Day 29 Task: Jenkins Important interview Questions.
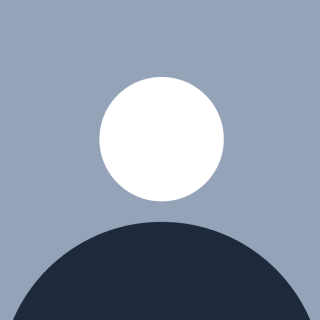
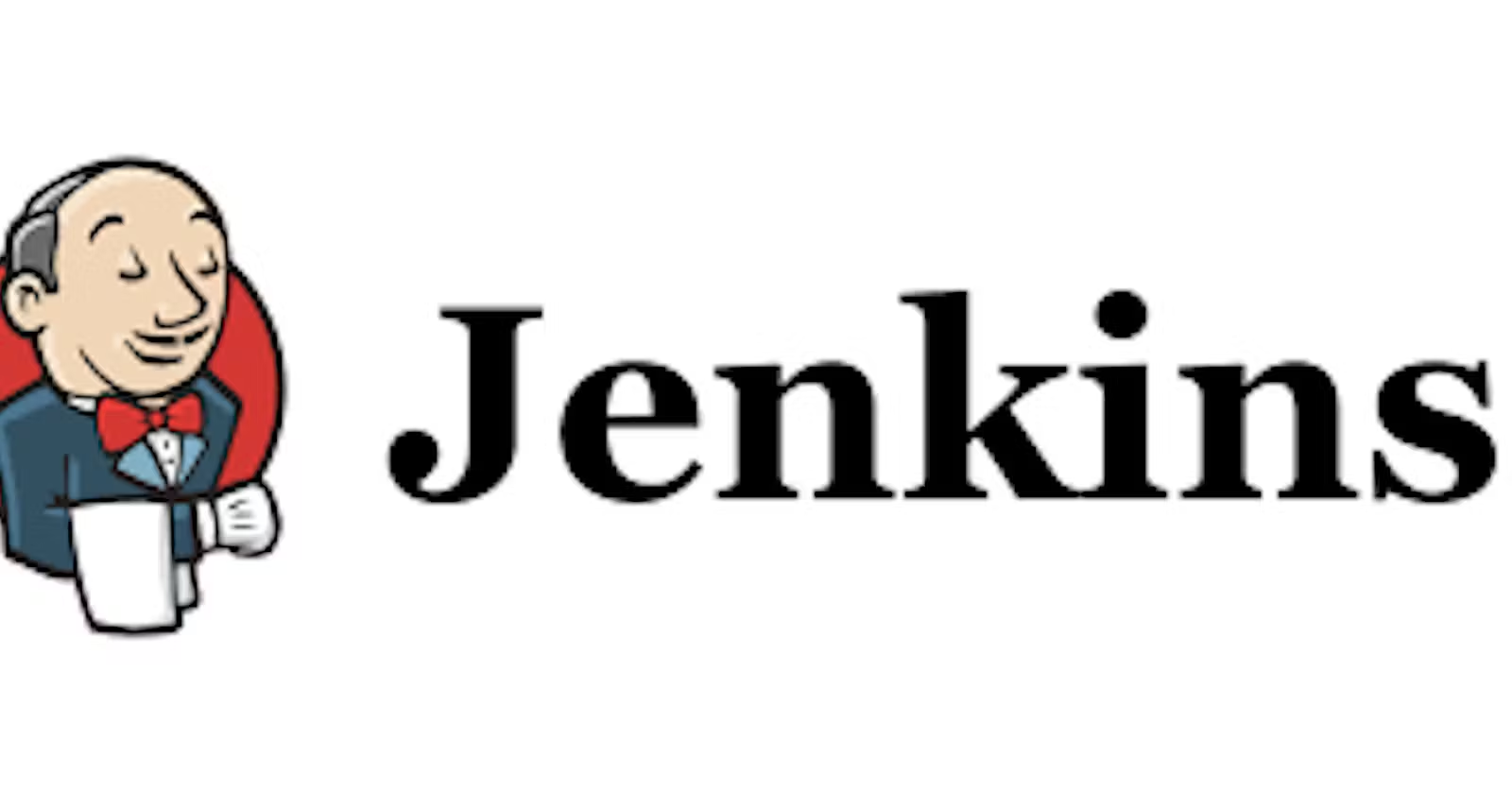
Here are some Jenkins-specific questions related to Docker that one can use during a DevOps Engineer interview:
Questions
- What’s the difference between continuous integration, continuous delivery, and continuous deployment?
