How to Create an Animated Multi Device Loader
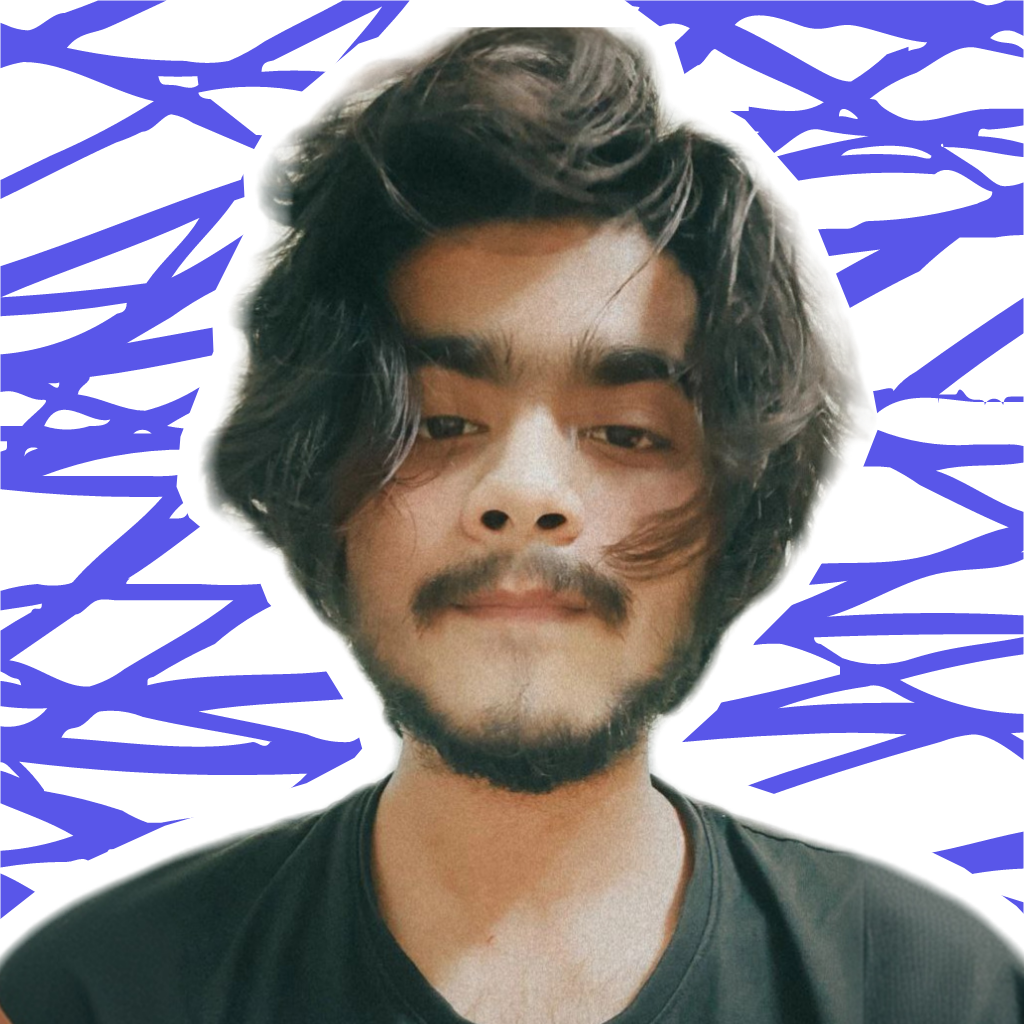
Table of contents
- Introduction
- Setting Up the HTML Structure
- Creating the Loader Container
- Styling the Body with CSS
- Styling the Loader Elements
- Adding Animation to Loaders
- Understanding CSS Animations
- Customizing Loader Colors and Sizes
- Responsive Design Considerations
- Debugging Common Issues
- Enhancing with Additional Features
- Optimizing Performance
- Deploying the Loader
- Conclusion
- FAQs
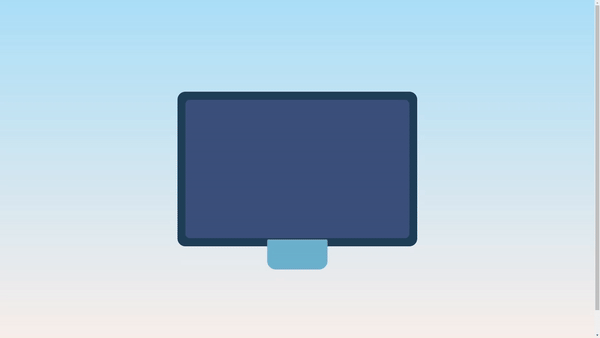
Introduction
Loaders are an essential aspect of web development, providing users with visual feedback while content loads. In this guide, we'll create an animated multi-device loader, a perfect addition to your web projects. This tutorial is part of the #100DaysOfCode Challenge, specifically Day 44. Let's dive into the HTML and CSS required to build this engaging loader.
