Jumping Ball Progress
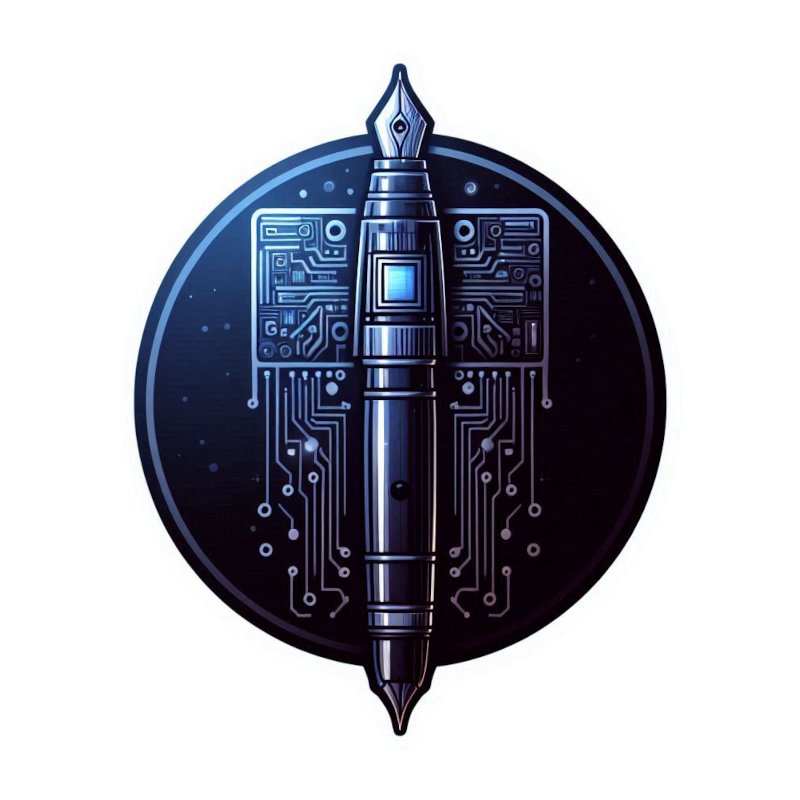
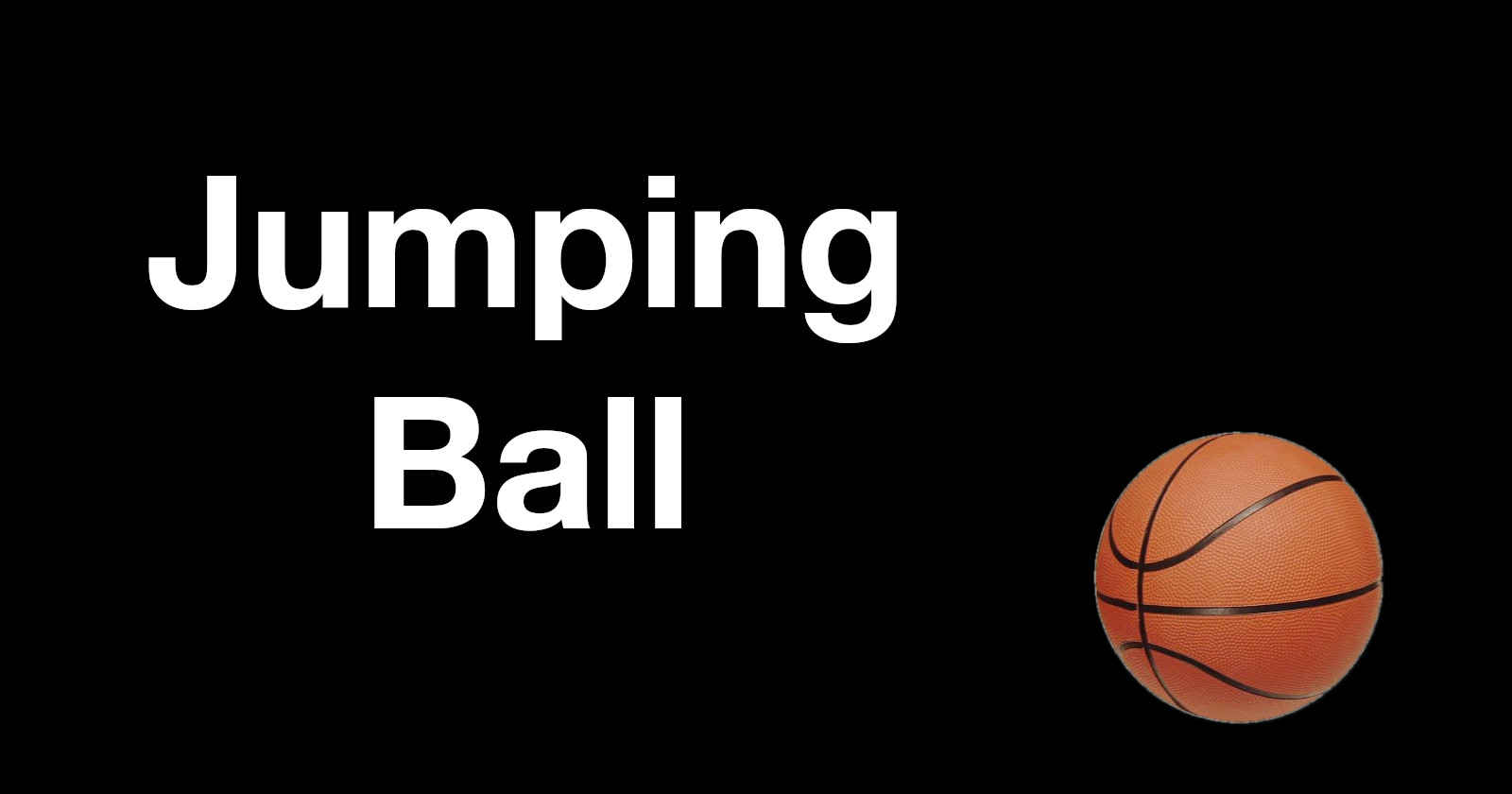
It took me quite a bit to figure out how to handle the ball's behaviour. Using print statements and studying how a ball should work, I managed to write some logic for it. I added new attributes to the ball struct and used them to manage the calculations for the ball's behavior.
