How to Create a Stunning 3D Credit Card Flip Animation Using HTML and CSS
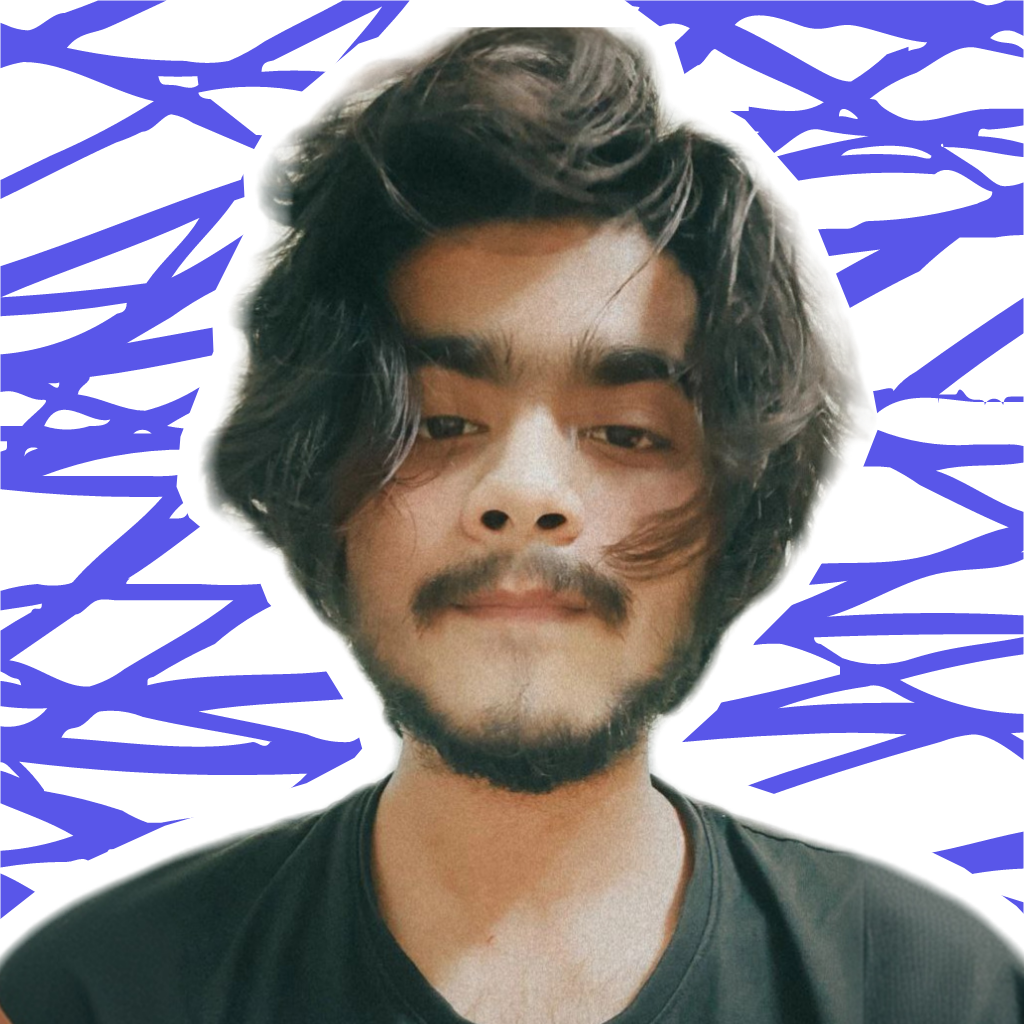

Creating visually appealing animations can significantly enhance the user experience on your website. In this article, we'll guide you through a step-by-step process to create a 3D credit card flip animation using HTML and CSS. This tutorial is part of day 43 of the #100DaysOfCode Challenge, and the full source code can be downloaded here. If you have any questions, feel free to contact me here.
Introduction
Animations can bring life to your web projects, making them more engaging and interactive. In this tutorial, we'll create a 3D credit card flip animation using just HTML and CSS. This project will help you understand how to use CSS transformations and transitions to create a 3D effect.
Prerequisites
Before we begin, you should have a basic understanding of HTML and CSS. Familiarity with CSS animations and transformations will be helpful but is not required.
Setting Up the HTML Structure
Creating the Basic HTML Skeleton
First, we need to set up our basic HTML skeleton. This includes the <!DOCTYPE html>
, <html>
, <head>
, and <body>
tags.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>3D Credit Card Flip Animation</title>
</head>
<body>
<!-- Container for the flip card -->
<div class="flip-card">
<!-- Inner container for the flip card -->
<div class="flip-card-inner">
<!-- Front side of the flip card -->
<div class="flip-card-front">
<!-- Content goes here -->
</div>
<!-- Back side of the flip card -->
<div class="flip-card-back">
<!-- Content goes here -->
</div>
</div>
</div>
</body>
</html>
Adding the Flip Card Container
The flip card container will hold both the front and back sides of our credit card.
