How to use Hugging Face Models with Langchain??
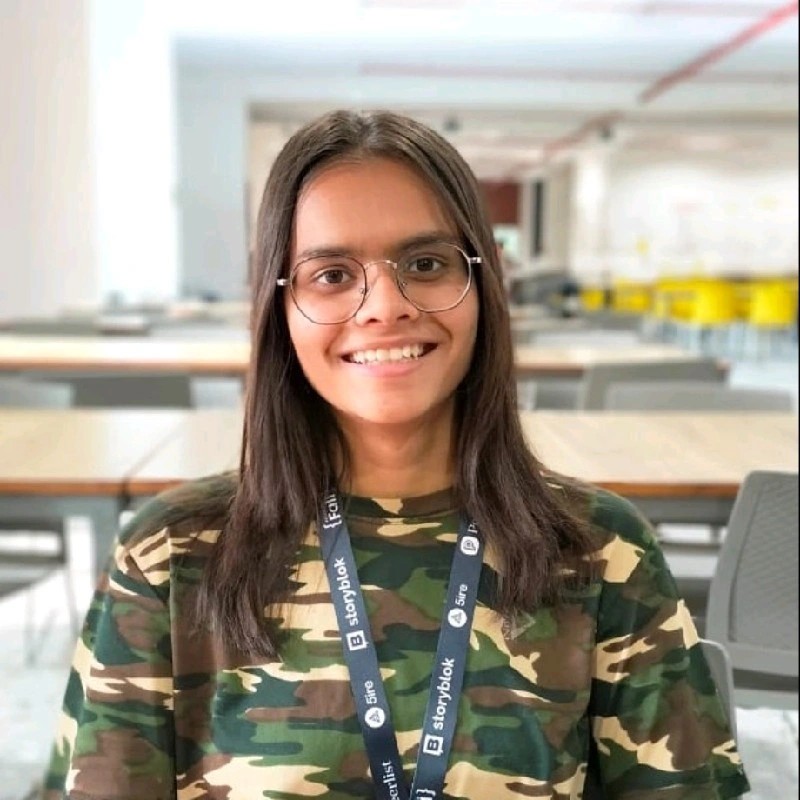
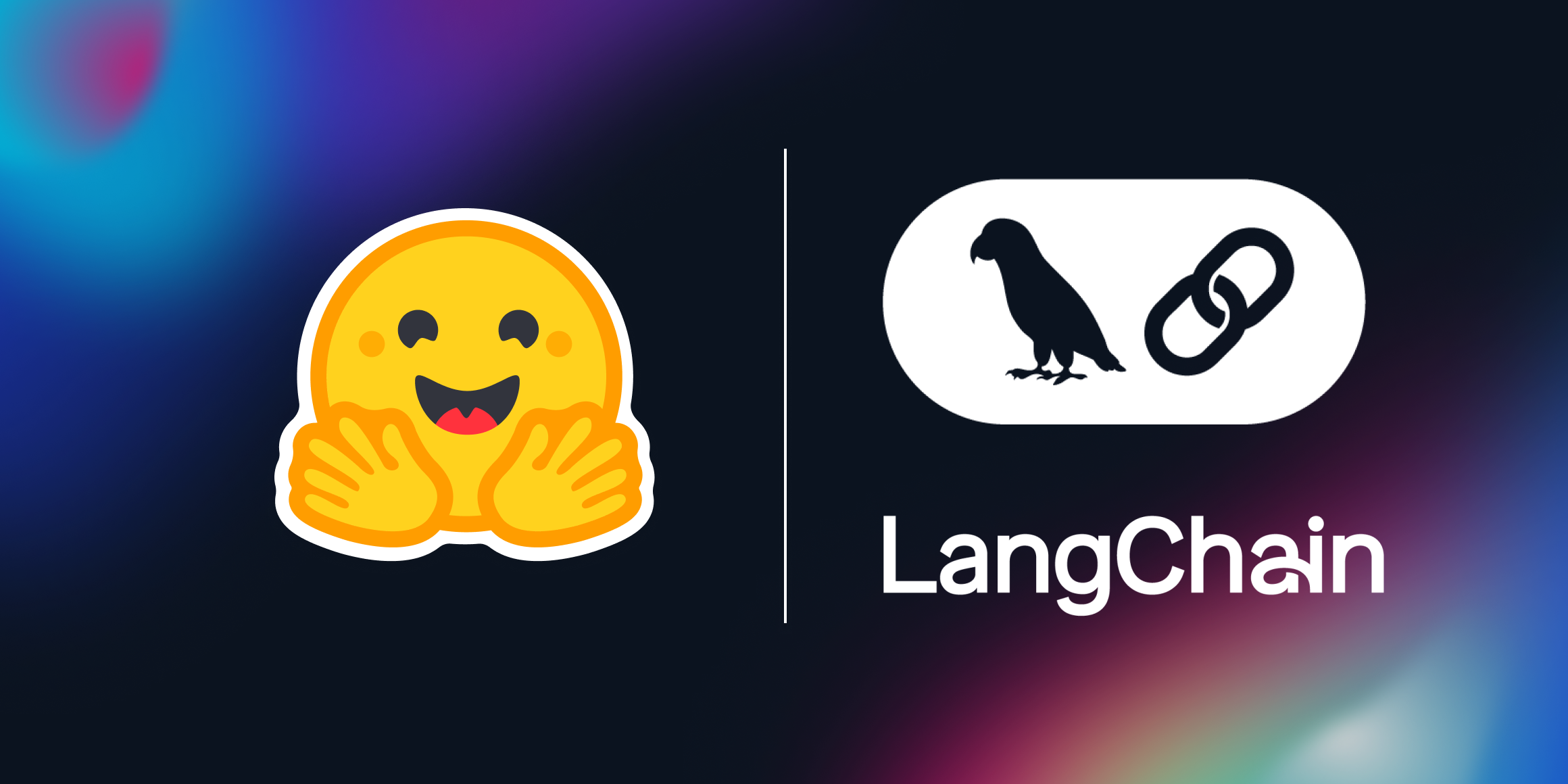
Introduction
Artificial Intelligence is transforming software development. With platforms like Hugging Face, developers now have easy access to powerful pre-trained models. In this blog post, we'll see how to use the Hugging Face Access Token with LangChain to leverage hugging face models. We'll be using the mistralai/Mixtral-8x7B-Instruct-v0.1
for this example.
