DSA Basics: A Beginnerβs Guide to Arrays and Linked Lists
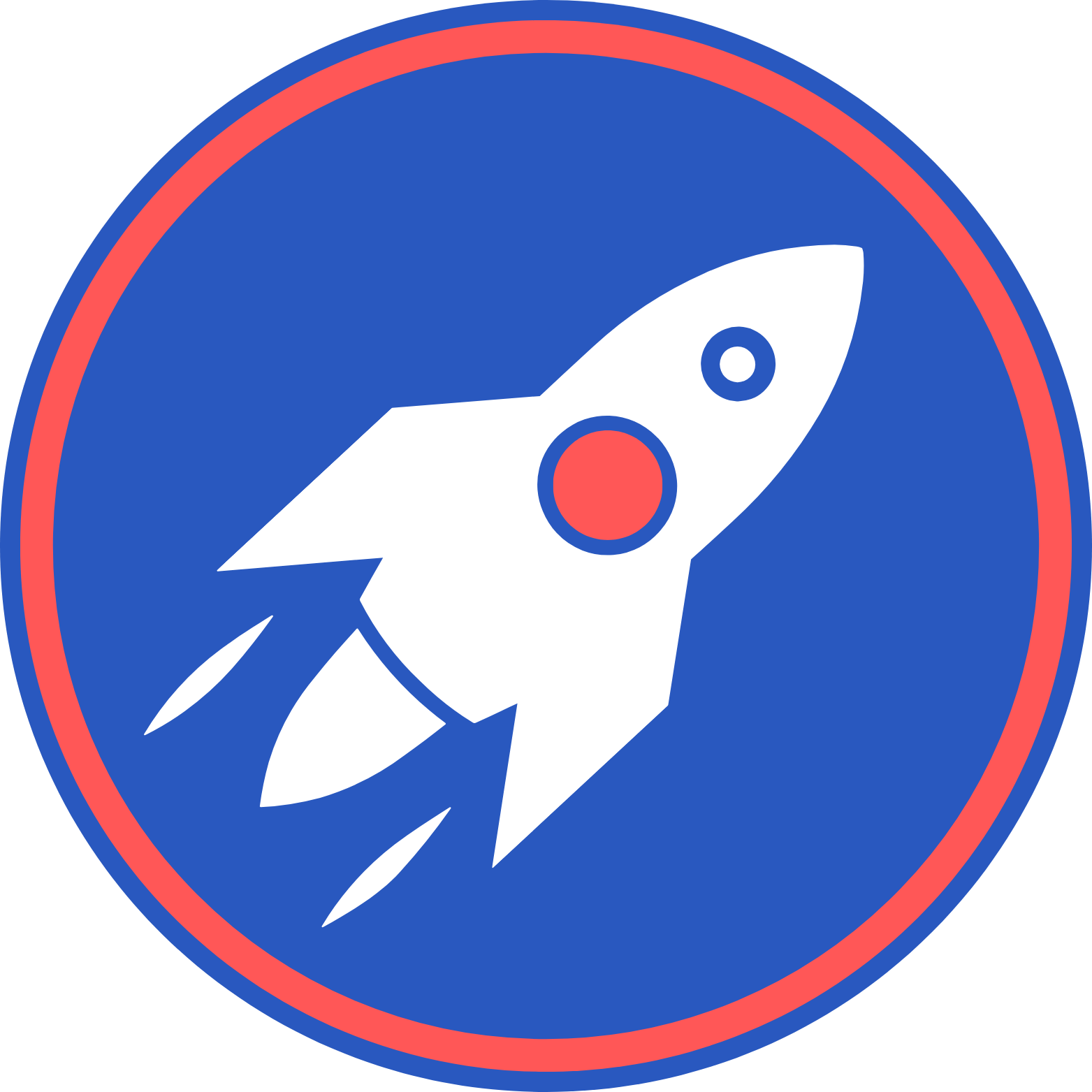
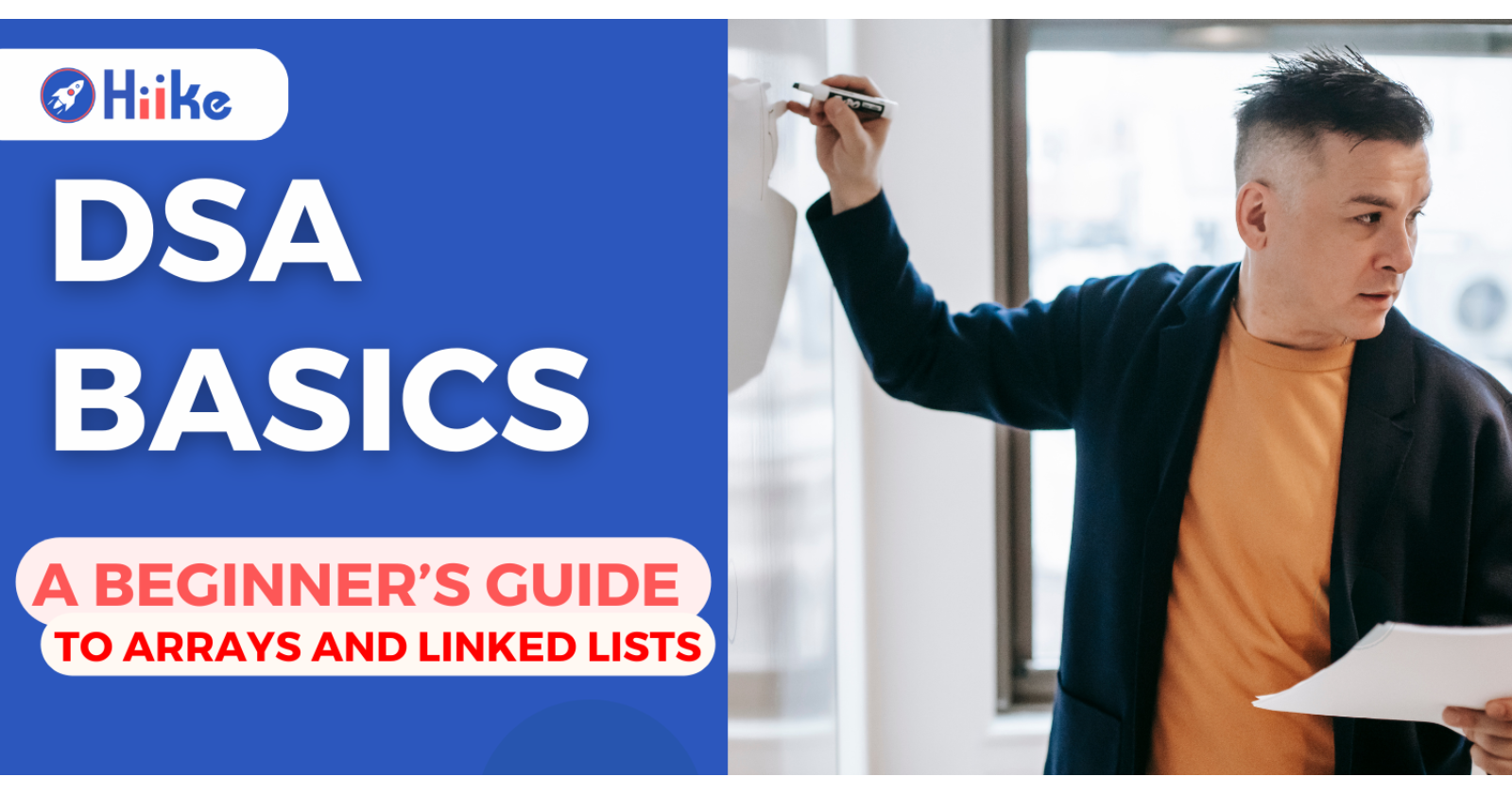
Data Structures and Algorithms (DSA) form the backbone of computer science, enabling developers to solve complex problems efficiently. Among the foundational data structures are arrays and linked lists. Understanding these basics is crucial for anyone aspiring to excel in software development. In this guide, we'll delve into the core concepts, operations, and applications of arrays and linked lists, providing you with a solid foundation to build upon.
