Redis as a Cache in Django
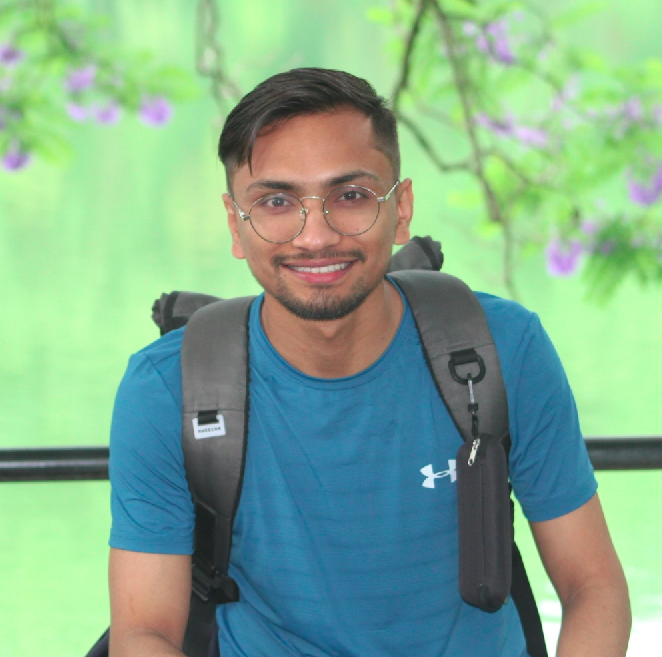
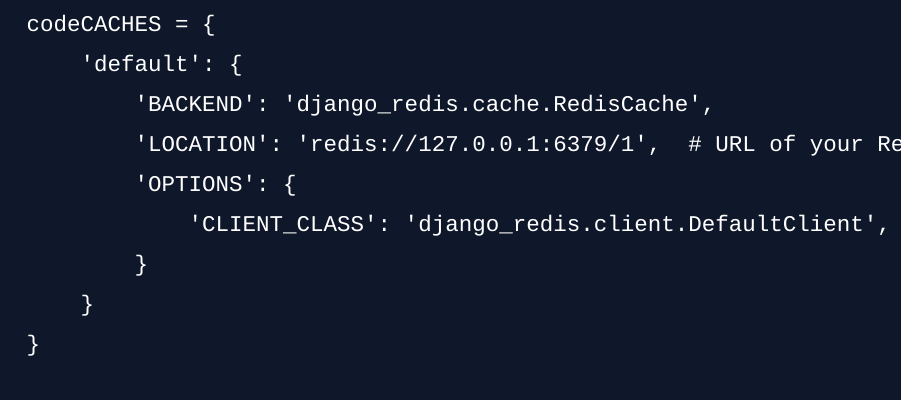
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. It supports various data structures like strings, hashes, lists, sets, and sorted sets, making it versatile for a wide range of use cases.
Key Features of Redis:
In-Memory Data Storage: Redis stores data in memory, making it extremely fast for read and write operations.
Persistence Options: Redis supports different persistence options like snapshotting and append-only files to ensure data durability.
Data Structures: Redis provides a rich set of data structures like strings, hashes, lists, sets, and sorted sets, allowing for flexible data modeling.
Atomic Operations: Redis supports atomic operations on data structures, making it suitable for building high-performance applications.
Pub/Sub Messaging: Redis offers pub/sub messaging for building real-time applications and message queues.
Replication and Sharding: Redis supports replication and sharding for high availability and scalability.
Lua Scripting: Redis allows you to write Lua scripts for complex data manipulation and processing.
Policies in Redis:
Eviction Policies: Redis allows you to configure different eviction policies for handling memory constraints, such as LRU (Least Recently Used), LFU (Least Frequently Used), and Random.
LRU : Suppose a cache has a memory limit of 100 keys and it currently holds 120 keys. When a new key needs to be added to the cache, Redis checks the access timestamps of all keys. It then selects the 20 keys that were accessed the longest time ago and evicts them from the cache to make space for the new key.LFU: Suppose a cache has a memory limit of 100 keys and it currently holds 120 keys. When a new key needs to be added to the cache, Redis checks the access counts of all keys. It then selects the 20 keys with the lowest access counts and evicts them from the cache to make space for the new key.
Expiration Policies: Redis supports setting expiration times for keys, after which they will automatically be removed from the database.
