Mastering Closures in Go
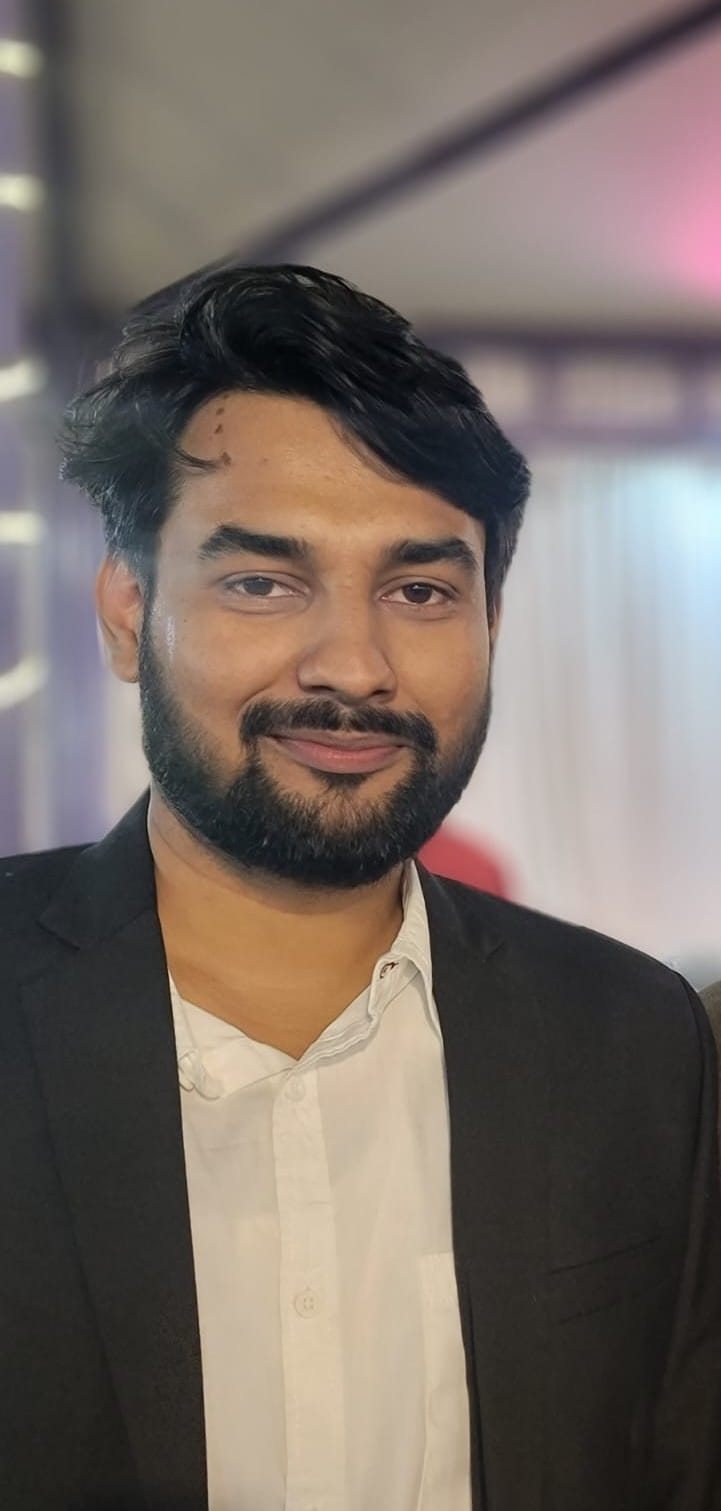
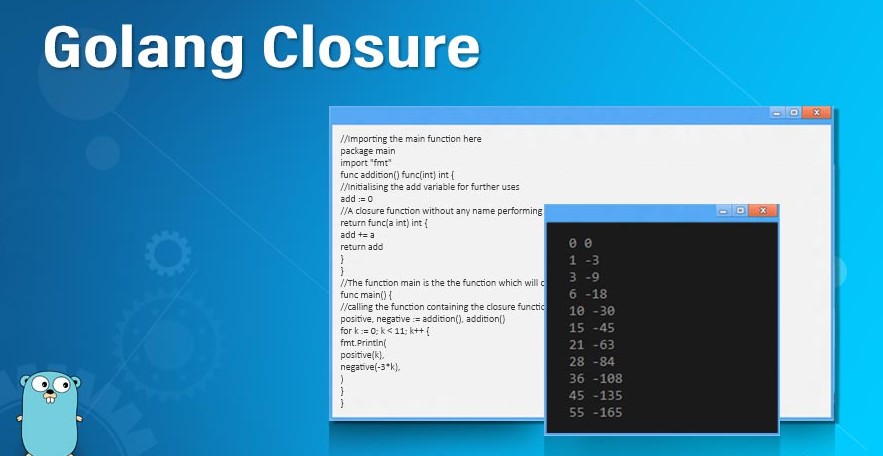
Closures are a fundamental concept in programming that allow functions to capture and use variables from their surrounding scope. In Go, closures are used extensively to create higher-order functions and to implement functional programming concepts. If you're familiar with closures in JavaScript, you'll find that they work similarly in Go.
What are Closures?
A closure is a function that has access to its own scope and the scope of its surrounding environment. This means that a closure can use and modify variables from its surrounding scope, even after the surrounding function has returned.
