Forms, Semantic Tags and Links
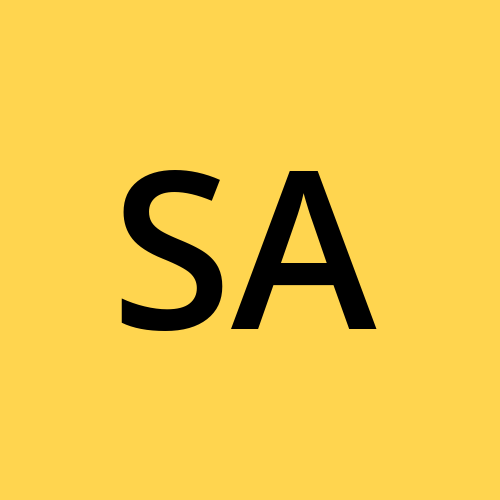
Table of contents
Forms and Input types
HTML forms are essential for collecting user input and submitting it to a server for processing. Forms can include various types of input elements like text fields, checkboxes, radio buttons, and submit buttons. Here’s an in-depth explanation of forms, input elements, and labels, including their attributes.
HTML Form (<form>
)
The <form>
element is a container for different types of input elements. It defines how the form data will be sent to the server.
Attributes:
action
: Specifies the URL where the form data will be sent when the form is submitted.- Example:
<form action="/submit-form">
- Example:
method
: Defines the HTTP method to be used when sending the form data. Common values areGET
(default) andPOST
.- Example:
<form method="post">
- Example:
name
: Specifies the name of the form.- Example:
<form name="myForm">
- Example:
target
: Specifies where to display the response received after submitting the form. Common values are_self
(default),_blank
,_parent
,_top
.- Example:
<form target="_blank">
- Example:
autocomplete
: Specifies whether the form should have autocomplete enabled. Possible values areon
(default) andoff
.- Example:
<form autocomplete="off">
- Example:
HTML Input (<input>
)
The <input>
element is used to create various types of user input fields.
Common Attributes:
type
: Specifies the type of input. Common values includetext
,password
,submit
,reset
,checkbox
,radio
,file
,hidden
,image
,button
,color
,date
,datetime-local
,email
,month
,number
,range
,search
,tel
,time
,url
, andweek
.<input type="text"> <input type="password"> <input type="submit"> <input type="reset"> <!-- ....And so on -->
name
: Specifies the name of the input element, used to identify the form data after it’s submitted.- Example:
<input type="text" name="username">
- Example:
value
: Specifies the initial value of the input element.- Example:
<input type="text" value="Aquib Ali">
- Example:
placeholder
: Provides a hint to the user of what can be entered in the input field.- Example:
<input type="text" placeholder="Enter your name">
- Example:
required
: Specifies that the input field must be filled out before submitting the form.- Example:
<input type="text" required>
- Example:
readonly
: Makes the input field read-only.- Example:
<input type="text" readonly>
- Example:
maxlength
: Specifies the maximum number of characters allowed in the input field.- Example:
<input type="text" maxlength="10">
- Example:
pattern
: Specifies a regular expression the input’s value must match for the form to be submitted.- Example:
<input type="text" pattern="[A-Za-z]{3,}">
- Example:
autofocus
: Specifies that the input field should automatically get focus when the page loads.- Example:
<input type="text" autofocus>
- Example:
autocomplete
: Specifies whether the input field should have autocomplete enabled. Possible values areon
andoff
.- Example:
<input type="text" autocomplete="on">
- Example:
min
: Specifies the minimum value fornumber
,range
,date
,datetime-local
,month
,week
,time
inputs.- Example:
<input type="number" min="1">
- Example:
max
: Specifies the maximum value fornumber
,range
,date
,datetime-local
,month
,week
,time
inputs.- Example:
<input type="number" max="10">
- Example:
HTML Label (<label>
)
The <label>
element is used to define a label for an input element. Clicking on the label focuses the associated input element.
