Understanding the Factory Pattern in Android Development
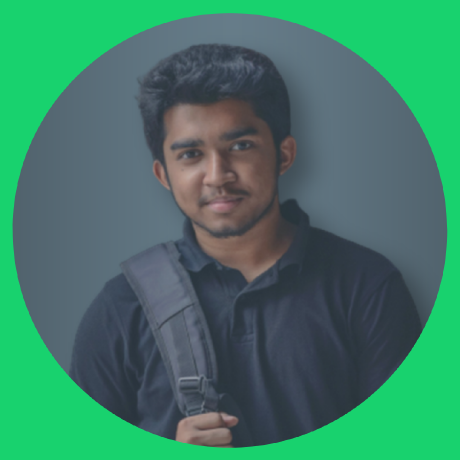
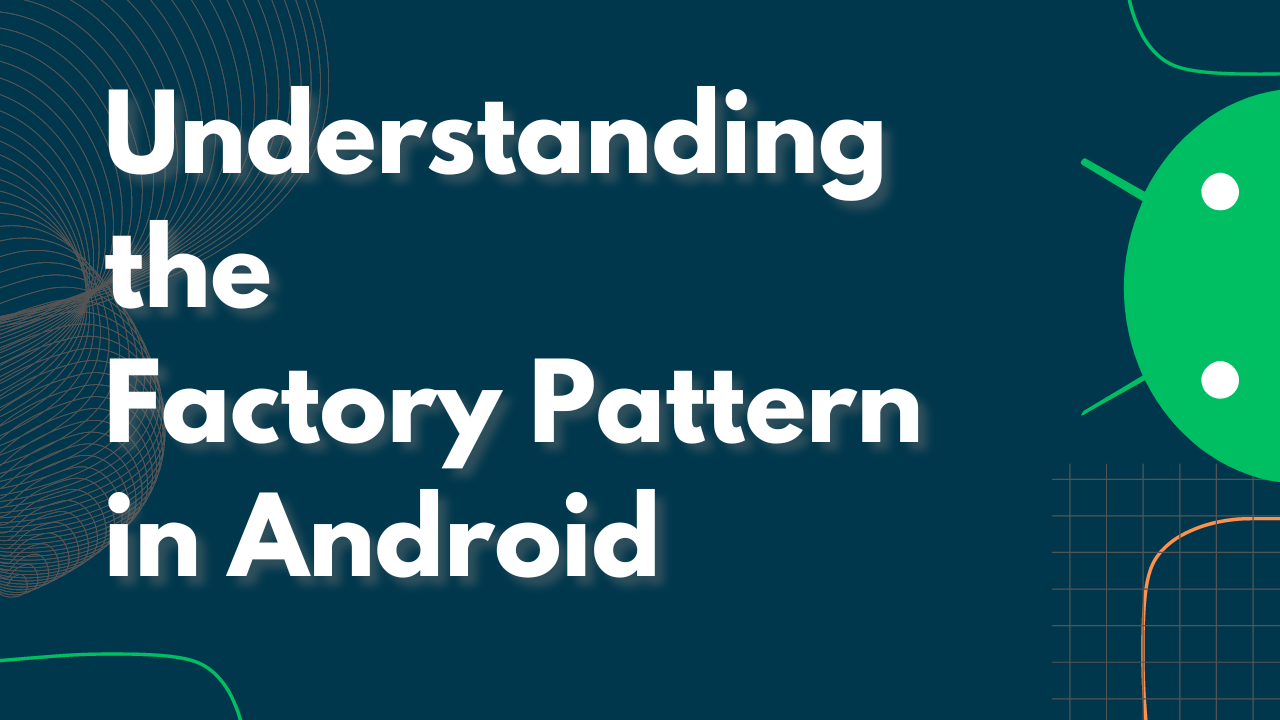
Introduction
The Factory Pattern is a widely used design pattern in software development. It provides an interface for creating objects but allows subclasses to alter the type of objects that will be created. This pattern is especially useful in Android development for creating instances of classes without having to specify the exact class of the object that will be created. In this blog, we'll explore the Factory Pattern, its benefits, and how to implement it in Android.
What is the Factory Pattern?
The Factory Pattern belongs to the creational patterns category. Its primary purpose is to define an interface for creating an object, but it allows subclasses to change the type of objects that are created. This pattern is advantageous when the exact type of object to be created cannot be determined until runtime.
Key Components:
Factory Interface: Declares a method for creating objects.
Concrete Factory: Implements the method to create a specific type of object.
Product Interface: Declares methods that all products must implement.
Concrete Product: Implements the product interface.
Why Use the Factory Pattern in Android?
In Android development, the Factory Pattern can help manage the complexity of object creation, enhance code readability, and promote code reusability. It's particularly useful in scenarios where you need to create objects that share a common interface but have different implementations.
Benefits:
Decoupling: The client code is decoupled from the specific classes it needs to instantiate.
Flexibility: New types of products can be introduced without changing existing client code.
Maintainability: Code is easier to maintain and extend.
Implementing the Factory Pattern in Android
Let's implement a simple example to demonstrate the Factory Pattern in an Android application. Imagine an application that needs to create different types of notifications (e.g., email, SMS, push notification).
Define the Product Interface
// Notification.kt interface Notification { fun notifyUser() }
Create Concrete Products
// EmailNotification.kt class EmailNotification : Notification { override fun notifyUser() { // Send an email notification } } // SMSNotification.kt class SMSNotification : Notification { override fun notifyUser() { // Send an SMS notification } } // PushNotification.kt class PushNotification : Notification { override fun notifyUser() { // Send a push notification } }
Define the Factory Interface
// NotificationFactory.kt interface NotificationFactory { fun createNotification(type: String): Notification? }
Implement the Concrete Factory
// ConcreteNotificationFactory.kt class ConcreteNotificationFactory : NotificationFactory { override fun createNotification(type: String): Notification? { return when (type) { "EMAIL" -> EmailNotification() "SMS" -> SMSNotification() "PUSH" -> PushNotification() else -> throw IllegalArgumentException("Unknown notification type") } } }
Use the Factory in Client Code
// MainActivity.kt class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val factory = ConcreteNotificationFactory() val emailNotification = factory.createNotification("EMAIL") emailNotification?.notifyUser() val smsNotification = factory.createNotification("SMS") smsNotification?.notifyUser() val pushNotification = factory.createNotification("PUSH") pushNotification?.notifyUser() } }
Conclusion
The Factory Pattern is a powerful tool in Android development that promotes decoupling, flexibility, and maintainability. By abstracting the instantiation process, it allows developers to create more modular and adaptable code. Implementing this pattern can help manage complexity and improve the overall architecture of your application. Try incorporating the Factory Pattern in your next Android project to see the benefits firsthand!
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
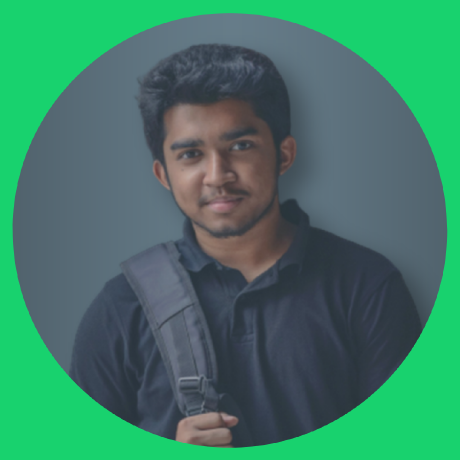
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator