17 Java - Control Flow Statements
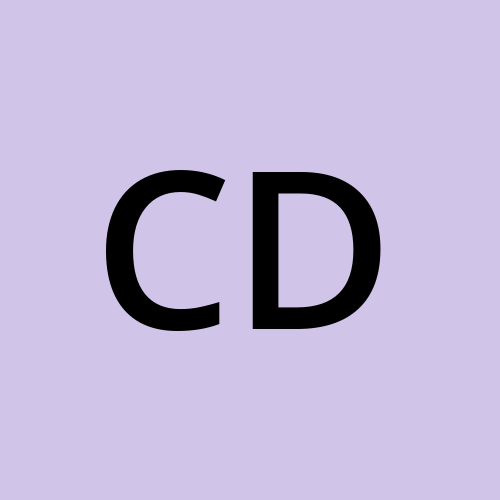
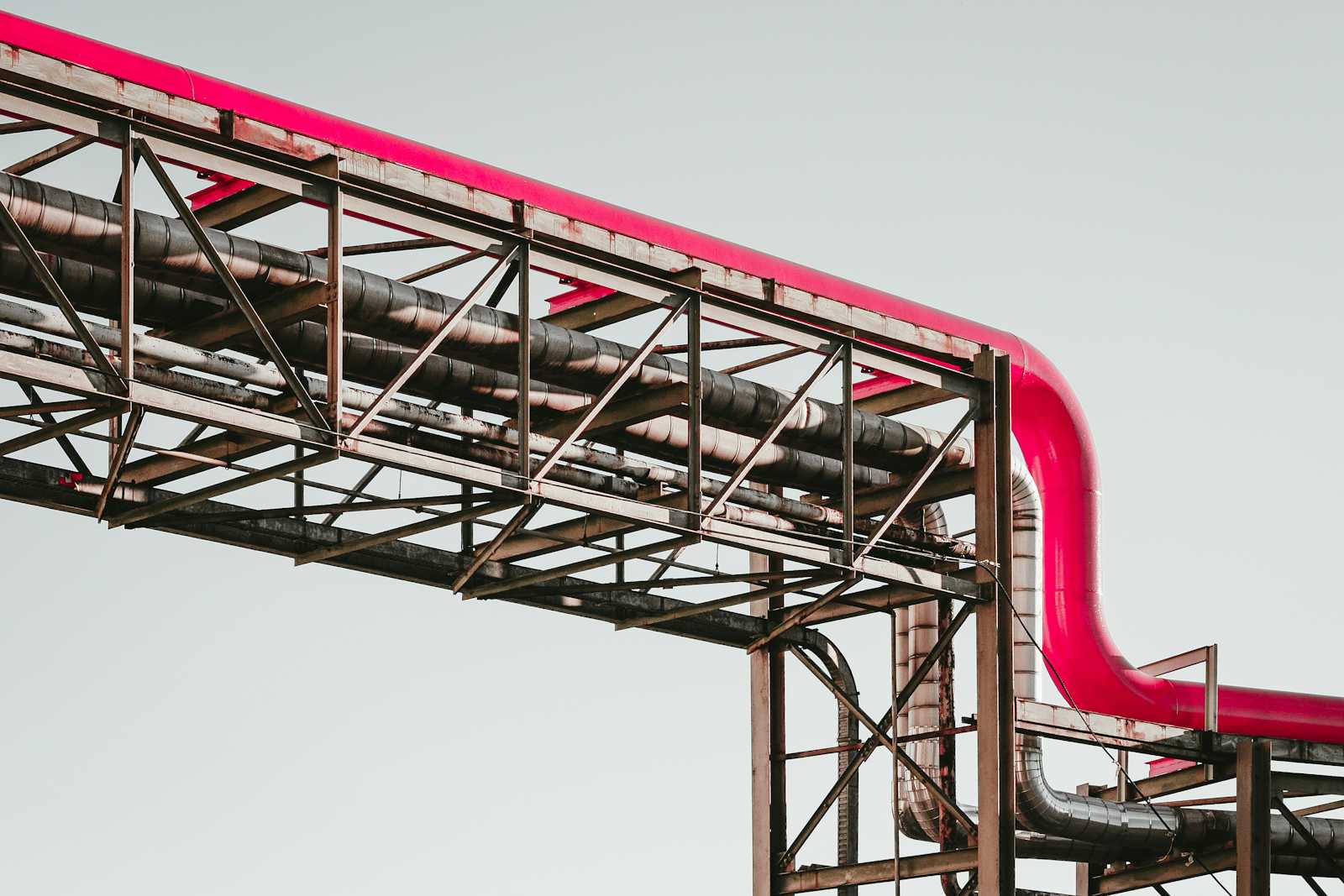
Decision Making Statements
If then (Simple if)
- If Condition is true, then if-block will get executed.
