How PDF Converters are Developed: A Step-by-Step Guide
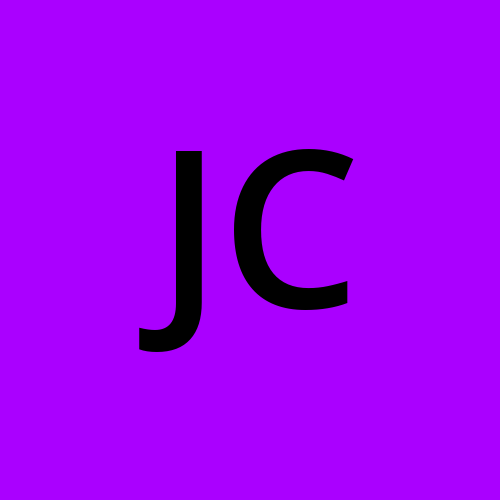
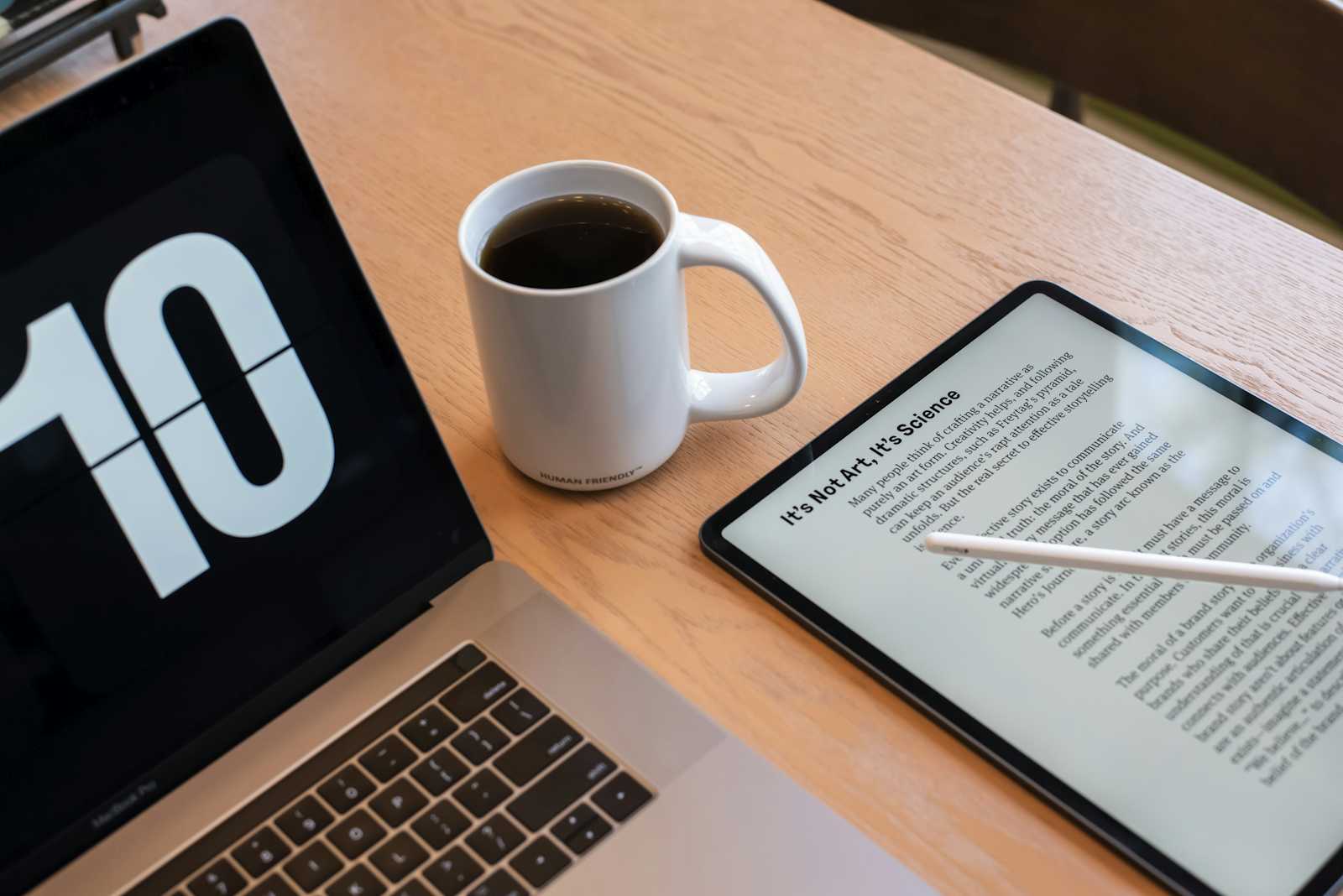
In the digital age, the need for converting documents into PDF format and vice versa is indispensable. PDF converters facilitate the seamless transition between various file formats and PDF, a format renowned for its reliability and versatility. This article delves into the intricate process of coding PDF converters, bringing them to life, and making them accessible online.