Introduction to Arrays and Linked Lists
Arrays
An array is a collection of elements, typically of the same data type, stored in contiguous memory locations. Arrays allow for efficient indexing and iteration, making them ideal for situations where you need to quickly access elements by their position.
Characteristics of Arrays:
Fixed Size: The size of an array is determined at the time of its creation and cannot be changed.
Efficient Access: Elements can be accessed in constant time using an index.
Homogeneous Elements: All elements in an array are of the same type.
Linked Lists
A linked list is a linear data structure where each element (node) contains a reference (link) to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation, making them more flexible for dynamic data scenarios.
Characteristics of Linked Lists:
Dynamic Size: Linked lists can grow and shrink in size dynamically.
Sequential Access: Elements are accessed sequentially, which can be less efficient than direct indexing.
Heterogeneous Elements: Nodes can store different types of data, though typically, a single linked list stores homogeneous data types.
Basic Operations and Implementations
Arrays
- Declaration and Initialization:
int[] array = new int[10]; // Java
int array[10]; // C++
- Accessing Elements:
int element = array[2]; // Accessing the third element
- Updating Elements:
array[2] = 5; // Updating the third element to 5
- Iterating through an Array:
for (int i = 0; i < array.length; i++) {
System.out.println(array[i]);
}
Linked Lists
- Node Structure:
class Node {
int data;
Node next;
Node(int data) {
this.data = data;
this.next = null;
}
}
- Creating a Linked List:
Node head = new Node(1);
head.next = new Node(2);
head.next.next = new Node(3);
- Inserting a Node at the Beginning:
Node newNode = new Node(0);
newNode.next = head;
head = newNode;
- Iterating through a Linked List:
Node current = head;
while (current != null) {
System.out.println(current.data);
current = current.next;
}
Comparison Between Arrays and Linked Lists
Feature | Arrays | Linked Lists |
Size | Fixed | Dynamic |
Memory Allocation | Contiguous | Non-contiguous |
Access Time | O(1) | O(n) |
Insertion/Deletion | Costly (shifting elements) | Efficient (constant time, O(1)) |
Memory Usage | Less memory overhead | More memory overhead (pointers) |
Practical Examples and Exercises
Example 1: Implementing an Array in Python
# Declaration
array = [1, 2, 3, 4, 5]
# Accessing elements
print(array[2]) # Output: 3
# Updating elements
array[2] = 10
# Iterating through the array
for element in array:
print(element)
Example 2: Implementing a Linked List in Python
class Node:
def init(self, data):
self.data = data
self.next = None
class LinkedList:
def init(self):
self.head = None
def insert_at_beginning(self, data):
new_node = Node(data)
new_node.next = self.head
self.head = new_node
def print_list(self):
current = self.head
while current:
print(current.data)
current = current.next
# Creating a linked list and adding elements
ll = LinkedList()
ll.insert_at_beginning(3)
ll.insert_at_beginning(2)
ll.insert_at_beginning(1)
# Printing the linked list
ll.print_list() # Output: 1 2 3
Common Use Cases and Applications
Arrays
Storing multiple elements of the same type: Useful for storing a fixed number of elements, such as scores in a game or the RGB values of an image.
Implementing other data structures: Arrays can be used to implement stacks, queues, and hash tables.
Linked Lists
Dynamic memory allocation: Ideal for applications where the number of elements is unknown or varies, such as a browser's history or a playlist.
Insertion and deletion operations: Efficient for applications requiring frequent insertions and deletions, such as managing a task scheduler.
Conclusion
Understanding arrays and linked lists is fundamental to mastering DSA. These data structures form the basis for more complex structures and algorithms. Whether you're preparing for technical interviews or aiming to enhance your coding skills, grasping these basics is crucial.
At Hiike, we specialize in helping developers like you achieve mastery in Data Structures, Algorithms, and System Design. Our Top 30 Program offers advanced training, practical application, and real-world scenarios, ensuring you're thoroughly prepared to excel in your career. Join us at Hiike and take the next step towards becoming a top-tier software engineer.