Example in Go
Here's an example of a closure in Go:
package main
import "fmt"
func outer() func() int {
x := 0
return func() int {
x++
return x
}
}
func main() {
f := outer()
fmt.Println(f()) // prints 1
fmt.Println(f()) // prints 2
fmt.Println(f()) // prints 3
}
In this example, the outer
function returns a closure that increments a variable x
each time it is called. The closure has access to the variable x
from its surrounding scope, even after the outer
function has returned.
Benefits of Closures
Closures provide several benefits, including:
Encapsulation: Closures allow you to encapsulate data and behavior together, making it easier to manage complex logic.
Higher-Order Functions: Closures enable the creation of higher-order functions, which are functions that take other functions as arguments or return functions as values.
Functional Programming: Closures are a key component of functional programming, which emphasizes the use of pure functions and immutability.
Closures in JavaScript
If you're familiar with JavaScript, you'll find that closures work similarly in Go. In JavaScript, closures are used extensively to create higher-order functions, implement callbacks, and manage scope. Here's an example of a closure in JavaScript:
function outer() {
let x = 0;
return function inner() {
x++;
return x;
};
}
let f = outer();
console.log(f()); // prints 1
console.log(f()); // prints 2
console.log(f()); // prints 3
This code demonstrates how the inner
function has access to the variable x
from its surrounding scope, even after the outer
function has returned.
Conclusion
Closures are a powerful tool in Go that allow you to create higher-order functions and implement functional programming concepts. By understanding how to use closures effectively, you can write more concise, efficient, and scalable code.
If you're familiar with closures in JavaScript, you'll find that they work similarly in Go. Mastering closures can help you write more expressive and flexible code in both languages.
Subscribe to my newsletter
Read articles from Lokesh Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
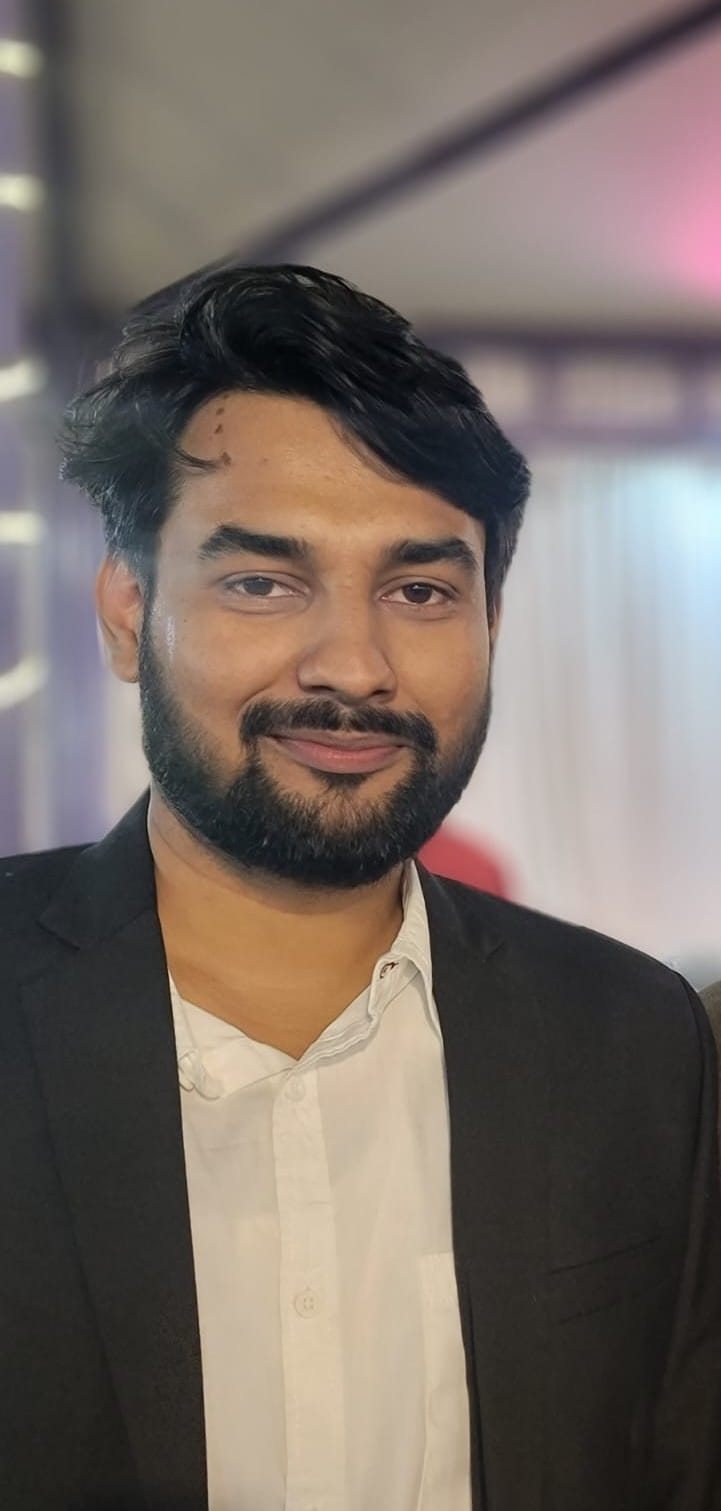
Lokesh Jha
Lokesh Jha
I am a senior software developer and technical writer who loves to learn new things. I recently started writing articles about what I've learned so that others in the community can gain the same knowledge. I primarily work with Node.js, TypeScript, and JavaScript, but I also have past experience with Java and C++. Currently, I'm learning Go and may explore Web3 in the future.