The Building Blocks: Understanding PDF and File Formats
Before delving into the coding aspect, it's essential to grasp the basics of the PDF format and other common file types. PDFs (Portable Document Format) are standardized, device-independent files created by Adobe. They encapsulate text, images, and other elements in a fixed layout, making them ideal for sharing documents across different platforms without altering their appearance.
Other file formats, such as DOCX (Word documents), JPG (images), and HTML (web pages), each have unique structures and encoding methods. Converting between these formats and PDFs requires parsing, transforming, and reconstructing the data accurately.
Coding the Core: The Conversion Algorithms
Parsing and Data Extraction
The first step in any file conversion process is parsing. Parsing involves reading and interpreting the data structure of the source file. For instance, when converting a DOCX file to PDF, the converter reads the DOCX file's XML content, extracting text, images, styles, and other elements.
import docx
def extract_text_from_docx(docx_path):
doc = docx.Document(docx_path)
text = ""
for paragraph in doc.paragraphs:
text += paragraph.text + "\n"
return text
In the example above, the extract_text_from_docx
function reads a DOCX file and extracts its text content. Similar methods are employed for other file formats, utilizing libraries tailored to each format's intricacies.
Data Transformation
After extraction, the data must be transformed into a structure suitable for PDF formatting. This involves converting text styles, images, and other elements into PDF-compatible formats. Libraries like ReportLab for Python facilitate this transformation by providing tools to create PDFs programmatically.
from reportlab.lib.pagesizes import letter
from reportlab.pdfgen import canvas
def create_pdf_from_text(text, pdf_path):
c = canvas.Canvas(pdf_path, pagesize=letter)
width, height = letter
# Simple text insertion example
c.drawString(100, height - 100, text)
c.save()
text = "Hello, this is a PDF conversion example."
create_pdf_from_text(text, "output.pdf")
In this snippet, the create_pdf_from_text
function generates a simple PDF from the provided text. More complex converters handle styles, fonts, and images, building a fully formatted PDF.
Rendering and Output
The final step is rendering the transformed data into a PDF file. This step consolidates the parsed and transformed data into a cohesive document, ensuring all elements are correctly positioned and formatted.
Bringing Converters to Life: Web Integration
Backend Development
To make PDF converters accessible online, backend development is crucial. The backend server handles user requests, processes files, and returns the converted output. Frameworks like Flask (Python), Express (Node.js), or Django (Python) are commonly used for this purpose.
from flask import Flask, request, send_file
import io
app = Flask(__name__)
@app.route('/convert', methods=['POST'])
def convert_file():
file = request.files['file']
text = extract_text_from_docx(file.stream)
pdf_buffer = io.BytesIO()
create_pdf_from_text(text, pdf_buffer)
pdf_buffer.seek(0)
return send_file(pdf_buffer, as_attachment=True, download_name='output.pdf', mimetype='application/pdf')
if __name__ == "__main__":
app.run(debug=True)
This Flask application defines an endpoint /convert
where users can upload a file. The server processes the file and returns a converted PDF.
