The Mediator Design Pattern: Facilitating Object Communication
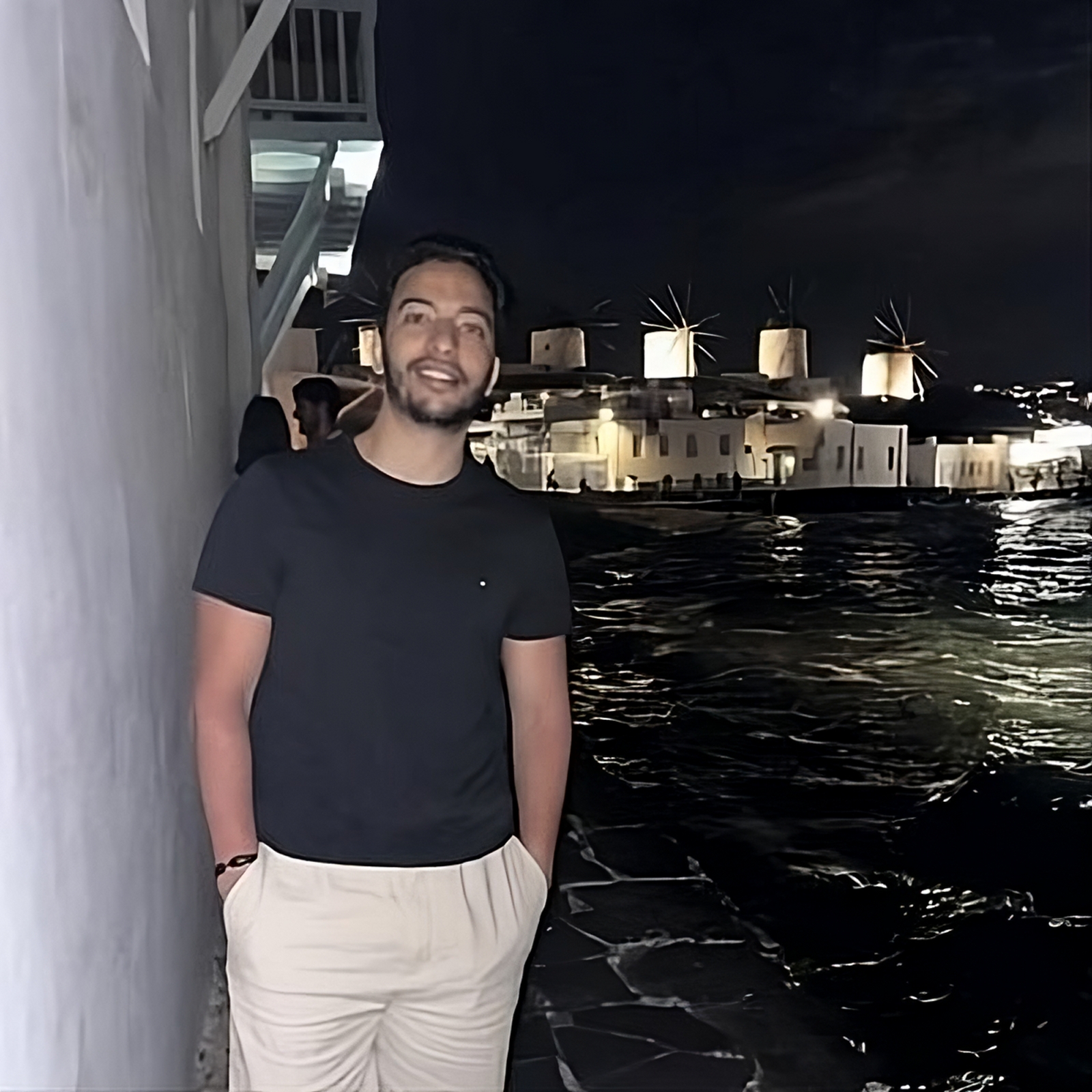
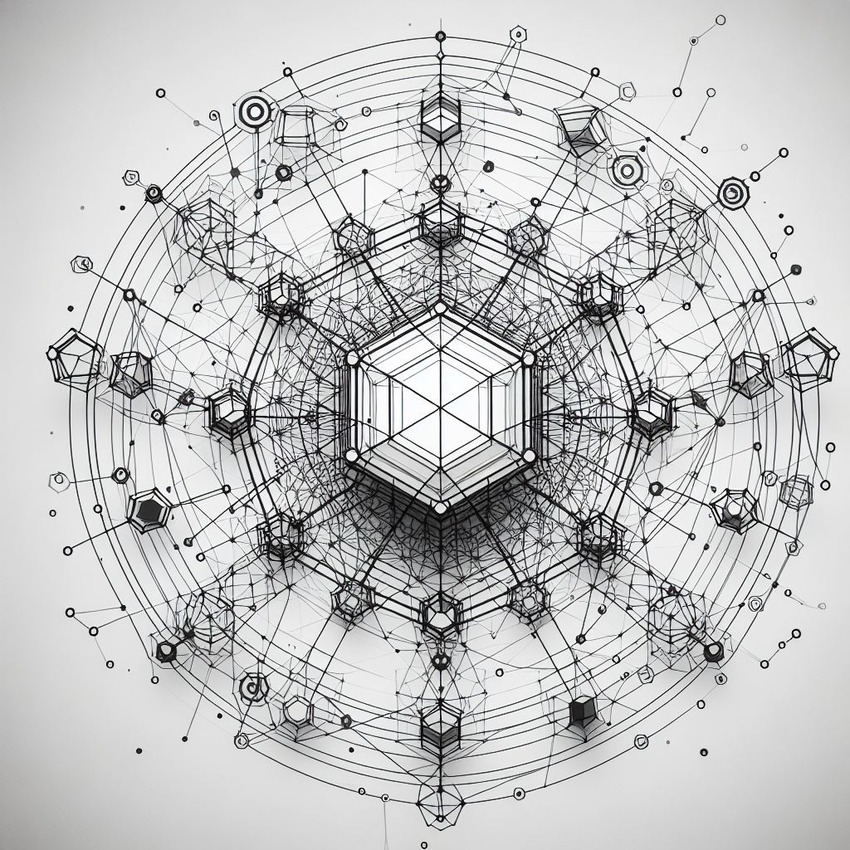
In software design, objects often need to communicate and collaborate with each other to achieve complex functionality. However, direct communication between objects can lead to tight coupling, making the system difficult to maintain and extend. This is where the Mediator design pattern comes into play. The Mediator pattern promotes loose coupling by introducing a mediator object that handles the communication between other objects, known as colleagues.
What is the Mediator Design Pattern?
The Mediator design pattern is a behavioral pattern that defines an object (the mediator) that encapsulates how a set of objects (colleagues) interact with each other. Instead of direct communication between colleagues, all communication is channeled through the mediator, which acts as a central hub for coordinating the interactions.
The pattern involves three main components:
Mediator: The central object that handles communication between colleagues.
Colleague: The objects that need to communicate with each other.
ConcreteMediator: The concrete implementation of the Mediator interface, which defines the communication logic between colleagues.
Here's how the Mediator pattern works:
Each colleague knows about the mediator object and communicates with it instead of directly communicating with other colleagues.
When a colleague needs to interact with another colleague, it sends a request to the mediator.
The mediator receives the request and decides how to handle it, potentially involving other colleagues as needed.
The mediator then coordinates the appropriate actions or responses among the relevant colleagues.
By centralizing the communication logic in the mediator, this pattern promotes loose coupling between colleagues, making the system more maintainable, extensible, and testable.
Real-World Use Cases
The Mediator design pattern is commonly used in various scenarios where objects need to communicate with each other in a decoupled and organized manner. Here are some real-world examples:
User Interface (UI) Components: In GUI applications, mediators can coordinate the communication between different UI components, such as buttons, text fields, and menus.
