Easy Way to Add Environment Variables in React
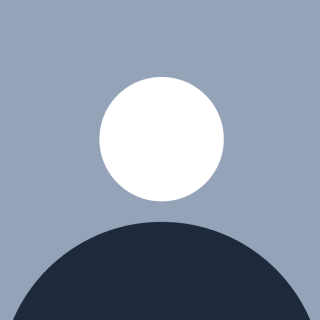
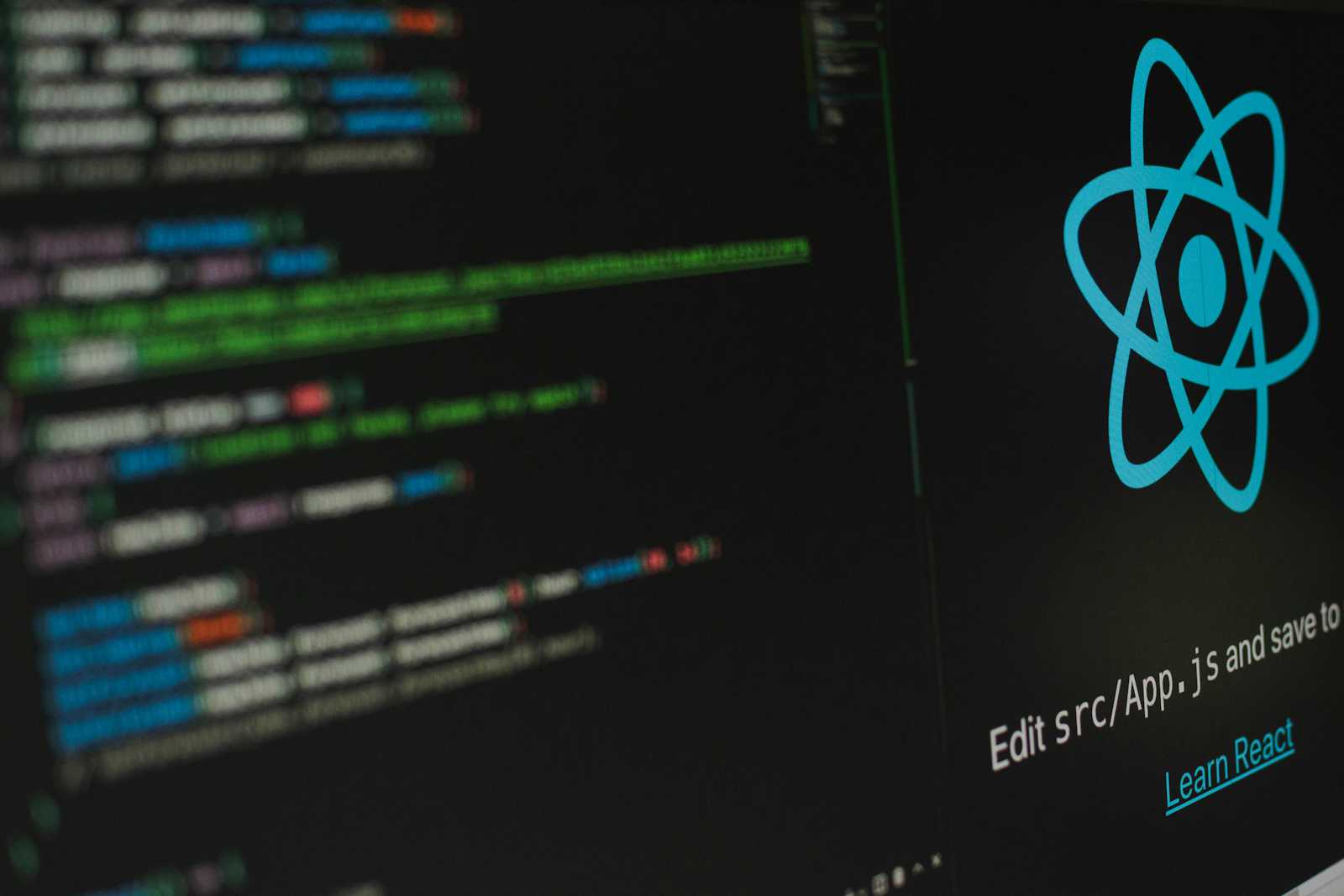
Environment variables are crucial in software development, enabling different configurations for development, testing, and production environments. In React, they help manage these settings efficiently without hardcoding sensitive information into your application.
Learn how to efficiently manage environment variables in your React applications with this comprehensive guide. Discover best practices, step-by-step setups using create-react-app and Vite, and ensure secure, maintainable configurations across different environments.
What are environment variables?
Environment variables are key-value pairs used to configure applications. They allow developers to define various settings outside the codebase, making it easier to manage different configurations across multiple environments (e.g., development, testing, production). Common use cases include setting API endpoints, feature flags, and other configuration details that might differ between environments.
Setting Up Environment variables in React using npx create-react-app
Create a '.env' file:
At the root of your React project, create a
.env
file. This file will contain your environment variables.REACT_APP_API_URL=https://api.example.com REACT_APP_SECRET_KEY=14dfs24adfdf324
NOTE:
All environment variables in React must be prefixed with
REACT_APP_
. This convention ensures that only variables meant to be exposed to the frontend are included.Access Environment Variables In Code
const apiUrl = process.env.REACT_APP_API_URL; const secret = process.env.REACT_APP_SECRET_KEY; console.log('API uri:', apiUrl); console.log('Secret key:', secret);
Restart the Development Server
npm run start
Setting Up Environment variables in React using npm create vite
Create a '.env' file:
At the root of your React project, create a
.env
file. This file will contain your environment variables.VITE_API_URL=https://api.example.com VITE_SECRET_KEY=14dfs24adfdf324
NOTE:
All environment variables in React must be prefixed with
VITE_
. This convention ensures that only variables meant to be exposed to the frontend are included.Access The Environment Variable
const apiUrl = import.meta.env.VITE_API_URL;
const secret = import.meta.env.VITE_SECRET_KEY;
console.log('API uri:', apiUrl);
console.log('Secret key:', secret);
NOTE: Here import.meta.env
is used instead of process.env
Restart the Development server
npm run dev
or
npx vite
Using Environment Variables in build Scripts
The best way to set environment variables in build scripts is by using
dotenv
Install
dotenv
npm install dotenv
Best Practices To Follow:
Use Different Files for Different Environments: Maintain separate environment variable files for different environments (e.g.,
.env.development
,.env.production
,.env.test
).Do Not Commit
.env
Files to Version Control: Environment variable files often contain sensitive information. Add them to your.gitignore
to avoid committing them to version control..env .env.*
Conclusion:
Environment variables are a powerful tool for managing different configurations in a React application. By following best practices and using the right tools, you can ensure that your application is secure, maintainable, and easy to configure across different environments. Whether you're working on a small project or a large enterprise application, understanding and effectively managing environment variables will significantly enhance your development workflow.
Subscribe to my newsletter
Read articles from Divij Pirankar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by