ENCAPSULATION - Java OOPs Simplified Part 6

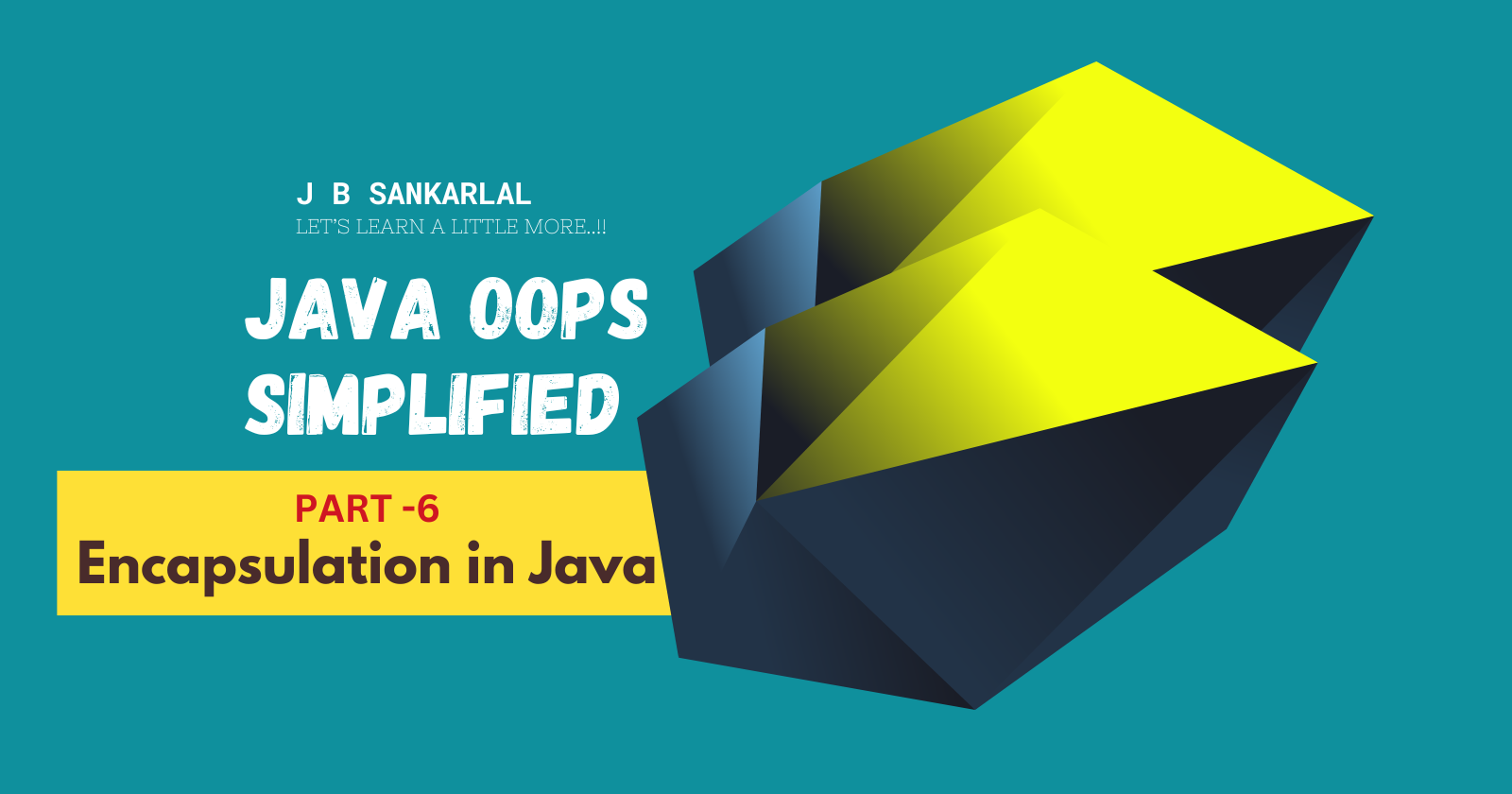
This is one of the four pillars of Object-Oriented Programming. So let's dive in detail to understand what it really is.
What is Encapsulation?
Just imagine a capsule pill. The medicine inside the capsule is not exposed directly. Instead, it's encapsulated within a shell. You can only access the medicine by consuming the capsule. This ensures that the medicine is protected and delivered in the right way.