What is Hugging Face and LangChain?
Hugging Face: Hugging Face provides access to state-of-the-art Natural Language Processing (NLP) models. You can easily incorporate these models into various applications for text classification, translation, summarization, and more.
LangChain: LangChain is a framework that seamlessly integrates with models and services, making it easy to build AI-powered applications.
Step 1: Getting the Hugging Face Access Token
Sign Up: Visit the Hugging Face website and sign up for an account if you haven't already.
Generate Access Token:
Go to Settings.
Click on "New token".
Name your token, select permissions, and create it.
Copy the generated token as you will need it later.
Step 2: Installing Required Libraries
Open your terminal and install the necessary libraries:
pip install langchain langchain_community huggingface_hub
Step 3: Setting Up Your Environment
Here's a step-by-step guide to setting up LangChain with the Hugging Face Endpoint:
Import Libraries:
import os from langchain.llms import HuggingFaceEndpoint
Note: Please replace
"your_hugging_face_token"
with your actual Hugging Face token. For security and best practices, use environment variables to store token.
Step 4: Using Hugging Face Access Tokens with LangChain
Example Code :
import os
from langchain.llms import HuggingFaceEndpoint
def generate_text(prompt, hf_token):
os.environ["HUGGINGFACEHUB_API_TOKEN"] = hf_token
repo_id = "mistralai/Mixtral-8x7B-Instruct-v0.1"
llm = HuggingFaceEndpoint(repo_id=repo_id, api_key=hf_token)
response = llm.generate([prompt])
text = response.generations[0][0].text
return text
if __name__ == "__main__":
prompt = "What is Langchain?"
hf_token = "your_hugging_face_token"
text = generate_text(prompt, hf_token)
print("Generated Text:")
print(text)
Steps in the Code:
Initializing Hugging Face Endpoint:
The Model ID
mistralai/Mixtral-8x7B-Instruct-v0.1
is specified.The
HuggingFaceEndpoint
fromlangchain
is initialized with the providedrepo_id
andapi_key
.
Generating Text:
The
generate_text
function takes a prompt and the Hugging Face token as inputs.It sets the environment variable, initializes the model, and generates text based on the given prompt.
The main section of the code sets up a prompt and calls the
generate_text
function.
Conclusion
By following the steps outlined, you can integrate advanced NLP capabilities into your applications seamlessly. The Mixtral-8x7B-Instruct-v0.1
model is just one example, and the approach can be adapted to various other models available on Hugging Face.
You can reach out to me on LinkedIn, X, and GitHub or via tishaghevariya@gmail.com.
Happy Coding ! ๐
Subscribe to my newsletter
Read articles from Teesha Ghevariya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
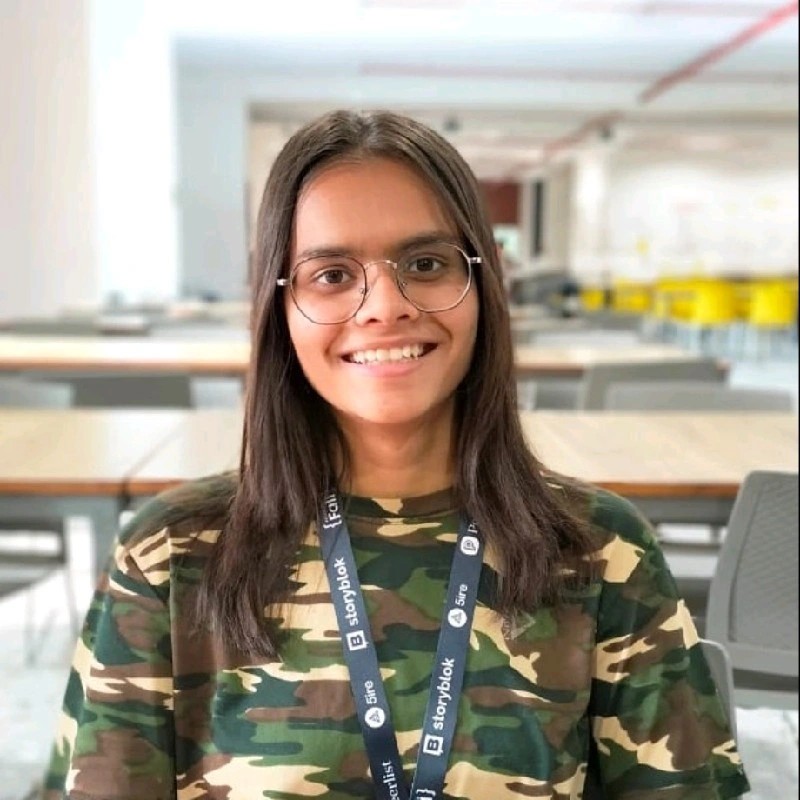
Teesha Ghevariya
Teesha Ghevariya
AI Engineer at DhiWise