The Journey of a C File: From Source Code to Execution
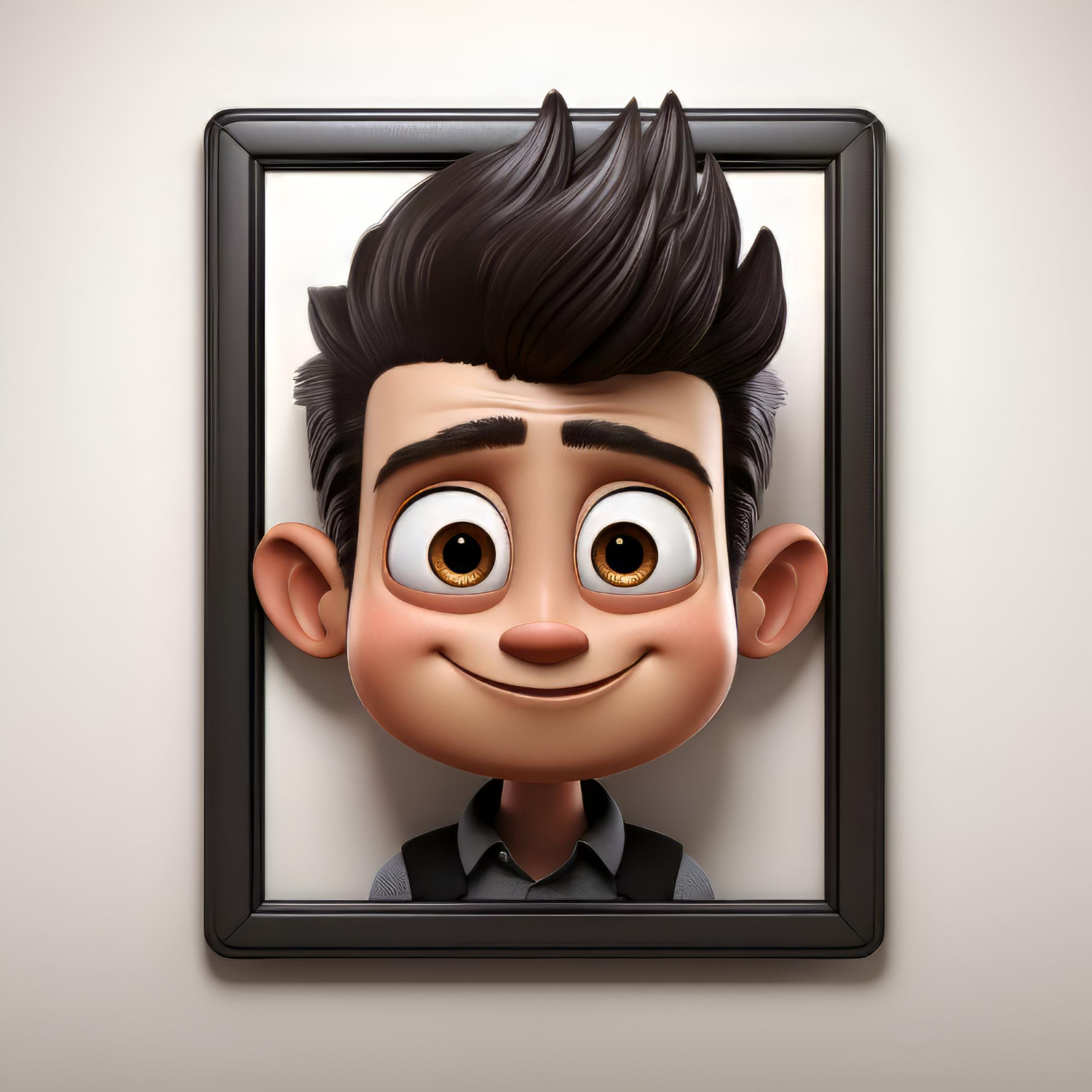
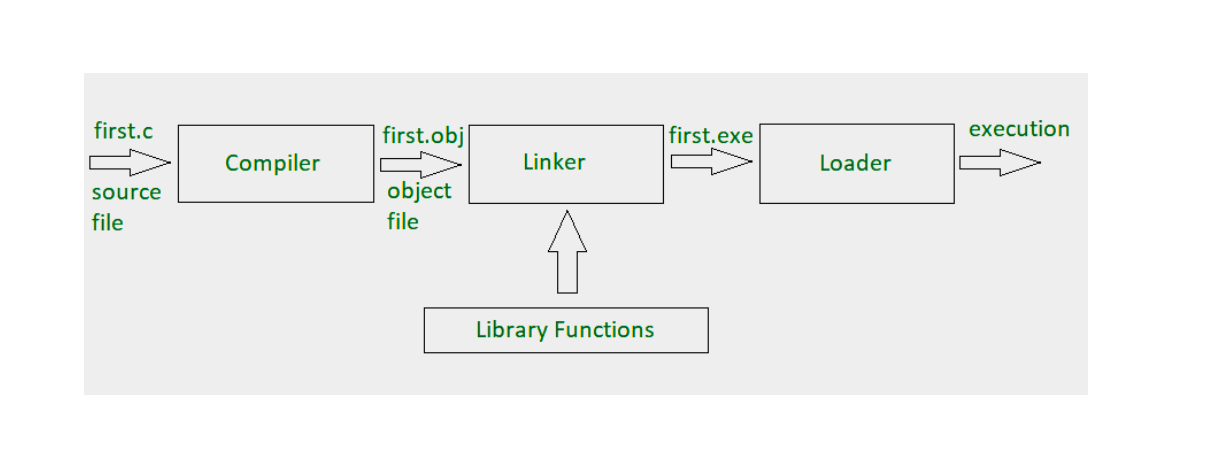
Understanding how a C program goes from a source file to running as an executable on your computer involves several steps. Let's walk through the entire process.