<div class="flip-card">
<div class="flip-card-inner">
<div class="flip-card-front">
<!-- Front side content -->
</div>
<div class="flip-card-back">
<!-- Back side content -->
</div>
</div>
</div>
Designing the Front Side of the Card
Inside the .flip-card-front
div, we'll add various elements like the Mastercard logo, chip, card number, expiry date, and cardholder's name.
<div class="flip-card-front">
<p class="heading_8264">MASTERCARD</p>
<svg class="logo" xmlns="http://www.w3.org/2000/svg" x="0px" y="0px" width="36" height="36" viewBox="0 0 48 48">
<!-- SVG paths for the Mastercard logo -->
</svg>
<svg version="1.1" class="chip" id="Layer_1" xmlns="http://www.w3.org/2000/svg" x="0px" y="0px" width="30px" height="30px" viewBox="0 0 50 50">
<!-- SVG paths for the chip -->
</svg>
<svg version="1.1" class="contactless" id="Layer_1" xmlns="http://www.w3.org/2000/svg" x="0px" y="0px" width="20px" height="20px" viewBox="0 0 50 50">
<!-- SVG paths for the contactless symbol -->
</svg>
<p class="number">5781 3976 5976 9438</p>
<p class="valid_thru">VALID THRU</p>
<p class="date_8264">12 / 24</p>
<p class="name">AARZOO ISLAM</p>
</div>
Designing the Back Side of the Card
The back side will include the magnetic strip, a middle strip, and a signature strip with a security code.
<div class="flip-card-back">
<div class="strip"></div>
<div class="mstrip"></div>
<div class="sstrip">
<p class="code">***</p>
</div>
</div>
Styling with CSS
Importing Google Fonts
We'll import Google Fonts to give our card a sleek look.
@import url('https://fonts.googleapis.com/css2?family=Inconsolata:wght@200..900&family=Inter:wght@100..900&display=swap');
Resetting Margins and Paddings
It's good practice to reset margins and paddings for all elements to ensure consistency.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
Styling the Body
We'll center our flip card on the page and add a nice background gradient.
body {
display: flex;
align-items: center;
justify-content: center;
min-height: 100vh;
background-image: linear-gradient(-20deg, #e9defa 0%, #fbfcdb 100%);
font-family: "Inconsolata", monospace;
}
Styling the Flip Card Container
The flip card needs a perspective for the 3D effect, and we'll set its width, height, and colors.
.flip-card {
background-color: transparent;
width: 240px;
height: 154px;
perspective: 1000px;
color: white;
}
Adding the 3D Flip Animation
We'll add the rotation and transition effects to create the 3D flip animation.
.flip-card-inner {
position: relative;
width: 100%;
height: 100%;
text-align: center;
transition: transform 0.8s;
transform-style: preserve-3d;
}
.flip-card:hover .flip-card-inner {
transform: rotateY(180deg);
}
Adding Details to the Credit Card
Adding the Mastercard Logo
Positioning the Mastercard logo using absolute positioning.
.logo {
position: absolute;
top: 6.8em;
left: 11.7em;
}
Adding the Chip
Positioning the chip icon on the card.
.chip {
position: absolute;
top: 2.3em;
left: 1.5em;
}
Adding the Contactless Payment Symbol
Positioning the contactless payment symbol.
.contactless {
position: absolute;
top: 3.5em;
left: 12.4em;
}
Adding the Card Number and Expiry Date
Styling and positioning the card number and expiry date.
.number {
position: absolute;
font-weight: bold;
font-size: .6em;
top: 8.3em;
left: 1.6em;
}
.valid_thru {
position: absolute;
font-weight: bold;
top: 635.8em;
font-size: .01em;
left: 140.3em;
}
.date_8264 {
position: absolute;
font-weight: bold;
font-size: 0.5em;
top: 13.6em;
left: 3.2em;
}
Adding the Cardholder's Name
Positioning the cardholder's name.
.name {
position: absolute;
font-weight: bold;
font-size: 0.5em;
top: 16.1em;
left: 2em;
}
Designing the Magnetic Strip
Creating the magnetic strip on the back side.
.strip {
position: absolute;
background-color: black;
width: 15em;
height: 1.5
em;
top: 1.3em;
left: .7em;
}
Designing the Signature Strip and Security Code
Adding the signature strip and security code.
.sstrip {
position: absolute;
background-color: white;
width: 9em;
height: 1.4em;
top: 6.4em;
left: .7em;
}
.code {
position: absolute;
color: black;
top: .3em;
left: 9.8em;
}
Implementing the Flip Animation
The CSS we added to the flip card container earlier enables the flip animation. Hovering over the card triggers the 180-degree rotation, creating a smooth 3D effect.
Final Touches
You can further refine the design by adjusting colors, adding shadows, and fine-tuning positions to suit your needs. Feel free to experiment and add your own creativity to make the card look more realistic or fit the theme of your website.
