18 Java - Collection Framework
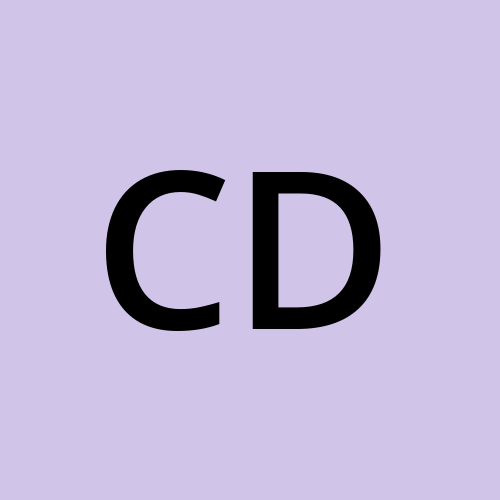
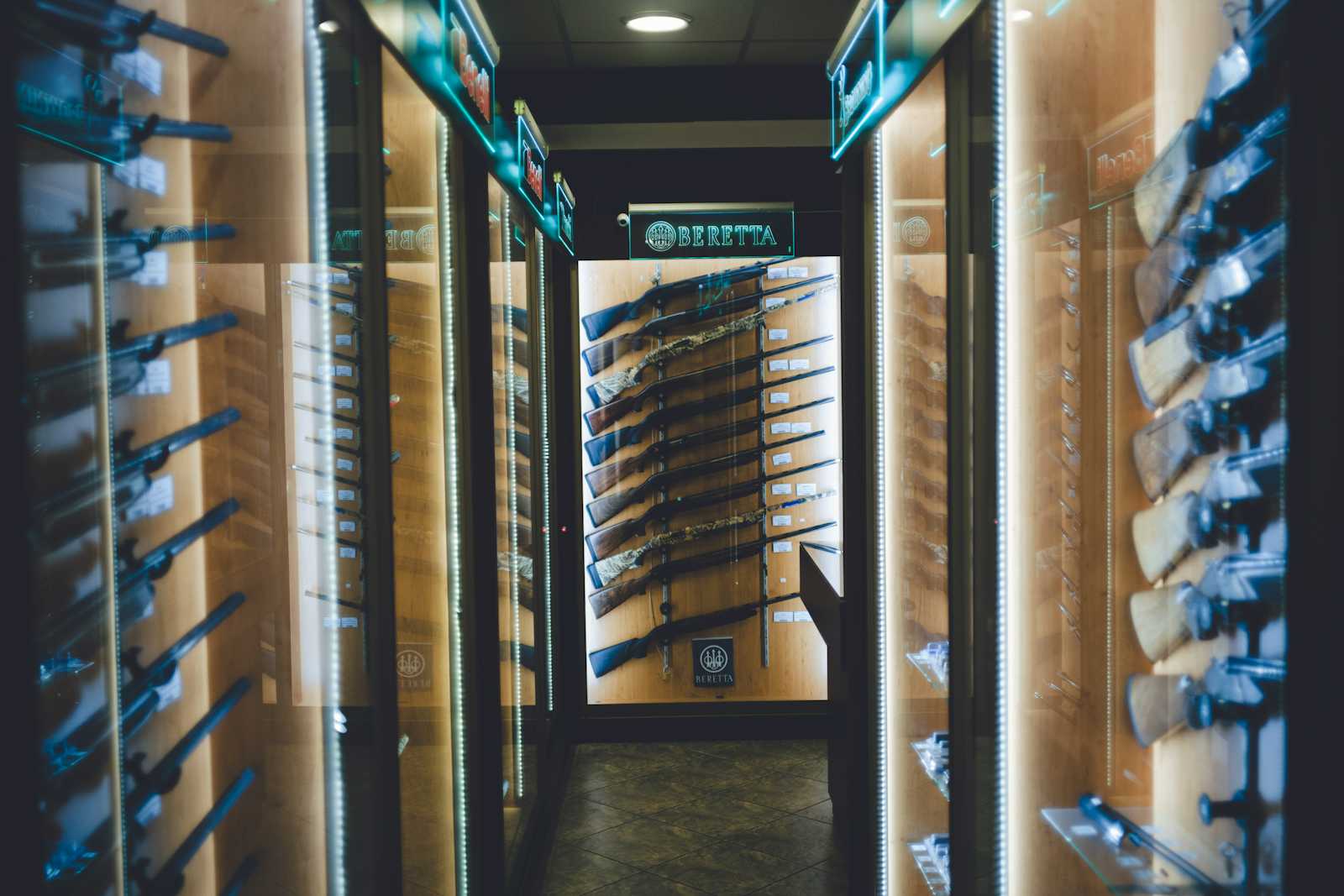
What is Java Collections Framework - JCF?
JCF Added in Java version 1.2
Collections is nothing but a group of Objects.
Present in java.util package.
Framework provide us the architecture to manage these "group of objects" i.e add, update, delete, search etc.
Why we need Java Collections Framework?
Prior to JCF, we have Array, Vector, Hash tables.
But problem with that is, there is no common interface, so its difficult to remeber the methods for each.
public class Main {
public static void main(String[] args) {
int arr[] = new int[4];
//insert an element in an array
arr[0] = 1;
//get front element
int val = arr[0];
Vector<Integer> vector = new Vector<>();
//insert an element in vector
vector.add(1);
//get element
vector.get(0);
}
}
Java Collections Framework Hierarchy
Map is not part of Collection
Iterable
Used to TRAVERSE the collection.