Step 1: Writing the Source Code
Creating the Source File:
You write the source code for your C program using a text editor and save it with a
.c
extension, for example,program.c
.This file is stored in RAM while you are editing it, and once you save it, it is written to the HDD.
Step 2: Compilation
Preprocessing:
- The preprocessor reads the source file and processes preprocessor directives (e.g.,
#include
,#define
).
- The preprocessor reads the source file and processes preprocessor directives (e.g.,
Compilation:
The compiler takes the preprocessed file and translates it into assembly language, and further converts the assembly code into machine code, producing an object file with a
.o
or.obj
extension.During this phase, the compiler also performs syntax checking to ensure the code adheres to the C language rules.
Step 3: Linking
Linking:
The linker takes the object file and links it with other necessary object files and libraries (e.g., standard C library).
It resolves references to functions and variables, ensuring that all code and data addresses are correctly assigned.
The output of the linker is an executable file (e.g.,
program.exe
on Windows orprogram
on Unix-based systems), which contains machine code in the form of 0s and 1s.
Step 4: Loading and Execution
Loading:
The loader, part of the operating system, loads the executable file into RAM.
It sets up the execution environment, including allocating memory for the program’s code, data, and stack segments.
The loader also resolves any final address bindings and prepares the program to run.
Execution:
The CPU begins executing the machine code instructions from the executable file now loaded in RAM.
During execution, the program's instructions and data reside in RAM, allowing the CPU to access them quickly.
Detailed Breakdown
Compilation Details
Preprocessing:
The preprocessor handles directives like
#include <stdio.h>
by including the contents of the specified header file into the source code.Macros defined with
#define
are expanded.
Compilation:
The compiler translates high-level C code into assembly instructions specific to the target architecture.
This assembly code is then assembled into machine code (binary form) by the assembler, producing an object file.
Linking Details
Static Linking:
The linker combines all object files and static libraries into a single executable.
It resolves external references (e.g., function calls to standard library functions) by including the necessary machine code from the libraries.
Dynamic Linking:
Instead of copying the library code into the executable, dynamic linking defers the linking of some libraries until the program is run.
This means the executable will contain references to shared libraries (e.g., DLLs on Windows or
.so
files on Unix).
Loading and Execution
Loader:
When you execute a program, the OS loader reads the executable file’s header to determine how much memory is needed and where the program segments should be loaded.
The loader copies the program code and data into RAM and initializes the program counter to the entry point of the executable.
Execution:
The CPU fetches instructions from RAM, decodes, and executes them.
It performs operations such as arithmetic calculations, memory access, and control flow changes.
Advantages and Disadvantages of HDD and RAM
Hard Disk Drive (HDD):
Advantages:
Large storage capacity at a low cost.
Non-volatile storage (retains data without power).
Disadvantages:
Slower access speeds compared to RAM and SSDs.
Susceptible to mechanical failure due to moving parts.
Random Access Memory (RAM):
Advantages:
High-speed data access and transfer rates.
Crucial for running programs and processes efficiently.
Disadvantages:
Volatile memory (data is lost when power is off).
More expensive per gigabyte compared to HDDs.
A Brief Note on Solid State Drives (SSD)
Solid State Drive (SSD):
Advantages:
Much faster access speeds compared to HDDs, improving overall system performance.
More durable with no moving parts, leading to higher reliability.
Lower power consumption.
Disadvantages:
More expensive per gigabyte than HDDs.