For more information, visit our website and explore our comprehensive programs designed to elevate your skills and open doors to opportunities at leading tech firms.
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
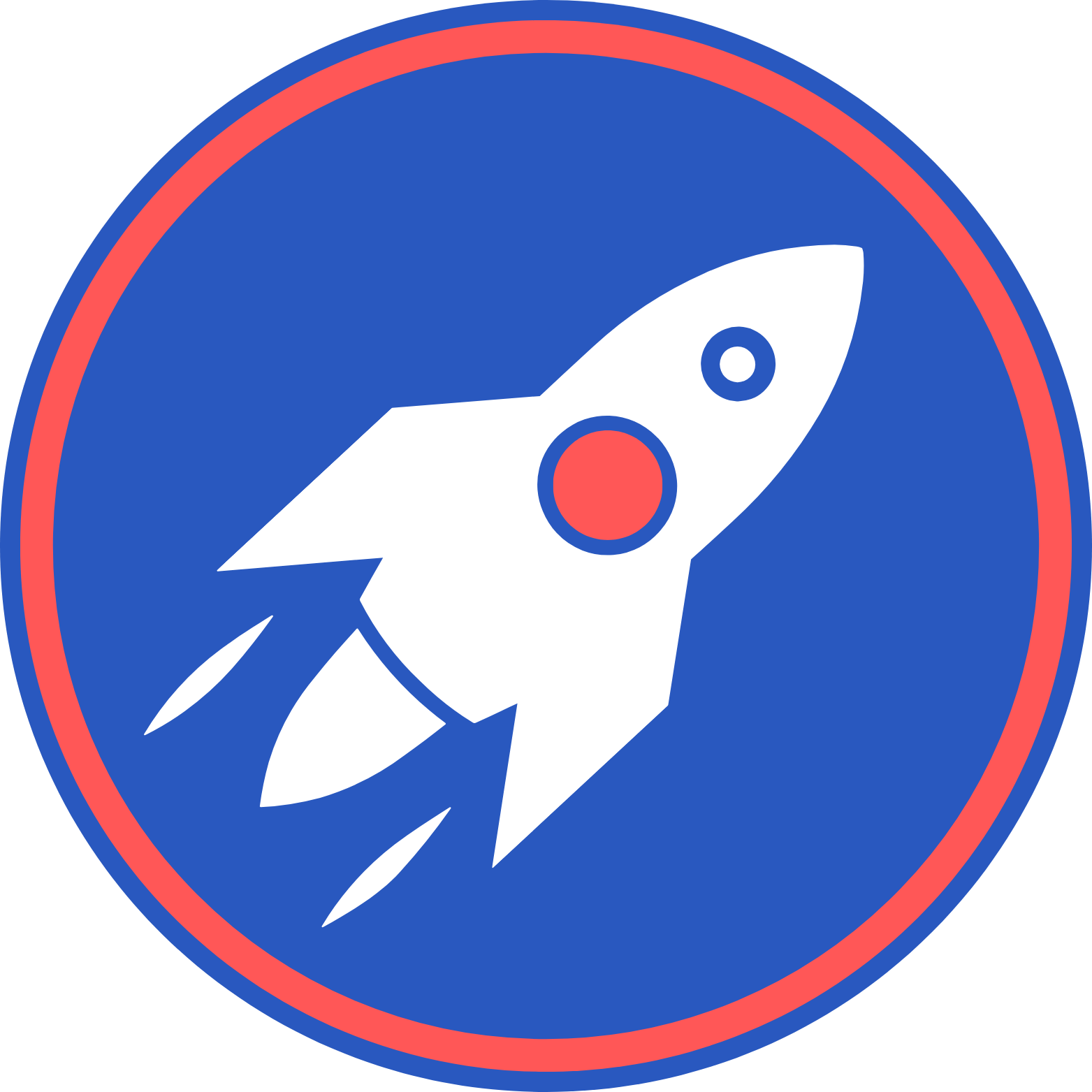
Hiike
Hiike
πππ’π’π€π - Crafting Your Tech Odyssey! π Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!