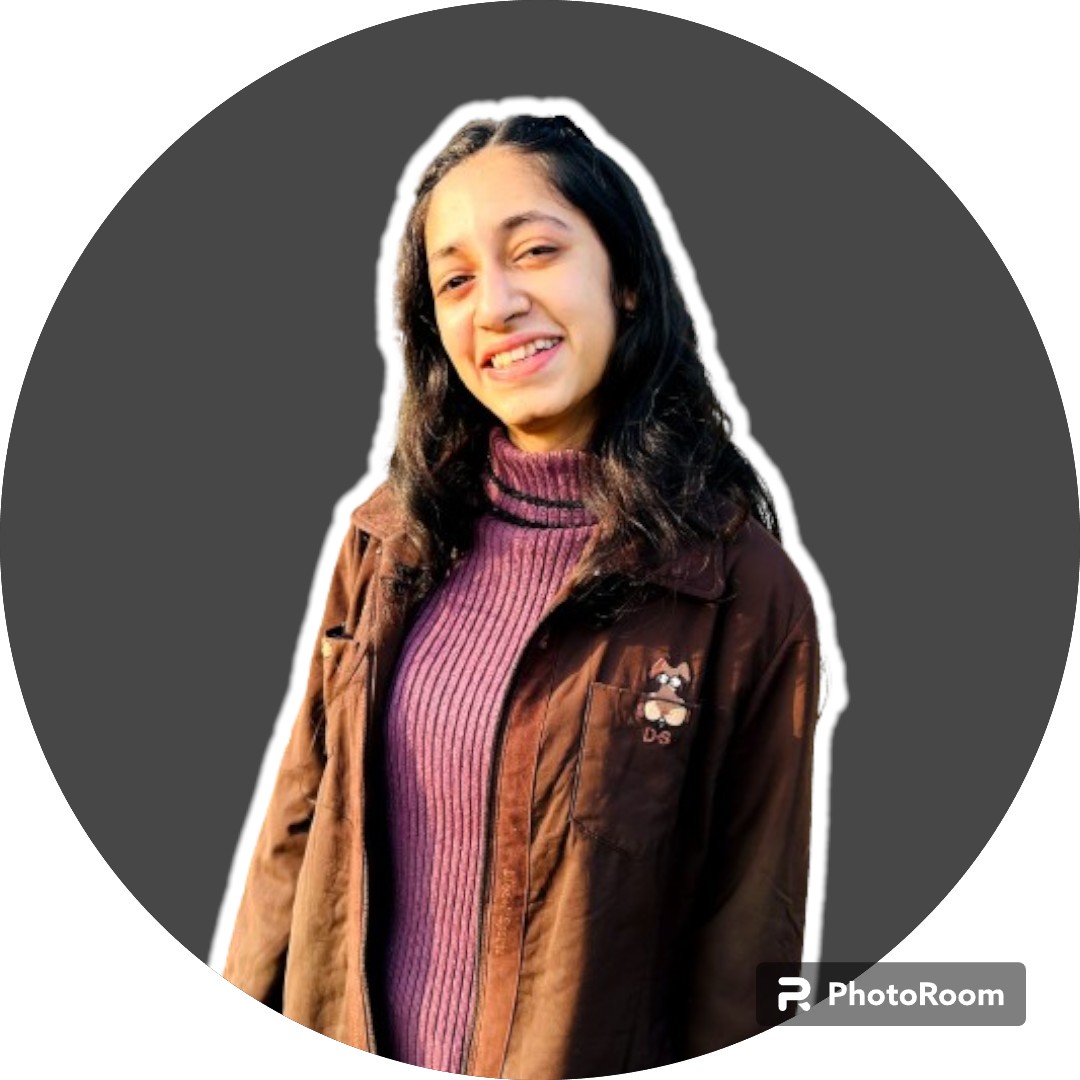
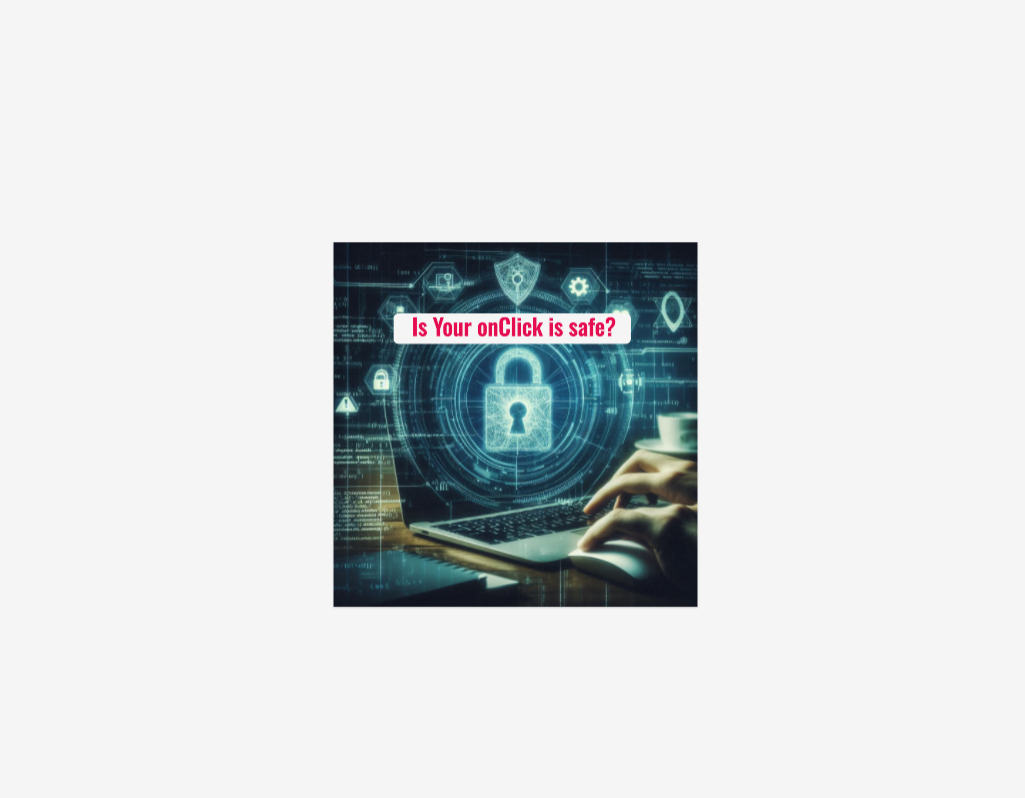
In the world of web development, ensuring the security of user interactions is paramount. One common area where security vulnerabilities can arise is in handling onclick
events in JavaScript. These events are crucial for creating interactive web applications but, if not managed properly, they can open the door to severe security risks such as Cross-Site Scripting (XSS) attacks. In this blog post, we’ll discuss the potential security risks associated with onclick
events and offer best practices for mitigating these risks. We'll also explore how using frameworks and libraries can help enhance security.
Understanding the Risks: Cross-Site Scripting (XSS)
What is XSS?
Cross-Site Scripting (XSS) is a type of security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. These scripts can steal cookies, session tokens, or other sensitive information, and even perform actions on behalf of the user.
How onclick
Events Contribute to XSS
onclick
events can be particularly vulnerable to XSS attacks if they involve user input that is not properly sanitized or validated. For example, if a malicious user is able to insert a script into a webpage that gets executed when another user clicks on an element, it can compromise the security of the application.
Best Practices for Securing onclick
Events
1. Avoid Inline JavaScript
Inline JavaScript is more susceptible to XSS attacks because it is easier for attackers to inject malicious code. Instead of using inline onclick
attributes, use event listeners.
Inline JavaScript:
<button onclick="alert('Hello')">Click Me</button>
Event Listener:
<button id="myButton">Click Me</button>
<script>
document.getElementById('myButton').addEventListener('click', () => {
alert('Hello');
});
</script>
2. Sanitize User Input
Always sanitize any user input that might be used within onclick
event handlers. This ensures that any malicious code is neutralized before it can cause harm.
function sanitizeInput(input) {
const div = document.createElement('div');
div.appendChild(document.createTextNode(input));
return div.innerHTML;
}
// Example usage