Attributes:
for
: Specifies which form element a label is bound to by matching theid
of the input element.- Example:
<label for="username">Username:</label> <input type="text" id="username" name="username">
- Example:
Here’s a complete example of a form with various input elements and labels:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Form Example</title>
</head>
<body>
<form action="/submit-form" method="post">
<div>
<label for="username">Username:</label>
<input type="text" id="username" name="username" placeholder="Enter your username" required maxlength="15">
</div>
<div>
<label for="password">Password:</label>
<input type="password" id="password" name="password" placeholder="Enter your password" required>
</div>
<div>
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter your email" required>
</div>
<div>
<label for="birthdate">Birthdate:</label>
<input type="date" id="birthdate" name="birthdate" required>
</div>
<div>
<label for="file">Upload File:</label>
<input type="file" id="file" name="file">
</div>
<div>
<label for="color">Favorite Color:</label>
<input type="color" id="color" name="color" value="#000000">
</div>
<div>
<label for="range">Volume:</label>
<input type="range" id="range" name="range" min="0" max="100" step="10">
</div>
<div>
<input type="checkbox" id="subscribe" name="subscribe">
<label for="subscribe">Subscribe to newsletter</label>
</div>
<div>
<input type="radio" id="male" name="gender" value="male">
<label for="male">Male</label>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label>
</div>
<div>
<input type="submit" value="Submit">
<input type="reset" value="Reset">
</div>
</form>
</body>
</html>
Description of the code:
Form Container: The
<form>
element hasaction
andmethod
attributes.Text Input: The
username
input field with attributes likeplaceholder
,required
, andmaxlength
.Password Input: The
password
input field with arequired
attribute.Email Input: The
email
input field withplaceholder
andrequired
attributes.Date Input: The
birthdate
input field with arequired
attribute.File Input: The
file
input field for file uploads.Color Input: The
color
input field to select a color.Range Input: The
range
input field to select a value within a range.Checkbox: The
subscribe
checkbox with a label.Radio Buttons:
gender
radio buttons for selecting gender with labels.Submit and Reset Buttons:
submit
andreset
input types to submit or reset the form data.
Links
HTML links are created using the <a>
(anchor) element. Links can be used to navigate to other web pages, download files, initiate phone calls, send emails, and more. The primary attribute used with the <a>
element is href
, which specifies the destination of the link.
Basic HTML Link
- The most common use of an
<a>
element is to create a hyperlink to another web page.
<a href="">Add your link inside the href's ""</a>
Linking to an External Website
- To link to an external website, we use the
href
attribute with the full URL of the destination.
<a href="https://www.google.com">Go to Google</a>
<!-- Do never forget to add a full address of the link as shown above,
we included https:// as well because thats how a real link looks like.
-->
- In this example, clicking the text "Go to Google" will take the user to "https://www.google.com".
Linking to a Specific Section on the Same Page
- You can link to a specific section on the same page using the
id
attribute of an element.
<!-- Section to link to -->
<h2 id="section1">Section 1</h2>
<p>This is section 1 content.</p>
<!-- Link to the section -->
<a href="#section1">Go to Section 1</a>
- This is commonly used when we want to make a navigation section where we link to each section of our topics. Just like how hashnode has done where you are reading this article right now. (There's a table section at top of this article that navigates you to various topics I have discussed so far.)
Linking to a Different Page and a Specific Section on That Page
- You can also link to a specific section on a different page.
<a href="otherpage.html#section2">Go to Section 2 on Other Page</a>
Opening Links in a New Tab or Window
- To open a link in a new tab or window, use the
target
attribute with the value_blank
.
<a href="https://www.example.com" target="_blank">Open Example.com in a new tab</a>
Linking to an Email Address
- To create a link that opens the user's default email client with a pre-filled recipient email address, use the
mailto:
scheme.