Limited write cycles, although this has improved significantly with modern technology.
Conclusion
The process of transforming a C source file into a running program involves multiple stages: writing the source code, preprocessing, compiling, linking, and loading. Each stage has a specific role in converting human-readable code into machine-executable instructions. Understanding this process helps in debugging, optimizing, and effectively developing software. The interplay between different types of memory—HDD for storage, RAM for quick access, and registers/cache for immediate processing—highlights the complexity and efficiency of modern computing systems.
Subscribe to my newsletter
Read articles from Alok Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
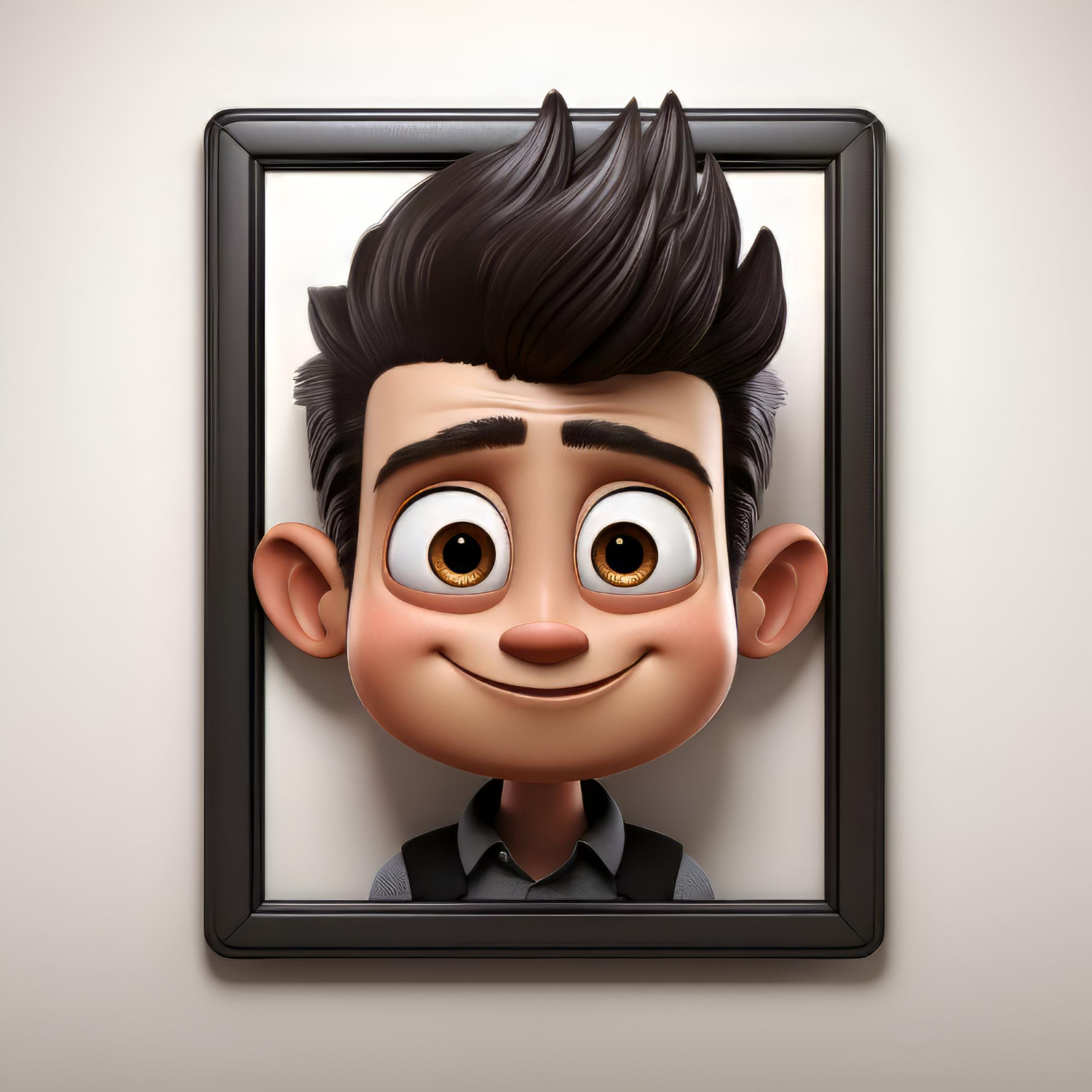
Alok Gupta
Alok Gupta
I’m an aspiring web developer keen on learning new things. I’m dedicated to keeping up to date with the latest stuffs in web development. I always lookout for chances to grow and learn. When I’m not coding, I enjoy reading about India’s fascinating history. It helps satisfy my curiosity and teaches me about the diverse India.