In the same way, in Java, encapsulation is like putting your data (variables) inside a protective shell (class) and controlling access to it through methods.
Now let's look at an Example.
Let's say we have a Student
class. We want to keep the student's age private and control how it's accessed and modified.
Define the class with private fields:
- We make the
age
field private so it cannot be accessed directly from outside the class.
- We make the
Provide public methods to access and modify the age:
- We create public getter and setter methods to access and update the age.
Let's look at the code illustration for this.
public class Student{
// declare age as private field
private int age;
// a public method to get the age
public int getAge() {
return age;
}
// now, a public method to set the age with some validation
public void setAge(int age) {
if (age >= 0) { // Validating that age cannot be negative
this.age = age;
} else {
System.out.println("Age cannot be negative");
}
}
}
Now, let's look how can we use the "Student" class
public class Main {
public static void main(String[] args) {
Student s1= new Student();
// Set the age using the setter method
s1.setAge(16);
// Get the age using the getter method
System.out.println("Student's age: " + s1.getAge());
// Try to set an invalid age
s1.setAge(-5); // This will print "Age cannot be negative"
}
}
So, here we are implementing two things,
- Setting the Age:
s1.setAge(16);
This line calls the
setAge
method on theStudent
object, passing "16" as an argument. ThesetAge
method updates the privateage
field with this value.
Getting the Name:
System.out.println("Student's age: " + s1.getAge());
This line calls the
getAge
method on thestudent
object. ThegetAge
method returns the value of the privateage
field, which is then printed to the console.
Data Hiding : The
age
field is private, so it cannot be accessed directly from outside theStudent
class. This means the internal state of theStudent
is hidden from the outside world.Controlled Access : We provide public methods
getAge()
andsetAge(int age)
to access and modify theage
field. This allows us to add validation logic inside thesetAge()
method to ensure that the age cannot be set to a negative value.
Why we use Encapsulation?
There are several advantages for using Encapsulation;
Data Protection: By making fields private, you can prevent external code from changing the state of the object in unexpected ways.
Controlled Access: By providing public getter and setter methods, you can control how the internal state of an object is accessed and modified. This allows you to add validation, logging, or other logic when the state is changed.
Easy to maintain: Encapsulation helps to keep the code modular. So that every class can be developed, tested and maintained independently.
Resuability: It encourages the creation of reusable components. By exposing only the necessary details through public methods, you can create classes that can be reused across different parts of an application.
Getter and Setter Methods
We have already mentioned about the getter and setter methods above. So now lets understand what are these in detail.
In Java, getter and setter methods are used to access and modify the private fields of a class from outside the class. They are part of the encapsulation concept.
Getter Method: This method is used to read or "get" the value of a private field.
Setter Method: This method is used to update or "set" the value of a private field.
Why we use these Getter and Setter methods?
Since fields in a class are often made private to protect them from direct access, getter and setter methods provide a way to access and update these private fields indirectly. This allows for controlled access and modification.
So, let's take the same example from above and explain it again. This will help beginners understand it with more clarity.
Imagine a simple class called Student
with a private field name
.
Step - 1
Define the class with a private field.
public class Student {
private String name; // private field
}
Here, name
is a private field, meaning it cannot be accessed directly from outside the Student
class.
Step - 2
Create setter and getter methods - to access and modify the name field.
public class Student{
private String name;
//getter method
public String getName() // getter and setter are not predefined method names.
{
return name;
}
//setter method
public String setName(){
this.name= name
}
}
Step -3
Now let's see how can we access and update the name field value in the above code.
public class Main {
public static void main(String[] args) {
Student s1= new Student(); // Create a new Student object
// Set the name using the setter method
s1.setName("Sankarlal");
// Get the name using the getter method
System.out.println("Student's name: " + s1.getName()); // Output: Student's name: Sankarlal
}
}
Setting the Name:
s1.setName("Sankarlal");
This line calls the
setName
method on thestudent
object, passing "Sankarlal" as an argument. ThesetName
method updates the privatename
field with this value.
Getting the Name:
System.out.println("Student's name: " + s1.getName());
This line calls the
getName
method on thestudent
object. ThegetName
method returns the value of the privatename
field, which is then printed to the console.
I think now you have a good understanding of Encapsulation and how getter and setter methods work.
Subscribe to my newsletter
Read articles from J B Sankarlal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