if(boolean condition){
//Code here get executed when condition is true
}
if-else
- If condition is true, if-block will get executed else if condition is false, then else-block will get executed.
if(boolean condition){
//Code here get executed when condition is true
}
else{
// Code here get executed when condition is false
}
if-else-if Ladder
It contains if-statements with multiple (chain of) else-if statements. It evaluate the condition from top and goes down and any condition gets true, its block will get executed.
public class Main {
public static void main(String[] args) {
int val = 13;
if(val==1){
System.out.println("val is 1");
}
else if (val == 2) {
System.out.println("val is 2");
}
else if (val == 3) {
System.out.println("val is 3");
}
else{
System.out.println("val is: "+ val);
}
}
}
Nested-if statement
if-else statement within if-block or else-block
public class Main {
public static void main(String[] args) {
int val = 13;
if(val>8){
System.out.println("val is greater than 8");
if (val<15){
System.out.println("value is greater than 8 but less than 15");
}
else {
System.out.println("value is greater than 15");
}
}
else{
System.out.println("val is less than 8 ");
}
}
}
Switch Statement
It is similar to if-else-if ladder, based on condition particular block will get executed based out of many alternatives.
Syntax
switch(expression){
case value1:
//code statments
break;
case value2:
//code statments
break;
...
...
case valueN:
//code statments
break;
default:
//default code statements
break;
}
Examples
Example 1:
public class Main {
public static void main(String[] args) {
int a = 1;
int b =2;
switch (a+b){
case 1:
System.out.println("a+b is 1");
break;
case 2:
System.out.println("a+b is 2");
break;
case 3:
System.out.println("a+b is 3");
break;
default:
System.out.println(a+b);
break;
}
}
}
/*
a+b is 3
*/
Example 2:
public class Main {
public static void main(String[] args) {
int a = 1;
int b =2;
switch (a+b){
case 1:
System.out.println("a+b is 1");
break;
case 2:
System.out.println("a+b is 2");
break;
case 3:
System.out.println("a+b is 3");
case 4:
System.out.println("a+b is 4");
default:
System.out.println(a+b);
}
}
}
/*
a+b is 3
a+b is 4
3
*/
Example 3:
public class Main {
public static void main(String[] args) {
int a = 1;
int b = 2;
switch (a+b){
case 1:
System.out.println("a+b is 1");
break;
default:
System.out.println(a+b);
case 2:
System.out.println("a+b is 2");
break;
}
}
}
/*
3
a+b is 2
*/
Example 4:
public class Main {
public static void main(String[] args) {
String month = "March";
switch (month){
case "January":
case "February":
case "March":
System.out.println("month value is in Q1");
break;
case "April":
case "May":
case "June":
System.out.println("month value is in Q2");
break;
default:
System.out.println("month value is in Q3 or Q4");
}
}
}
/*
month value is in Q1
*/
Example 5: Same
public class Main {
public static void main(String[] args) {
String month = "March";
switch (month){
case "January","February","March":
System.out.println("month value is in Q1");
break;
case "April","May","June":
System.out.println("month value is in Q2");
break;
default:
System.out.println("month value is in Q3 or Q4");
}
}
}
/*
month value is in Q1
*/
Few things we need to take care
Two cases cannot have the same value.
Switch expression data type and case values/constant data type should be same.
Case value should be either LITERAL or CONSTANT
Incorrect Usage
Correct Usage
public class Main {
public static void main(String[] args) {
final int value = 1;
switch (2+1-2){
case value:
System.out.println("some code here");
default:
System.out.println("default code here");
}
}
}
/*
some code here
*/
All use cases need not to be handled
public class Main {
public static void main(String[] args) {
Day dayEnumVal = Day.FRIDAY;
int outputValue = 0;
switch (dayEnumVal){
case MONDAY:
outputValue = 1;
break;
case TUESDAY:
outputValue = 2;
break;
case WEDNESDAY:
outputValue = 3;
break;
case THURSDAY:
outputValue = 4;
break;
}
System.out.println(outputValue);
}
}
/*
0
*/
Nested Switch statement is possible
public class Main {
public static void main(String[] args) {
Day dayEnumVal = Day.MONDAY;
int outpuValue = 0;
switch (dayEnumVal){
case MONDAY:
outputValue = 1;
switch (outpuValue) {
case 1:
System.out.println("output val is 1");
break;
case 2:
System.out.println("output val is 2");
break;
default:
System.out.println("output val is "+ outpuValue);
}
break;
case TUESDAY:
outputValue = 2;
break;
case WEDNESDAY:
outputValue = 3;
break;
case THURSDAY:
outputValue = 4;
break;
}
System.out.println(outputValue);
Supported Data Types
4 Primitive types: int, short, byte, char
Wrapper Types of above primitive data types i.e. Integer, Short, Byte, Character.
