Mastering List Copying Techniques in Python
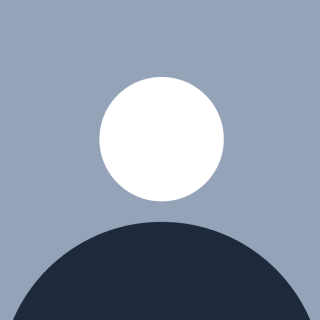
Table of contents
Lists are a fundamental data structure in Python, and understanding how to copy them effectively is crucial for any programmer. In this blog post, we'll explore various methods to copy lists in Python, highlighting their use cases and potential pitfalls.
Using the
copy
Method Python lists come with a built-incopy
method that creates a shallow copy of the list.original_list = [1, 2, 3, 4] copied_list = original_list.copy()
Using Slicing Slicing is a common technique to create a shallow copy of a list.
original_list = [1, 2, 3, 4] copied_list = original_list[:]
Using the
list
Constructor Thelist
constructor can also be used to create a shallow copy of a list.original_list = [1, 2, 3, 4] copied_list = list(original_list)
Using
copy
Module For deep copies, you can use thecopy
module'sdeepcopy
function.import copy original_list = [[1, 2], [3, 4]] copied_list = copy.deepcopy(original_list)
Using a List Comprehension A list comprehension can be used to create a shallow copy of a list.
original_list = [1, 2, 3, 4] copied_list = [item for item in original_list]
Using
*
Operator The*
operator can be used to create a shallow copy of a list.original_list = [1, 2, 3, 4] copied_list = [*original_list]
Shallow vs. Deep Copy
Shallow Copy: Copies the list structure but not the elements if they are mutable objects.
Deep Copy: Copies both the list structure and the elements, creating independent copies.
Conclusion
Choosing the right method to copy a list depends on your specific needs. For most cases, a shallow copy will suffice, but for nested lists or more complex data structures, a deep copy might be necessary. Understanding these methods will help you write more efficient and bug-free Python code.
Subscribe to my newsletter
Read articles from Chandra Prakash Tekwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by