Tackling the Code Challenge: Articles - without SQLAlchemy: A Programmer's Journey

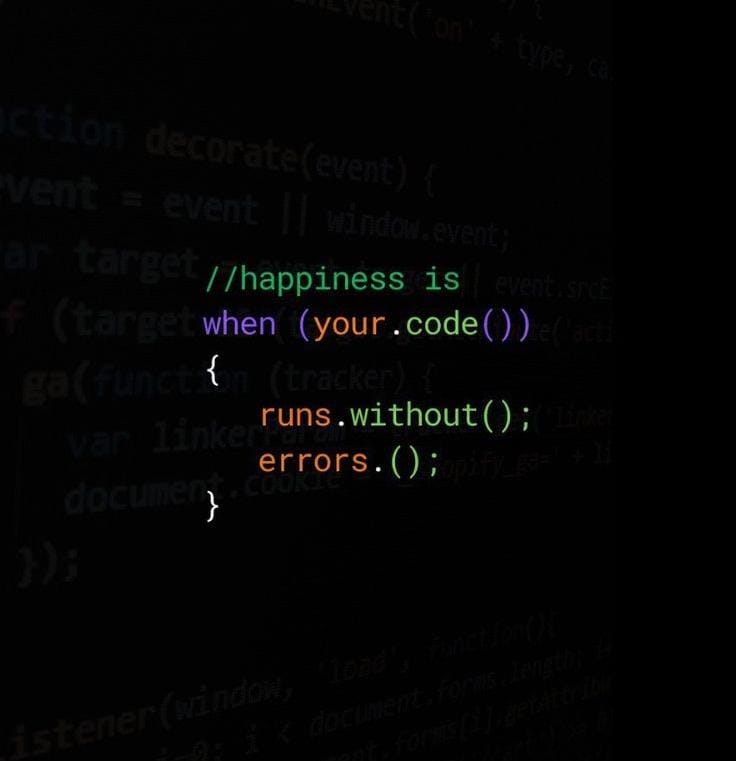
Introduction
As programmers, we constantly face challenges that push our understanding and skills to the limit. Recently, I embarked on a code challenge that involved implementing a many-to-many relationship in Python. This article will walk you through my experience, detailing the requirements, my approach, and the lessons learned along the way.
The Challenge
The objective was to create a system for managing articles, authors, and magazines. The requirements included:
1. Article Class:
Initialize with a title, author, and magazine.
Title must be an immutable string between 5 and 50 characters.
Association with
Author
andMagazine
classes.
2. Author Class:
Initialize with a name that is a non-empty string and immutable.
Methods to return the author's articles, magazines, and unique topic areas.
Ability to add a new article.
3. Magazine Class:
Initialize with a name (2-16 characters) and category, both non-empty strings.
Methods to return the magazine's articles, contributors, and unique article titles.
Identify authors who have written more than two articles for the magazine.
Determine the top publisher based on the number of articles.
Implementation
Article Class
The Article
class was straightforward. Ensuring the title met the criteria required additional checks, but the main complexity was maintaining the many-to-many relationship with authors and magazines.
Author Class
The Author
class needed methods to handle dynamic relationships. Implementing articles()
, magazines()
, and topic_areas()
involved iterating through associated articles and filtering unique entries. Creating new articles with add_article
was another layer of complexity.
Magazine Class
The Magazine
class required careful handling of its articles and contributors. Implementing articles()
, contributors()
, article_titles()
, and contributing_authors()
required robust list and set operations to ensure data integrity. The top_publisher()
method needed to iterate over all magazines to find the one with the most articles.
Testing
Testing was comprehensive, covering initialization, attribute constraints, and method outputs. Running pytest
ensured all edge cases were handled:
$ pytest
This command confirmed that all 32 tests passed, validating the robustness of my implementation.
Challenges and Lessons Learned
Understanding Requirements: Ensuring a clear understanding of the requirements was crucial. Misinterpreting even a small detail could lead to incorrect implementations.
Maintaining Relationships: Handling many-to-many relationships dynamically was challenging but rewarding. It required a deep understanding of list and set operations in Python.
Testing: Writing comprehensive tests was essential. It not only verified the correctness of the code but also helped identify edge cases early.
Repo Link
Here is the link to the repository:
Conclusion
This code challenge was a fantastic opportunity to deepen my understanding of Python. It was challenging, but the sense of accomplishment after all the tests passed was immense. If you're looking to improve your Python skills, I highly recommend tackling similar challenges. Happy coding!
Subscribe to my newsletter
Read articles from Deactivated User directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
