What Are RESTful APIs and How Do They Work?
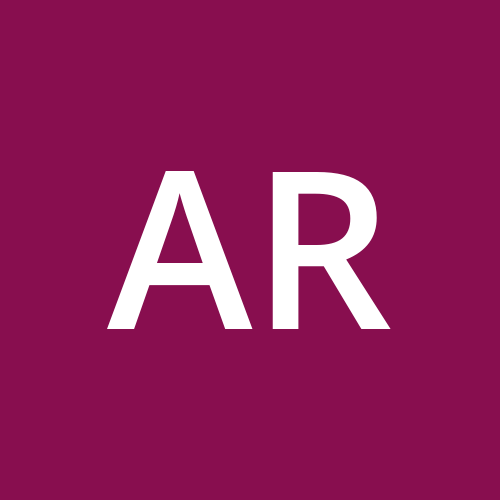
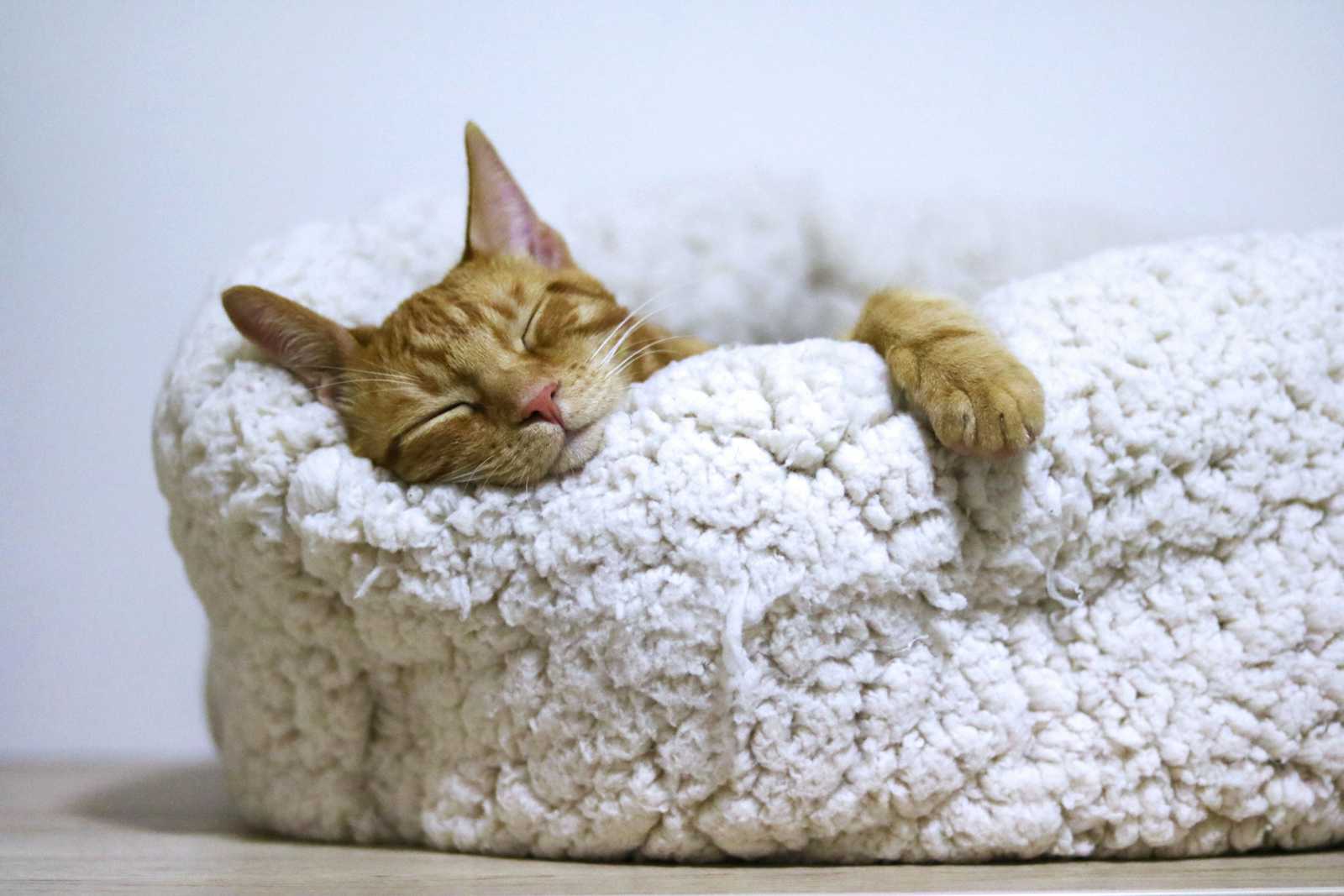
In today's interconnected world, APIs (Application Programming Interfaces) play a crucial role in enabling different software systems to communicate with each other.
Imagine you have a friend who speaks a different language. To talk to each other, you both need a translator who knows both languages. An API (Application Programming Interface) is like that translator for computers. It helps different software programs talk to each other and share information.
API stands for Application Programming Interface. Think of it as a set of rules, like a instruction, that tells one software program how to ask another program for information and how to get an answer back. APIs help computers work together even if they are very different.
One special type of API is called a RESTful API. It’s very popular because it’s simple and can handle lots of users at the same time (scalable). In this guide, we will dive into the world of RESTful APIs, understanding their principles, how to design and implement them, and exploring some common tools for testing.
What is a RESTful API?