Using Redis in Django with Hands-On Example:
To use Redis in Django for caching, follow these steps:
Install Redis Server and Python Redis Client:
Install Redis server on your system or use a cloud-based service like Redis Labs.
Install the Python Redis client using pip:
pip install django-redis
Configure Redis Cache Backend in Django Settings:
Open your Django project's
settings.py
file and configure Redis as the cache backend:codeCACHES = { 'default': { 'BACKEND': 'django_redis.cache.RedisCache', 'LOCATION': 'redis://127.0.0.1:6379/1', # URL of your Redis instance 'OPTIONS': { 'CLIENT_CLASS': 'django_redis.client.DefaultClient', } } }
Use Redis Cache in Django Views:
You can now use Django's cache framework to set and retrieve data from Redis in your views:
from django.core.cache import cache def my_view(request): # Set cache cache.set('my_key', 'my_value', timeout=3600) # Cache for 1 hour # Get cache value = cache.get('my_key')
```
Cache Decorators:
Django provides cache decorators to cache the output of views for a specified amount of time:
from django.views.decorators.cache import cache_page @cache_page(60 * 15) # Cache for 15 minutes def my_cached_view(request): # View logic
```
Cache Middleware:
You can also use cache middleware to cache entire pages or parts of pages in your Django application:
codeMIDDLEWARE = [ ... 'django.middleware.cache.UpdateCacheMiddleware', 'django.middleware.common.CommonMiddleware', 'django.middleware.cache.FetchFromCacheMiddleware', ... ]
Redis CLI for Monitoring:
You can use the Redis CLI to monitor cache activity and inspect cache keys:
$ redis-cli 127.0.0.1:6379> KEYS *
By following these steps, you can integrate Redis caching into your Django applications to improve performance and scalability. Redis offers various features and policies that you can leverage to optimize your caching strategy based on your application's requirements.
To change the default eviction policy to use LRU (Least Recently Used), you need to set the maxmemory-policy
option in the Redis configuration to volatile-lru
. Here's how you can modify the Django configuration to achieve this:↳
CACHES = {
'default': {
'BACKEND': 'django_redis.cache.RedisCache',
'LOCATION': 'redis://127.0.0.1:6379/1', # URL of your Redis instance
'OPTIONS': {
'CLIENT_CLASS': 'django_redis.client.DefaultClient',
'MAXMEMORY_POLICY': 'volatile-lru', # Set the eviction policy to LRU
}
}
}
By adding the MAXMEMORY_POLICY
option with the value volatile-lru
to the OPTIONS
dictionary, you instruct Redis to use the LRU eviction policy among the keys with an expiration set (volatile-lru
). This means that Redis will evict keys based on their last access time when memory is full, prioritizing keys with an expiration set (e.g., keys set with a TTL). You can also change the policy from redis.conf file.
Subscribe to my newsletter
Read articles from Deven directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
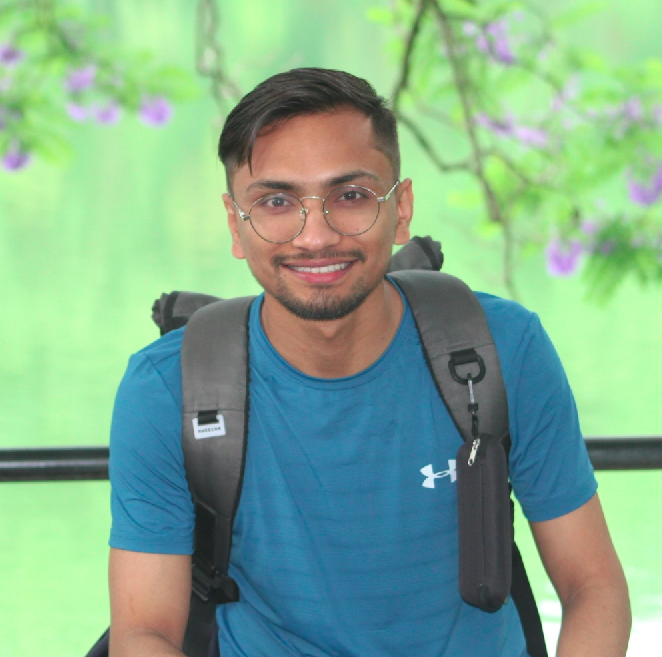
Deven
Deven
"Passionate software developer with a focus on Python. Driven by a love for technology and a constant desire to explore and learn new skills. Constantly striving to push the limits and create innovative solutions.”