<a href="mailto:someone@example.com">Send Email</a>
- You can also include a subject and body text.
<a href="mailto:someone@example.com?subject=Hello&body=How are you?">Send Email with Subject and Body</a>
Linking to a Phone Number
- To create a link that initiates a phone call on mobile devices, use the
tel:
scheme.
<a href="tel:+9123456789">Call +91 23456789</a>
Downloading a File
- To create a link that downloads a file, use the
download
attribute.
<a href="files/example.pdf" download>Download PDF</a>
Creating an Image Link
- You can use an image as a link by placing the
<img>
element inside an<a>
element.
<a href="https://www.example.com">
<img src="images/logo.png" alt="Example Logo">
</a>
Semantic Elements
Semantic elements in HTML provide meaning to the web content and help search engines and other devices understand the structure and importance of web pages. These elements are designed to define different parts of a web page more clearly and semantically. Here’s an explanation of some key semantic elements:
<article>
The <article>
element represents a self-contained piece of content that is intended to be independently reusable. This could be a blog post, a news article, a forum post, or any other independent item of content.
<article>
<h2>Understanding HTML Semantic Elements</h2>
<p>Semantic elements in HTML provide meaning to the web content and help search engines...</p>
</article>
<aside>
The <aside>
element represents content that is tangentially related to the content around it. This is often used for sidebars, pull quotes, advertising, or groups of related content that are off to the side.
<aside>
<h3>Related Articles</h3>
<ul>
<li><a href="#">HTML5 New Features</a></li>
<li><a href="#">CSS Grid Layout</a></li>
</ul>
</aside>
<figure>
The <figure>
element is used to encapsulate media content, such as images, illustrations, diagrams, code snippets etc.
<figure>
<img src="diagram.png" alt="A process flow diagram">
<p>Figure 1: Process flow diagram of the system architecture.</p>
</figure>
<figcaption>
The <figcaption>
element is used to provide a caption or legend for a <figure>
element. It typically describes the image, chart, or other media contained within the <figure>
.
<figure>
<img src="image.jpg" alt="A descriptive image">
<figcaption>A beautiful scenery of mountains during sunset.</figcaption>
</figure>
<!-- In the before example we were using p element to render our paragraph,
but in this example we used figcaption since it is the replacement of p element
in figure section -->
<footer>
The <footer>
element represents a footer for its nearest sectioning content or sectioning root element (e.g., a section, article, or the entire page). It typically contains information about the author, copyright information, links to related documents, etc.
<footer>
<p>© 2024 Example Company. All rights reserved.</p>
<!-- The © entity is used to display the copyright
symbol (©) in web content. -->
<nav>
<ul>
<li><a href="#">Privacy Policy</a></li>
<li><a href="#">Terms of Service</a></li>
</ul>
</nav>
</footer>
<header>
The <header>
element represents introductory content. It can contain headings, navigational links, logos, and other elements.
<header>
<h1>My Website</h1>
<div>
<ul>
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</div>
</header>
<main>
The <main>
element represents the main content of the <body>
of a document. It should contain content that is unique to the document, excluding things like sidebars, navigation links, headers, and footers.
<main>
<article>
<h2>Welcome to My Blog</h2>
<p>This is the first post on my new blog...</p>
</article>
</main>
<nav>
The <nav>
element represents a section of a page that links to other pages or to parts within the page: a section with navigation links.
<header>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#services">Services</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<!-- In the header explanation before, we did not use nav element.
We should always use nav element if we are creating a navigation bar.