Chat Applications: Chat applications often use a mediator to handle the communication between multiple participants and manage the message routing and delivery.
Middleware Systems: Mediators are commonly used in middleware systems to facilitate the communication between different components or services in a distributed environment.
Workflow Management Systems: In workflow management systems, a mediator can coordinate the execution of tasks and manage the flow of data between different processes or activities.
Example
Here's a TypeScript example that demonstrates the implementation of the Mediator pattern in a simplified chat application:
// Mediator interface
interface Mediator {
sendMessage(message: string, sender: Colleague): void;
}
// Colleague interface
interface Colleague {
name: string;
mediator: Mediator;
receiveMessage(message: string, sender: string): void;
}
// Concrete Mediator
class ChatRoomMediator implements Mediator {
private colleagues: Colleague[] = [];
addColleague(colleague: Colleague): void {
this.colleagues.push(colleague);
colleague.mediator = this;
}
sendMessage(message: string, sender: Colleague): void {
for (const colleague of this.colleagues) {
if (colleague !== sender) {
colleague.receiveMessage(message, sender.name);
}
}
}
}
// Concrete Colleague
class User implements Colleague {
constructor(public name: string, private mediator: Mediator) {
this.mediator = mediator;
}
sendMessage(message: string): void {
this.mediator.sendMessage(message, this);
}
receiveMessage(message: string, sender: string): void {
console.log(`${sender} to ${this.name}: ${message}`);
}
}
// Usage
const mediator = new ChatRoomMediator();
const ayoub = new User('Ayoub', mediator);
const jeremy = new User('Jérémy', mediator);
const bob = new User('Bob', mediator);
mediator.addColleague(ayoub);
mediator.addColleague(jeremy);
mediator.addColleague(bob);
ayoub.sendMessage('Hello, everyone!');
jeremy.sendMessage('Hi, Ayoub! How are you?');
In this example, we have a Mediator
interface that defines the sendMessage
method for sending messages to colleagues. The Colleague
interface defines the receiveMessage
method for receiving messages from other colleagues.
The ChatRoomMediator
class is a concrete implementation of the Mediator
interface, responsible for managing the list of colleagues and forwarding messages to the appropriate recipients.
The User
class is a concrete implementation of the Colleague
interface, representing a user in the chat application. Users can send messages through the mediator and receive messages from other users.
In the usage example, we create a ChatRoomMediator
instance, three User
instances (John, Jane, and Bob), and add them as colleagues to the mediator. John sends a message, which is broadcasted to Jane and Bob through the mediator. Jane then sends a message back to John, also via the mediator.
Benefits of the Mediator Pattern
Using the Mediator design pattern offers several benefits:
Loose Coupling: By introducing a mediator, the colleagues become loosely coupled, as they no longer need to know about each other's implementation details or communicate directly.
Centralized Communication Logic: The communication logic is centralized in the mediator, making it easier to maintain and modify the communication logic without affecting the colleague objects.
Extensibility: New colleagues can be added or removed without affecting the existing colleagues, as long as they follow the established communication protocol with the mediator.
Reusability: The mediator and colleague objects can be reused in different contexts or applications, as they are decoupled from each other.
Simplified Communication: Complex communication workflows between multiple colleagues can be simplified by using a mediator to coordinate the interactions.
However, it's important to remember that introducing a mediator can also add complexity to the system, especially if the mediator becomes too complex or takes on too many responsibilities. As with any design pattern, it's crucial to evaluate the trade-offs and apply the Mediator pattern judiciously based on the specific requirements of your project.
The Mediator design pattern is a powerful tool for facilitating communication between objects while promoting loose coupling and maintainability. By centralizing the communication logic in a mediator object, this pattern helps to create more modular and extensible software systems.