const userInput = sanitizeInput('<script>alert("XSS")</script>');
3. Use Safe DOM Manipulation
When adding user input to the DOM, use textContent or other methods that do not parse HTML.
Unsafe:
element.innerHTML = userInput;
Safe:
element.textContent = userInput;
4. Content Security Policy (CSP)
Implementing a Content Security Policy (CSP) can prevent XSS by specifying which sources are allowed to execute scripts. CSP helps to mitigate the risk by blocking the execution of unauthorized scripts.
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self';">
5. Use Trusted Libraries and Frameworks
Using well-maintained libraries and frameworks can significantly enhance security. For example, React and Angular include built-in mechanisms to handle and mitigate XSS risks.
React Example: React automatically escapes any values embedded in JSX to prevent XSS attacks.
const userInput = '<script>alert("XSS")</script>';
const element = <div>{userInput}</div>; // This is safe
Angular Example: Angular uses a built-in sanitizer to clean any potentially dangerous content.
<div [innerHtml]="userInput"></div> <!-- Angular automatically sanitizes userInput -->
6. Validate and Encode Data
Ensure that any data passed to the onclick
event is properly validated and encoded. Never trust user input directly.
Validation Example:
function isValidInput(input) {
const regex = /^[a-zA-Z0-9]*$/; // Example validation for alphanumeric input
return regex.test(input);
}
if (isValidInput(userInput)) {
// Safe to use input
}
7. Regular Security Audits
Conduct regular security audits of your codebase to identify and fix vulnerabilities. Use automated tools like ESLint with security plugins to catch potential issues early.
Conclusion
Securing onclick
events in JavaScript is crucial to protecting your web applications from XSS attacks and other security threats. By following best practices such as avoiding inline JavaScript, sanitizing user input, using safe DOM manipulation methods, implementing Content Security Policies, leveraging trusted frameworks, validating and encoding data, and conducting regular security audits, you can significantly reduce the risk of security vulnerabilities.