Setting Up the HTML Structure
First, we need to create the basic structure of our HTML document. We'll use a simple HTML5 boilerplate to get started.
<!DOCTYPE html>
<html lang="en">
<head>
<!-- Specifies the character encoding for the HTML document -->
<meta charset="UTF-8">
<!-- Ensures the page is responsive by setting the viewport to match the device's width and initial scale -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Links to an external CSS stylesheet for styling the page -->
<link rel="stylesheet" href="style.css">
<!-- Title of the webpage that appears in the browser tab -->
<title>Animated Multi Device Loader</title>
</head>
<body>
<!-- Container div that holds the loader elements -->
<div class="container">
<!-- Individual loader elements (3 in total) -->
<div class="loader"></div>
<div class="loader"></div>
<div class="loader"></div>
</div>
</body>
</html>
This code sets up the basic HTML structure with meta tags for character encoding and viewport settings, a link to an external CSS file, and a simple title. The body contains a container div that will hold our loader elements.
Creating the Loader Container
The container is essential for holding and styling the loader elements. Here, we define three loader elements within the container.
<div class="container">
<div class="loader"></div>
<div class="loader"></div>
<div class="loader"></div>
</div>
These div elements will be styled and animated using CSS to create our loader effect.
Styling the Body with CSS
Let's move on to styling our HTML elements using CSS. We'll start by centering the content and applying a gradient background to the body.
/* Styling for the body element */
body {
/* Use flexbox layout to center content horizontally and vertically */
display: flex;
align-items: center;
justify-content: center;
/* Set the minimum height to full viewport height */
min-height: 100vh;
/* Apply a linear gradient background */
background-image: linear-gradient(to top, #fff1eb 0%, #ace0f9 100%);
}
This CSS uses Flexbox to center the container both horizontally and vertically within the viewport. The background is a smooth gradient transitioning from a light peach color to a soft blue.
Styling the Loader Elements
Next, we'll define the general styles for the loader elements and then apply specific styles to each loader.
/* Styling for the loader elements */
.loader {
/* Position the loader absolutely in the center */
position: absolute;
top: 50%;
left: 50%;
z-index: 10;
/* Set initial dimensions and positioning */
width: 160px;
height: 100px;
margin-left: -80px;
margin-top: -50px;
/* Round the corners of the loader */
border-radius: 5px;
/* Set the background color */
background: #1e3f57;
/* Apply animation */
animation: dot1_ 3s cubic-bezier(0.55, 0.3, 0.24, 0.99) infinite;
}
/* Styling for the second loader element */
.loader:nth-child(2) {
z-index: 11;
/* Set initial dimensions and positioning */
width: 150px;
height: 90px;
margin-top: -45px;
margin-left: -75px;
/* Round the corners differently */
border-radius: 3px;
/* Set a different background color */
background: #3c517d;
/* Use a different animation */
animation-name: dot2_;
}
/* Styling for the third loader element */
.loader:nth-child(3) {
z-index: 12;
/* Set initial dimensions and positioning */
width: 40px;
height: 20px;
margin-top: 50px;
margin-left: -20px;
/* Round only the bottom corners */
border-radius: 0 0 5px 5px;
/* Set another different background color */
background: #6bb2cd;
/* Use another different animation */
animation-name: dot3_;
}
Each loader has unique dimensions, positions, border-radius settings, and background colors. The animations for each loader will create the dynamic effect.
Adding Animation to Loaders
Now, we'll define the keyframes for the animations of each loader element. These animations control the changes in size and position over time.