-->
Here’s how these semantic elements can be used together in a complete HTML webpage:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Semantic HTML Example</title>
<style>
body {
font-family: Arial, sans-serif;
}
header, nav, main, aside, footer {
margin: 10px 0;
padding: 10px;
border: 1px solid #ccc;
}
header {
background-color: #f2f2f2;
}
footer {
background-color: #f2f2f2;
text-align: center;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin-right: 10px;
}
</style>
</head>
<body>
<header>
<h1>My Website</h1>
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<article>
<h2>Welcome to My Blog</h2>
<p>This is the first post on my new blog...</p>
</article>
<aside>
<h3>Related Articles</h3>
<ul>
<li><a href="#">HTML5 New Features</a></li>
<li><a href="#">CSS Grid Layout</a></li>
</ul>
</aside>
</main>
<footer>
<p>© 2024 Example Company. All rights reserved.</p>
<nav>
<ul>
<li><a href="#">Privacy Policy</a></li>
<li><a href="#">Terms of Service</a></li>
</ul>
</nav>
</footer>
</body>
</html>
Code explanation:
<header>
: Contains the main heading of the website and a navigation menu.<nav>
: Provides navigation links within the header and footer sections.<main>
: Encloses the main content area of the page, including an article and an aside.<article>
: Represents a blog post within the main content.<aside>
: Contains related links, acting as a sidebar.<footer>
: Contains copyright information and a secondary navigation menu.
WHY SEMANTIC ELEMENTS? : Using semantic HTML elements makes your web pages more accessible, SEO (search engine optimized) friendly, and easier to maintain. They provide a clear structure that both browsers and search engines can understand, leading to better performance and user experience. It can also improves the accessibility of your webpage.
(BONUS) Meta Tags
Meta tags in HTML provide metadata about the HTML document, which is used by browsers, search engines, and other web services. These tags are placed within the <head>
section of an HTML document and do not display any content to users directly. Instead, they communicate with search engines and browsers to provide information such as page description, keywords, author, and viewport settings.
Common Meta Tags
1. charset
- Specifies the character encoding for the HTML document.
<meta charset="UTF-8">
2. name
and content
- Used for a variety of purposes such as specifying the document’s description, keywords, author, and more.
Description:
- Provides a summary of the page content. Search engines often use this in search results.
<meta name="description" content="This is an example of a meta description.">
Keywords:
- Lists keywords relevant to the content. This is less important for SEO today.
<meta name="keywords" content="HTML, CSS, JavaScript, web development">
Author:
- Specifies the author of the document.
<meta name="author" content="Aquib Ali">
Viewport:
- Controls the layout on mobile browsers.
<meta name="viewport" content="width=device-width, initial-scale=1.0">
4. property
and content
- Used for Open Graph protocol to integrate web pages with social media platforms like Facebook, Twitter, etc.
Open Graph:
- Specifies the title, type, image, and description of the page.
<meta property="og:title" content="Example Title">
<meta property="og:type" content="website">
<meta property="og:image" content="https://example.com/image.jpg">
<meta property="og:description" content="This is an example description for social media.">
WHY META TAGS? : Using meta tags effectively can improve your website's search engine optimization (SEO), enhance user experience, and ensure better compatibility with browsers and social media platforms.
Subscribe to my newsletter
Read articles from Syed Aquib Ali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
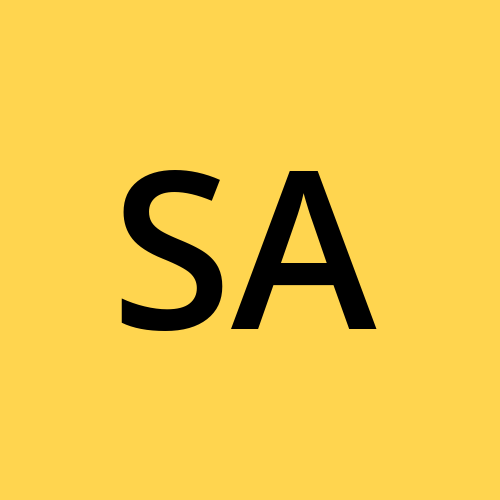
Syed Aquib Ali
Syed Aquib Ali
I am a MERN stack developer who has learnt everything yet trying to polish his skills 🥂, I love backend more than frontend.