Day 10 of #100DaysOfCode - Simple Calculator
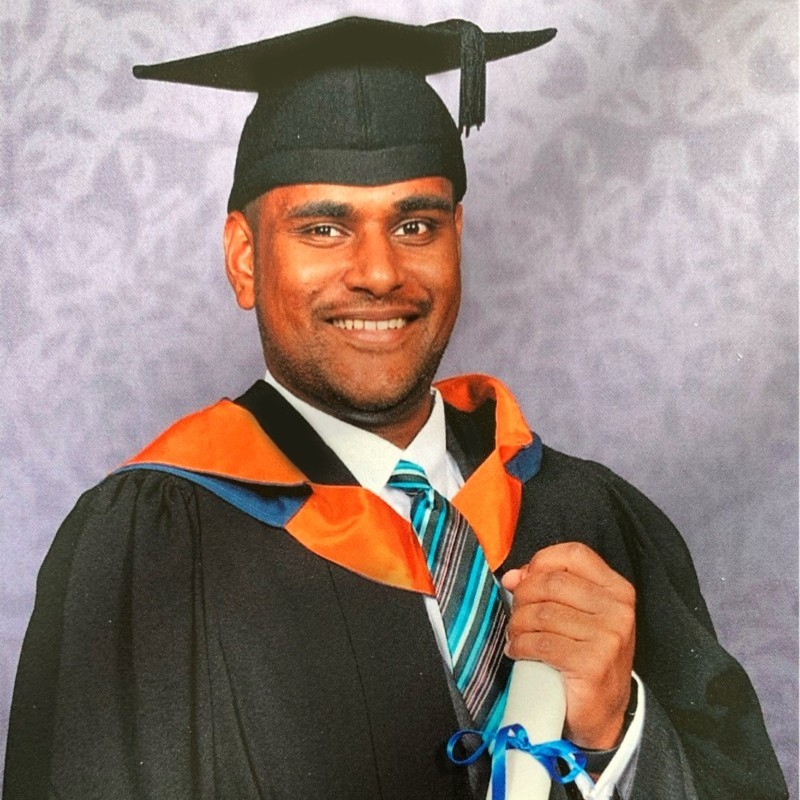
On Day 10 of #100DaysofCode, I have created a Simple Calculator using the following python concepts I have learned from Dr Angela Yu's 100DaysOfCode: The Complete Python Bootcamp Udemy course:
Functions with Outputs
Recursion
Python Dictionary
While loops
For loops
Data Types
The Simple Calculator program prompts the user to enter the first number and choose an operation (addition, subtraction, multiplication, or division), followed by entering the second number. It then calculates and displays the result. The user can choose to continue using the current result with another operation and number. If they choose to continue, they select another operation and number, and the program calculates and displays the new result. If the user does not wish to continue, the program asks if they want to start a new calculation. If they do, they input a new first number. If not, the program ends. This design allows users to perform continuous or new calculations as desired.
Python Concepts Used:
Functions with Outputs
The purpose of using functions with outputs in the simple calculator program is to pass the two numbers that the user inputs and performs the operation to returning the result as the output. The output then gets stored into a variable called answer
.
# Define the addition operation
def addition(n1, n2):
return n1 + n2
Recursion
Recursion is used in the simple calculator program when the user inputs 'y'
to start a new calculation, it changes the current flag from True to False which exits the loop and clears the console. It then calls the calculator()
function recursively, which restarts the calculator from the beginning. This allows them to enter new numbers and operations. The recursive call, resets the state of the calculator, allowing a sequence of calculations that are independent from the previous calculations.
if new_calculation == "y":
continue_calculation = False
os.system("clear") # Clears the current calculation
calculator() # Recursive call takes place to restart the calculator.
Python Dictionary
The purpose of having a python dictionary is to map the current mathematical operations to the corresponding operations that the user has chosen. Having a python dictionary allows the program to directly access the function of an mathematical operation that the user has chosen instead of using if and elif statements to determine the function it should call based on the user selection.
calculator_operations["+"] = addition
calculator_operations["-"] = subtraction
calculator_operations["*"] = multiplication
calculator_operations["/"] = division
While loop
The while loop extends the capability for users to perform continuous calculations within a single session, eliminating the need to create independent calculations for each operation. It continues running until the user opts to start a new calculation or exits the Simple Calculator program.
For loop
The purpose of the for loop is to display the operation symbols, providing users with clear options to select their desired mathematical operation for calculating the two numbers. This approach eliminates the necessity of individually displaying each operation symbol, as each symbol can be presented in each iteration of the loop.
Data Types
In this program, we opt for the float data type. This choice enables the simple calculator to handle decimal numbers accurately, ensuring precise results for both integer and non-integer values.
Reflections on the Simple Calculator
While working on the mini project, I encountered a notable issue. The challenge revolved around the inability to convert the data type from string to float. The error message, "TypeError: unsupported operand type(s) for -: 'str' and 'str'", underscored this difficulty, indicating that the input function treated the first and second numbers as strings rather than floats. Resolving this issue necessitated modifying the data type to facilitate calculations within the appropriate mathematical operation functions. Despite this obstacle, I found pleasure in developing this program, drawing upon Python concepts I've learned both recently and in the past. For access to the full code, https://github.com/keiransystem14/Python100daysofcode/tree/main/Day%2010%20Project%20-%20Simple%20Calculator
Subscribe to my newsletter
Read articles from Keiran Krishnavenan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
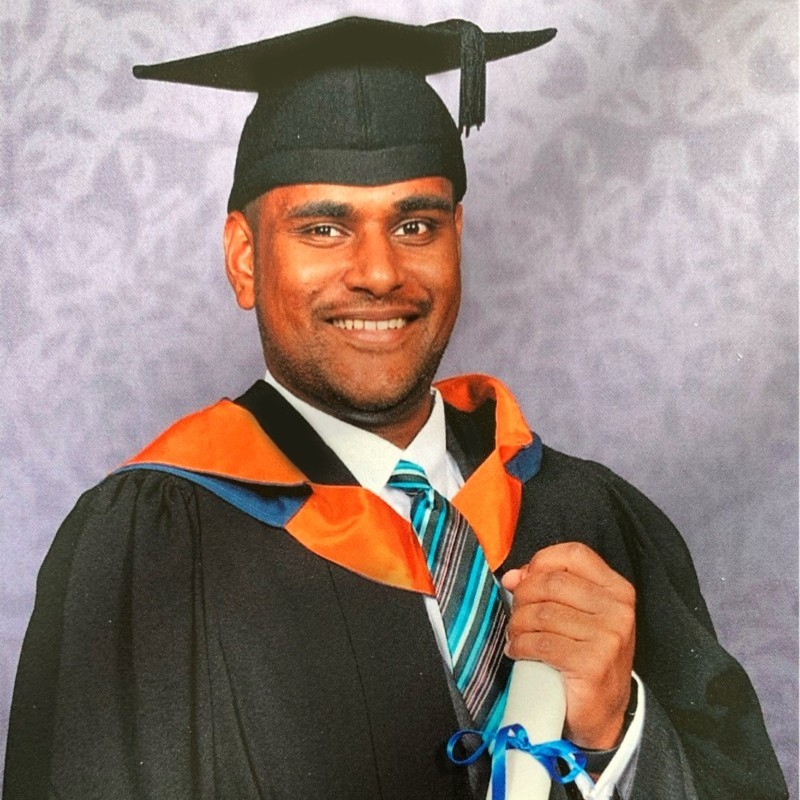