/* Keyframes for the first loader's animation */
@keyframes dot1_ {
3%, 97% {
width: 160px;
height: 100px;
margin-top: -50px;
margin-left: -80px;
}
30%, 36% {
width: 80px;
height: 120px;
margin-top: -60px;
margin-left: -40px;
}
63%, 69% {
width: 40px;
height: 80px;
margin-top: -40px;
margin-left: -20px;
}
}
/* Keyframes for the second loader's animation */
@keyframes dot2_ {
3%, 97% {
height: 90px;
width: 150px;
margin-left: -75px;
margin-top: -45px;
}
30%, 36% {
width: 70px;
height: 96px;
margin-left: -35px;
margin-top: -48px;
}
63%, 69% {
width: 32px;
height: 60px;
margin-left: -16px;
margin-top: -30px;
}
}
/* Keyframes for the third loader's animation */
@keyframes dot3_ {
3%, 97% {
height: 20px;
width: 40px;
margin-left: -20px;
margin-top: 50px;
}
30%, 36% {
width: 8px;
height: 8px;
margin-left: -5px;
margin-top: 49px;
/* Round the entire element */
border-radius: 8px;
}
63%, 69% {
width: 16px;
height: 4px;
margin-left: -8px;
margin-top: -37px;
/* Round the entire element differently */
border-radius: 10px;
}
}
These keyframes dictate the transformation of each loader element at different stages of the animation cycle, creating a dynamic and engaging loader effect.
Understanding CSS Animations
To fully grasp the power of these animations, it's essential
to understand the animation
property and how to use keyframes effectively.
The Animation Property: This property combines several sub-properties, including
animation-name
,animation-duration
,animation-timing-function
, and more, allowing for detailed control over animations.Using Keyframes: Keyframes define the styles at various points within the animation. By specifying multiple states, we create smooth transitions and intricate animations.
Animation Timing Functions: The
cubic-bezier
function used here customizes the pacing of the animation, making it feel more natural and less linear.
Customizing Loader Colors and Sizes
One of the best parts about this loader is its customizability. You can easily change the colors and sizes to fit your project's aesthetic.
Changing Background Colors: Adjust the
background
property of each.loader
class to match your desired color scheme.Adjusting Dimensions and Positions: Modify the
width
,height
,margin-left
, andmargin-top
properties within the keyframes and initial styles to change the loader's appearance.
Responsive Design Considerations
Ensuring your loader works well on all devices is crucial. Test your loader on various screen sizes to make sure it looks good everywhere.
Ensuring Loaders Work on All Devices: Use responsive design principles and test your loaders on different devices to ensure compatibility.
Testing Responsiveness: Utilize browser developer tools to simulate different screen sizes and orientations.
Debugging Common Issues
Sometimes, things don't go as planned. Here are a couple of common issues and how to fix them.
Handling Alignment Problems: Ensure that the
position
,top
,left
, andtransform
properties are correctly set to center the loaders.Fixing Animation Glitches: Check the
animation
properties and keyframes for any typos or logical errors that could disrupt the animation flow.
Enhancing with Additional Features
Want to take your loader to the next level? Here are a few enhancements you can try.
Adding More Loaders: Add additional
.loader
divs and customize their styles and animations for more complexity.Customizing Animations Further: Experiment with different
keyframes
andanimation-timing-function
values to create unique effects.
Optimizing Performance
Performance is key to a good user experience. Here are some tips to optimize your loader.
Minimizing CSS and HTML: Reduce the size of your CSS and HTML files to improve load times.
Improving Load Times: Optimize images and other resources to ensure your page loads quickly, even with animations.
Deploying the Loader
Finally, it's time to integrate your loader into your web projects and share it with the world.
Integrating into Web Projects: Copy and paste the HTML and CSS into your project's files.
Hosting and Sharing: Use platforms like GitHub or CodePen to host your project and share the link with others.
Conclusion
Creating an animated multi-device loader is a fantastic way to enhance your web projects and improve user experience. By following this guide, you’ve learned how to set up the HTML structure, style elements with CSS, and add engaging animations. Now it's your turn to experiment and make it your own!
FAQs
What is the purpose of a loader?
- Loaders provide visual feedback to users, indicating that content is being loaded in the background.