Conclusion
Creating a 3D credit card flip animation with HTML and CSS is a fantastic way to enhance your web development skills and add a dynamic element to your projects. By following this tutorial, you've learned how to use CSS transformations and transitions to achieve a stunning 3D effect. Keep experimenting with different styles and animations to continue improving your web design abilities.
FAQs
Q1: Can I use images instead of SVG for the card elements?
Yes, you can use images instead of SVGs. Simply replace the SVG elements with <img>
tags and link to your image files.
Q2: How can I make the card flip automatically without hovering?
You can use JavaScript to add a class that triggers the flip animation automatically after a certain time. For example:
document.addEventListener("DOMContentLoaded", function() {
setTimeout(function() {
document.querySelector('.flip-card-inner').classList.add('flipped');
}, 2000); // Flip after 2 seconds
});
Q3: How do I make the card responsive?
Use relative units like percentages or em
for sizing and positioning elements. Additionally, use media queries to adjust the layout for different screen sizes.
Q4: Can I add more details to the card?
Absolutely! You can add additional elements like card issuer logos, holograms, or any other details you like.
Q5: Is it possible to use this animation in a real payment system?
While this animation is great for visual effects and user interfaces, real payment systems require secure, server-side processing. Use this animation as a visual enhancement rather than a functional payment system.
Subscribe to my newsletter
Read articles from Aarzoo Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
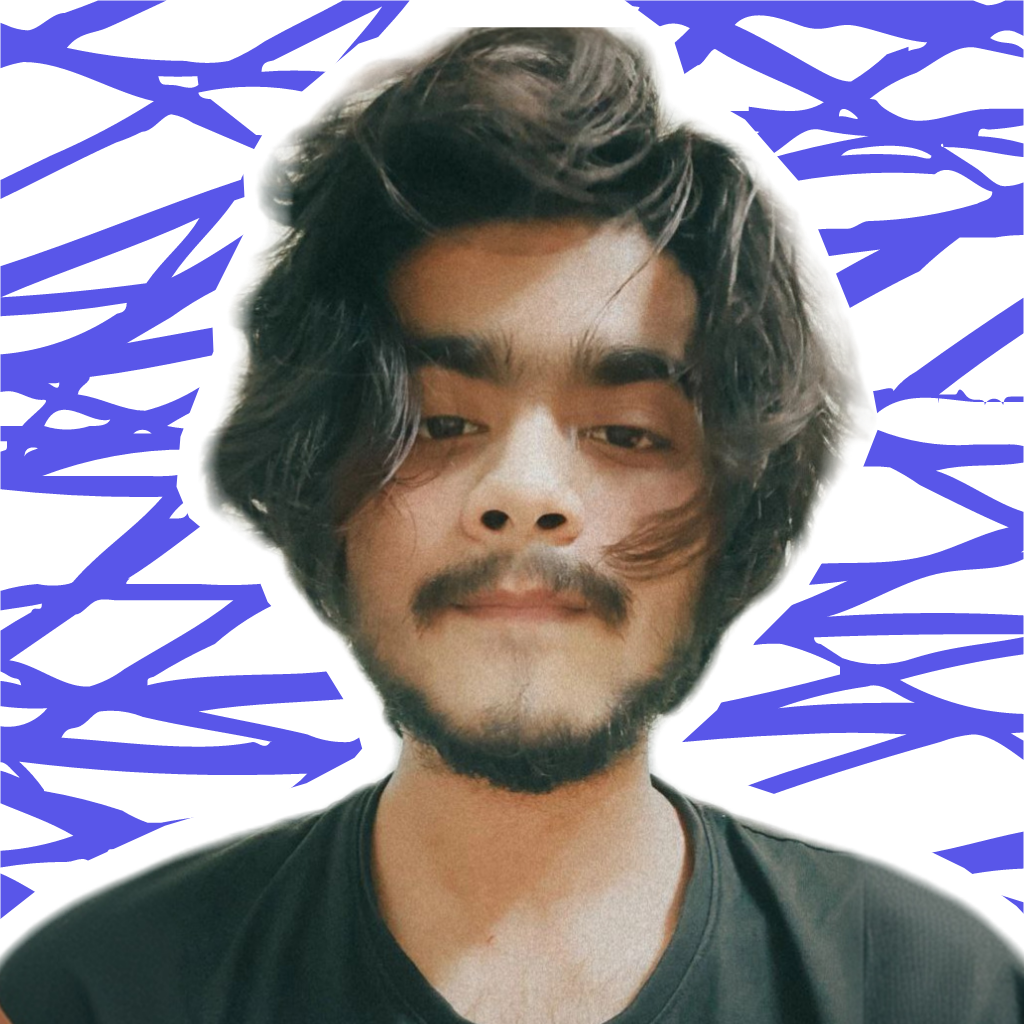
Aarzoo Islam
Aarzoo Islam
Founder of AroNus ๐ | Full-stack web developer ๐จ๐ปโ๐ป | Sharing web development tips and showcasing projects ๐ | Transforming ideas into digital realities ๐