Frontend Development
The frontend provides the user interface, enabling users to interact with the converter seamlessly. Technologies like HTML, CSS, and JavaScript are used to create an intuitive experience. For example, a simple HTML form can be used to upload files.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PDF Converter</title>
</head>
<body>
<h1>PDF Converter</h1>
<form action="/convert" method="post" enctype="multipart/form-data">
<input type="file" name="file" accept=".docx" required>
<button type="submit">Convert to PDF</button>
</form>
</body>
</html>
Deployment
The final step is deploying the application online. Cloud platforms like AWS, Google Cloud, or Heroku provide scalable infrastructure for hosting web applications. CI/CD pipelines ensure that updates are seamlessly integrated into the live environment.
Ensuring Quality and Reliability
Testing
Thorough testing is imperative to ensure the converter handles various file types and edge cases gracefully. Unit tests, integration tests, and user acceptance tests are conducted to identify and fix bugs.
Security
Security measures are implemented to protect user data. This includes securing file uploads, preventing malicious file executions, and ensuring data privacy. HTTPS encryption and secure file handling practices are standard protocols.
Performance Optimization
Optimizing the conversion process for speed and efficiency is crucial, especially for large files. Techniques like caching, parallel processing, and efficient algorithms contribute to a responsive user experience.
Conclusion
Creating a PDF converter involves a blend of parsing, data transformation, rendering, and web integration. From coding the core algorithms to building user-friendly interfaces and deploying the application online, each step requires meticulous attention to detail. As technology evolves, so too will the sophistication of PDF converters, making document management more seamless and accessible for users worldwide.
Some Great PDF Converters Online are available.
iLovePDF3
Website: iLovePDF3
Features: Offers a wide range of PDF tools For Free, including conversion to and from PDF, merging, splitting, compressing, and editing PDFs. It supports various file formats such as Word, Excel, PowerPoint, and images.
Smallpdf
Website: Smallpdf
Features: Provides a comprehensive suite of PDF tools, including conversion, compression, editing, and e-signing. Smallpdf is known for its user-friendly interface and fast processing speeds.
PDF Converter
Website: PDF Converter
Features: Focuses on converting PDFs to and from multiple file formats, such as Word, Excel, PowerPoint, and images. It also offers tools for merging, splitting, and compressing PDFs.
PDF2Go
Website: PDF2Go
Features: A versatile platform that provides PDF conversion, editing, and optimization tools. It supports various input and output formats and allows for online and offline use through its mobile app.
Soda PDF
Website: Soda PDF
Features: Offers a full suite of PDF tools, including conversion, editing, reviewing, and e-signing. Soda PDF also provides cloud integration, allowing users to access and manage their files from anywhere.
Each of these websites offers robust features and a user-friendly experience for all your PDF conversion and editing needs.
Subscribe to my newsletter
Read articles from Jackson Campbell directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
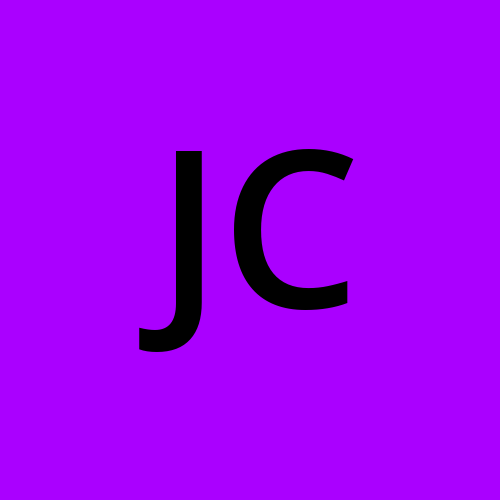
Jackson Campbell
Jackson Campbell
Hello, I am JackSon i am a Website developer and Writer. I like to write about new tech and news related to it stay tuned for it.