Title: Writing and Implementing Custom Form Validator in Laravel 11

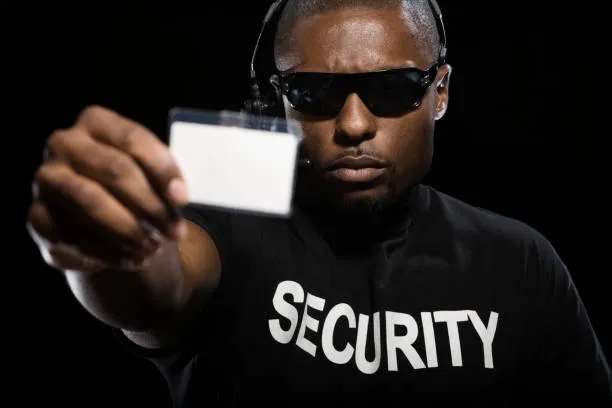
A crucial part of developing websites is validation, which makes sure that information submitted on forms satisfies requirements before it is approved. Strong form validation features are offered by the well-known PHP framework Laravel, including the option to design unique validation rules that are suited to particular needs. We'll go through how to create and use a custom form validator in Laravel 11 in this article. To be more precise, we will design a custom rule for Ncell and NTC, the two main mobile service providers in Nepal, to validate phone numbers.
Step 1: Creating the Custom Rule The first step is to generate a new custom rule using Laravel's Artisan command-line tool. Open your terminal and run the following command:
php artisan make:rule NcellNtcContactNumber
This command will generate a new rule file named NcellNtcContactNumber.php
in the app/Rules
directory.
Step 2: Adding Validation Logic to the Rule Open the newly created rule file (app/Rules/NcellNtcContactNumber.php
) in your code editor. This file contains a skeleton for the custom validation rule. Replace its contents with the following code:
<?php
namespace App\Rules;
use Closure;
use Illuminate\Contracts\Validation\Rule;
class NcellNtcContactNumber implements Rule
{
/**
* Determine if the validation rule passes.
*
* @param string $attribute
* @param mixed $value
* @return bool
*/
public function passes($attribute, $value)
{
// Define the regular expression pattern for Ncell and NTC contact numbers
$pattern = '/^(980\d{7}|981\d{7}|982\d{7}|984\d{7}|985\d{7}|986\d{7})$/';
// Check if the contact number matches the pattern
return preg_match($pattern, $value);
}
/**
* Get the validation error message.
*
* @return string
*/
public function message()
{
return 'The :attribute must be a valid Ncell or NTC contact number.';
}
}
Step 3: Using the Custom Rule in Controller Now, you can use this custom rule in your controller's validation logic. Open your desired controller file and add the following code snippet:
use App\Rules\NcellNtcContactNumber;
$request->validate([
'full_name' => 'required|min:2',
'address' => 'required|min:3',
'dob' => 'required',
'email' => 'required|email',
'contact_number' => ['required', 'min:10', 'numeric', new NcellNtcContactNumber],
'gender' => 'required',
'roleSelect' => 'required',
'salary' => 'required|numeric',
'is_teacher' => 'bool'
]);
Step 4: Displaying Validation Errors To display validation errors in your Blade view file, add the following code snippet within your form:
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
This code will loop through any validation errors and display them in a formatted list.
That's it! You've successfully created and implemented a custom form validator in Laravel 11 for validating Ncell and NTC contact numbers. Custom validators provide flexibility and allow you to enforce specific validation rules tailored to your application's needs. Experiment with different validation rules and enhance the user experience of your Laravel applications.
Subscribe to my newsletter
Read articles from Prakash Tajpuriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Prakash Tajpuriya
Prakash Tajpuriya
Web Developer