Sealed Classes - JDK 17
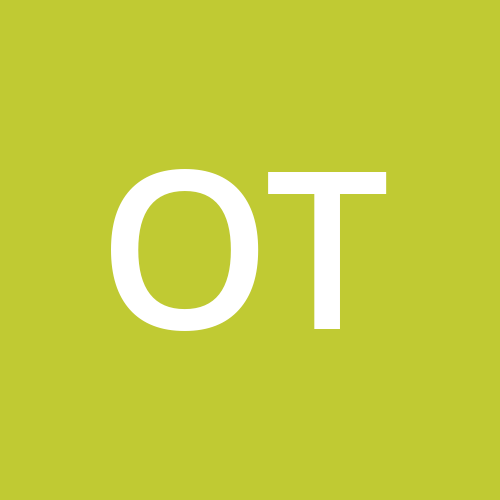
JDK 17 has introduced a lot of new features, one of them is Sealed Classes
. Let's understand what are sealed classes and why were they introduced.
We will not use the Animal -> Dog -> Red Dog -> Multicolor Dog or House -> Door -> Mouse type examples as they don't represent what domains do Software Systems deal with.
Consider a scenario where you are building a system that provides the user with home loan interest rates of various banks and lenders. A common dilemma buyers face is opting between a Fixed Interest Loan or a Floating Interest Loan, if you are not aware of these terms, please have a quick read here.
Please be aware that Floating Interest Rates are offered by Nationalized Banks and Fixed Interest Rates are offered by NBFCs in India. If you are not aware of the difference between the two, please have a quick read here. Please be aware that only Nationalized Banks can offer Floating Interest Loans and NBFCs can offer Fixed Interest Loans. This is the because the former is directly and regulated by RBI and the latter isn't. Here is a quick explainer regarding the difference between the two NBFC vs Banks in Home Loans.
Hopefully, you now have a brief idea about the banking/finance terms we have mentioned previously.
Let's assume that we have to build a Java based system, we will declare an interface FloatingInterest
that any class can implement. But wait, we don't want anyone to implement FloatingInterest interface as only Nationalized Banks can offer that. Hence we would like to restrict who implements this interface. This is where we can use the concept of sealed interfaces
. With that understood, let's delve into the most fun part that is the code.
Notice the sealed
keyword before the interface
keyword and the permits
keyword after the interface name. The sealed
keyword must be used the permits
keyword to restrict who can implement the interface. Here we have made the FloatingInterestRate
interface sealed and permitted only NationalBank to implement/extend it, hence restricting the misuse of the interface.
Let's take a look at the FixedInterestRate
interface.
We keep the interface sealed
and permit only NBFCs to implement/extend it. Hence we prevent any other Financial Institutions misusing this functionality as it can only be provided by a restricted class(NBFC).
Let's now look at the NationalBank
class that implements the FloatingInterestRate
interface.
This class is again a sealed one as any class/interface that extends/implements a sealed super type must be declared assealed
or non-sealed
or final
. Hence we declare NationalBank
as sealed and permit a few known nationalized Banks in India. Here are the nationalized banks by RBI, google search results.
Here is class SBI
representing the largest nationalized bank in India.
We have declared it as final
as we are extending a sealed
class.
Here is another sub-class of the NationalBank
class.
Notice that this class is also final
.
Let's see what we have done with the FixedInterestRate
interface.
The sealed
NBFC class implements it and permits only few NBFCs in India to extend it.
Here are a few sub-classes of the NBFC class:-
Notice that the interest rates are way higher than what the Nationalized Banks offer, before this turns into a Home Loan advice blog, please notice that we extend the sealed
NBFC class and thus keep our class as final and override the methods.
Hence, we can conclude that if we want to restrict inheritance and reusability in our code to keep it domain specific, we should use the sealed
keyword.
All the code discussed above can be found in this repository on GitHub.
An argument can be made saying that "Why does the NationalBank class restrict the sub-types to a few?". The reason being, establishment of a Nationalized Bank does not happen frequently and thus we keep the NationalBank
class sealed until new sub-types are available.
Note:- Any sub-classes/sub-interfaces you mention after the permits
keyword must be present in the same package as the super-type(sealed type).
And that's it. I know it was a long read for a short feature but we understood with a real-world use case where sealed classes will be extremely useful.
Feel free to discuss in the comments below or drop me a mail regarding suggestions to improve!
Subscribe to my newsletter
Read articles from Omkar Terbhai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
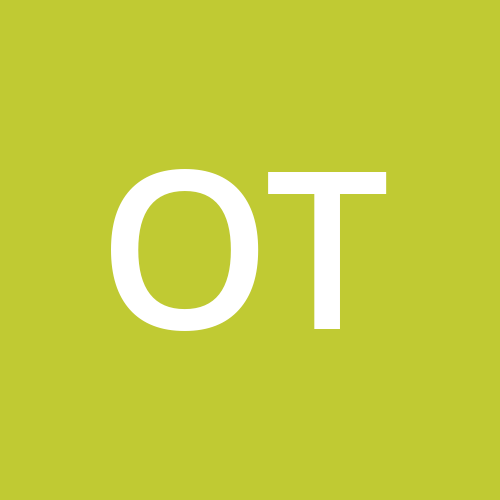