Can I use this loader in any web project?
- Yes, this loader can be easily integrated into any web project by copying the HTML and CSS.
How can I customize the loader’s appearance?
- You can change the colors, sizes, and animations by modifying the CSS properties.
Why is responsive design important for loaders?
- Responsive design ensures that loaders look good on all devices, providing a consistent user experience.
What tools can I use to test my loader’s responsiveness?
- Use browser developer tools to simulate different screen sizes and orientations, ensuring your loader works well across various devices.
Subscribe to my newsletter
Read articles from Aarzoo Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
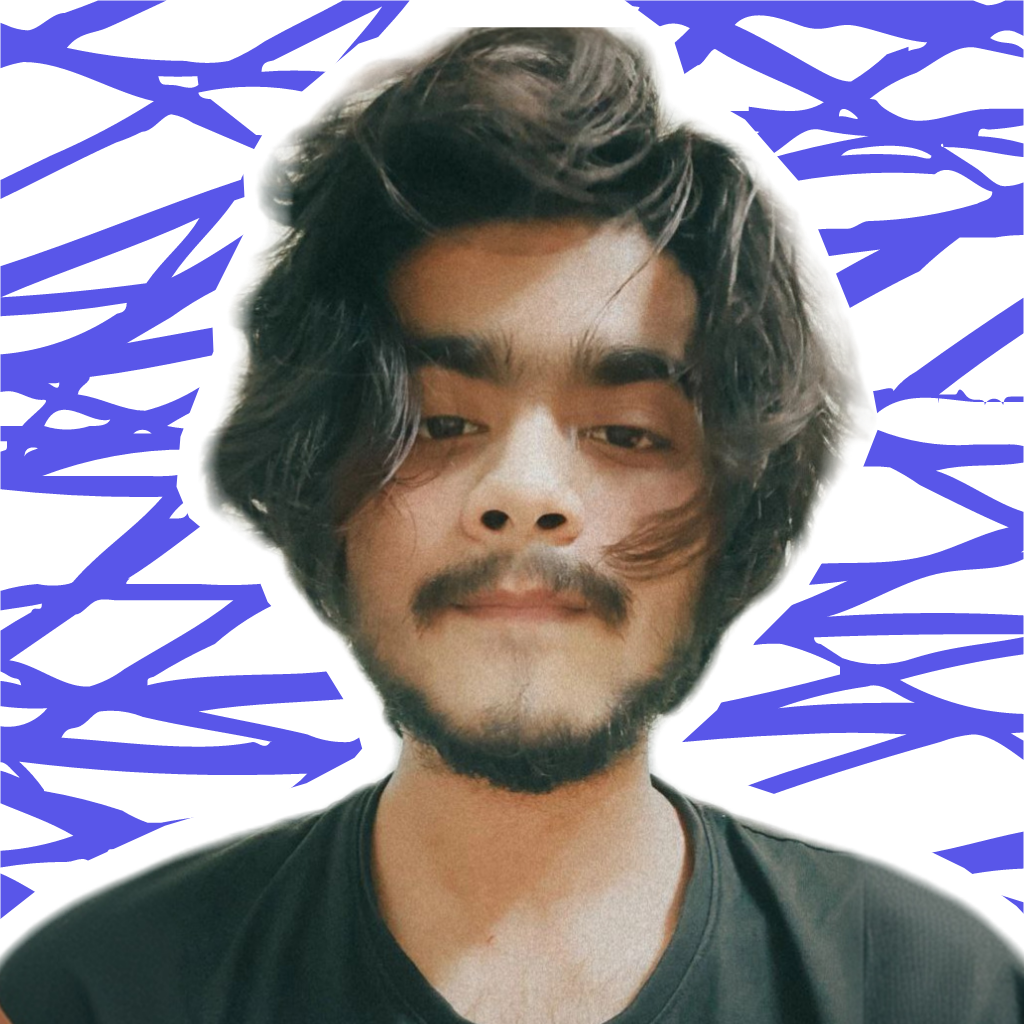
Aarzoo Islam
Aarzoo Islam
Founder of AroNus 🚀 | Full-stack web developer 👨🏻💻 | Sharing web development tips and showcasing projects 📂 | Transforming ideas into digital realities 🌟