Flutter with Riverpod State Management

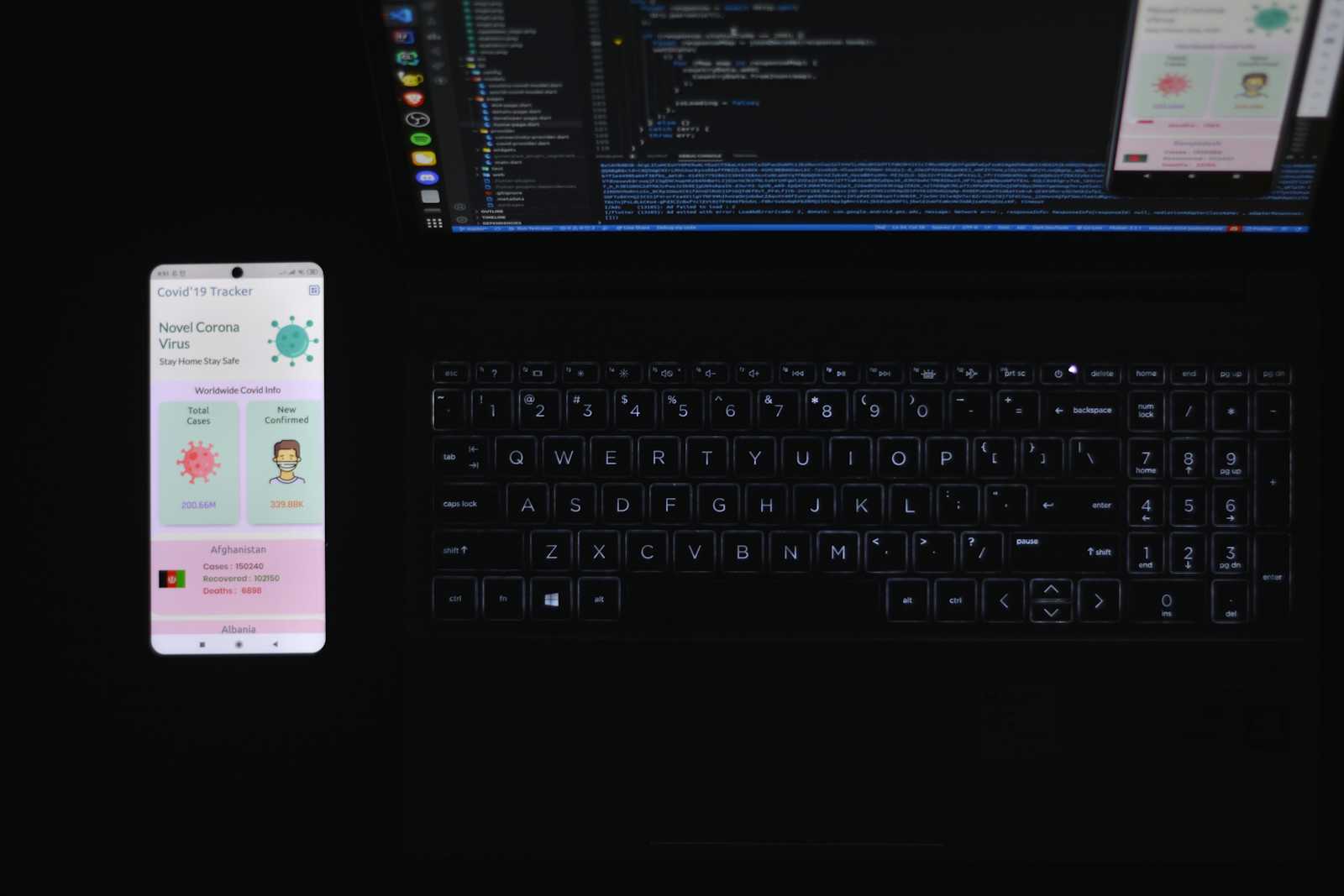
Back at it, this time we are doing flutter. So there are several state management tools that can be used in flutter that can manage both local and global state. Riverpod is one such management tool. It was recently introduced and the whole scope of riverpod is built of provider. For the flutter OG's you guys know provider. So just like provider riverpod is easy to implement but riverpod is good as it is better at building for more complex systems and is more robust.
Setup
First you can create your flutter application with the simple flutter command flutter create <app_name>
After that your application main.dart
file should look a little something like this:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Theme.of(context).colorScheme.inversePrimary,
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.headlineMedium,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
), // This trailing comma makes auto-formatting nicer for build methods.
);
}
}
This is flutter's boilerplate for any new application now let us modify it so that we can use riverpod.
Create a new file called app.dart
this is where we are going to setup the application uses and wrappers that we want to be accessed globally in the whole application. One such package that I personally love using is screenutil this allows for you to set adapting font sizes and screen depending on the different screen layout. Ok now let us put it together see how it looks:
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_screenutil/flutter_screenutil.dart';
class Snow extends StatelessWidget {
const Snow({super.key});
@override
Widget build(BuildContext context) {
return ScreenUtilInit( // makes the plugin global accessed
builder: (BuildContext context, Widget? child) {
return MaterialApp(
title: 'Snow snippets',
debugShowCheckedModeBanner: false,
theme: ThemeData.dark(), // application theme color
);
},
);
}
}
Looks easy to modify right now that we have set the global plugins that we want to use now we can get to riverpod.
Riverpod installation
Now we have all the application perquisites that we would want now let us put the state management. Head over to pub.dev and search for riverpod. The package that we are going to use is flutter_riverpod, once we have this we can add it to the pubspec.yaml
this is the file that all our dependencies go to. I will show an example as I set the flutter riverpod
dependencies:
flutter:
sdk: flutter
# any other dependancy can go under here
cupertino_icons: ^1.0.6
flutter_screenutil: ^5.9.3 # screenutil dependancy
flutter_riverpod: ^2.5.1 # riverpod dependancy
Almost there, Now let us wrap our application with the riverpod provider scope. This ensures that the whole system can read the riverpod providers when they are used even in constant variable files.
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'package:zeus/app.dart';
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
runApp(
const ProviderScope(
child: Snow(),
),
);
}
Conclusion
And just like that you are all set to use riverpod in the application for your state management needs. Simple right well take the article above and go give it a try. This can not limit you. If you decide then you can layer other state management tools over it we will do that in a later article. Till then Snow signing out.
Subscribe to my newsletter
Read articles from George Onyango directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
