Static Site Generation (SSG) with Next.js: Boosting Performance and SEO
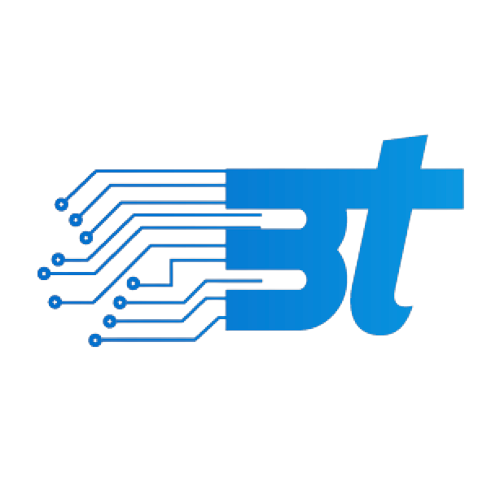
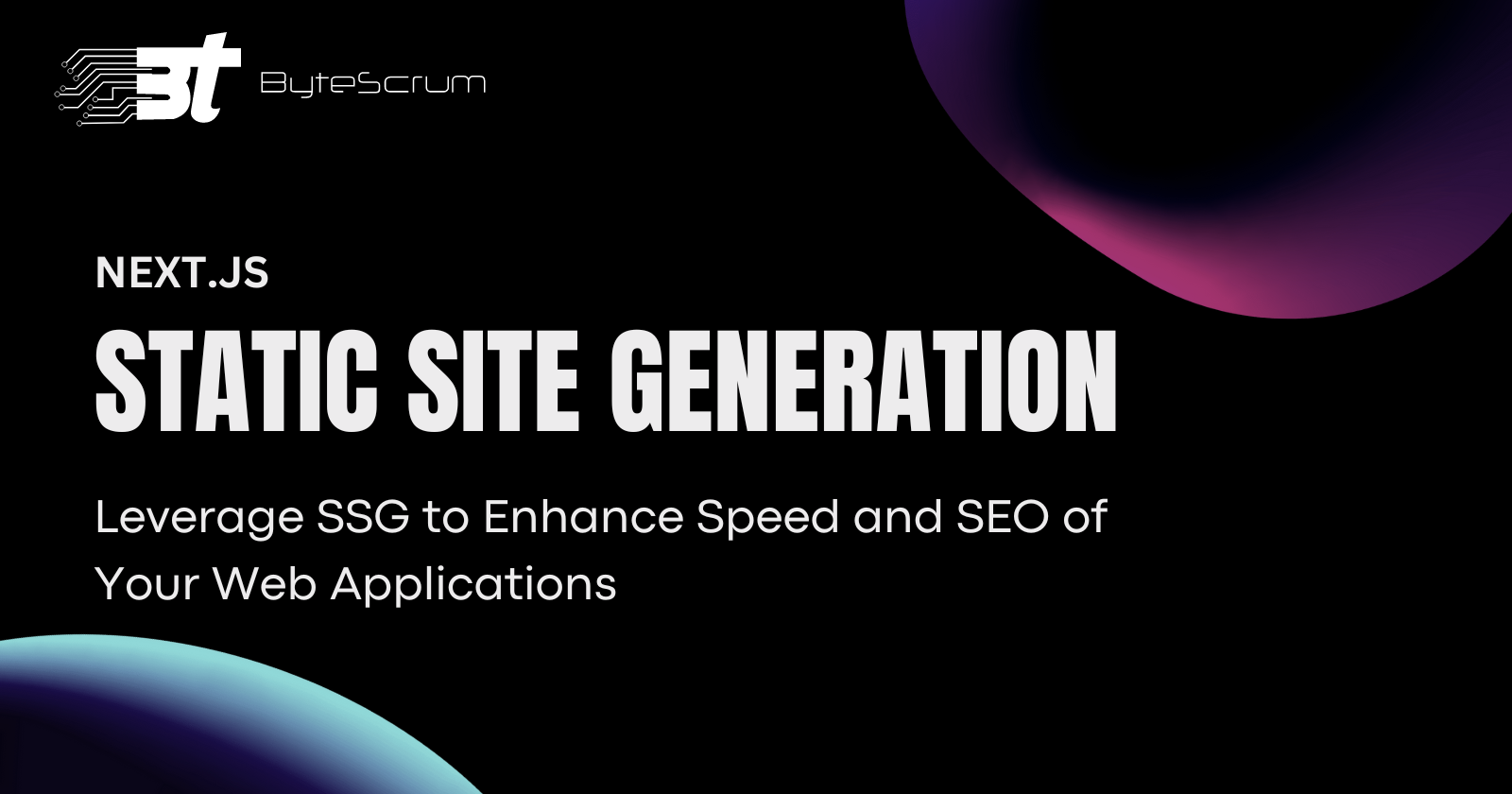
Static Site Generation (SSG) is a powerful feature of Next.js that allows you to pre-render pages at build time. This results in fast, SEO-friendly, and highly performant web applications. In this blog, we’ll delve into the concept of SSG, its benefits, and how to implement it in Next.js.