Continuous Integration (CI) is the practice of frequently integrating code changes into a shared repository, where automated tests are run to ensure that the changes do not break the existing codebase.
Continuous Delivery (CD) is the practice of ensuring that code changes can be released to production at any time by automating the software release process, including testing, deployment, and infrastructure provisioning.
Continuous Deployment (CD) is an extension of continuous delivery where every change that passes automated tests is automatically deployed to production without manual intervention.
In short:
CI: Integrating code changes frequently with automated testing.
CD: Automating the process of delivering code changes to production.
Continuous Deployment: Automatically deploying code changes to production after passing automated tests.
Benefits of CI/CD
Faster Time to Market: Automating build, test, and deployment processes reduces manual errors and speeds up software delivery.
Increased Efficiency: Automated testing and deployment streamline development workflows, allowing teams to focus more on coding and less on repetitive tasks.
Enhanced Quality: Continuous integration ensures that code changes are tested early and frequently, leading to fewer bugs and higher-quality software.
Improved Collaboration: CI/CD encourages collaboration among developers, testers, and operations teams, leading to better communication and faster issue resolution.
Risk Reduction: Automated testing and deployment catch issues early, minimizing the risk of deploying faulty code into production environments.
Scalability: CI/CD pipelines are easily scalable, allowing teams to handle increasing workloads without compromising efficiency or quality.
Feedback Loop: Continuous integration provides rapid feedback on code changes, enabling developers to iterate and improve code quality continuously.
Cost Savings: By reducing manual effort and catching errors early, CI/CD ultimately saves time and resources, leading to cost savings for organizations.
What is meant by CI-CD?
CI/CD stands for Continuous Integration and Continuous Delivery (or Continuous Deployment). It's a software development practice where code changes are frequently integrated into a shared repository, and automated tests and builds are run. This allows for rapid and reliable delivery of code changes into production.
What is Jenkins Pipeline?
Jenkins Pipeline is a suite of plugins that allows you to define and manage your continuous delivery pipeline as code. It enables you to script your build, test, and deployment processes, making them repeatable, scalable, and easier to manage.
How do you configure the job in Jenkins?
OR
How build job in Jenkins?
To configure a job in Jenkins, follow these steps:
Log in to Jenkins and navigate to the dashboard.
Click on "New Item" to create a new job.
Enter a name for your job and select the type of job you want to create (e.g., Freestyle project, Pipeline).
Configure the job settings such as source code management, build triggers, build steps, post-build actions, etc.
Save your configuration.
Where do you find errors in Jenkins?
In Jenkins, errors can typically be found in the build console output, which provides detailed information about the build process, including any errors encountered. Additionally, Jenkins logs stored in the Jenkins server's file system can also provide insights into errors.
In Jenkins how can you find log files?
In Jenkins, log files are typically located in the "logs" directory within the Jenkins home directory. You can find them by navigating to
$JENKINS_HOME/logs
. The Jenkins home directory is usually/var/lib/jenkins
or/Users/your_username/.jenkins
, depending on your operating system.Jenkins workflow and write a script for this workflow?