Ensuring the security of your onclick
events not only protects your users but also maintains the integrity and trustworthiness of your web application. Stay vigilant and proactive in applying these security measures to build robust and secure applications.
Happy coding!
Subscribe to my newsletter
Read articles from Mahak Pandey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
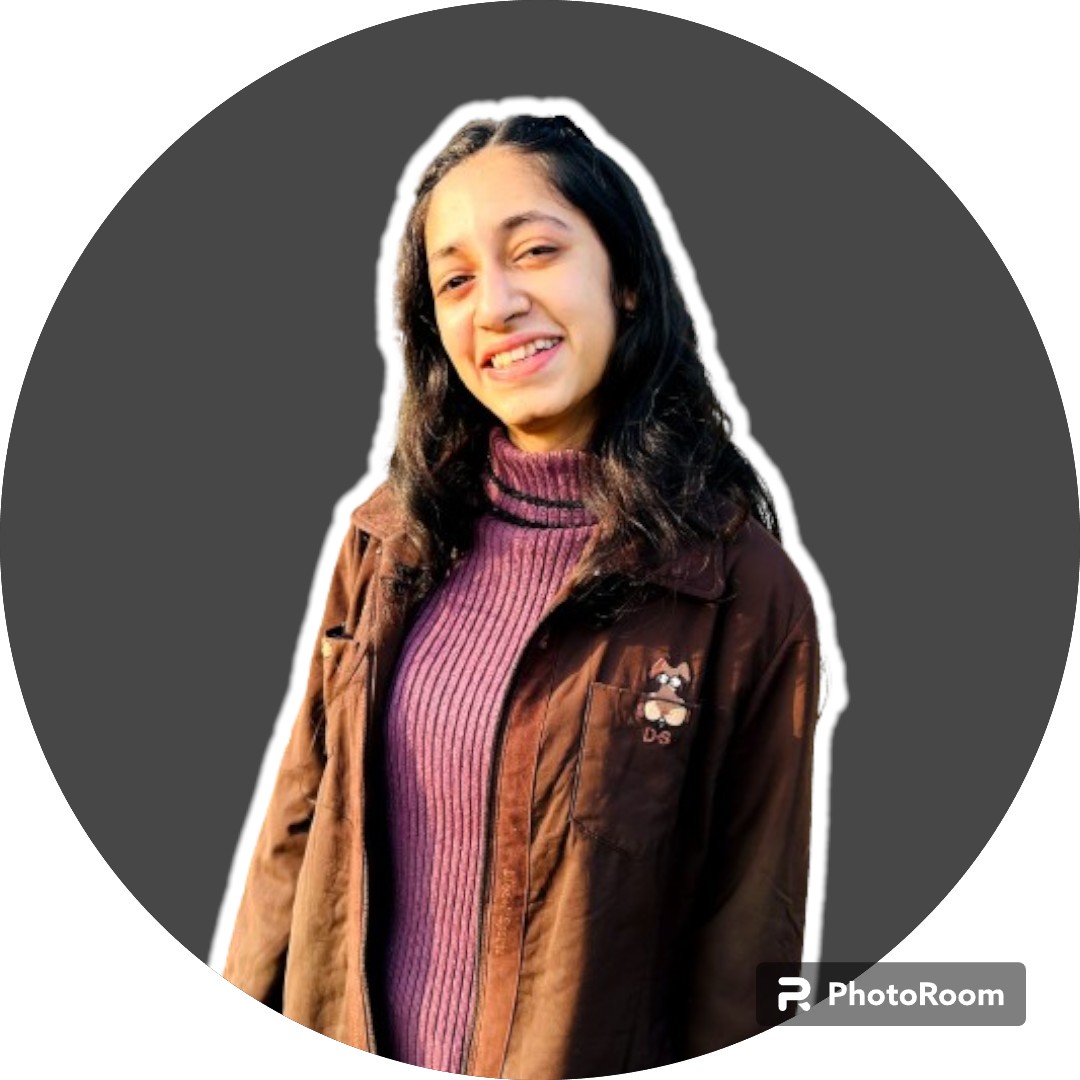
Mahak Pandey
Mahak Pandey
Hey, I am currently a 4th year student majoring in Computer science & Information Technology. I have a strong academic background with coursework in software development, database management, Operating System, OOPS and cybersecurity, and I’ve maintained a CGPA of 9.28. I am proficient in Java, HTML, CSS, Figma, JavaScript, Learning React and core Knowledge Of MERN STack. Additionally, I have hands-on experience with version control systems like Git, and I am familiar with Visual studio code IDE, working experience with Blender for 3D modeling and rendering, Figma for UI design and Prototyping, Canva for Designing assets. Here to share knowledge i've learned and learning. I recently publish the "Cyber Security" Book as a Co-Author. I like to learn and build in public. If you want to connect with me do follow my socials.