What is Static Site Generation (SSG)?
Static Site Generation refers to the process of generating HTML pages at build time rather than at runtime. These pre-rendered pages are served directly to the client, providing a fast and efficient user experience. Unlike Server-Side Rendering (SSR), which renders pages on each request, SSG generates the HTML once and serves the same content for every request.
Benefits of SSG
Improved Performance:
- Pre-rendered pages load faster since they don’t require server-side processing on each request.
Enhanced SEO:
- Search engines can easily crawl and index static pages, improving your site's visibility and ranking.
Better Scalability:
- Serving static pages reduces the load on your server, allowing it to handle more traffic efficiently.
Cost Efficiency:
- Hosting static sites is typically cheaper and requires less infrastructure compared to dynamic sites.
Implementing SSG in Next.js
Next.js makes it easy to implement SSG using the getStaticProps
and getStaticPaths
functions.
Example: Implementing SSG
Let’s build an example where we fetch and display a list of blog posts using SSG.
Set Up Your Project
Ensure you have a Next.js project set up. If not, create a new one:
npx create-next-app ssg-example cd ssg-example
Create a Page to List Blog Posts
Create a new file called
pages/posts.js
:touch pages/posts.js
Add the following content to
pages/posts.js
:// pages/posts.js import React from 'react'; const Posts = ({ posts }) => { return ( <div> <h1>Blog Posts</h1> <ul> {posts.map(post => ( <li key={post.id}> <h2>{post.title}</h2> <p>{post.body}</p> </li> ))} </ul> </div> ); }; export const getStaticProps = async () => { const res = await fetch('https://jsonplaceholder.typicode.com/posts'); const posts = await res.json(); return { props: { posts, }, }; }; export default Posts;
In this example:
The
getStaticProps
function fetches the blog posts data at build time.The
posts
data is passed as props to thePosts
component.The
Posts
component renders the list of blog posts.
Create a Dynamic Page for Each Blog Post
Create a new file called
pages/posts/[id].js
:mkdir -p pages/posts touch pages/posts/[id].js
Add the following content to
pages/posts/[id].js
:// pages/posts/[id].js import React from 'react'; const Post = ({ post }) => { return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); }; export const getStaticPaths = async () => { const res = await fetch('https://jsonplaceholder.typicode.com/posts'); const posts = await res.json(); const paths = posts.map(post => ({ params: { id: post.id.toString() }, })); return { paths, fallback: false, }; }; export const getStaticProps = async ({ params }) => { const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${params.id}`); const post = await res.json(); return { props: { post, }, }; }; export default Post;
In this example:
The
getStaticPaths
function fetches all blog posts and generates paths for each post based on its ID.The
getStaticProps
function fetches the data for a single post based on its ID at build time.The
Post
component renders the individual blog post.
Run the Development Server
Start the development server:
npm run dev
Navigate to
http://localhost:3000/posts
to see the list of blog posts. Click on any post to view its details.
SSG vs. SSR
While both SSG and SSR are useful for rendering pages, they serve different purposes:
SSG is ideal for pages with static content that doesn’t change frequently, such as blog posts, documentation, and marketing pages.
SSR is suitable for pages with dynamic content that needs to be fetched and rendered at request time, such as personalized dashboards and real-time data.
Advanced SSG Features in Next.js
Next.js offers several advanced features for SSG, including:
Incremental Static Regeneration (ISR):
- Update static content after the site has been built without needing a full rebuild.
Preview Mode:
- Preview draft content from your CMS without rebuilding the entire site.
API Routes:
- Combine static generation with server-side functionality using API routes.
Environment Variables:
- Use environment variables to manage sensitive data and configuration.
Conclusion
Explore the official Next.js documentation on SSG to learn more about advanced SSG techniques and best practices.
Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
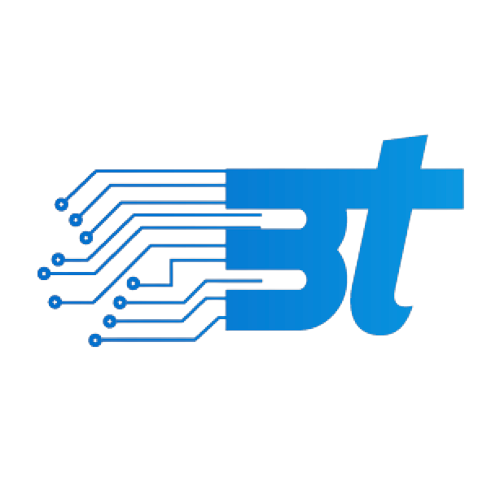
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.