Jenkins workflow is a way to define a series of tasks or steps to automate software development processes. Here's a basic example of a Jenkins workflow script:
pipeline { agent any stages { stage('Build') { steps { // Perform build steps here echo 'Building...' } } stage('Test') { steps { // Run tests here echo 'Testing...' } } stage('Deploy') { steps { // Deploy code to server or environment echo 'Deploying...' } } } post { success { echo 'Pipeline succeeded!' // Additional actions to take on success } failure { echo 'Pipeline failed :(' // Additional actions to take on failure } } }
This script defines a pipeline with three stages: Build, Test, and Deploy. Each stage contains steps to perform specific actions. The post section defines actions to take after the pipeline execution, such as displaying success or failure messages.
How to create continuous deployment in Jenkins?
To set up continuous deployment in Jenkins:
Install necessary plugins: Install plugins like Pipeline, Git, and others depending on your project needs.
Configure your project: Set up a Jenkins pipeline job using a Jenkinsfile that defines your deployment steps.
Define your pipeline stages: Outline stages like build, test, and deploy in your Jenkinsfile. Use Jenkins Pipeline syntax to define these stages.
Integrate version control: Connect Jenkins to your version control system (like Git) to trigger builds automatically when changes are pushed.
Automate deployment: Use tools like Docker, Kubernetes, or custom scripts within your Jenkins pipeline to automate deployment to your target environment.
Configure notifications and alerts: Set up notifications to inform teams about build status, deployment success, or failure.
Monitor and iterate: Monitor your pipeline's performance, gather feedback, and iterate to improve your deployment process.
Why we use pipeline in Jenkins?
In short, we use pipelines in Jenkins to automate and orchestrate the entire software delivery process, from code commit to deployment, ensuring consistency, repeatability, and efficiency in the software development lifecycle.
Is Only Jenkins enough for automation?
Jenkins is a powerful automation tool for continuous integration and continuous delivery (CI/CD), but it might not be sufficient for all automation needs. Depending on the complexity and diversity of your automation requirements, you may need additional tools or frameworks to complement Jenkins, such as Ansible, Puppet, or Kubernetes for infrastructure automation, or Selenium for web application testing.
How will you handle secrets?
In Jenkins, you can handle secrets using the built-in credentials plugin. This allows you to securely store sensitive information such as passwords, API keys, and SSH keys. You can then reference these credentials in your Jenkins jobs without exposing them directly in your configuration scripts. Additionally, you can use plugins like HashiCorp Vault integration for more advanced secret management.
Explain diff stages in CI-CD setup?
CI/CD (Continuous Integration/Continuous Delivery) setups typically involve the following stages:
Code Commit: Developers commit their code changes to a version control system like Git.
Automated Build: The CI system pulls the latest code changes, builds the application, and runs automated tests to ensure the code is functional.
Automated Testing: Various types of tests, including unit tests, integration tests, and sometimes even performance tests, are executed automatically to validate the code changes.
Deployment: If all tests pass, the code is deployed to a staging or production environment automatically.
Monitoring and Feedback Loop: Continuous monitoring of the deployed application helps to identify any issues or performance bottlenecks, providing feedback to developers for further improvements.
Name some of the plugins in Jenkins?
Git Plugin: Integrates Jenkins with Git, allowing for source code management.
Pipeline Plugin: Enables the creation of complex continuous delivery pipelines as code.
Credentials Plugin: Manages various credentials like usernames, passwords, API tokens, etc.
JUnit Plugin: Parses JUnit XML files to display test results within Jenkins.
Email Extension Plugin: Sends customizable email notifications based on build results.
Docker Plugin: Integrates Jenkins with Docker for building, packaging, and running containers.
Maven Plugin: Integrates Jenkins with Apache Maven for building Java projects.
SSH Plugin: Allows for executing commands over SSH from Jenkins.
Matrix Authorization Plugin: Provides fine-grained control over user permissions.
SonarQube Scanner Plugin: Integrates Jenkins with SonarQube for static code analysis.
Subscribe to my newsletter
Read articles from Vaishnavi Shivde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Vaishnavi Shivde
Vaishnavi Shivde
Aspiring DevOps Engineer | Linux | Git & Github | Shell Scripting | Docker | CI/CD Jenkins | Kubernetes | AWS | Terraform | JIRA | Python |