Enum
String
Switch Expression
Return is not possible within Switch case.
There are 2 ways to do it:
Using "case N ->" Label
Using "yield" statement
Use of "case N ->"
public class Main {
public static void main(String[] args) {
String day = "";
int val = 1;
String outputVal = switch (val){
case 1 -> "One";
case 2 -> "Two";
default -> "None";
};
System.out.println(day);
}
}
All possible use cases need to be handled for the expression.
Using this "->" we can not have block of statements. If we want block statements and return the value, we need to use "yield"
Use of "yield"
public class Main {
public static void main(String[] args) {
String day = "";
int val = 1;
String outputVal = switch (val){
case 1 -> {
//some code logic here
yield "One";
}
case 2 -> {
//some code logic here
yield "Two";
}
default -> "None";
};
System.out.println(day);
}
}
Iterative Statements
for loop
Syntax
for(initialization of variable; condition check; increment/decrement of varaible){
//statement block
}
public class Main {
public static void main(String[] args) {
for (int val=1; val<=5; val++){
System.out.println(val);
}
}
}
/*
1
2
3
4
5
*/
Can also have a for loop inside a for loop
public class Main {
public static void main(String[] args) {
for (int x=1; x<=3; x++){
for (int y=1; y<=3;y++){
System.out.println("x="+x+" y="+y);
}
}
}
}
/*
x=1 y=1
x=1 y=2
x=1 y=3
x=2 y=1
x=2 y=2
x=2 y=3
x=3 y=1
x=3 y=2
x=3 y=3
*/
while loop
initialize variable;
while(condition check){
//block of statments
increment/decrement variable
}
public class Main {
public static void main(String[] args) {
int val = 1;
while (val<=5){
System.out.println(val);
val++;
}
}
}
/*
1
2
3
4
5
*/
do-while loop
initialize variable;
do{
//block of statments
increment/decrement variable
}while(condition check);
public class Main {
public static void main(String[] args) {
int val = 1;
do{
System.out.println(val);
val++;
}while (val<=5);
}
}
/*
1
2
3
4
5
*/
for-each loop
public class Main {
public static void main(String[] args) {
int valArray[] = {1,2,3,4,5};
for (int val: valArray){
System.out.println(val);
}
}
}
/*
1
2
3
4
5
*/
Branching Statements
Break Statement
public class Main {
public static void main(String[] args) {
for (int val=1; val<=10; val++){
if (val == 3){
break;
}
System.out.println(val);
}
}
}
/*
1
2
*/
public class Main {
public static void main(String[] args) {
for (int outerLoop = 1; outerLoop<=5; outerLoop++){
for (int innerLoop =1; innerLoop<=5; innerLoop++){
if (innerLoop ==2){
break;
}
System.out.println(outerLoop+", "+innerLoop);
}
}
}
}
/*
1, 1
2, 1
3, 1
4, 1
5, 1
*/
Continue Statement
public class Main {
public static void main(String[] args) {
for (int val=1; val<=10; val++){
if (val == 3){
continue;
}
System.out.println(val);
}
}
}
/*
1
2
4
5
6
7
8
9
10
*/
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
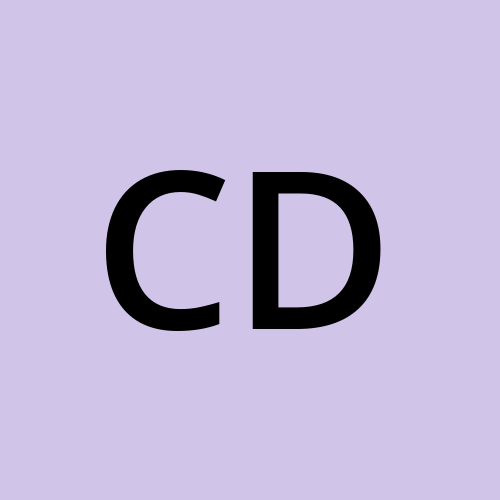
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.