A REST API (Representational State Transfer) is a type of API that follows specific rules to handle requests and responses between a client (like your web browser) and a server (where the data is stored). These rules make it easier for different programs to talk to each other and ensure the communication is efficient and reliable, allowing different programs to interact without remembering past conversations (stateless).
The main goal of REST is to create APIs that can easily handle many users at once (scalable) and work fast (performant) by using the existing internet structure.
Key Principles of REST
Statelessness: Every time you ask the server for something, you have to give it all the details it needs right in that request. The server doesn’t remember anything about what you asked before, so each time you talk to it, it’s like a brand-new conversation.
Client-Server Architecture: The client and server have distinct responsibilities. The client handles the user interface and user experience, while the server manages data storage and business logic.
Uniform Interface: This is a way to make sure that all the different parts of an API talk to each other in the same way, making everything easier to understand and work with. Here are the main parts:
Resource Identification: Think of resources like items in a library. Each item (resource) has a special address (URI) so you can find it easily.
Resource Representation: When you get a resource, it's shown in a format like JSON or XML, which are like different ways of writing down the same information.
Self-descriptive Messages: Each time you send a request or get a response, it includes all the information needed to understand it, so you don’t need extra instructions.
Hypermedia as the Engine of Application State (HATEOAS): When you get information from the server, it also gives you links to other related information, just like how you can click on links to go to other pages on a website. This helps you know what actions you can take next.
Layered System: Imagine a big cake with many layers. Each layer of the cake has a different flavor and purpose. In a similar way, an API can be made up of different layers, and each layer has its own job. For example, one layer might handle security, another might deal with storing data, and another might manage communication. This way, the API is easier to manage and can grow bigger without getting messy, just like adding more layers to the cake.
Cacheability: Think about when you play a video game and it saves your progress so you don’t have to start from the beginning each time. In a similar way, an API can save (cache) some of the answers it gives you so it doesn’t have to find the same answer again and again. If the response is cacheable, the next time you ask for the same thing, you get the answer faster because it was saved. If it’s not cacheable, the API has to find the answer again from scratch each time.
Designing a RESTful API
When designing a RESTful API, consider the following steps:
Identify Resources: Determine the primary entities your API will manage (e.g., users, products, orders) and create URIs to represent them.
Define Endpoints: Create endpoints for each resource. Use HTTP methods to perform CRUD operations:
GET: Retrieve a resource or a list of resources.
POST: Create a new resource.
PUT: Update an existing resource.
DELETE: Remove a resource.
Use Meaningful Resource Names: Use nouns to name your resources rather than verbs. For example,
/users
is preferable to/getUsers
.Implement Filtering, Sorting, and Pagination: Allow clients to filter, sort, and paginate through resources to improve performance and usability.
Handle Errors Gracefully: Return appropriate HTTP status codes and error messages. Common status codes include:
200 OK
: The request was successful.201 Created
: A new resource was created successfully.400 Bad Request
: The request was invalid or cannot be processed.404 Not Found
: The requested resource was not found.500 Internal Server Error
: An unexpected server error occurred.
Secure Your API: Implement authentication and authorization to protect your API. Use HTTPS to encrypt data in transit.
Implementing a RESTful API
Let's walk through a simple example of implementing a RESTful API using Node.js and Express:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
let users = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' }
];
// Get all users
app.get('/users', (req, res) => {
res.json(users);
});
// Get a single user
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.json(user);
});
// Create a new user
app.post('/users', (req, res) => {
const user = {
id: users.length + 1,
name: req.body.name
};
users.push(user);
res.status(201).json(user);
});
// Update an existing user
app.put('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
user.name = req.body.name;
res.json(user);
});
// Delete a user
app.delete('/users/:id', (req, res) => {
const userIndex = users.findIndex(u => u.id === parseInt(req.params.id));
if (userIndex === -1) return res.status(404).send('User not found');
users.splice(userIndex, 1);
res.status(204).send();
});
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
In this example, we define a simple API for managing users with endpoints to get all users, get a single user by ID, create a new user, update an existing user, and delete a user. This basic setup can be expanded to include more complex functionality and error handling.
Testing RESTful APIs
Several tools are available for testing RESTful APIs:
Postman: A popular tool for testing APIs, allowing you to create, send, and save requests, as well as automate tests.
Insomnia: An easy-to-use API client for designing and debugging RESTful APIs.
Swagger: A powerful framework for designing, building, and documenting RESTful APIs. It provides a user-friendly interface for testing endpoints.
Curl: A command-line tool for making HTTP requests, useful for quick testing and scripting.
Conclusion
Understanding RESTful APIs is essential for modern web development. By adhering to REST principles and best practices, you can design and implement APIs that are scalable, maintainable, and enable seamless communication between different software systems. Whether you're building a new API or working with existing ones, mastering RESTful concepts will significantly enhance your development skills and open up new opportunities in your career.
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
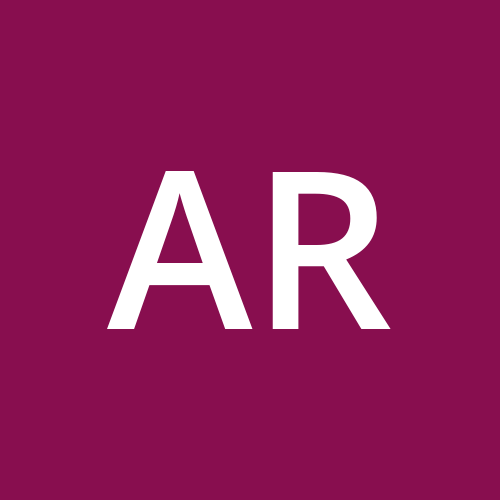