Results struct
struct Results
{
double maxHeight; // calculate max height (remove)
double timeToMaximumHeight; // time to maximum height (remove)
double timeToLand; // time to land (remove)
int initialVelocity; // original text box value
int operatedVelocity; // used for ball movement
double gravity; // earth's gravitational force
bool doResultsExist; // Check if the results can be applied
bool upwardsMovement; // The ball goes up or down?
};
I used the simple but useful distance = speed * time
formula to handle upwards and downwards movement. For each bounce I divide the initial velocity by 1.1 to lower the height of each bounce.
When the operated velocity reaches zero or the ball hits the ground, the ball changes direction. If the ball hits the ground, the initial velocity decreases. This behavior continues as long as the initial velocity is greater than 1. Once the initial velocity is 1 or less, the simulation ends, and the application resets.
Ball movement behavior
if(hasSimStarted)
{
if (executionResults.operatedVelocity > 0)
{
if (executionResults.upwardsMovement)
{
ball.y -= (double)executionResults.operatedVelocity;
executionResults.operatedVelocity -= (int)executionResults.gravity * deltaTime;
}else
{
ball.y += (double)executionResults.operatedVelocity;
executionResults.operatedVelocity += (int)executionResults.gravity * deltaTime;
if (ball.y > windowHeight - ball.radius)
{
executionResults.operatedVelocity = 0;
}
}
}else if (executionResults.operatedVelocity <= 0)
{
if (!executionResults.upwardsMovement && executionResults.initialVelocity > 1)
{
executionResults.initialVelocity = executionResults.initialVelocity / 1.1;
}
executionResults.upwardsMovement = !executionResults.upwardsMovement;
executionResults.operatedVelocity = executionResults.initialVelocity;
if (executionResults.initialVelocity <= 1)
{
hasSimStarted = false;
}
}
startButton.text = "Stop";
}else
{
ball.y = windowHeight - ball.radius;
startButton.text = "Start";
}
GenerateBall(ball, appRenderer);
Demonstration
Subscribe to my newsletter
Read articles from Chris Douris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
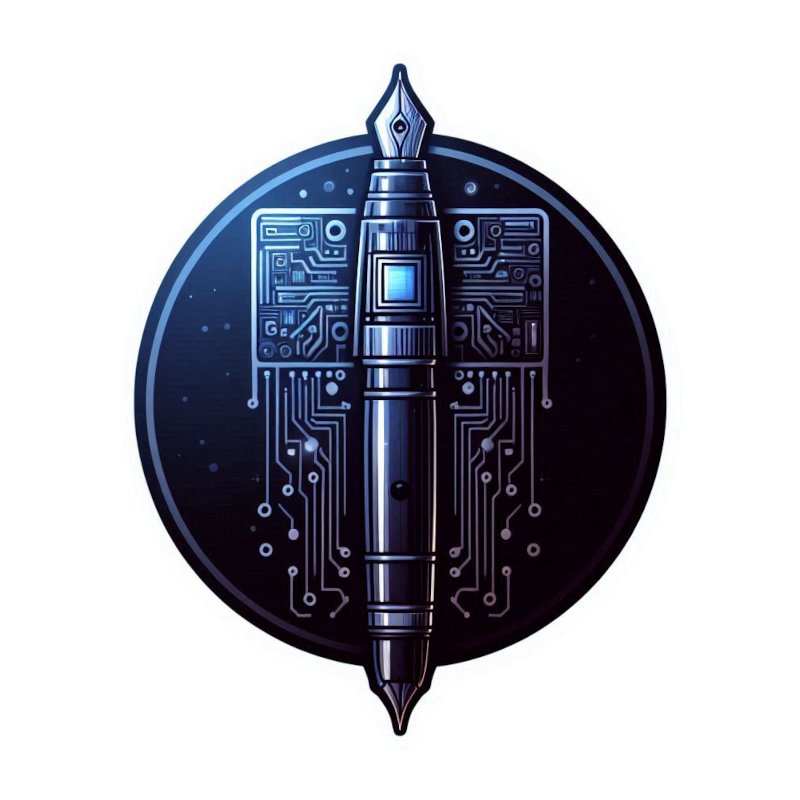
Chris Douris
Chris Douris
AKA Chris, is a software developer from Athens, Greece. He started programming with basic when he was very young. He lost interest in programming during school years but after an unsuccessful career in audio, he decided focus on what he really loves which is technology. He loves working with older languages like C and wants to start programming electronics and microcontrollers because he wants to get into embedded systems programming.