Below are the 2 methods which are frequently used.
Iterator()
Available in Java 1.5
It returns the Iterator Object, which provides below methods to iterate the collection.
Method Name | Usage |
hasNext() | returns true, if there are more elements in the collection. |
next() | returns the next element in the iteration |
remove() | Removes the last element returned by iterator |
forEach()
Available in Java 1.8
Iterate using lambda expression. Lambda expression is called for each element in the collection.
public class Main {
public static void main(String[] args) {
List<Integer> values = new ArrayList<>();
values.add(1);
values.add(2);
values.add(3);
values.add(4);
//using iterator
System.out.println("Interating the values using iterator method");
Iterator<Integer> valuesIterator = values.iterator();
while (valuesIterator.hasNext()){
int val = valuesIterator.next();
System.out.println(val);
if (val==3){
valuesIterator.remove();
}
}
System.out.println("Iterating the values using for each loop");
for (int val: values){
System.out.println(val);
}
//using forEach method
System.out.println("testing forEach method");
values.forEach((Integer val) -> System.out.println(val));
}
}
/*
Interating the values using iterator method
1
2
3
4
Iterating the values using for each loop
1
2
4
testing forEach method
1
2
4
*/
Collection
It represents the group of Objects. Its an interface which provides methods to work on group of objects.
Below are the most common used methods which are implemented by its child classes like ArrayList, Stack, LinkedList etc. Stream and parllel stream introduced in Java 1.8 and remaining all are introduced in Java 1.2
size, isEmpty, equals, contains
toArray, clear
add, addAll, remove, removeAll,
stream & parallelStream, Iterator
Methods | Usage |
size() | It returns the total number of elements present in the collection |
isEmpty() | Used to check if collection is empty or has some value. It return true/false |
contains() | Used to search an element in the collection |
toArray() | It convert collection into an Array. |
add() | Used to insert an element in the collection |
remove() | Used to remove an element from the collection |
addAll() | Used to insert one collection in another collection. |
removeAll() | Remove all the elements from the collections, which are present in the collection passed in the parameter |
clear() | Remove all the elements from the collection |
equals() | Used to check if 2 collections are equal or not. |
stream() and parallelStream() | Provide effective way to work with collection like filtering, processing data ..etc. |
Iterator() | As iterable interface added in java 1.5, so before this , this method was used to iterate the collection and still can be used. |
public class Main {
public static void main(String[] args) {
List<Integer> values = new ArrayList<>();
values.add(2);
values.add(3);
values.add(4);
//size
System.out.println("size: "+ values.size());
//isEmpty
System.out.println("isEmpty: "+values.isEmpty());
//contains
System.out.println("contains: "+values.contains(5));
//add
values.add(5);
System.out.println("added: "+values.contains(5));
//remove using index
values.remove(3);
System.out.println("remove using index: "+values.contains(5));
//remove using Object, removes the first occurrence of the value
values.remove(Integer.valueOf(3));
System.out.println("removed using object: "+values.contains(3));
Stack<Integer> stackValues = new Stack<>();
stackValues.add(6);
stackValues.add(7);
stackValues.add(8);
//addAll
values.addAll(stackValues);
System.out.println("addAll test using containsAll: "+values.containsAll(stackValues));
//containsAll
values.remove(Integer.valueOf(7));
System.out.println("containsAll after removing 1 element: "+values.containsAll(stackValues));
//removeAll
values.removeAll(stackValues);
System.out.println("removeAll: "+values.contains(8));
//clear
values.clear();
System.out.println("clear: "+values.isEmpty());
}
}
/*
size: 3
isEmpty: false
contains: false
added: true
remove using index: false
removed using object: false
addAll test using containsAll: true
containsAll after removing 1 element: false
removeAll: false
clear: true
*/
Collection vs Collections
Collection is part of Java Collection Framework. And its an interface, which expose various methods which is implemented by various collection classes like ArrayList, Stack, LinkedList etc.
Collections is a Utility class and provide static methods, which are used to operate on collections like sorting, swapping, searching, reverse, copy etc.
Methods |
Sort |
binarySearch |
get |
reverse |
shuffle |
swap |
copy |
min |
max |
rotate |
unmodifiableCollection |
public class Main {
public static void main(String[] args) {
List<Integer> values = new ArrayList<>();
values.add(1);
values.add(3);
values.add(2);
values.add(4);
System.out.println("max value: "+Collections.max(values));
System.out.println("min value: "+Collections.min(values));
Collections.sort(values);
System.out.println("sorted");
values.forEach((Integer val) -> System.out.println(val));
}
}
max value: 4
min value: 1
sorted
1
2
3
4
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
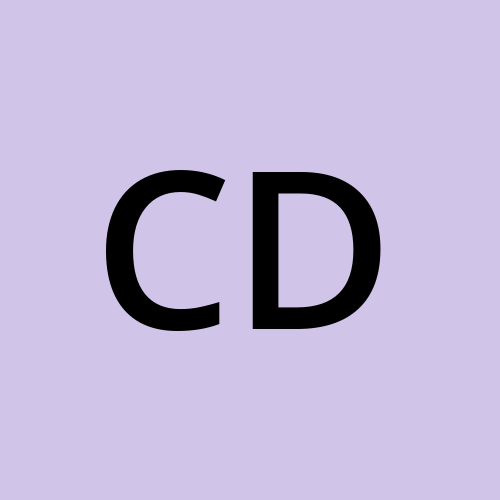
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.