Subscribe to my newsletter
Read articles from Ayoub Touba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
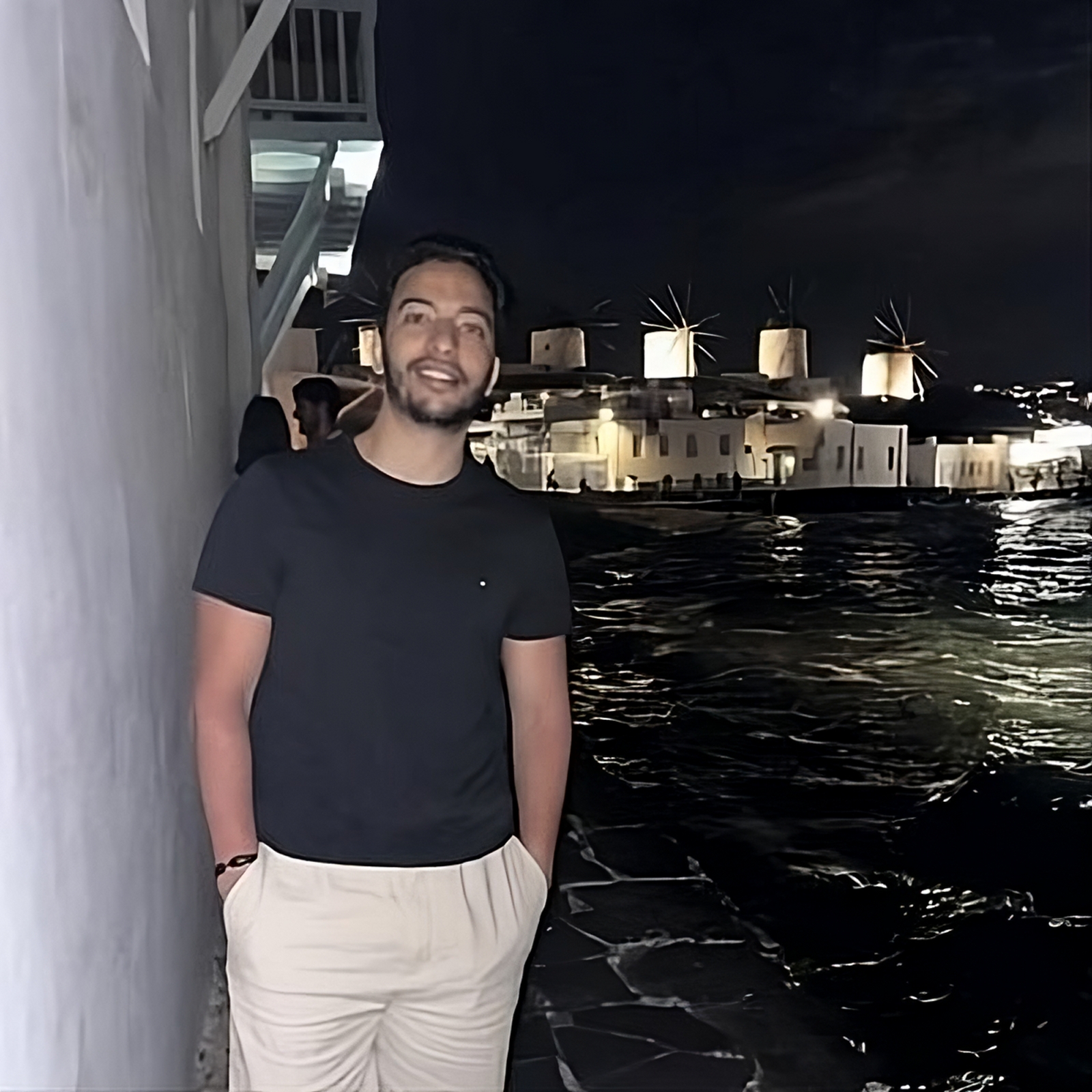
Ayoub Touba
Ayoub Touba
With over a decade of hands-on experience, I specialize in building robust web applications and scalable software solutions. My expertise spans across cutting-edge frameworks and technologies, including Node.js, React, Angular, Vue.js, and Laravel. I also delve into hardware integration with ESP32 and Arduino, creating IoT solutions.